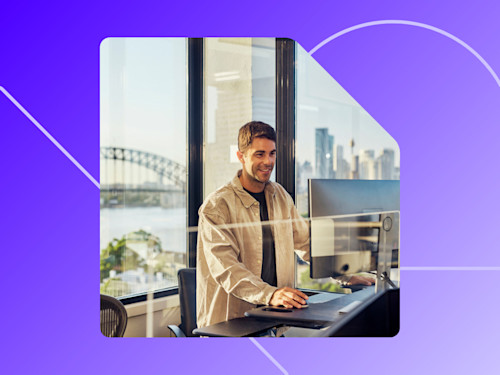
Common API Tasksđ: Cloning an envelope
Get sample code for making a copy of an existing envelope using the eSignature REST API.
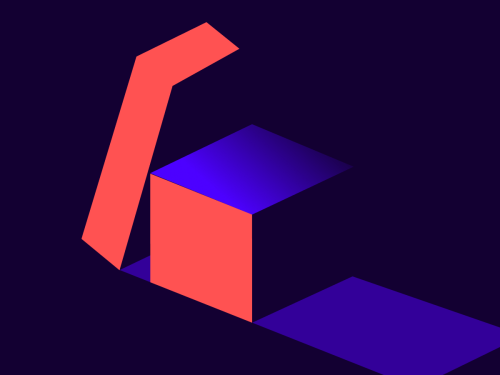
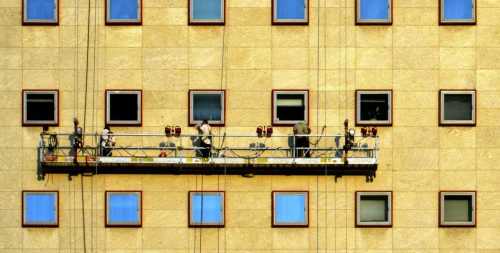
Welcome to another edition of the CAT (Common API Tasks) blog. This blog series is all about giving you useful information about using the Docusign APIs. In each post I provide all you need to complete small, specific, SDK-supported tasks using one of our APIs.Â
You can find all articles in this series on the Docusign developer blog.Â
For this issue I picked the topic of clones. Did you know that you can clone an entire envelope and create a copy of that original envelope? This can be very useful for various scenariosâmostly situations where you want to send a brand new envelope with the same information (documents) in it, but you donât already have a template set up to do so.Â
As with most of my blog posts in this series, the activity I talk about can be performed from the Docusign web application. In this case, you can go to the Manage tab of the Docusign eSignature web app, select an envelope, open the small menu just right of it, and select Create a Copy.Â
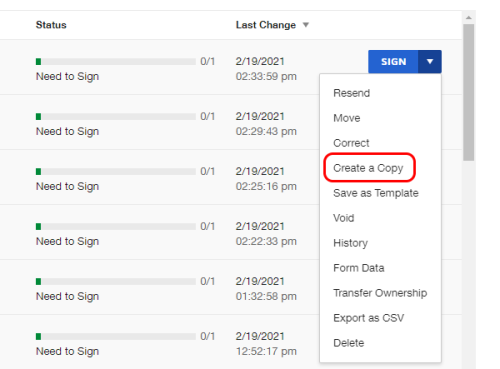
The web application will first call the Docusign eSignature REST API to create a clone of the envelope you selected, and then redirect you to the Prepare screen so you can continue to work on it. The new envelope is created in draft status, so it can be edited before it is sent out.
Only envelopes that have been sent (either in transit, waiting for signature, or completed when all recipients have acted on it) can be cloned. Draft envelopes cannot be cloned. Because you can just keep working on them in their current state, thereâs no need to clone; after all, the purpose of cloning is to create a draft envelope.
When it comes to doing this programmatically, itâs rather simple. All you have to do is provide the original envelopeId (which must be from the same Docusign account) and you can optionally specify whether you want the recipients to be copied over as well. Thatâs it. Youâll get back a new envelopeId representing the new copy that was just created, and you can take it from there. Let's code this in our usual six SDK languages.
