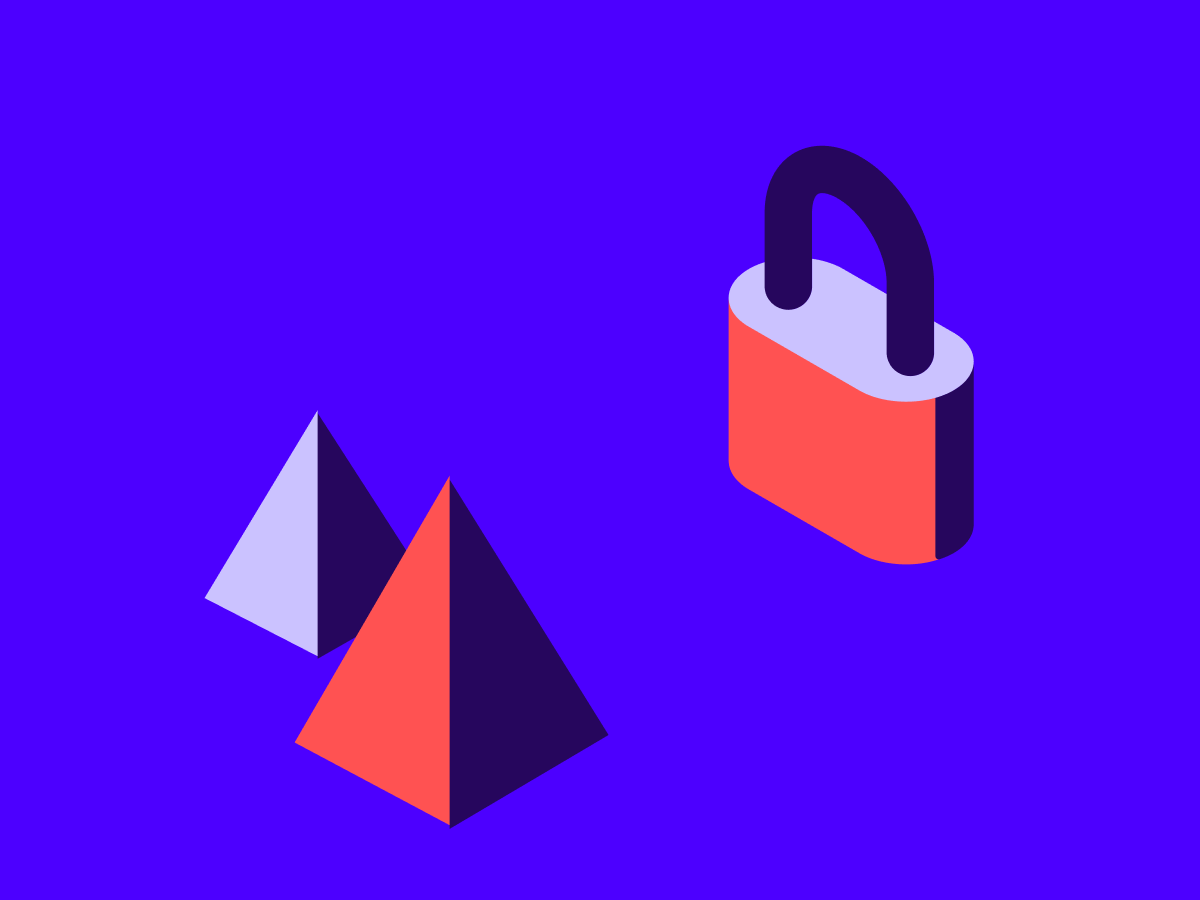
Common API Tasks🐈: Sign in Each Location
Developers can add security to their envelopes by using the API to require signers to draw their signature every place they sign a document.
