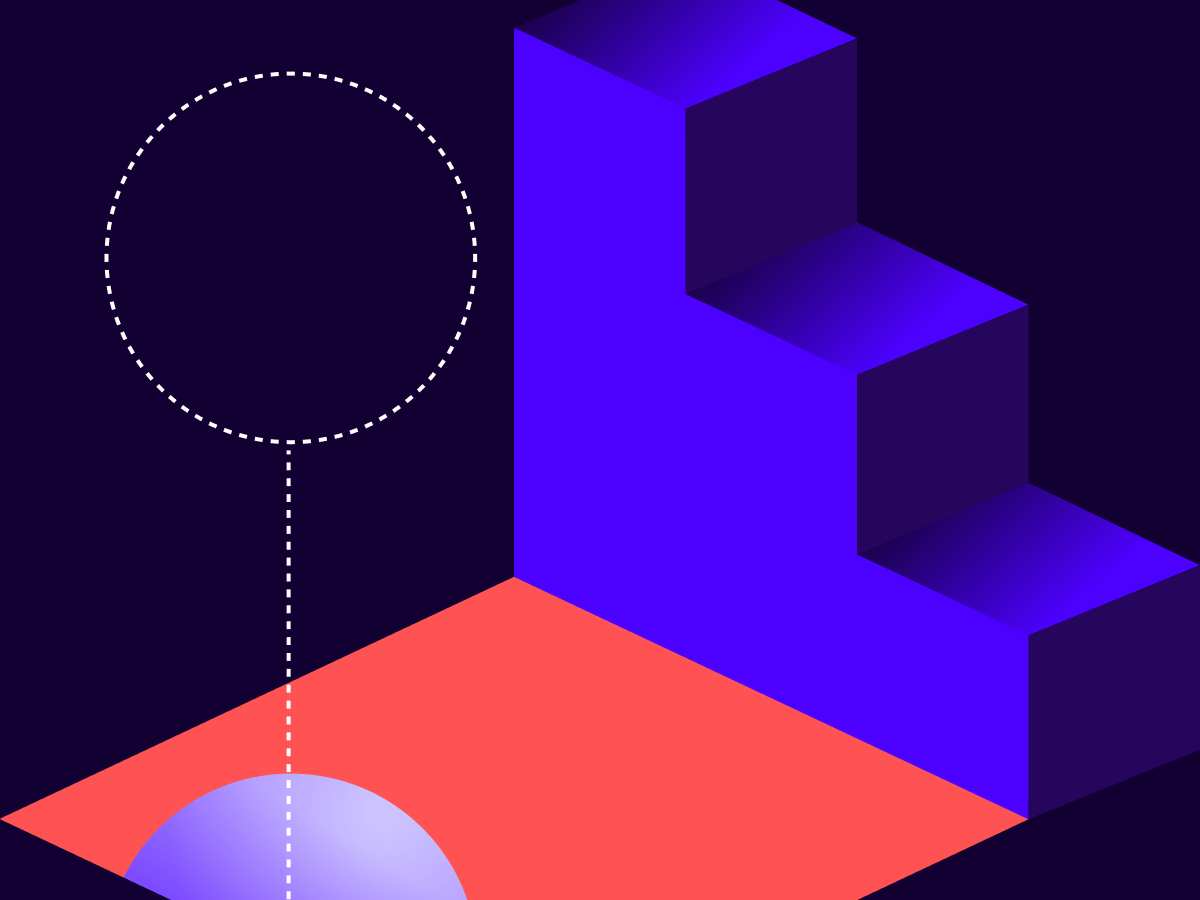
Common API Tasksđ: Adding a recipient to an in-flight envelope
Learn how to use the Docusign eSignature REST API to update a recipient after an envelope was sent
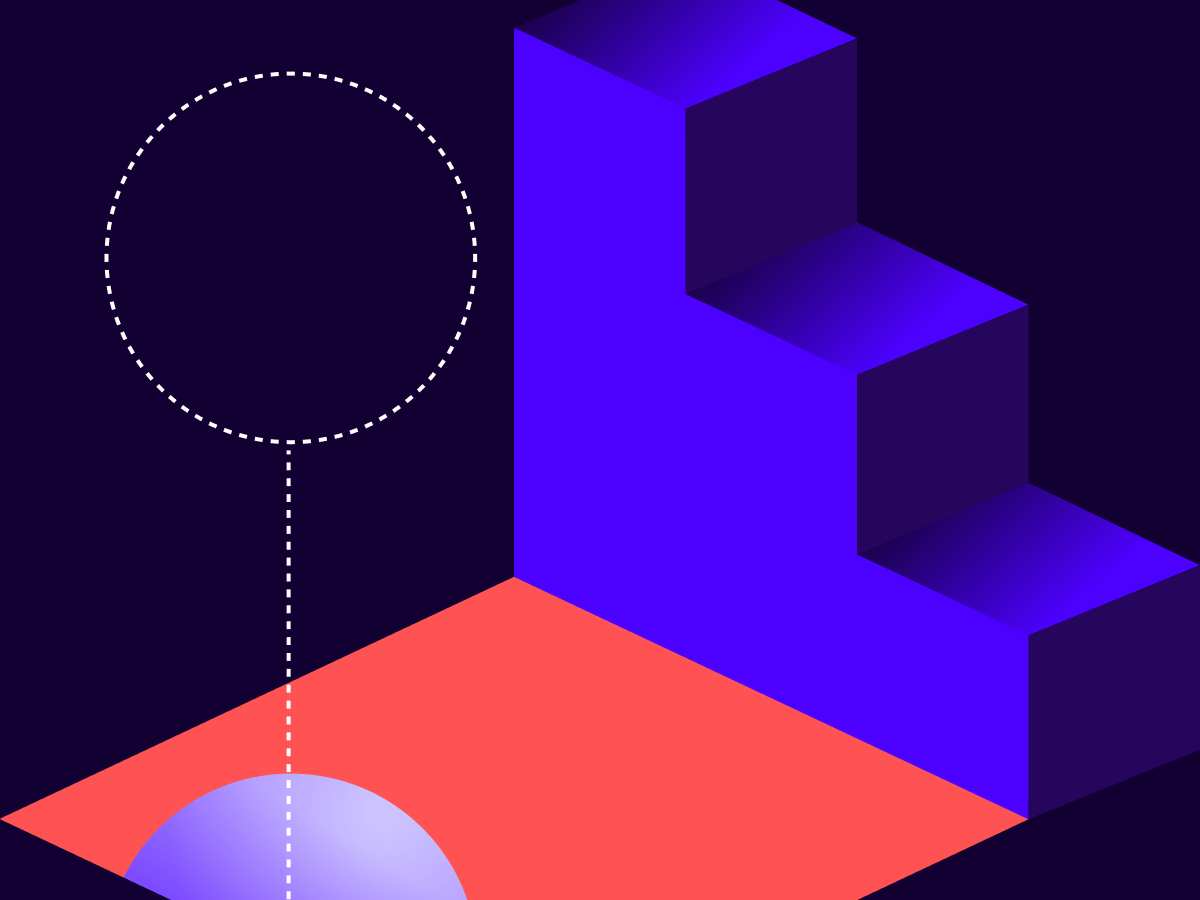
If youâre like me, before you send an email, or a text message, or an envelope, you double-check it and make sure that it's all correct. Your customers using your integrations to send envelopes probably do that as well. But, alas, sometimes mistakes happen and we forget to add that new hire in accounting; she needs to get a copy of the expense report at the end. Sounds familiar? The envelope has already been sent, and now you need to add another recipient. Itâs even possible someone already signed it (but not everyone, itâs not complete yet). You can add a recipient programmatically using the eSignature REST API, and thatâs what Iâm going to show you in this blog post.Â
This post is part of a series I call Common API Tasks (CAT) and is meant to help you with small, specific, SDK-supported tasks. That means I always give you six code examples in our primary coding languages: C#, Java, Node.js, PHP, Python, and Ruby. No developer is left behind (well, unless youâre a COBOL developer; then youâre out of luck :).
