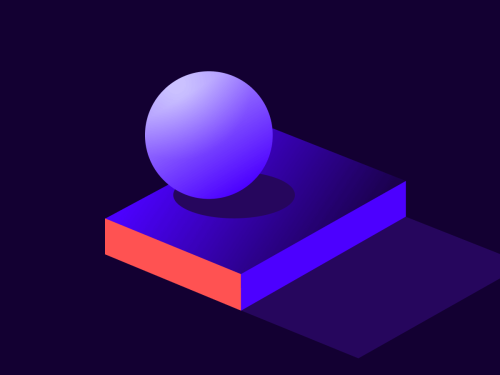
Common API Tasksđ: Use regex validation in envelopesâ tabs
Learn to use regular expressions (regex) to provide Docusign with data templates that enable you to validate the information you get from document tabs.
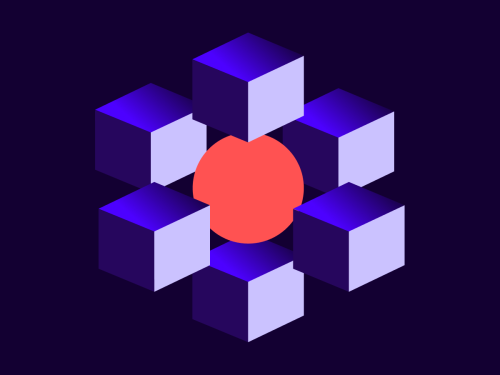
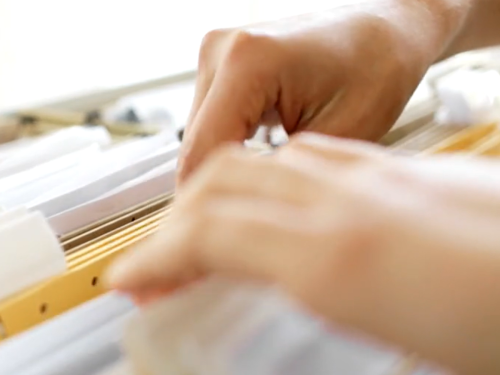
Welcome to a splendiferous new edition of the CATđ (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
We have all probably heard the expression âGarbage in, garbage outâ from time to time. It refers to the fact that what we do with our data is only as good as the data we receive to begin with. If we have poor-quality information coming into our software, we cannot expect high-quality results. This is why we need validation of the input coming from end users. Humans, unlike machines, make mistakes, and thatâs where data validation comes in. For us it means validation of the input fields, or tabs, that the recipients fill in when they act on envelopes coming from Docusign. There are various ways to validate data inside tabs, and in this blog post Iâm going to show how to use regular expressions (or regex) to enforce patterns in text tabs.Â
Regular expressions are a common tool that software engineers use to find patterns in text. Theyâre very useful for simple types of validations where you have a consistent pattern for the data. For example, if you want a ten-digit phone number and the pattern you want your customers to use is ###-###-#### (three groupings with dashes in between) you can use _^[1-9]\d{2}-\d{3}-\d{4}_
as your regular expression. That expression is true for phone numbers that comply with your requirements and false otherwise. Docusign will evaluate the expression in real time (client-side) when recipients are interacting with the document (that is, when signers sign the document) and prevent them from completing and finalizing the envelope if they donât comply with the pattern. You can also provide a validation message that will appear whenever the data is invalid. This message should be informative to help the users understand what they did wrong and fix the information.
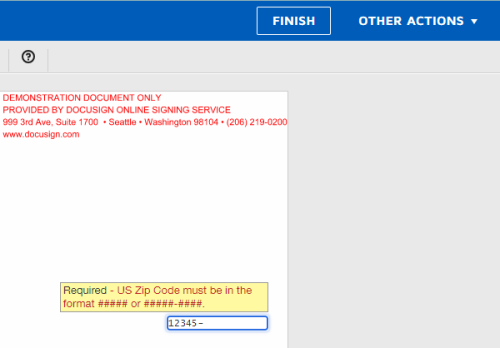
In this example, I will show you code snippets to use for a text tab that validates the data for US ZIP code. In the US ZIP codes have two formats, either a five-digit number, or two numbers, the first five digits and the last four digits separated by a dash. Therefore, the regex pattern we will use is _^[0-9]{5}(-[0-9]{4})?$_
which allows for both options. The code snippet I have below is not a full working code, but it includes the recipients
and tabs
objects that are part of the envelopeDefinition
object. To use this code, youâll have to first get an access code using OAuth, build a full envelope that includes one or more documents (or you can use templates), send it, and then either have users sign remotely or make another API call for embedded signing.
Note also that I use fixed positioning to place the tab in a specific location on the first page of the document with documentId
â1â. This may not work in your case and you may need to use anchor tags (or Auto-Place tags) to ensure the text tab is positioned correctly.