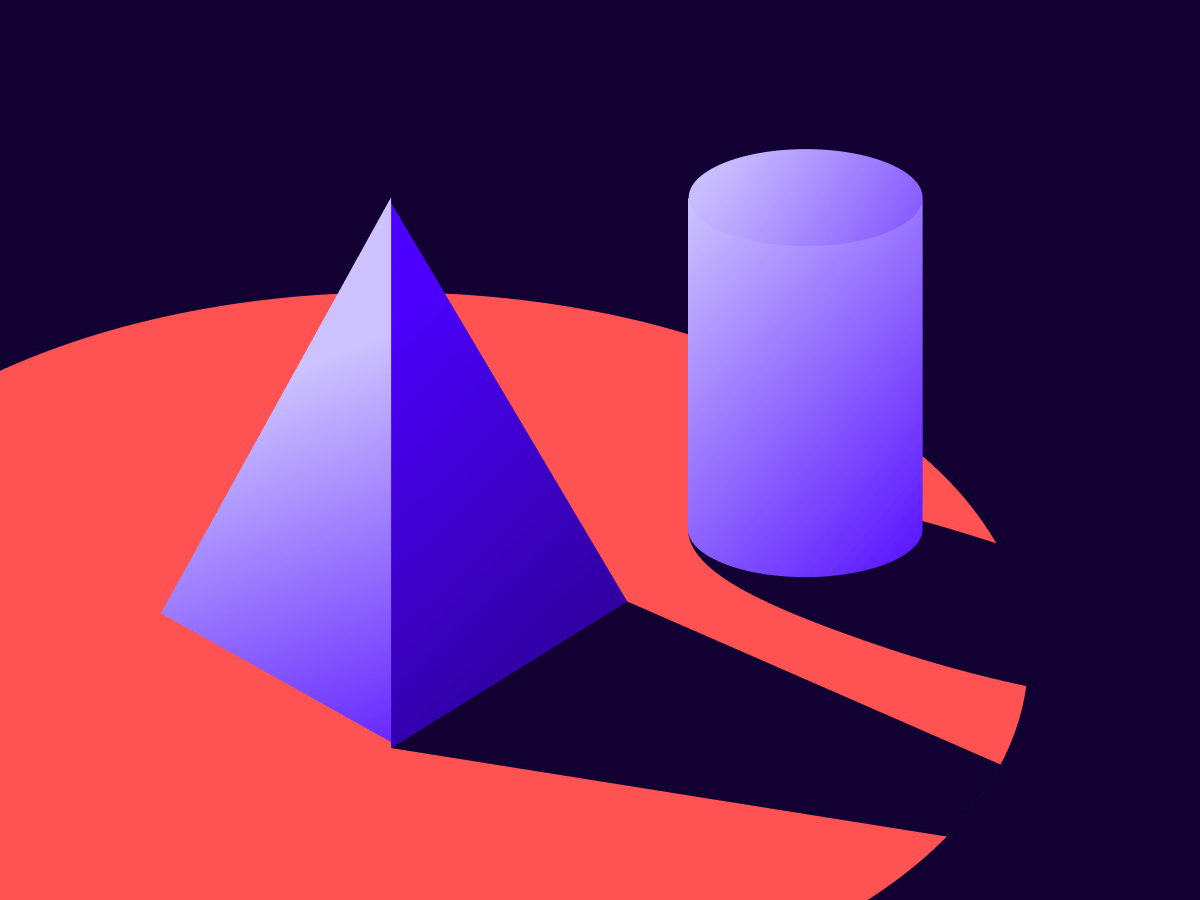
Welcome to another edition of the CAT (Common API Tasks) blog. This blog series is all about giving you useful information about using the Docusign APIs. In each post I provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign developer blog.
For this one I’d like to talk about a feature that often helps me when I discuss eSignature with people who may still be using paper-based processes, and have some resistance to switching over. In these discussions (okay, arguments) I often hear that clicking a mouse button or tapping your screen does not have the same level of obligation as signing your name on paper. They argue that various research shows that if we click, say, 10 times, we eventually don’t even notice what we click, whereas if we have to physically sign our name, that’s a higher level of commitment. They usually concede the argument when they hear of the “Sign in Each Location” feature.
The Sign in Each Location feature is very simple to explain. If you enable it for a specific signer, that signer will have to draw their signature for each and every signing element (either signature tabs or initial tabs). Drawing the signature using a mouse may not be ideal, so using a phone or a tablet is recommended (especially if you know your signer has a stylus for their device).
This feature is available from the Docusign web application as long as you have it enabled for your account. For developer accounts (demo), if your account was created prior to 2021, you need to contact our support team to enable this feature for you. It may be easier and quicker to simply create a new (and free!) developer account.
First, I’ll quickly show you how to use this feature from the web app. When you add recipients to your envelope, you can select the CUSTOMIZE button and pick Advanced Settings from the menu.
Once you do that you get a new dropdown called Signing Settings that only has one option (other than None). Then you would select Draw a new signature for each signature or initial field. That’s it. Your signer (or other recipient types) would have to draw their signature now for each tab you add for them (only SignHereTab and InitialTab).
