Common API Tasks🐈: Adding initials in each page of each document of your envelope
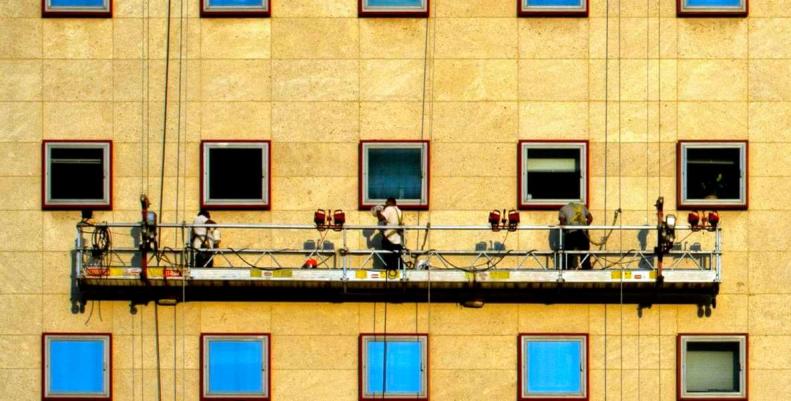
Welcome to another edition of the CAT🐈 (Common API Tasks) blog. This blog series is about giving you all you need to complete small, specific, SDK-supported tasks using one of our APIs.
You can find all articles in this series on the DocuSign developer blog.
Our task this time is pretty straightforward to explain, but maybe not as trivial to implement in your code. Assume you have a set of documents that you need to have someone initial—meaning, you just need them to put their initial on every single page of every single document. Back in ancient times (using paper), the person asking you to sign would simply indicate, “please initial on every page…”. How would you do this with DocuSign?
One option is to use DocuSign free-form signing, where the signer can then drag-and-drop their initials into each page. That option is not ideal because the user can make mistakes and might forget one of the pages, and then you’ll have to start all over again.
As a user, it’s much easier to just click through all the pages as directed by the DocuSign signing UI. To enable that, you must tag the envelope and the documents in the way you want them to be presented to the signer.
Code snippets
Before I give you the code in our usual six languages, it’s important to mention that this code assumes you already have a draft envelope with documents and recipients (and you grabbed its envelopeId, which is the GUID uniquely identifying an envelope). You may already have additional tabs (signing elements) as well, and this code won’t impact them. This code simply adds a single initial tab for each signer on each page of each document.
Note that this code makes more than one API call. It first makes an API call to get all the information about the documents and recipients in the envelope. Then, for each recipient the code adds new tabs using the EnvelopeRecipientTabs:create endpoint.
C#
// You need to obtain an access token using your chosen authentication flow var apiClient = new ApiClient(basePath);
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
EnvelopesApi.GetEnvelopeOptions options = new EnvelopesApi.GetEnvelopeOptions { include = "documents,recipients" };
Envelope env = envelopesApi.GetEnvelope(accountId, envelopeId, options);
foreach (Signer signer in env.Recipients.Signers)
{
signer.Tabs = new Tabs();
signer.Tabs.InitialHereTabs = new List<InitialHere>();
foreach (EnvelopeDocument doc in env.EnvelopeDocuments)
{
foreach (Page page in doc.Pages)
{
int width = int.Parse(page.Width);
int height = int.Parse(page.Height);
var initial = new InitialHere();
// 100 pixels higher and to the left from the top bottom corner
initial.XPosition = (width - 100).ToString();
initial.YPosition = (height - 100).ToString();
initial.PageNumber = page.Sequence;
initial.DocumentId = doc.DocumentId;
initial.RecipientId = signer.RecipientId;
signer.Tabs.InitialHereTabs.Add(initial);
}
}
envelopesApi.CreateTabs(accountId, env.EnvelopeId, signer.RecipientId, signer.Tabs);
}
Java
// You need to obtain an access token using your chosen authentication flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(config);
EnvelopesApi.GetEnvelopeOptions options = envelopesApi.new GetEnvelopeOptions();
options.setInclude("documents,recipients");
Envelope env = envelopesApi.getEnvelope(accountId, envelopeId, options);
for (Signer signer : env.getRecipients().getSigners())
{
signer.setTabs(new Tabs());
signer.getTabs().setInitialHereTabs(new java.util.List<InitialHere>());
for (EnvelopeDocument doc : env.getEnvelopeDocuments())
{
for (Page page : doc.getPages())
{
int width = Integer.parseInt(page.getWidth());
int height = Integer.parseInt(page.getHeight());
InitialHere initial = new InitialHere();
// 100 pixels higher and to the left from the top bottom corner
int x = width - 100;
int y = height - 100;
initial.setXPosition(x.toString());
initial.setYPosition(y.toString());
initial.setPageNumber(page.getSequence());