C#
// You need to obtain an access token using your chosen authentication flow
var apiClient = new ApiClient(basePath);
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
var envelopeDefinition = new EnvelopeDefinition();
envelopeDefinition.EnvelopeId = "bc4799a2-xxxx-xxxx-xxxx-20d1921aeb87"; // Your original envelope you're cloning
envelopeDefinition.CopyRecipientData = "false"; // Change to also copy recipients
EnvelopeSummary results = envelopesApi.CreateEnvelope(accountId, envelopeDefinition);
string envelopeId = results.EnvelopeId; // Cloned envelope
Java
// You need to obtain an access token using your chosen authentication flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(config);
EnvelopeDefinition envelopeDefinition = new EnvelopeDefinition();
envelopeDefinition.setEnvelopeId("bc4799a2-xxxx-xxxx-xxxx-20d1921aeb87"); // Your original envelope you're cloning
envelopeDefinition.setCopyRecipientData("false"); // Change to also copy recipients
EnvelopeSummary results = envelopesApi.CreateEnvelope(accountId, envelopeDefinition);
string envelopeId = results.getEnvelopeId(); // Cloned envelope
Node.js
// You need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
let envelopeDefinition = new docusign.EnvelopeDefinition();
envelopeDefinition.envelopeId = 'bc4799a2-xxxx-xxxx-xxxx-20d1921aeb87'; // Your original envelope you're cloning
envelopeDefinition.copyRecipientData = 'false'; // Change to also copy recipients
let results = envelopesApi.createEnvelope(accountId, envelopeDefinition);
var envelopeId = results.envelopeId; // Cloned envelope
PHP
# You need to obtain an access token using your chosen authentication flow
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \Docusign\Api\EnvelopesApi($config);
$envelope_definition = new \Docusign\eSign\Model\docusign.EnvelopeDefinition();
$envelope_definition->setEnvelopeId('bc4799a2-xxxx-xxxx-xxxx-20d1921aeb87'); # Your original envelope you're cloning
$envelope_definition.setCopyRecipientData('false'); # Change to also copy recipients
$results = $envelopes_api->createEnvelope($account_id, $envelope_definition);
$envelope_id = results->getEnvelopeId(); # Cloned envelope
Python
# You need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.host = base_path
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
envelope_definition = EnvelopeDefinition()
envelope_definition.envelope_id ='bc4799a2-xxxx-xxxx-xxxx-20d1921aeb87' # Your original envelope you're cloning
envelope_definition.copy_recipient_data = 'false' # Change to also copy recipients
results = envelopes_api.create_envelope(account_id, envelope_definition)
envelope_id = results.envelope_id # Cloned envelope
Ruby
# You need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
envelope_definition = DocuSign_eSign::EnvelopeDefinition().new
envelope_definition.envelope_id ='bc4799a2-xxxx-xxxx-xxxx-20d1921aeb87' # Your original envelope you're cloning
envelope_definition.copy_recipient_data = 'false' # Change to also copy recipients
results = envelopes_api.create_envelope(account_id, envelope_definition)
envelope_id = results.envelope_id # Cloned envelope
I hope you found this useful. As usual, if you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
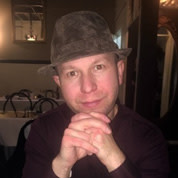
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
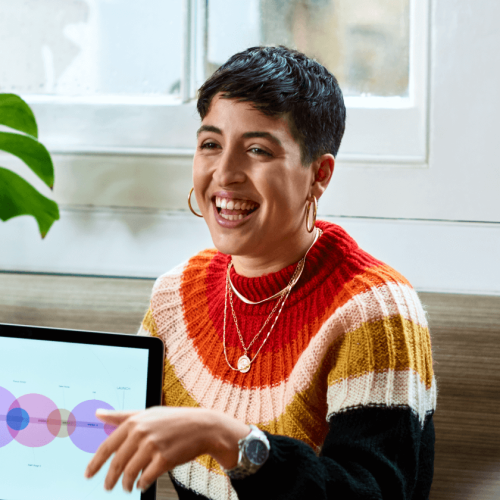