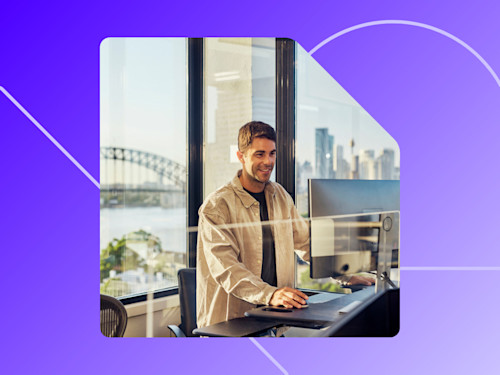
Common API Tasksđ: Let your signers decide where to place the tabs for you
Docusign lets you send an envelope with Freeform Signing, which allows your recipient to set their own tabs. Inbar shows you how.
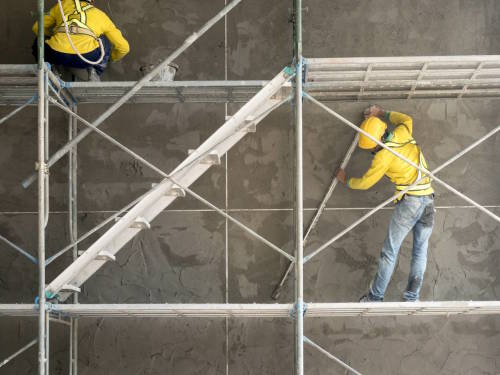
Welcome to a sensational new edition of the CATđ (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
Docusign eSignature involves documents, recipients and tabs. All these things are part of envelopes that are the containers for transactions. The envelope must contain one or more of each of these things if you want it to be complete (âsignedâ is the term we use day to day, but what if an envelope has two documents and you only signed one of them? Or two recipients and only one of them signed? Then the envelope is not yet complete.)
So, you must have at least one tab in your envelope. Most examples you will see on Dev Center show how you, as the owner and sender of the envelope, place the tabs. There are different ways of placing the tabs, using anchor strings and with fixed positioning, calculating pixels and figuring out on what page of the document each of them goes.
What if, and hear me out here, you are lazy, and you want your signers to do the hard work? Let them figure out where each signature tab should be placed, or where to put initials and dates. You donât have time for all of this, so letâs delegate!Â
Well, you can do that with Docusign. You can indeed send an envelope to your recipients without placing a single tab in it. Or you can use embedded signing with such an envelope. If Docusign doesnât find any tabs on the envelope, it will ask the signer to place the tabs by showing them a toolbar, and enabling the signer to drag and drop tabs (tap and drop on mobile) to the document(s) as they see fit. This ability of the signer to tag the document or place the tabs themselves is what we sometimes call Freeform Signing, suggesting that the owner/sender of the envelope let the signer freely decide where to place the tabs.
Pros: You donât need to spend the time to make sure the tabs are placed correctly.
Cons: Your signer may not place them correctly. You cannot enforce this. But maybe you donât care? Also note that you cannot force the signer to place more than one tab. Docusign requires a minimum of one tab to complete an envelope; this minimum is global and cannot be modified by you. If you expect your signer to sign in two places, they may just sign in one of them and call it a day.Â
Still, if that works for you, here is how you do it programmatically.
C#
var docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
var envelopesApi = new EnvelopesApi(docuSignClient);
var envelope = new EnvelopeDefinition();
envelope.EmailSubject = "Add tabs where needed and sign this for me";
// Adding the signer
var signer1 = new Signer();
signer1.Email = "inbar.gazit@docusign.com";
signer1.Name = "Inbar Gazit";
signer1.RecipientId = "1";
envelope.Recipients = new Recipients { Signers = new List<signer> { signer1 } };
// Adding the document
var doc1 = new Document();
doc1.DocumentBase64 = "VGhhbmtzIGZvciByZXZpZXdpbmcgdGhpcyEKCldlJ2xsIG1vdmUgZm9yd2FyZCBhcyBzb29uIGFzIHdlIGhlYXIgYmFjay4=";
doc1.DocumentId = "1";
doc1.Name = "Welcome";
doc1.FileExtension = "txt";
envelope.Documents = new List<document> { doc1 };
envelope.Status = "sent";
EnvelopeSummary results = envelopesApi.CreateEnvelope(accountId, envelope);
</document></signer>
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
// Generate an object with the envelopeâs metadata
EnvelopeDefinition envelope = new EnvelopeDefinition();
envelope.setEmailSubject("Add tabs where needed and sign this for me");
// Add the document
Document doc1 = new Document();
doc1.setDocumentBase64("VGhhbmtzIGZvciByZXZpZXdpbmcgdGhpcyEKCldlJ2xsIG1vdmUgZm9yd2FyZCBhcyBzb29uIGFzIHdlIGhlYXIgYmFjay4=");
doc1.setDocumentId("1");
doc1.setName("Welcome");
doc1.setFileExtension("txt");
envelope.setDocuments().Add(Arrays.asList(doc1));