So, letâs get to business. We have an envelope that has been sent. You have to know the envelopeId, which is a GUID that uniquely identifies an envelope in the Docusign system. Each envelope in Docusign also has a specific status. The envelope in this case is in sent status. That means that the recipients can start signing it. Itâs possible, for example, that the envelope was sent to two recipients, both of whom received an email (or signed using embedded signing), but one of them has not signed yet. It's important that at least one recipient has yet to act on the envelope. That is because, if all recipients acted on the envelope, then it automatically becomes complete. A complete envelope is done, finished, and cannot be modified. So we have to ensure our envelope is not complete.Â
So, we have an envelope that was sent for two recipients to sign. We would like to add Jane Doe as a Carbon Copy recipient, and ensure she only gets her copy when both signers finish signing. We use the UpdateRecipients method of the SDK. This method can be used to modify existing recipients as well, but in our case, we just want to add a new one.Â
C#
var apiClient = new ApiClient(basePath);
// You will need to obtain an access token using your chosen authentication flow
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
var envelopesApi = new EnvelopesApi(apiClient);
string envelopeId = "2a4fe901-xxxx-xxxx-xxxx-0844734eb7a0";
var recipients = new Recipients { CarbonCopies = new List<carboncopy>() };
recipients.CarbonCopies.Add(new CarbonCopyÂ
{Â
    Email = "janedoe@example.com",Â
    Name = "Jane Doe",Â
    DeliveryMethod = "email",Â
   RecipientId = "11", // This has to be different only for this envelope; GUIDs are OK, too
   RoutingOrder = "3" // We already had two recipients; Jane will get the copy after they have both signed
});
// When making the API call, we get back a summary of what was updated. If there are errors, no exception will be thrown; instead, the summary will include the error information
var summary = envelopesApi.UpdateRecipients(accountId, envelopeId, recipients);</carboncopy>
Java
// You will need to obtain an access token using your chosen authentication flowÂ
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(config);
String envelopeId = "2a4fe901-xxxx-xxxx-xxxx-0844734eb7a0";
Recipients recipients = new Recipients();
recipients.setCarbonCopies(new java.util.List<CarbonCopy>());
CarbonCopy cc = new CarbonCopy();
cc.setEmail("janedoe@example.com",);
cc.setName("Jane Doe");
cc.setDelieveryMethod("email");
cc.setRecipientId("11"); // This has to be different only for this envelope; GUIDs are OK, too
cc.setRoutingOrder("3") // We already had two recipients; Jane will get the copy after they have both signed
recipients.getCarbonCopies().Add(cc);
// When making the API call, we get back a summary of what was updated. If there are errors, no exception will be thrown; instead, the summary will include the error information
RecipientsUpdateSummary summary = envelopesApi.UpdateRecipients(accountId, envelopeId, recipients);
Node.js
// You will need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
let recipients = new docusign.Recipients { CarbonCopies = []};
recipients.carbonCopies.push(new docusign.CarbonCopy
{
Email = 'janedoe@example.com',
Name = 'Jane Doe',
DeliveryMethod = 'email',
RecipientId = '11', // This has to be different only for this envelope; GUIDs are OK, too
