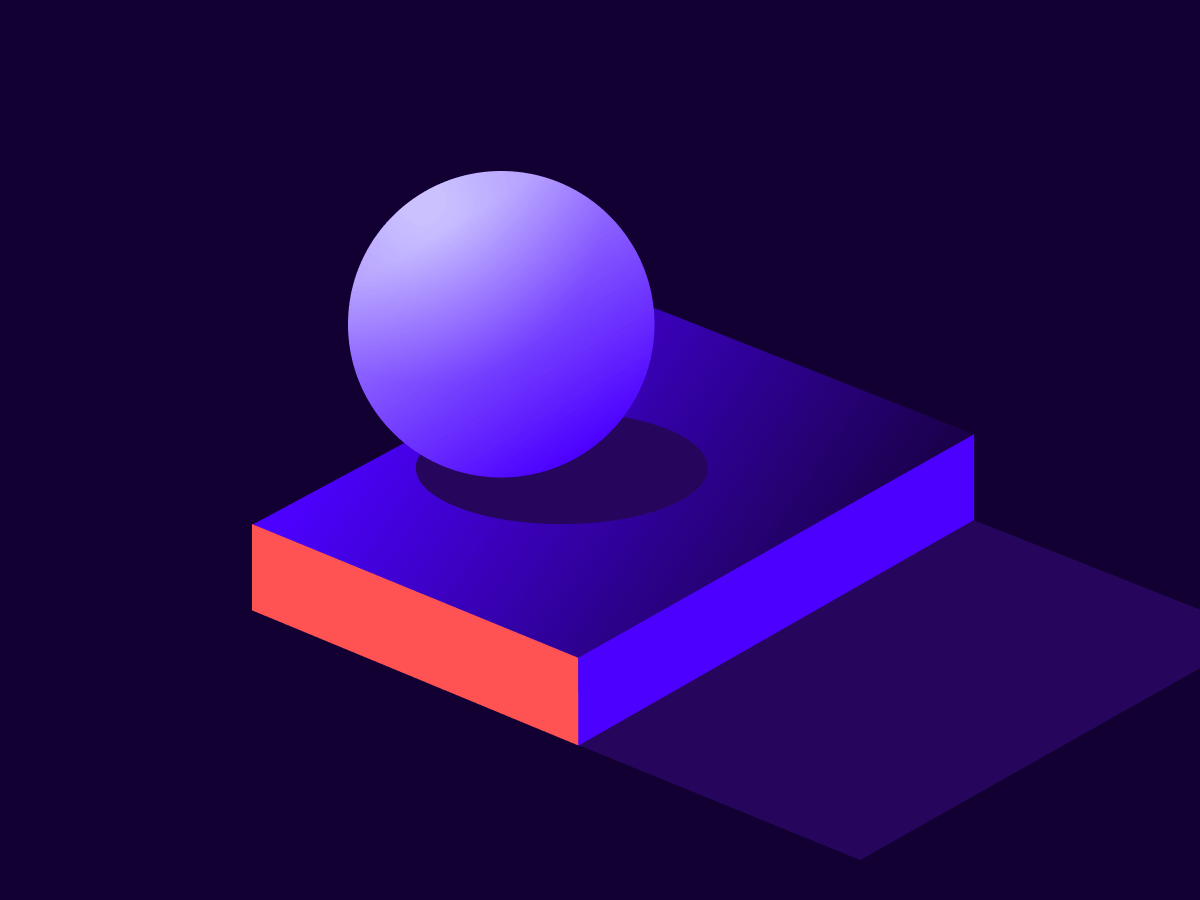
Common API Tasks🐈: Apply an existing template if it matches your envelope's documents
Template matching can help your customers streamline their signature processes. See how to do template matching in the eSignature REST API.
