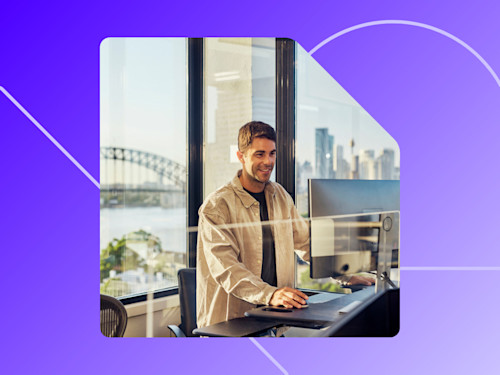
Common API Tasksđ: Update password rules
Inbar shows you how to use the API to set password rules for length and required characters.
Table of contents
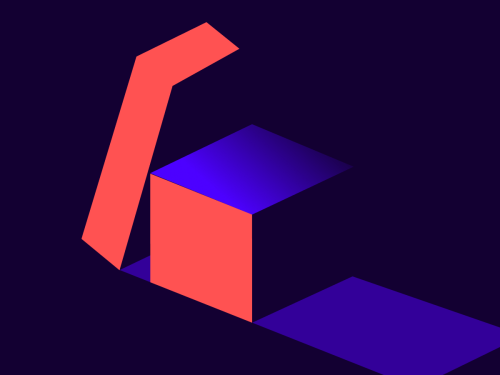
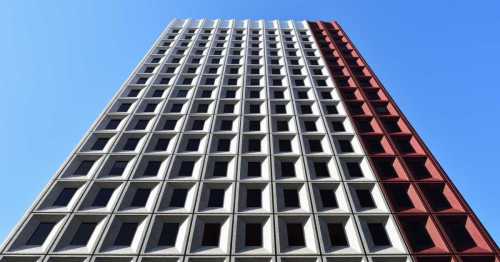
Welcome to a marvelous new edition of the CATđ (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.Â
In todayâs edition weâre going to talk about passwords: those little annoying pieces of text one has to enter to authenticate into some online service. In our case, weâre talking about the Docusign account password. Administrators of a given Docusign account can control the password rules for every user of the account. They can decide how complex a password must be in order to be used to access Docusign. This includes the minimum character length as well as requirements to include certain characters.Â
The administrators can modify these requirements by going to the Docusign eSignature Settings page and, under Security Settings, selecting SHOW PASSWORD RULES, which will pop up the following dialog:
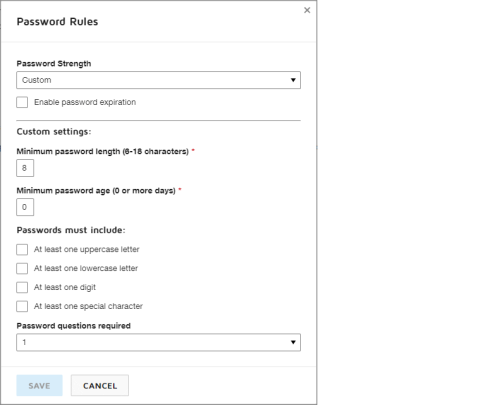
However, all of the settings in this dialog can also be modified programmatically using the eSignature REST API, and thatâs what Iâm about to show you.Â
The following code snippets demonstrate how to first check the minimum allowed password length. Then, if itâs not at least 8 characters, the code makes another API call to update the password rules and set the minimum password length to 8. There are other things you can change here and you can explore them on your own.Â
Note: The access token for making API calls in the code examples below must come from a user with administrator privileges. These settings are account-wide and are not just for the logged-in user.Â
Also note that in this case, if a user had a password with, say, 7 characters, they would still be able to log in to Docusign after you make this change. However, after they log in, theyâll be prompted to change their password and make it compliant with the new policy.