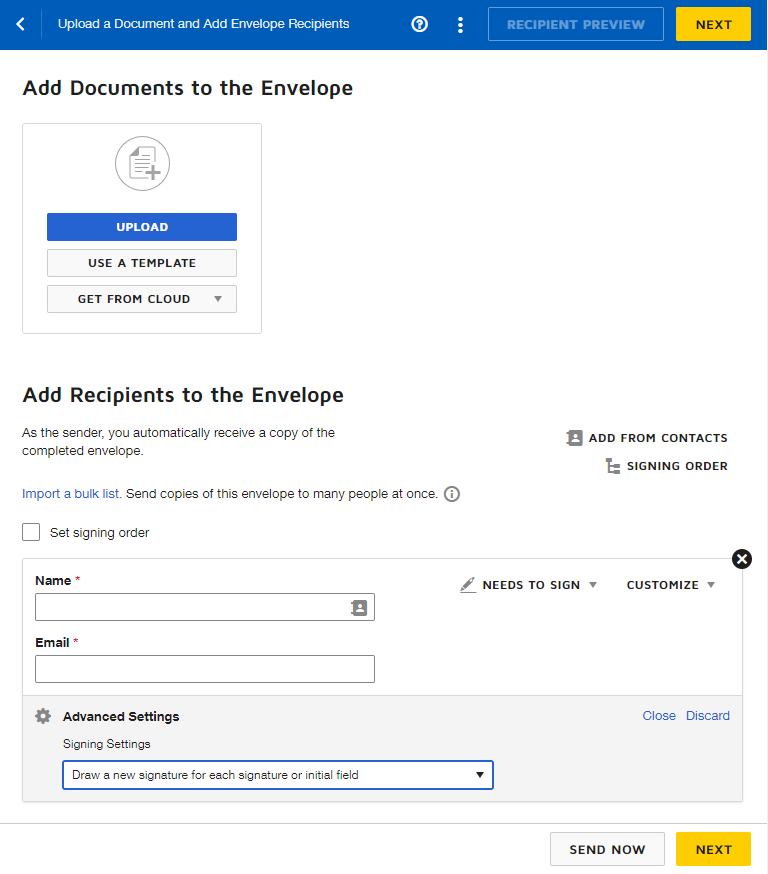
Now, we get to the fun part. How would you do that in your application? As usual, I’m going to provide six code snippets. The code here shows you how to add a recipient that has two tabs and has to sign in each location (meaning, draw their signature twice).
Note that this is not a complete code example. You’ll need to get an access token, add at least one document, set the envelope message and status, and maybe do a few other things if you want this to send an envelope for your integration.
C#
Signer signer1 = new Signer
{
Email = "test@sample.com",
Name = "some name",
ClientUserId = "1",
RecipientId = "1",
SignInEachLocation = "true", // Signer must draw their signature in each tab
};
SignHere signHere1 = new SignHere
{
XPosition = "200",
YPosition = "200",
DocumentId = "1",
PageNumber = "1"
};
SignHere signHere2 = new SignHere
{
XPosition = "100",
YPosition = "100",
DocumentId = "1",
PageNumber = "1"
};
Tabs signer1Tabs = new Tabs
{
SignHereTabs = new List { signHere1, signHere2 }
};
signer1.Tabs = signer1Tabs;
Recipients recipients = new Recipients
{
Signers = new List { signer1 }
};
envelopeDefinition.Recipients = recipients;
Java
Signer signer = new Signer();
signer.setEmail("test@sample.com");
signer.setName("some name");
signer.clientUserId("1");
signer.recipientId("1");
signer.setSignInEachLocation("true"); // Signer must draw their signature in each tab
SignHere signHere1 = new SignHere();
signHere1.setXPosition("200");
signHere1.setYPosition("200");
signHere1.setDocumentId("1");
signHere1.setPageNumber("1");
signHere2.setXPosition("100");
signHere2.setYPosition("100");
signHere2.setDocumentId("1");
signHere2.setPageNumber("1");
Tabs signer1Tabs = new Tabs();
SignHereTab[] signHereTabs = {signHere1, signHere2};
signer1Tabs.setSignHereTabs(Arrays.asList(signHereTabs));
signer.setTabs(signer1Tabs);
Recipients recipients = new Recipients();
recipients.setSigners(Arrays.asList(signer));
envelopeDefinition.setRecipients(recipients);
Node.js
let signer1 = docusign.Signer.constructFromObject({
email: 'test@sample.com',
name: 'some name',
clientUserId: '1',
recipientId: '1',
signInEachLocation: 'true' // Signer must draw their signature in each tab
});
let signHere1 = docusign.SignHere.constructFromObject({
yPosition: '200',
xPosition: '200',
documentId: '1',
pageNumber: '1'
});
let signHere2 = docusign.SignHere.constructFromObject({
yPosition: '100',
xPosition: '100',
documentId: '1',
pageNumber: '1'
});