Table of contents
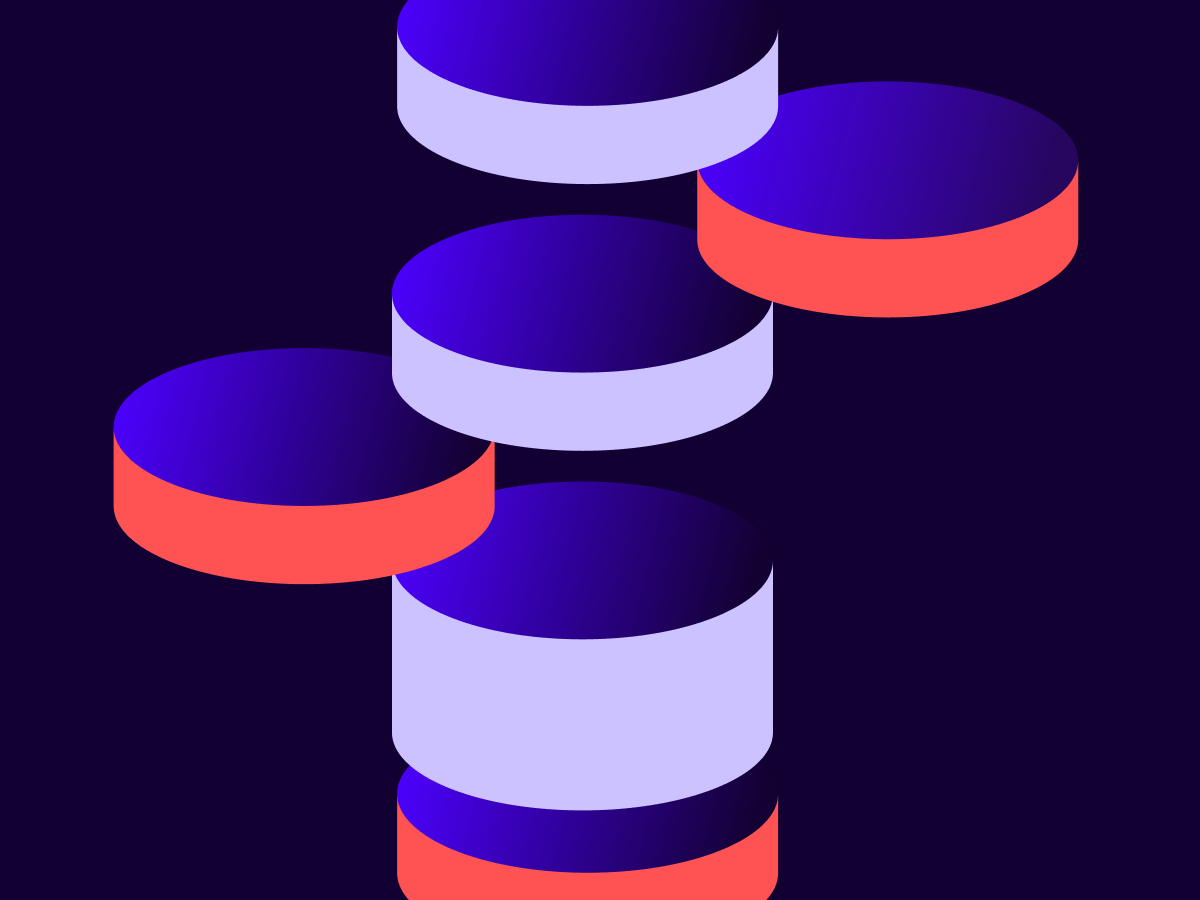
Welcome to an exceptional new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
Do you ever wish the day had 25 hours? Or even 30 hours; would be nice, wouldn’t it? All of us, our customers, everyone we know is super-busy and constantly looking for ways to save time. Today I’m going to show you a Docusign feature that does just that. I’m also going to show you how you can integrate this feature into your own applications when you use the Docusign eSignature REST API.
Template matching
Template matching is a feature that can help your users when they need to request signatures. To understand how this feature works, let’s start with basic concepts. Envelopes in Docusign are containers for documents, recipients, and tabs (also known as tags, fields, or signing elements). To help expedite common or repeating processes, Docusign provides eSignature templates. Templates are just like envelopes: they also contain documents, recipients, and tabs. The difference is that you cannot directly use a template to start gathering signatures. You have to first use it to create an envelope. Only envelopes can be signed or sent to recipients.
Now, users of your applications may not be aware of the existence of a template that can help them quickly finish their work. The template may have been created by a different user or may have been created a long time ago, and they did not realize such a template existed. The users may just think they’re doing a “one-off” activity and forgo using a template. They will start by creating an envelope, then adding one or more documents to it. They would then typically need to add the recipients, and finally the tabs. The way template matching can help them is by comparing the document(s) that the user has added to their envelopes to documents that are stored in existing templates (within the same Docusign account). The system can quickly compare all templates that are eligible for template matching (you can choose to exclude some templates from this practice for various reasons) to the document(s) in the newly created envelope. The system then tells the user if they find templates that match, which templates these are, what pages in the document(s) match, and to what percentage they match. (The comparison is done using visualizations of the documents, so they may not be identical, but we can calculate how similar they are.)