And without further ado, here are the six code snippets:
C#
var apiClient = new ApiClient(basePath);
// You will need to obtain an access token using your chosen authentication flow
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
AccountsApi accountsApi = new AccountsApi(apiClient);
var accountPasswordRules = accountsApi.GetPasswordRules(accountId);
int minPassword = int.Parse(accountPasswordRules.MinimumPasswordLength);
if (minPassword
<h2>Java</h2>
<code class="language-java" data-uuid="vXuuwF48">// You will need to obtain an access token using your chosen authentication flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
AccountsApi accountsApi = new AccountsApi(config);
AccountPasswordRules accountPasswordRules = accountsApi.GetPasswordRules(accountId);
int minPassword = parseInt(accountPasswordRules.getMinimumPasswordLength());
if (minPassword
<h2>Node.js</h2>
<code class="language-javascript" data-uuid="uCHBlO5N">// You will need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let accountsApi = new docusign.AccountsApi(apiClient);
let accountPasswordRules = accountsApi.getPasswordRules(accountId);
let minPassword = accountPasswordRules.MinimumPasswordLength;
if (minPassword
<h2>PHP</h2>
<code class="language-php" data-uuid="xczrAc7t"># You will need to obtain an access token using your chosen authentication flow
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$account_api = new \Docusign\eSign\Api\AccountsApi($api_client);
$account_password_rules = $accounts_api->getPasswordRules(accountId);
$min_password = intval($account_password_rules->getMinimumPasswordLength());
if ($min_password setMinimumPasswordLength("8");
$accounts_api->updatePasswordRules($account_id, $account_password_rules);
}
</code>
<h2>Python</h2>
<code class="language-python" data-uuid="xPLlGCFH"># You will need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
accounts_api = AccountsApi(api_client)
account_password_rules = accounts_api.get_password_rules(account_id)
min_password = int(account_password_rules.minimum_password_length)
if min_password
<h2>Ruby</h2>
<code class="language-ruby" data-uuid="iDmwqQQy"># You will need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
accounts_api = DocuSign_eSign::AccountsApi.new api_client
account_password_rules = accounts_api.get_password_rules(account_id)
min_password = account_password_rules.minimum_password_length + 0
if min_password
<p>And thatâs a wrap! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to <a href="mailto:inbar.gazit@docusign.com">email me</a>. Until next time...</p>
<h2>Additional resources</h2>
<ul><li><a href="https://developers.docusign.com/">Docusign Developer Center</a></li>
<li><a href="https://twitter.com/docusignapi">@DocuSignAPI on Twitter</a></li>
<li><a href="https://www.linkedin.com/showcase/docusign-for-developers/">Docusign for Developers on LinkedIn</a></li>
<li><a href="https://www.youtube.com/playlist?list=PLXpRTgmbu4opxdx2IThm4pDYS8tIKEb0w">Docusign for Developers on YouTube</a></li>
<li><a href="https://developers.docusign.com/#newsletter-signup">Docusign Developer Newsletter</a></li>
</ul></code></code></code></code>
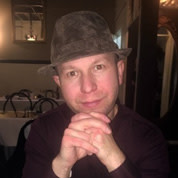
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
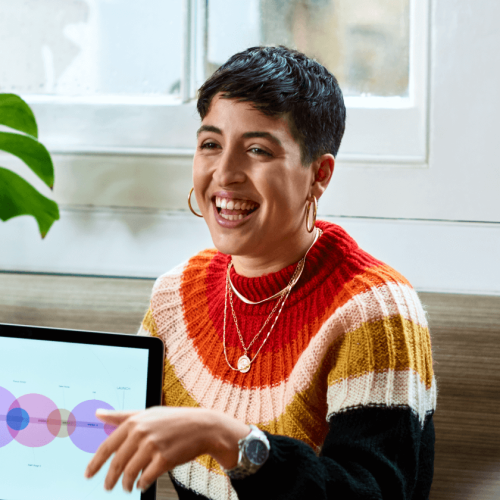