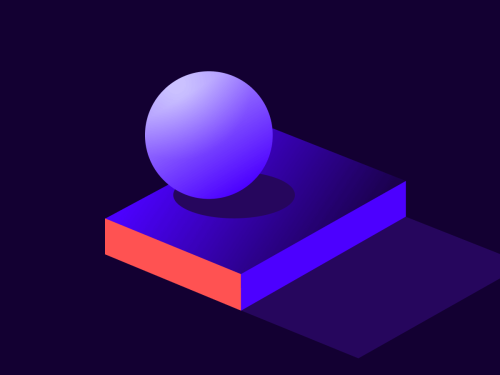
Common API Tasks🐈: Sharing templates
Learn how to use the Docusign eSignature REST API to share templates with other users of the Docusign account
Welcome to another post of the Common API Tasks blog series for developers! This blog series is focused on simple, yet useful pieces of functionality that can be accomplished using one of the Docusign APIs. In past posts I helped you with things like how to void an envelope or how to retrieve tab data. In my last post, I showed you how you can add more users to a Docusign account using the eSignature REST API. In this issue I’ll be focusing on how to share templates with other users of the Docusign account programmatically.
It may not be obvious, but by default, when a Docusign user creates a template, even if that user is an administrator, the template is only visible to that user. So when an account is used in an organization that has multiple users, this may lead to some confusion. It is very common for a business process to require a Docusign template to enable multiple users in the organization to fill out an expense report, for example. In fact, here in Docusign we use Docusign templates for almost anything. These templates are created by one user (perhaps the one your app was impersonating), but may need to be used by other users. You can easily do that using the Docusign web application, but what if you want your integration to create the template and share it with one or more users? That’s where I come in to help.
Let’s just jump in and show you how to code this in our usual languages:
C#
// You will need to obtain an access token using your chosen authentication flow
var config = new Configuration(new ApiClient(basePath));
config.AddDefaultHeader("Authorization", "Bearer " + accessToken);
var accountsApi = new AccountsApi(config);
var accountSharedAccess = new AccountSharedAccess();
accountSharedAccess.SharedAccess = new List<MemberSharedItems>();
var msi = new MemberSharedItems();
msi.Templates = new List<TemplateSharedItem>();
// Set up information about templates to be shared
var tsi = new TemplateSharedItem();
tsi.TemplateId = "43c25406-xxxx-xxxx-xxxx-1fd499ad7153";
tsi.Shared = "shared_to";
tsi.SharedUsers = new List<UserSharedItem>();
// Set up information about users who will share the templates
var usi = new UserSharedItem();
usi.User = new UserInfo { UserId = "5925476b-xxxx-xxxx-xxxx-b4546f9c467e"};
tsi.SharedUsers.Add(usi);
msi.Templates.Add(tsi);
accountSharedAccess.SharedAccess.Add(msi);
// Call the eSignature API
accountsApi.UpdateSharedAccess(accountId, accountSharedAccess, new AccountsApi.UpdateSharedAccessOptions ({ itemType = "templates" });
Java
// You will need to obtain an access token using your chosen authentication flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
AccountsApi accountsApi = new AccountsApi(config);
AccountSharedAccess accountSharedAccess = new AccountSharedAccess();
accountSharedAccess.setSharedAccess(new java.util.List<MemberSharedItems>());
MemberSharedItems msi = new MemberSharedItems();
msi.setTemplates(new java.util.List<TemplateSharedItem>());
// Set up information about templates to be shared
TemplateSharedItem tsi = new TemplateSharedItem();
tsi.setTemplateId("43c25406-xxxx-xxxx-xxxx-1fd499ad7153");
tsi.setShared("shared_to");
tsi.setSharedUsers(new java.util.List<UserSharedItem>());
// Set up information about users who will share the templates
UserSharedItem usi = new UserSharedItem();
usi.setUser(new UserInfo());
usi.getUser().setUserId("5925476b-xxxx-xxxx-xxxx-b4546f9c467e");
tsi.getSharedUsers().add(usi);
msi.getTemplates().add(tsi);
accountSharedAccess.getSharedAccess().add(msi);
// Call the eSignature API
AccountsApi.UpdateSharedAccessOptions options = new AccountsApi.UpdateSharedAccessOptions();
options.setItemType("templates");
accountsApi.updateSharedAccess(accountId, accountSharedAccess, options);
Node.js
// You will need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let accountsApi = new docusign.AccountsApi(dsApiClient);
let accountSharedAccess = new docusign.AccountSharedAccess();