// Add the recipient
envelope.setRecipients(new Recipients());
Signer signer1 = new Signer();
signer1.setEmail("inbar.gazit@docusign.com");
signer1.setName("Inbar Gazit");
signer1.setRecipientId("1");
envelope.getRecipients().setSigners(Arrays.asList(signer1));
envelope.setStatus("sent");
EnvelopeSummary envelopeSummary = envelopesApi.CreateEnvelope(accountId, envelope);
Node.js
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
// Generate an object with the envelopeâs metadata
let envelope = new docusign.EnvelopeDefinition();
envelope.emailSubject = 'Add tabs where needed and sign this for me';
// Add the document
let doc2 = new docusign.Document();
doc1.documentBase64 = 'VGhhbmtzIGZvciByZXZpZXdpbmcgdGhpcyEKCldlJ2xsIG1vdmUgZm9yd2FyZCBhcyBzb29uIGFzIHdlIGhlYXIgYmFjay4=';
doc1.documentId = '1';
doc1.fileExtension = 'txt';
doc1.name = 'Welcome';
envelope.documents = [doc1];
// Add the recipient
envelopeDefinition.Recipients = new docusign.Recipients();
let signer1 = new docusign.Signer();
signer1.email = 'inbar.gazit@docusign.com';
signer1.name = 'Inbar Gazit';
signer1.recipientId = '1';
envelope.recipients.signers = [signer1];
envelope.status = 'sent';
let envelopeSummary = envelopesApi.createEnvelope(accountId, envelope);
PHP
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \Docusign\eSign\Api\EnvelopesApi($api_client);
# Generate an object with the envelopeâs metadata
$envelope = new \Docusign\eSign\Model\EnvelopeDefinition();
$envelope->setEmailSubject('Add tabs where needed and sign this for me');
# Add the document
$doc1 = new \Docusign\eSign\Model\Document();
$doc1->setDocumentBase64('VGhhbmtzIGZvciByZXZpZXdpbmcgdGhpcyEKCldlJ2xsIG1vdmUgZm9yd2FyZCBhcyBzb29uIGFzIHdlIGhlYXIgYmFjay4=');
$doc1->setDocumentId('1');
$doc1->setName('Welcome');
$doc1->setFileExtension('txt');
$envelope->setDocuments().Add([$doc1]);
# Add the recipient
$envelope->setRecipients(new \Docusign\eSign\Model\Recipients());
$signer1 = new \Docusign\eSign\Model\Signers();
$signer1->setEmail('inbar.gazit@docusign.com');
$signer1->setName('Inbar Gazit');
$signer1->setRecipientId('1');
$envelope->getRecipients()->setSigners([$signer1]);
$envelope->setStatus('sent');
$envelope_summary = envelopesApi->CreateEnvelope($account_id, $envelope);
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
envelope = EnvelopeDefinition()
envelope.email_subject = 'Add tabs where needed and sign this for me'
# Add the document
doc1 = Document()
doc1.document_base64 = 'VGhhbmtzIGZvciByZXZpZXdpbmcgdGhpcyEKCldlJ2xsIG1vdmUgZm9yd2FyZCBhcyBzb29uIGFzIHdlIGhlYXIgYmFjay4='
doc1.document_id = '1'
doc1.name = 'Welcome'
doc1.file_extension = 'txt'
envelope.documents = [doc1]
# Add the recipient
envelope.recipients = Recipients()
signer1 = Signer()
signer1.email = 'inbar.gazit@docusign.com'
signer1.name = 'Inbar Gazit'
signer1.recipient_id = '1'
envelope.recipients.signers = [signer]
envelope.status = 'sent'
envelope_summary = envelopes_api.create_envelope(account_id, envelope)
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
envelope = DocuSign_eSign::EnvelopeDefinition().new
envelope.email_subject = 'Add tabs where needed and sign this for me'
# Add the document
doc1 = DocuSign_eSign::Document().new
doc1.document_base64 = 'VGhhbmtzIGZvciByZXZpZXdpbmcgdGhpcyEKCldlJ2xsIG1vdmUgZm9yd2FyZCBhcyBzb29uIGFzIHdlIGhlYXIgYmFjay4='
doc1.document_id = '1'
doc1.name = 'Welcome'
doc1.file_extension = 'txt'
envelope.documents = [doc1]
# Add the recipient
envelope.recipients = DocuSign_eSign::Recipients().new
signer1 = DocuSign_eSign::Signer().new
signer1.email = 'inbar.gazit@docusign.com'
signer1.name = 'Inbar Gazit'
signer1.recipient_id = '1'
envelope.recipients.signers = [signer1]
envelope.status = 'sent'
envelope_summary = envelopes_api.create_envelope(account_id, envelope)
Thatâs all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
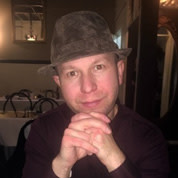
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
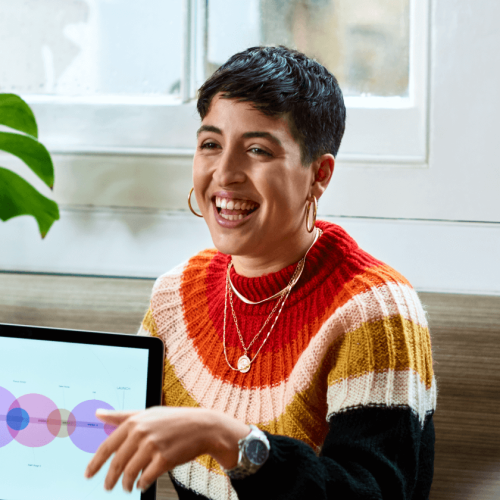