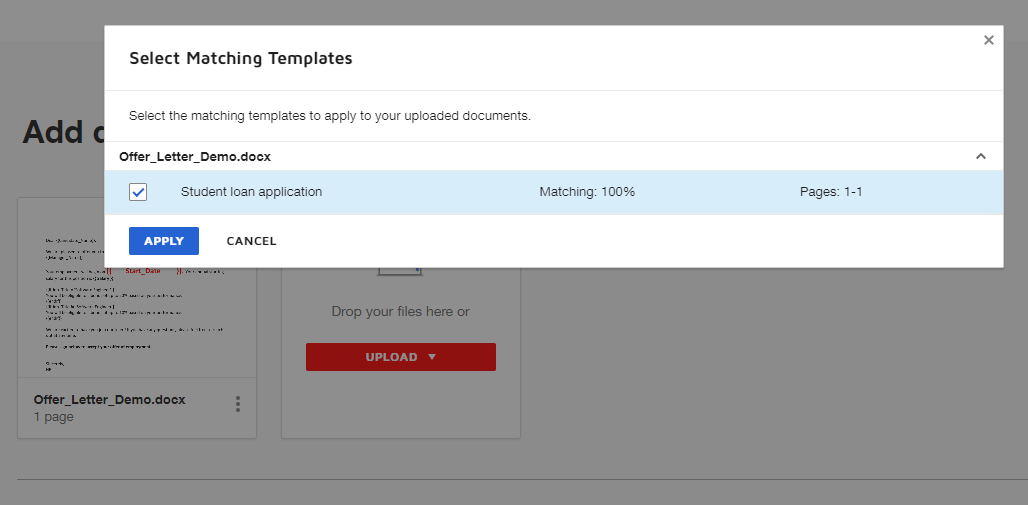
When users add a document to a new envelope, a pop-up dialog prompts them to choose a matching template.
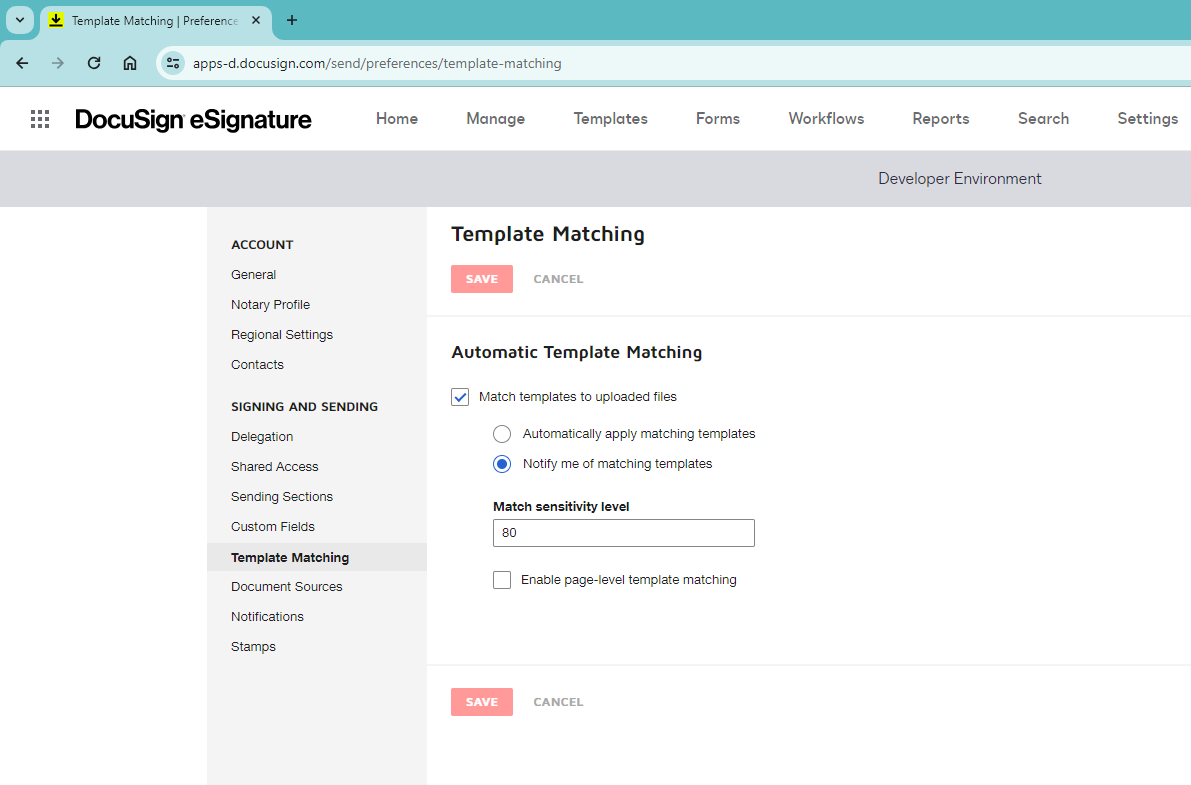
You can configure your own user’s template matching settings by selecting your account profile image, then selecting My Preferences.