accountSharedAccess.SharedAccess = [];
let msi = new docusign.MemberSharedItem();
msi.Templates = [];
// Set up information about templates to be shared
let tsi = new docusign.TemplateSharedItem();
tsi.templateId = '43c25406-xxxx-xxxx-xxxx-1fd499ad7153';
tsi.shared = 'shared_to';
tsi.sharedUsers = [];
// Set up information about users who will share the templates
let usi = new docusign.UserSharedItem();
usi.user = new docusign.UserInfo { UserId = '5925476b-xxxx-xxxx-xxxx-b4546f9c467e'};
tsi.sharedUsers.push(usi);
msi.templates.push(tsi);
accountSharedAccess.sharedAccess.push(msi);
// Call the eSignature API
let options = new docusign.AccountsApi.UpdateSharedAccessOptions();
options.itemType = 'templates';
accountsApi.updateSharedAccess(accountId, accountSharedAccess, options);
PHP
# You will need to obtain an access token using your chosen authentication flow
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$accounts_api = new \Docusign\eSign\Api\AccountsApi($config);
$account_shared_access = new \Docusign\eSign\Model\AccountSharedAccess();
$account_shared_access->setSharedAccess([]);
$msi = new \Docusign\eSign\Model\MemberSharedItems();
$msi->setTemplates([]);
# Set up information about templates to be shared
$tsi = new \Docusign\eSign\Model\TemplateSharedItem();
$tsi->setTemplateId('43c25406-xxxx-xxxx-xxxx-1fd499ad7153');
$tsi->setShared('shared_to');
$tsi->setSharedUsers([]);
# Set up information about users who will share the templates
$usi = new \Docusign\eSign\Model\UserSharedItem();
$usi->setUser(new \Docusign\eSign\Model\UserInfo());
$usi->getUser()->setUserId('5925476b-xxxx-xxxx-xxxx-b4546f9c467e');
array_push($tsi->getSharedUsers(), $usi);
array_push($msi->getTemplates(), $tsi);
array_push($account_shared_access->getSharedAccess(), $msi);
# Call the eSignature API
$options = new \Docusign\eSign\Api\AccountsApi\UpdateSharedAccessOptions();
$options->setItemType('templates');
$accountsApi->updateSharedAccess($account_id, $account_shared_access, $options);
Python
# You will need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
accounts_api = AccountsApi(config)
account_shared_access = AccountSharedAccess()
account_shared_access.shared_access = []
msi = MemberSharedItems()
msi.templates = []
# Set up information about templates to be shared
tsi = TemplateSharedItem()
tsi.template_id = '43c25406-xxxx-xxxx-xxxx-1fd499ad7153'
tsi.shared = 'shared_to'
tsi.shared_users = []
# Set up information about users who will share the templates
usi = UserSharedItem()
usi.user = UserInfo()
usi.user.user_id = '5925476b-xxxx-xxxx-xxxx-b4546f9c467e'
tsi.shared_users.append(usi)
msi.templates.append(tsi)
account_shared_access.shared_access.append(msi)
# Call the eSignature API
options = UpdateSharedAccessOptions()
options.item_type = 'templates'
accounts_api.update_shared_access(account_id, account_shared_access, options)
Ruby
# You will need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
accounts_api = DocuSign_eSign::AccountsApi.new api_client
account_shared_access = DocuSign_eSign::AccountSharedAccess.new
account_shared_access.shared_access = []
msi = DocuSign_eSign::MemberSharedItems.new
msi.templates = []
# Set up information about templates to be shared
tsi = DocuSign_eSign::TemplateSharedItem.new
tsi.template_id = '43c25406-xxxx-xxxx-xxxx-1fd499ad7153'
tsi.Shared = 'shared_to'
tsi.SharedUsers = []
# Set up information about users who will share the templates
usi = DocuSign_eSign::UserSharedItem.new
usi.user = DocuSign_eSign::UserInfo.new
usi.user.user_id = '5925476b-xxxx-xxxx-xxxx-b4546f9c467e'
tsi.shared_users.push(usi)
msi.templates.push(tsi)
account_shared_access.shared_access.push(msi)
# Call the eSignature API
options = DocuSign_eSign::UpdateSharedAccessOptions.new
options.item_type = 'templates'
accountsApi.update_shared_access(account_id, account_shared_access, options)
I hope you found this useful. As usual, if you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
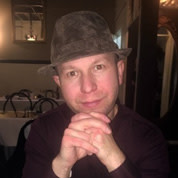
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
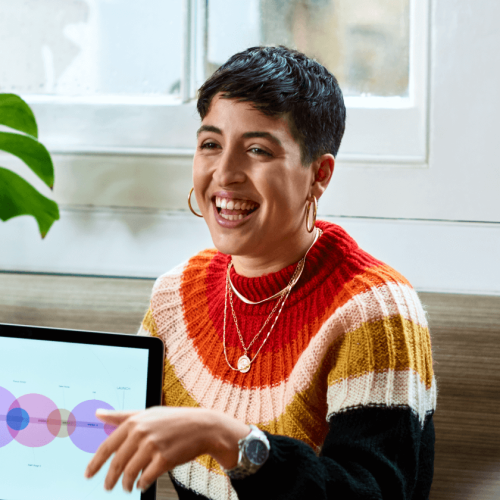