initial.setDocumentId(doc.getDocumentId());
initial.setRecipientId(signer.getRecipientId());
signer.getTabs().getInitialHereTabs().Add(initial);
}
}
envelopesApi.createTabs(accountId, env.getEnvelopeId(), signer.getRecipientId(), signer.getTabs());
}
Node.js
// You need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
let options = { include: 'documents,recipients' };
let env = envelopesApi.getEnvelope(accountId, envelopeId, options);
env.recipients.signers.forEach(signer => {
signer.tabs = new docusign.Tabs();
signer.tabs.initialHereTabs = [];
env.envelopeDocuments.forEach(doc => {
doc.pages.forEach(page => {
let initial = new docusign.InitialHere();
// 100 pixels higher and to the left from the top bottom corner
let x = page.width - 100;
let y = page.height - 100;
initial.xPosition = x;
initial.yPosition = y;
initial.pageNumber = page.sequence;
initial.documentId = doc.documentId;
initial.recipientId = signer.recipientId;
signer.tabs.initialHereTabs.push(initial);
});
});
envelopesApi.createTabs(accountId, env.envelopeId, signer.recipientId, { tabs: signer.tabs });
});
PHP
# You need to obtain an access token using your chosen authentication flow
$api_client = new \DocuSign\eSign\client\ApiClient($base_path);
$config = new \DocuSign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \DocuSign\Api\EnvelopesApi($config);
$options = new DocuSign\eSign\Api\EnvelopesApi\GetEnvelopeOptions();
$options->setInclude('documents,recipients');
$env = $envelopes_api->getEnvelope($account_id, $envelope_id, $options);
foreach ($env->getRecipients()->getSigners() as $signer)
{
$signer->setTabs(new \DocuSign\eSign\Model\Tabs());
$signer->getTabs()->setInitialHereTabs([]);
foreach ($env->getEnvelopeDocuments() as $doc)
{
foreach ($doc->getPages() as $page)
{
$initial = new \DocuSign\eSign\Model\InitialHere();
# 100 pixels higher and to the left from the top bottom corner
$x = $page->getWidth() - 100;
$y = $page->getHeight() - 100;
$initial->setXPosition($x);
$initial->setYPosition($y);
$initial->setPageNumber($page->getSequence());
$initial->setDocumentId($doc->getDocumentId());
$initial->setRecipientId($signer->getRecipientId());
array_push($signer->getTabs()->getInitialHereTabs(), $initial);
}
}
$envelopes_api->createTabs($account_id, $env->getEnvelopeId(), $signer->getRecipientId(), $signer->getTabs());
}
Python
# You need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.host = base_path
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
env = envelopes_api.getEnvelope(account_id, envelope_id, include = 'documents,recipients')
for signer in env.recipients.signers :
signer.tabs = Tabs()
signer.tabs.initial_here_tabs = []
for doc in env.envelope_documents :
for page in doc.pages :
initial = InitialHere()
# 100 pixels higher and to the left from the top bottom corner
x = page.width - 100
y = page.height - 100
initial.x_position = x
initial.y_position = y
initial.page_number = page.sequence
initial.document_id = doc.document_id
initial.recipient_id = signer.recipient_id
signer.tabs.initial_here_tabs.insert(initial)
envelopes_api.create_tabs(account_id, env.envelope_id, signer.recipient_id, signer.tabs)
Ruby
# You need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
options = DocuSign_eSign::GetEnvelopeOptions.new
options.include = 'documents,recipients'
env = envelopes_api.getEnvelope(account_id, envelope_id, options)
env.recipients.signers.each { |signer|
signer.tabs = DocuSign_eSign::Tabs.new
signer.tabs.initial_here_tabs = []
env.envelope_documents.each { |doc|
doc.pages.each { |page|
initial = InitialHere()
# 100 pixels higher and to the left from the top bottom corner
x = page.width - 100
y = page.height - 100
initial.x_position = x
initial.y_position = y
initial.page_number = page.sequence
initial.document_id = doc.document_id
initial.recipient_id = signer.recipient_id
signer.tabs.initial_here_tabs.append(initial)
}
}
envelopes_api.create_tabs(account_id, env.envelope_id, signer.recipient_id, signer.tabs)
}
That's it folks. As usual, if you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time…