RoutingOrder = '3' // We already had two recipients; Jane will get the copy after they have both signed
});
// When making the API call, we get back a summary of what was updated. If there are errors, no exception will be thrown; instead. the summary will include the error information
let summary = envelopesApi.updateRecipients(accountId, envelopeId, {recipients});
PHP
# You will need to obtain an access token using your chosen authentication flowÂ
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \Docusign\Api\EnvelopesApi($config);
$envelope_id = '2a4fe901-xxxx-xxxx-xxxx-0844734eb7a0';
$recipients = new \Docusign\eSign\Model\Recipients();
$recipients->setCarbonCopies([]);
$cc = new \Docusign\eSign\Model\CarbonCopy();
$cc->setEmail(âjanedoe@example.comâ);
$cc->setName = (âJane Doeâ);Â
$cc->setDeliveryMethod(âemailâ);Â
$cc->setRecipientId(â11â); # This has to be different only for this envelope; GUIDs are OK, too
$cc->setRoutingOrder(â3â);Â # We already had two recipients; Jane will get the copy after they have both signed
array_push($recipients->getCarbonCopies(), $cc);
# When making the API call, we get back a summary of what was updated. If there are errors, no exception will be thrown; instead, the summary will include the error information
$summary = $envelopes_api->updateRecipients($account_id, $envelope_id, $recipients);
Python
# You will need to obtain an access token using your chosen authentication flowÂ
api_client = ApiClient()
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(config)
envelope_id = '2a4fe901-xxxx-xxxx-xxxx-0844734eb7a0'
recipients = Recipients()
recipients.carbon_copies = []
cc = CarbonCopy()
cc.email = 'janedoe@example.com'
cc.name = 'Jane Doe'
cc.delivery_method ='email'
cc.recipient_id = '11' # This has to be different only for this envelope; GUIDs are OK, too
cc.routing_order = '3'Â # We already had two recipients; Jane will get the copy after they the both signed
recipients.carbon_copies.append(cc)
# When making the API call, we get back a summary of what was updated. If there are errors, no exception will be thrown; instead, the summary will include the error information
summary = envelopes_api.update_recipients(account_id, envelope_id, recipients)
Ruby
# You will need to obtain an access token using your chosen authentication flowÂ
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
envelope_id = '2a4fe901-xxxx-xxxx-xxxx-0844734eb7a0'
recipients = DocuSign_eSign::Recipients.new
recipients.carbon_copies = []
cc = DocuSign_eSign::CarbonCopy.new
cc.email = 'janedoe@example.com'
cc.name = 'Jane Doe'
cc.delivery_method ='email'
cc.recipient_id = '11' # This has to be different only for this envelope; GUIDs are OK, too
cc.routing_order = '3'Â # We already had two recipients; Jane will get the copy after they have both signed
recipients.carbon_copies.push(cc)
# When making the API call, we get back a summary of what was updated. If there are errors,- no exception will be thrown; instead, the summary will include the error information.
summary = envelopes_api.update_recipients(account_id, envelope_id, recipients)
I hope you found this useful. As usual, if you have any questions, comments, or suggestions for topics to feature in future Common API Tasks posts, feel free to email me. Until next timeâŚ
Additional resources
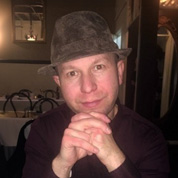
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
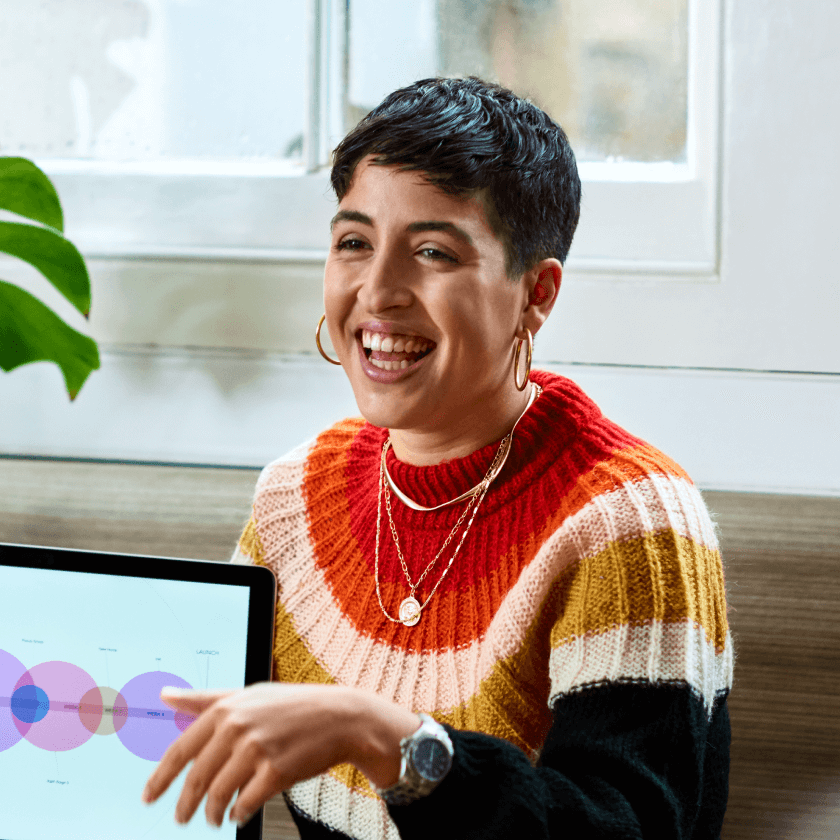