This functionality is also very useful when you build an integration. If your integration lets users select documents to be used for eSignature, then you may be able similarly to use template matching in your integration to help improve the process for your customers.
The code snippets I provide in this blog post do the following: They assume you already created an envelope, and that the envelope is in draft state and already includes one or more documents. The code then makes an API call to list all templates that match this envelope. Note that this same API call can be used to find out which templates were used when the envelope was created (if it was created from a template), but when you add the ?include=matching URL parameter (or doing it from SDKs, as I do in this series), you will ensure that the system checks for template matching. The code then proceeds to check that you got templates back, that you have at least one template, and finally that the template is at least a 90% match for the documents in your envelope. If all these conditions are met, then the code then makes another API call to apply this template to the envelope. In your own application, of course, you can decide what to do with the information, and how to proceed according to your own business requirements. Here are the code snippets for this:
C#
var docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
var envelopesApi = new EnvelopesApi(docuSignClient);
EnvelopesApi.ListTemplatesOptions options = new EnvelopesApi.ListTemplatesOptions();
options.include = "matching";
TemplateInformation templateInformation = envelopesApi.ListTemplates(accountId, envelopeId, options);
if (templateInformation.Templates != null)
{
if (templateInformation.Templates.Count > 0)
{
if (int.Parse(templateInformation.Templates[0].TemplateMatch.MatchPercentage) > 90)
{
var documentTemplateList = new DocumentTemplateList();
documentTemplateList.DocumentTemplates = new List<documenttemplate>();
documentTemplateList.DocumentTemplates.Add(new DocumentTemplate() { TemplateId = templateInformation.Templates[0].TemplateId });
envelopesApi.ApplyTemplate(accountId, envelopeId, documentTemplateList);
}
}
}
</documenttemplate>
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
EnvelopesApi.ListTemplatesOptions options = envelopesApi.new ListTemplatesOptions();
options.setInclude("matching");
TemplateInformation templateInformation = envelopesApi.ListTemplates(accountId, envelopeId, options);
if (templateInformation.getTemplates() != null)
{
if (templateInformation.getTemplates.size() > 0)
{
if (Integer.parseInt(templateInformation.getTemplates().get(0).getTemplateMatch().getMatchPercentage()) > 90)
{
DocumentTemplateList documentTemplateList = new DocumentTemplateList();
documentTemplateList.setDocumentTemplates(new List<DocumentTemplate>());
DocumentTemplate documentTemplate = new DocumentTemplate();
documentTemplate.setTemplateId(templateInformation.getTemplates().get(0).getTemplateId());
documentTemplateList.getDocumentTemplates().Add(documentTemplate);
envelopesApi.ApplyTemplate(accountId, envelopeId, documentTemplateList);
}
}
}
Node.js