let signer1Tabs = docusign.Tabs.constructFromObject({
signHereTabs: [signHere1, signHere2]});
signer1.tabs = signer1Tabs;
let recipients = docusign.Recipients.constructFromObject({
signers: [signer1]});
envelopeDefinition.recipients = recipients;
PHP
$signer = new Signer([
'email' => 'test@sample.com' ,
'name' => 'Some Name',
'recipient_id' => '1',
'routing_order' => '1',
'client_user_id' => '1',
'sign_in_each_location' => 'true' # Signer must draw their signature in each tab
]);
$sign_here1 = new SignHere([
'y_position' => '200', 'x_position' => '200',
‘document_id' => '1', 'page_number' => '1'
]);
$sign_here2 = new SignHere([
'y_position' => '100', 'x_position' => '100',
‘document_id' => '1', 'page_number' => '1'
]);
$signer->settabs(new Tabs(['sign_here_tabs' => [$sign_here1, $sign_here2]]));
$recipients = new Recipients(['signers' => [$signer]]);
$envelope_definition->setrecipients($recipients);
Python
signer = Signer(
email='test@sample.com',
name='some name',
recipient_id='1',
routing_order='1',
client_user_id='1',
sign_in_each_location='true' # Signer must draw their signature in each tab
)
sign_here1 = SignHere(
x_position='200',
y_position='200',
document_id = '1',
page_number = '1'
)
sign_here2 = SignHere(
x_position='100',
y_position='100',
document_id = '1',
page_number = '1'
)
signer.tabs = Tabs(sign_here_tabs=[sign_here1, sign_here2])
recipients=Recipients(signers=[signer])
envelope_definition.recipients = recipients
Ruby
signer1 = DocuSign_eSign::Signer.new ({
email: 'test@sample.com', name: 'some name',
clientUserId: '1', recipientId: '1',
signInEachLocation: 'true' # Signer must draw their signature in each tab
})
sign_here1 = DocuSign_eSign::SignHere.new
sign_here1.x_position = '200'
sign_here1.y_posiotion = '200'
sign_here1.document_id = '1'
sign_here1.page_number = '1'
sign_here2 = DocuSign_eSign::SignHere.new
sign_here2.x_position = '200'
sign_here2.y_posiotion = '200'
sign_here2.document_id = '1'
sign_here2.page_number = '1'
tabs = DocuSign_eSign::Tabs.new
tabs.sign_here_tabs = [sign_here1, sign_here2]
signer1.tabs = tabs
recipients = DocuSign_eSign::Recipients.new
recipients.signers = [signer1]
envelope_definition.recipients = recipients
I hope you found this useful. As usual, if you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
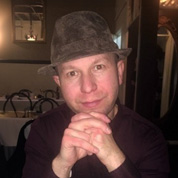
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Discover what's new with Docusign IAM or start with eSignature for free
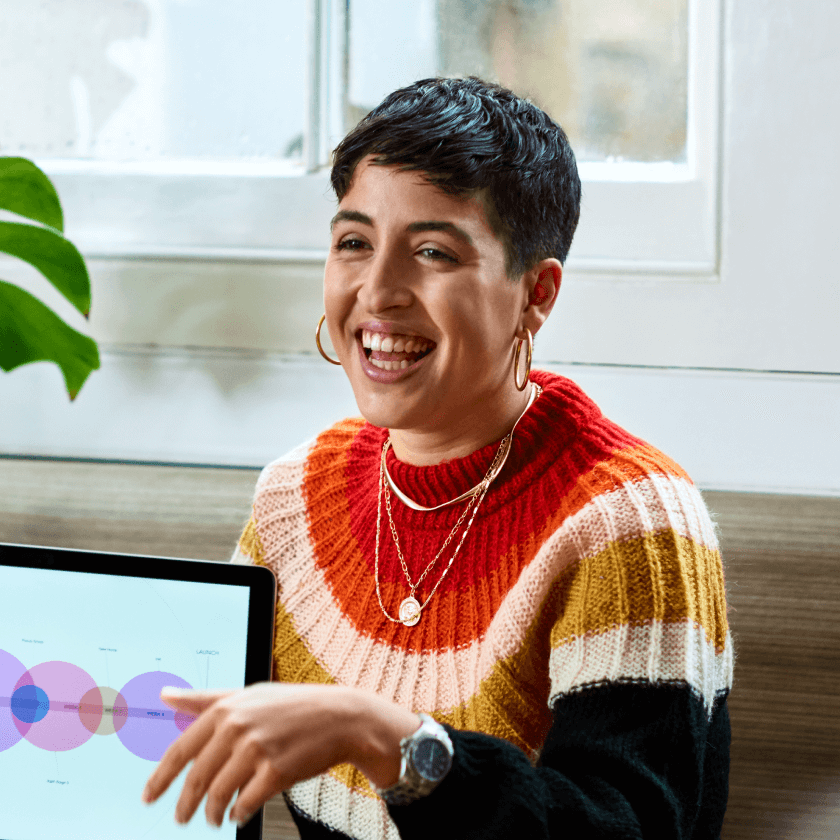