C#
// Create a Signer object
Signer signer1 = new Signer
{
Email = "inbar.gazit@docusign.com",
Name = "Inbar Gazit",
RecipientId = "1",
};
// Create a Text Tab
Text textTab = new Text
{
DocumentId = "1",
PageNumber = "1",
XPosition = "500",
YPosition = "200",
Width = "100",
ValidationMessage = "US Zip Code must be in the format ##### or #####-####.",
ValidationPattern = "^[0-9]{5}(-[0-9]{4})?$",
};
// Tabs are set per recipient / signer
Tabs signer1Tabs = new Tabs
{
TextTabs = new List<text> { textTab },
};
signer1.Tabs = signer1Tabs;
// Add the recipient to the envelope object
Recipients recipients = new Recipients
{
Signers = new List<signer> { signer1 },
};
envelopeDefinition.Recipients = recipients;
</signer></text>
Java
// Create a Signer object
Signer signer1 = new Signer();
signer1.setEmail("inbar.gazit@docusign.com");
signer1.setName("Inbar Gazit");
signer1.setRecipientId("1");
// Create a Text Tab
Text textTab = new Text();
textTab.setDocumentId("1");
textTab.setPageNumber("1");
textTab.setXPosition("500");
textTab.setYPosition("200");
textTab.setWidth("100");
textTab.setValidationMessage("US Zip Code must be in the format ##### or #####-####.");
textTab.setValidationPattern("^[0-9]{5}(-[0-9]{4})?$");
// Tabs are set per recipient / signer
Tabs signer1Tabs = new Tabs();
signer1Tabs.setTextTabs(Arrays.asList(textTab));
signer1.setTabs(signer1Tabs);
// Add the recipient to the envelope object
Recipients recipients = new Recipients();
recipients.setSigners(Arrays.asList(signer1));
envelopeDefinition.setRecipients(recipients);
Node.js
// Create a Signer object
let signer1 = docusign.Signer.constructFromObject({
'email': 'inbar.gazit@docusign.com',
'name': 'Inbar Gazit',
'recipientId': '1',
)};
// Create a Text Tab
let textTab = docusign.Text.constructFromObject({
'documentId': '1',
'pageNumber': '1',
'xPosition': '500',
'yPosition': '200',
'width' = '100',
'validationMessage': 'US Zip Code must be in the format ##### or #####-####.',
'validationPattern': '^[0-9]{5}(-[0-9]{4})?$',
});
// Tabs are set per recipient / signer
let signer1Tabs = new docusign.Tabs()
signer1Tabs.textTabs = [textTab];
signer1.tabs = signer1Tabs;
// Add the recipient to the envelope object
let recipients = new docusign.Recipients()
recipients.signers = [signer1];
envelopeDefinition.recipients = recipients;
PHP
# Create a Signer object
$signer1 = new Docusign\eSign\Model\Signer();
$signer1->setEmail('inbar.gazit@docusign.com');
$signer1->setName('Inbar Gazit');
$signer1->setRecipientId('1');
# Create a Text Tab
$text_tab = new Docusign\eSign\Model\Text();
$text_tab->setDocumentId('1');
$text_tab->setPageNumber('1');
$text_tab->setXPosition('500');
$text_tab->setYPosition('200');
$text_tab->setWidth('100');
$text_tab->setValidationMessage('US Zip Code must be in the format ##### or #####-####.');
$text_tab->setValidationPattern('^[0-9]{5}(-[0-9]{4})?$');
# Tabs are set per recipient / signer
$signer1_tabs = new Docusign\eSign\Model\Tabs();
$signer1_tabs->setTextTabs([$text_tab]);
$signer1->setTabs($signer1_tabs);
# Add the recipient to the envelope object
$recipients = new Docusign\eSign\Model\Recipients();
$recipients->setSigners([$signer1]);
$envelope_definition->setRecipients($recipients);
Python
# Create a Signer object
signer1 = Signer()
signer1.email = 'inbar.gazit@docusign.com'
signer1.name = 'Inbar Gazit'
signer1._recipient_id = '1'
# Create a Text Tab
text_tab = Text()
text_tab.document_id = '1'
text_tab.page_number = '1'
text_tab.x_position = '500'
text_tab.y_position = '200'
text_tab.width = '100'
text_tab.validation_message = 'US Zip Code must be in the format ##### or #####-####.'
text_tab.validation_pattern = '^[0-9]{5}(-[0-9]{4})?'
# Tabs are per recipient / signer
signer1_tabs = Tabs()
signer1_tabs.text_tabs = [text_tab]
signer1.tabs = signer1_tabs
# Add the recipient to the envelope object
recipients = Recipients()
recipients.signers = [signer1]
envelope_definition.recipients = recipients
Ruby
# Create a Signer object
signer1 = DocuSign_eSign::Signer.new
signer1.email = 'inbar.gazit@docusign.com'
signer1.name = 'Inbar Gazit'
signer1._recipient_id = '1'
# Create a Text Tab
text_tab = DocuSign_eSign::Text.new
text_tab.document_id = '1'
text_tab.page_number = '1'
text_tab.x_position = '500'
text_tab.y_position = '200'
text_tab.width = '100'
text_tab.validation_message = 'US Zip Code must be in the format ##### or #####-####.'
text_tab.validation_pattern = '^[0-9]{5}(-[0-9]{4})?'
# Tabs are per recipient / signer
signer1_tabs = DocuSign_eSign::Tabs.new
signer1_tabs.text_tabs = [text_tab]
signer1.tabs = signer1_tabs
# Add the recipient to the envelope object
recipients = DocuSign_eSign::Recipients.new
recipients.signers = [signer1]
envelope_definition.recipients = recipients
Thatâs all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
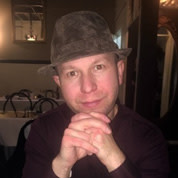
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
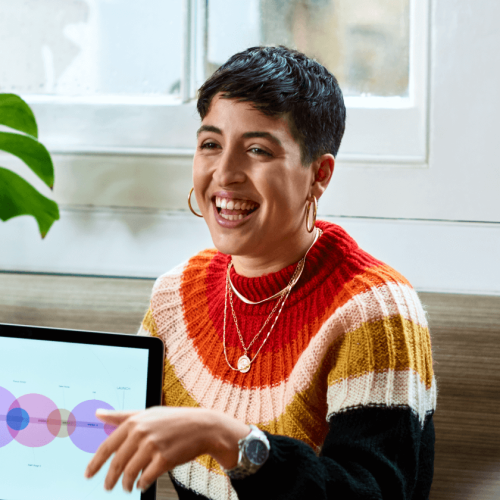