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
let options = new docusign.ListTemplatesOptions();
options.include = 'matching';
let templateInformation = envelopesApi.listTemplates(accountId, envelopeId, options);
if (templateInformation.templates != null)
{
if (templateInformation.templates.length > 0)
{
if (templateInformation.templates[0].templateMatch.matchPercentage > 90)
{
let documentTemplateList = new docusign.DocumentTemplateList();
let documentTemplate = new docusign.DocumentTemplate();
documentTemplate.templateId = templateInformation.templates[0].templateId;
documentTemplateList.documentTemplates.push(documentTemplate);
envelopesApi.applyTemplate(accountId, envelopeId, documentTemplateList);
}
}
}
PHP
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \Docusign\eSign\Api\EnvelopesApi($api_client);
$options = new \Docusign\eSign\Model\ListTemplatesOptions();
$options->setInclude('matching');
$template_information = $envelopes_api->ListTemplates($account_id, $envelope_Id, $options);
if ($template_information->getTemplates() != NULL)
{
if (count($template_information->getTemplates()) > 0)
{
if ($template_information->getTemplates()[0]->getTemplateMatch()->getMatchPercentage()) > 90)
{
$document_template_list = new \Docusign\eSign\Model\DocumentTemplateList();
$document_template = new \Docusign\eSign\Model\DocumentTemplate();
$document_template->setTemplateId($template_information->getTemplates()[0]->getTemplateId());
array_push($document_template_list->getDocumentTemplates(), $document_template);
$envelopes_api->ApplyTemplate($account_id, $envelope_id, $document_template_list);
}
}
}
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
options = ListTemplatesOptions()
options.include = 'matching'
template_information = envelopes_api.list_templates(account_id, envelope_id, options)
if not template_information.templates is None:
if len(template_information.templates) > 0:
if template_information.templates[0].template_match.match_percentage > 90:
document_template_list = DocumentTemplateList()
document_template = DocumentTemplate()
document_template.template_id = template_information.templates[0].template_id
document_template_list.document_templates.append(document_template)
envelopes_api.apply_template(account_id, envelope_id, document_template_list)
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
options = DocuSign_eSign::ListTemplatesOptions.new
options.include = 'matching'
template_information = envelopes_api.list_templates(account_id, envelope_id, options)
if template_information.templates
if template_information.templates.length() > 0
if template_information.templates[0].template_match.match_percentage > 90
document_template_list = DocuSign_eSign::DocumentTemplateList.new
document_template = DocuSign_eSign::DocumentTemplate.new
document_template.template_id = template_information.templates[0].template_id
document_template_list.document_templates.append(document_template)
envelopes_api.apply_template(account_id, envelope_id, document_template_list)
Additional resources
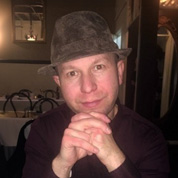
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
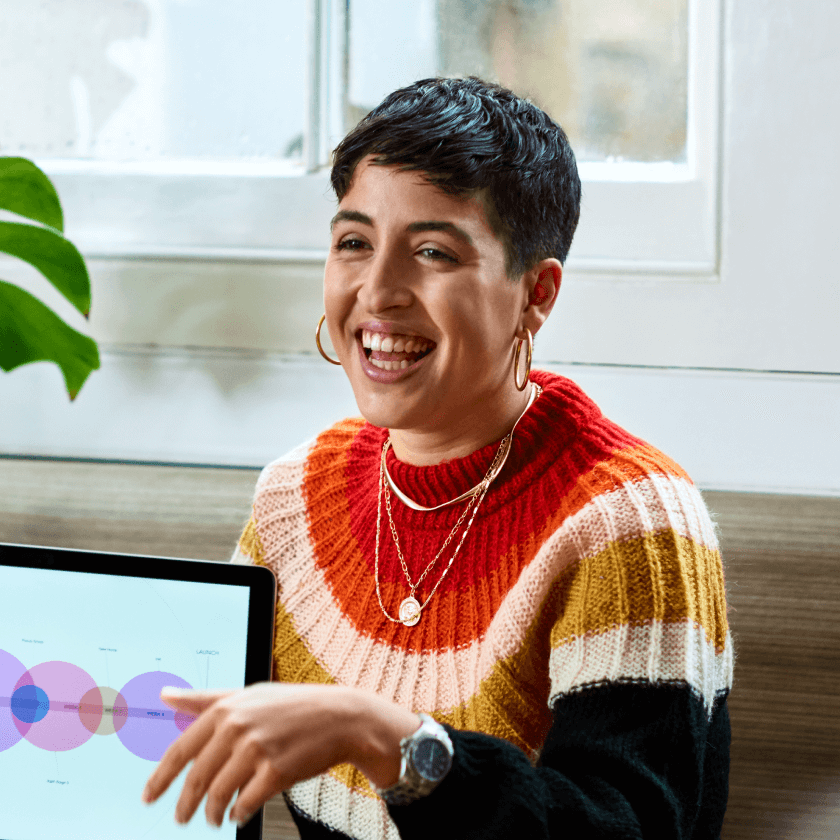