Common API Tasks🐈: Change your date/time format programmatically
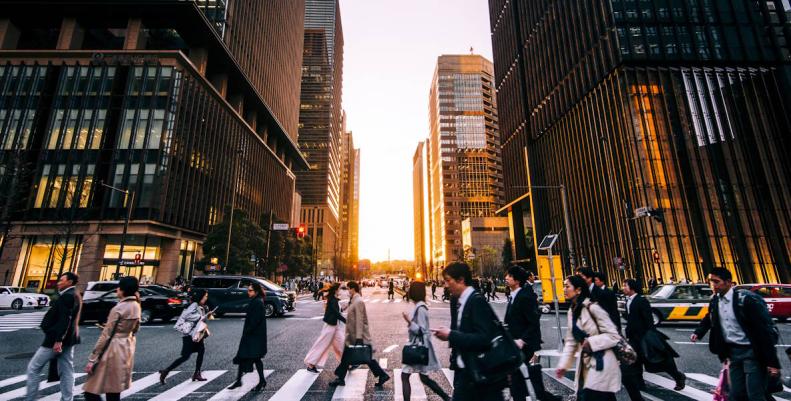
Welcome to an exciting new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the DocuSign Developer Blog.
In today’s edition I’m going to talk about dates and times. These show in various places in the DocuSign platform: for example, when you use a Date tab, which records the date and time the recipients signed a document, or when you look at the audit trail of an envelope to see all activity. Showing a date and time sounds simple, but it’s complicated by the fact that we live in a big world with different time zones as well as many different ways one can show the date and the time. For example, European countries typically have dates like 15/7/2023 where the day comes first and the month comes before the year, whereas in the US it would be more common to show it like this: 7/15/2023. The same date in Japan will be 2023/7/15 because in Japan you start with the year.
In DocuSign every user can decide how they want to show the date, and the decision is not controlled by the account administrator for all users. You do that by opening the My Preferences page from the profile menu, then select Regional Settings from the left nav, where you get to choose from a list of 17 different date/time formats as you can see here:
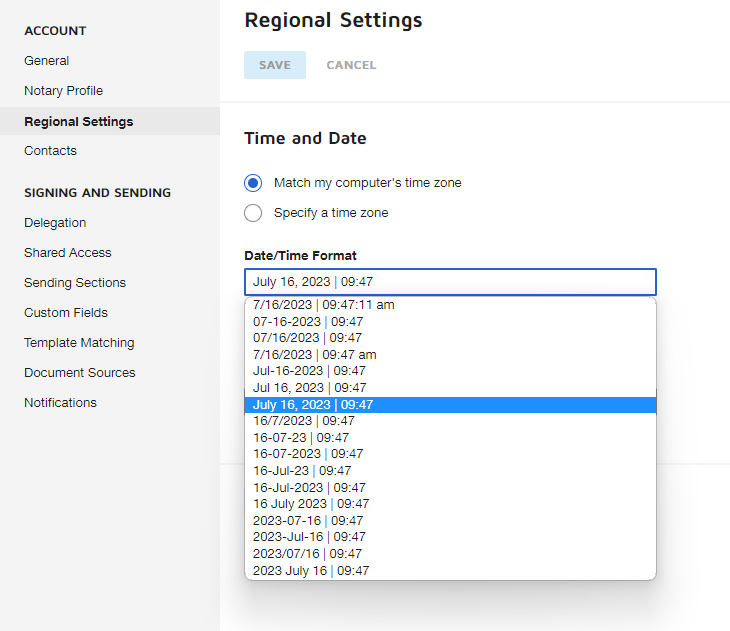
I’m sure our frequent readers can guess what comes next. Everything you can do from the web app can also be done using our APIs programmatically. This is what this blog series is all about!
Before you start, you need to know the user ID for the user you want to update. You can, as an administrator, update other users’ date/time format, or you can update your own. Now, the field used to update the date/time format for a specific user is called TimezoneMask, and it’s a string. The string uses lower- and uppercase letters to denote various aspects of the date and time. Here are some examples:
- yyyy - four-digit year
- MMMM - full month name
- MMM - three-letter month name
- MM - month number (1-12)
- HH - hour
- mm - minute
However, not every combination of the various letters is allowed. DocuSign only supports the following 17 date/time formats:
TimezoneMask string value | Example |
Default | 7/17/2023 | 10:47:58 am |
MM-dd-yyyy | HH:mm |
07-17-2023 | 10:47 |
MM/dd/yyyy | HH:mm |
07/17/2023 | 10:47 |
M/d/yyyy | hh:mm a |
7/17/2023 | 10:47 am |
MMM d, yyyy | HH:mm |
Jul-17-2023 | 10:47 |
MMMM d, yyyy | HH:mm |
July 17, 2023 | 10:47 |
d/M/yyyy | HH:mm |
17/7/2023 | 10:47 |
dd-MM-yy | HH:mm |
17-07-23 | 10:47 |
dd-MM-yyyy | HH:mm |
17-07-2023 | 10:47 |
dd-MMM-yy | HH:mm |
17-Jul-23 | 10:47 |
dd-MMM-yyyy | HH:mm |
17-Jul-2023 | 10:47 |
dd MMMM yyyy | HH:mm |
17 July 2023 | 10:47 |
yyyy-MM-dd | HH:mm |
2023-07-17 | 10:47 |
yyyy-MMM-dd | HH:mm |
2023-Jul-17 | 10:47 |
yyyy/MM/dd | HH:mm |
2023/07/17 | 10:47 |
yyyy MMMM d | HH:mm |
2023 July 17 | 10:47 |
Note: If you enter any other value into this string, you will not get an error message; the default format (top one) will be chosen in that case.
Now let’s look at some code, shall we?
C#
var docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
UsersApi usersApi = new UsersApi(docuSignClient);
UserSettingsInformation userSettingsInformation = new UserSettingsInformation();
userSettingsInformation.TimezoneMask = "MMMM d, yyyy | HH:mm";
usersApi.UpdateSettings(accountId, userId, userSettingsInformation);
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
UsersApi usersApi = new UsersApi(apiClient);
UserSettingsInformation userSettingsInformation = new UserSettingsInformation();
userSettingsInformation.setTimezoneMask("MMMM d, yyyy | HH:mm");
usersApi.updateSettings(accountId, userId, userSettingsInformation);
Node.js
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let usersApi = new docusign.UsersApi(dsApiClient);
let userSettingsInformation = new docusign.UserSettingsInformation();
userSettingsInformation.timezoneMask = 'MMMM d, yyyy | HH:mm';
usersApi.updateSettings(accountId, userId, userSettingsInformation);
PHP
$api_client = new \DocuSign\eSign\client\ApiClient($base_path);
$config = new \DocuSign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$users_api = new \DocuSign\eSign\Api\UsersApi($api_client);
$user_settings_information = new \DocuSign\eSign\Model\UserSettingsInformation();
$user_settings_information->setTimezoneMask('MMMM d, yyyy | HH:mm');
$users_api->updateSettings($account_id, $user_id, $user_settings_information);
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
users_api = UsersApi(api_client)
user_settings_information = UserSettingsInformation()
user_settings_information.timezoneMask = 'MMMM d, yyyy | HH:mm'
users_api.update_settings(account_id, user_id, user_settings_information)
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
user_api = DocuSign_eSign::UsersApi.new api_client
user_settings_information = DocuSign_eSign::UserSettingsInformation.new
user_settings_information.timezoneMask = 'MMMM d, yyyy | HH:mm'
users_api.update_settings(account_id, user_id, user_settings_information)
That’s all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...