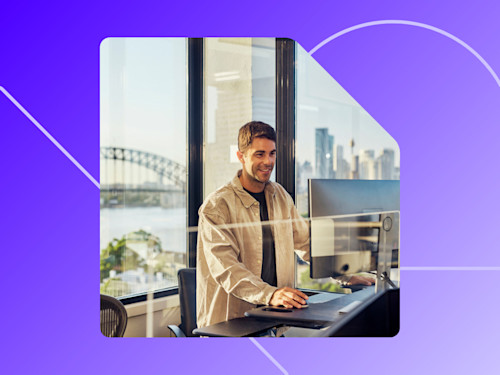
Common API Tasksđ: Change the language of your Docusign web app
See how to invoke the eSignature REST API through our SDKs to set the language of your web app users.
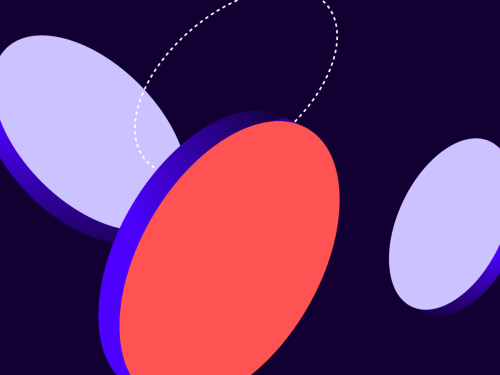
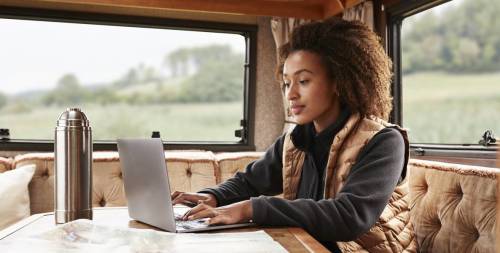
Welcome to an awesome new edition of the CATđ (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.Â
Did you know that the Docusign signing experience can be localized in 44 different languages? And that the Docusign web app supports 14 different languages? Docusign is a global company and we support signers in almost every country. The web app can currently work in 14 languages and more will be coming in the future. You can use the eSignature REST API to set the appropriate language both for signers as well as web app users. In this blog post Iâm going to show you how to do the latter.Â
The code snippets below are very simple. The language selection is applied to a specific user. As an administrator you can control other usersâ language, and if you run this in a context of a specific user, then you can always update that userâs language.
The method to update the user settings is used with the parameter called locale. A locale is a short form designation of the chosen language (note that it is case sensitive). Here is the list of 14 languages supported by the Docusign web app and each of their locals:
Language
Locale
English
en
German
de
Spanish
es
French
fr
Italian
it
Dutch
nl
Polish
pl
Brazilian Portuguese
pt_BR
Portuguese
pt
Russian
ru
Traditional Chinese
zh_TW
Simplified Chinese
zh_CN
Japanese
ja
Korean
ko
So, to summarize, you need three pieces of information:
accountId**:** that is needed for almost any API call.
userId**:** you can find it on the Apps and Keys page.
locale**:** the desired languages from the table above.
Once you have these, the code snippets are pretty straightforward. Here they are:
C#
var apiClient = new ApiClient(basePath);
// You will need to obtain an access token using your chosen authentication method
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
var usersApi = new UsersApi(apiClient);
var userSettingsInformation = new UserSettingsInformation { Locale = "es" };
// Make sure to find the values for accountId and userId
usersApi.UpdateSettings(accountId, userId, userSettingsInformation);
Java
// You will need to obtain an access token using your chosen authentication flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
UsersApi usersApi = new UsersApi(config);
UserSettingsInformation userSettingsInformation = new UserSettingsInformation();
userSettingsInformation.setLocale("es");
// Make sure to find the values for accountId and userId
usersApi.UpdateSettings(accountId, userId, userSettingsInformation);
Node.js
// You will need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let usersApi = new docusign.UsersApi(apiClient);
let userSettingsInformation = new docusign.UserSettingsInformation();
userSettingsInformation.locale = 'es';
// Make sure to find the values for accountId and userId
usersApi.updateSettings(accountId, userId, userSettingsInformation);
PHP
# You will need to obtain an access token using your chosen authentication flow
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$users_api = new \Docusign\eSign\Api\UsersApi($api_client);
$user_settings_information = new \Docusign\eSign\Model\UserSettingsInformation();
$user_settings_information->setLocale('es');
# Make sure to find the values for $account_id and $user_id
$users_api->updateSettings($account_id, $user_id, $user_settings_information);
Python
# You will need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
users_api = UsersApi(api_client)
user_settings_information = UserSettingsInformation()
user_settings_information.locale = 'es'
# Make sure to find the values for account_id and user_id
users_api.update_settings(account_id, user_id, user_settings_information)
Ruby
# You will need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
users_api = DocuSign_eSign::UsersApi.new api_client
user_settings_information = DocuSign_eSign::UserSettingsInformation.new
user_settings_information.locale = 'es'
# Make sure to find the values for account_id and user_id
users_api.update_settings(account_id, user_id, user_settings_information)
And thatâs a wrap! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
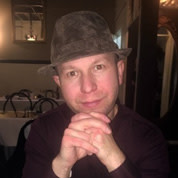
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
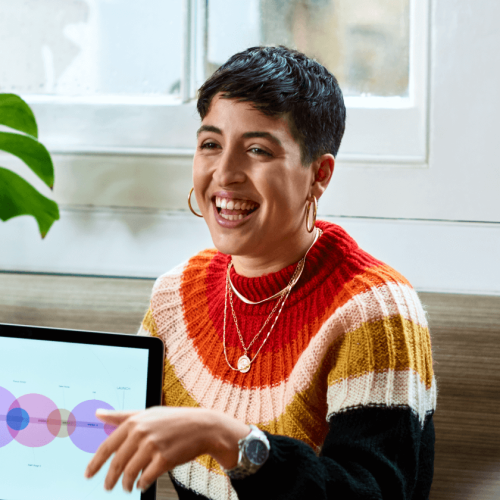