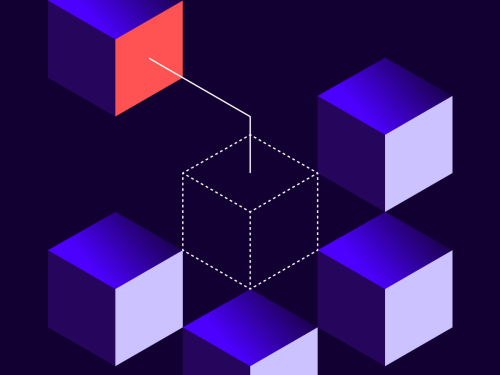
From the Trenches: Sending envelopes asynchronously
Karan Kaushik explains how to use asynchronous createEnvelope calls to free up Docusign resources for your apps.
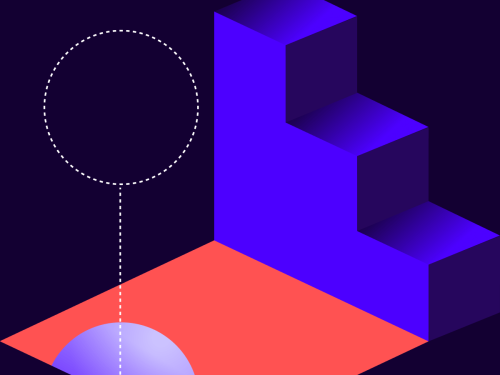
In my previous article on How to manage long-running CreateEnvelope calls, I went over various ways you can track and optimize your createEnvelope calls. In this post, I am going to explain how you can modify your createEnvelope
call to send envelopes asynchronously.
The need for asynchronous sending
Asynchronous sending allows your application to continue to perform other tasks while your createEnvelope
call is still processing instead of waiting for Docusign to finish processing and return a response.
How to use asynchronous sending
Asynchronous sending, available both in our SOAP and REST APIs, is identical to our synchronous createEnvelope
call with the addition of two parameters: asynchronous
and transactionId
.
Here’s an example of how to set these parameters in our Node.JS SDK:
let envelopeDefinition = docusign.EnvelopeDefinition.constructFromObject({
asynchronous: true, // set it true to mark the envelope as asynchronous
documents: YOUR_DOCUMENTS_HERE,
emailSubject: EMAIL_SUBJECT_HERE,
recipients: YOUR_RECIPIENTS_HERE,
status: "sent",
transactionId: "1" // Transaction Id must be specified
});
// Send Envelope as usual
When you make an asynchronous createEnvelope
call, Docusign will respond immediately with:
{
"status": "processing"
}
And no other details will be included, such as the envelopeId
, the recipients, and so on. The transactionId
being specified must be unique. Duplicate transactionIds
in your createEnvelope
calls will be ignored.
Server-side asynchronous vs. client-side asynchronous
You might be thinking, the createEnvelope
methods available in our SDKs are already asynchronous; how is this any different?
You are correct in that our createEnvelope
SDK methods are already asynchronous. But the key difference is that the createEnvelope
methods are client-side asynchronous; meaning, they won’t block your UI and will allow your application to do other things instead of waiting. However, by setting the asynchronous
property, you convert the call to a server-side asynchronous transaction. Server-side asynchronous mode is used to free up resources in your infrastructure (in this case, memory, threads, and more) to allow other parts of your application to use these resources and achieve greater scalability.
What happens when the envelope is processed
To get the status of the envelope, you can use the Envelope:ListStatus endpoint with the query parameter transaction_ids
followed by a list of transactionIds
, which will then return the statuses of all the envelopes associated with the specified transactionIds
. Alternatively, if you have Docusign Connect set up on your account with the Envelope Sent event subscribed, your listener will receive an event when the envelope has finished processing and is sent.
If your createEnvelope
call fails because of an error, it will not be immediately returned and you will need to query the Envelopes:ListStatus endpoint.
When implementing a new workflow, Docusign recommends testing the new envelope format synchronously first in your developer account to confirm there are no errors in the body.
Additional resources
Karan Kaushik began his Docusign career in January 2022. As a front-line developer support engineer, Karan enjoys working on complex technical problems. He is passionate about using technology to make people's day-to-day lives easier and simpler, leveraging his array of experience across information technology, cloud operations, and software development.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
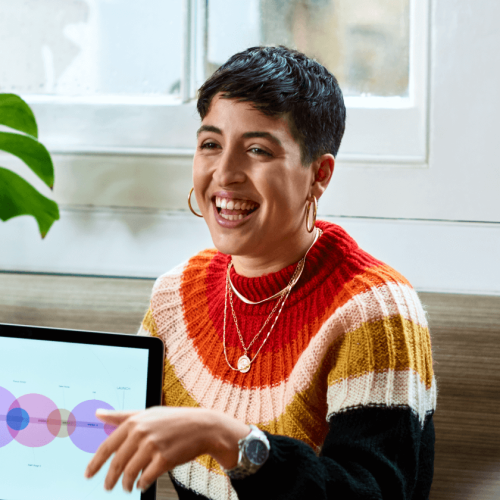