Common API Tasks🐈: Find a web form by name
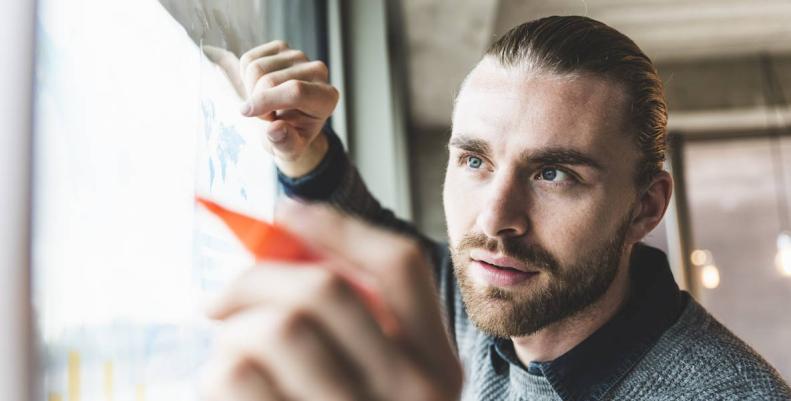
Welcome to a crackerjack new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the DocuSign Developer Blog.
Last month we announced the release of new Web Forms features and a new public API called the Web Forms API. So, I’m excited to write one of my CAT blogs about this topic. While you may be new to the DocuSign Web Forms feature, as you may imagine, this feature is not just super-useful, it has a variety of different use cases and capabilities. So, over time, your organization or your customers may create many web forms. Having an easy way to find a specific form is therefore an important capability. The DocuSign web app has a feature that lets you search for web forms. You can also do the same thing from your own integration using the Web Forms API.
Note that this blog post requires a different set of SDKs specifically for this new API, but we still support the same six coding languages you’re used to. So, here are the code snippets to find all the web forms that contain the word “cat” in their title.
For each web form returned by the code below, you will find general information about the form, such as its name, ID (GUID), date when it was created, and whether or not it’s published.
C#
// This is the developer account basePath
string basePath = "https://apps-d.docusign.com/api/webforms/v1.1/restapi";
var docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
FormManagementApi formManagementApi = new FormManagementApi(docuSignClient);
FormManagementApi.ListFormsOptions listFormsOptions = new FormManagementApi.ListFormsOptions();
listFormsOptions.search = "cat";
var filteredForms = formManagementApi.ListForms(accountId, listFormsOptions);
Java
// This is the developer account basePath
String basePath = "https://apps-d.docusign.com/api/webforms/v1.1/restapi";
ApiClient apiClient = new ApiClient(basePath);
// You will need to obtain an access token using your chosen authentication method
apiClient.addDefaultHeader("Authorization", "Bearer " + accessToken);
FormManagementApi formManagementApi = new FormManagementApi(apiClient);
var listFormsOption = formManagementApi.new ListFormsOptions();
listFormsOption.setSearch("cat");
var filteredForms = formManagementApi.listForms(accountId, listFormsOption);
Node.js
// This is the developer account basePath
let basePath = 'https://apps-d.docusign.com/api/webforms/v1.1/restapi';
let apiClient = new docusignWebForms.ApiClient();
apiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
apiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let formManagementApi = new docusignWebForms.FormManagementApi(apiClient);
let filteredForms = formManagementApi.listForms(args.accountId, { search: 'cat' });
PHP
# This is the developer account basePath
$base_path = 'https://apps-d.docusign.com/api/webforms/v1.1/restapi';
$config = new \DocuSign\eSign\Model\Configuration();
$config->setHost($base_path);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' . $access_token);
$api_client = new \DocuSign\eSign\client\ApiClient($config);
$form_management_api = new \DocuSign\WebForms\Api\FormManagementApi($api_client);
$list_forms_options = new FormManagementApi\ListFormsOptions();
$list_forms_options->setSearch('cat');
$filtered_forms = $form_management_api->listForms($account_id, $list_forms_options);
Python
# This is the developer account basePath
base_path = 'https://apps-d.docusign.com/api/webforms/v1.1/restapi'
api_client = ApiClient()
api_client.host = args[base_path]
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header(header_name='Authorization', header_value=f'Bearer {access_token}')
form_management_api = FormManagementApi(api_client)
filtered_forms = form_management_api.list_forms(account_id, 'cat')
Ruby
# This is the developer account basePath
base_path = 'https://apps-d.docusign.com/api/webforms/v1.1/restapi'
configuration = DocuSign_WebForms::Configuration.new
api_client = DocuSign_WebForms::ApiClient.new(configuration)
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', "Bearer #{access_token}")
form_management_api = DocuSign_WebForms::FormManagementApi.new(api_client)
options = DocuSign_WebForms::ListFormsOptions.new
options.search = args['cat']
form_management_api.list_forms(account_id, options)
That’s all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time…