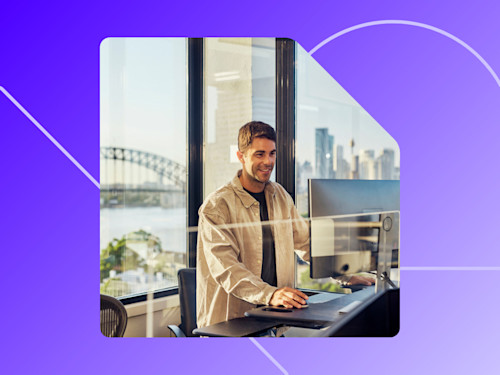
Common API Tasks🐈: Adding a contact to your Docusign address book
See how to use the eSignature REST API through your chosen SDK to add a contact to your Docusign address book.
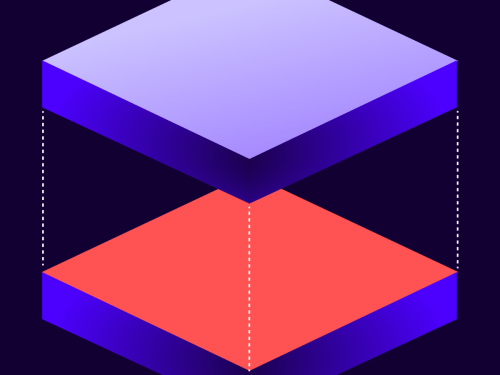
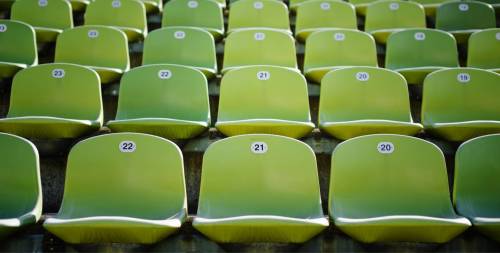
Welcome to an amazing new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
Did you know that Docusign has an address book? Your Docusign account includes your very own personal address book that can be used to store frequent contact information. To see your address book select the My Preferences option from the top-left menu and then select Contacts from the left navigation menu. You can find two types of contacts there: other users in your account, which we will not discuss today, and address book contacts: these can be shared with other members of your Docusign account, or they can be personal contacts for your use only. These contacts can be added from the web application; but as we always do in this blog series, I’m going to show you how to add a contact programmatically.
Note: These contacts will be used when you send an envelope in the web app and start typing recipient names and email addresses. The web app will make suggestions of contacts that match what you type to make it quicker and easier for you to fill the recipients for an envelope.
The code snippets below add a new contact to your address book. In addition to an email address, you can add one or more phone numbers, which can be used for things like SMS delivery.
C#
var apiClient = new ApiClient(basePath);
// You will need to obtain an access token using your chosen authentication method
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
UsersApi usersApi = new UsersApi(apiClient);
Contact contact = new Contact();
contact.Name = "Inbar Gazit";
contact.Emails = new List<string>();
contact.Emails.Add("inbar.gazit@docusign.com");
contact.Organization = "Docusign";
ContactPhoneNumber contactPhoneNumber = new ContactPhoneNumber();
contactPhoneNumber.PhoneNumber = "212-555-1234";
contactPhoneNumber.PhoneType = "mobile";
contact.ContactPhoneNumbers = new List<contactphonenumber>();
contact.ContactPhoneNumbers.Add(contactPhoneNumber);
ContactModRequest contactModificationRequest = new ContactModRequest();
contactModificationRequest.ContactList = new List<contact>();
contactModificationRequest.ContactList.Add(contact);
usersApi.PostContacts(accountId, contactModificationRequest);
</contact></contactphonenumber></string>
Java
// You will need to obtain an access token using your chosen authentication flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
UsersApi usersApi = new UsersApi(config);
UserProfile userProfile = new UserProfile();
Contact contact = new Contact();
contact.setName("Inbar Gazit");
contact.setEmails(new java.util.ArrayList<string>());
contact.getEmails().add("inbar.gazit@docusign.com");
contact.setOrganization("Docusign");
ContactPhoneNumber contactPhoneNumber = new ContactPhoneNumber();
contactPhoneNumber.setPhoneNumber("212-555-1234");
contactPhoneNumber.setPhoneType("mobile");
contact.setContactPhoneNumbers(new java.util.ArrayList<contactphonenumber>());
contact.getContactPhoneNumbers().add(contactPhoneNumber);
ContactModRequest contactModificationRequest = new ContactModRequest();
contactModificationRequest.setContactList(new java.util.ArrayList<contact>());
contactModificationRequest.getContactList().add(contact);
usersApi.postContacts(accountId, contactModificationRequest);
</contact></contactphonenumber></string>
Node.js
// You will need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
UserApi usersApi = new docusign.UsersApi(apiClient);
let contact = new Contact();
contact.name = 'Inbar Gazit';
contact.emails = ['inbar.gazit@docusign.com'];
contact.organization = 'Docusign';
let contactPhoneNumber = new docusign.ContactPhoneNumber();
contactPhoneNumber.phoneNumber = '212-555-1234';
contactPhoneNumber.phoneType = 'mobile';
contact.contactPhoneNumbers = [contactPhoneNumber];
let contactModificationRequest = new docusign.ContactModRequest();
contactModificationRequest.contactList = [contact];
usersApi.postContacts(accountId, contactModificationRequest);
PHP
# You will need to obtain an access token using your chosen authentication flow
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$users_api = new \Docusign\eSign\Api\UsersApi($api_client);
$contact = new Contact();
$contact->setName('Inbar Gazit');
$contact->setEmails(['inbar.gazit@docusign.com']);
$contact->setOrganization('Docusign');
$contact_phone_number = new \Docusign\eSign\Model\ContactPhoneNumber();
$contact_phone_number->setPhoneNumber('212-555-1234');
$contact_phone_number->setPhoneType('mobile');
$contact->setContactPhoneNumbers([$contact_phone_number]);
$contact_modification_request = new \Docusign\eSign\Model\ContactModRequest();
contactModificationRequest->setContactList([$contact]);
$users_api->postContacts($account_id, $contact_modification_request);
Python
# You will need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
users_api = UsersApi(api_client)
contact = Contact()
contact.name = 'Inbar Gazit'
contact.emails = ['inbar.gazit@docusign.com']
contact.organization = 'Docusign'
contact_phone_number = ContactPhoneNumber()
contact_phone_number.phone_number = '212-555-1234'
contact_phone_number.phone_type = 'mobile'
contact.contact_phone_numbers = [contact_phone_number]
contact_modification_request = ContactModRequest()
contact_modification_request.contact_list = [contact]
users_api.post_contacts(account_id, contact_modification_request)
Ruby
# You will need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
users_api = DocuSign_eSign::UsersApi.new api_client
contact = DocuSign_eSign::Contact.new
contact.name = 'Inbar Gazit'
contact.emails = ['inbar.gazit@docusign.com']
contact.organization = 'Docusign'
contact_phone_number = DocuSign_eSign::ContactPhoneNumber.new
contact_phone_number.phone_number = '212-555-1234'
contact_phone_number.phone_type = 'mobile'
contact.contact_phone_numbers = [contact_phone_number]
contact_modification_request = DocuSign_eSign::ContactModRequest.new
contact_modification_request.contact_list = [contact]
users_api.post_contacts(account_id, contact_modification_request)
That’s all folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
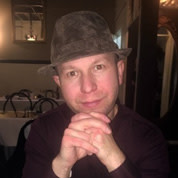
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
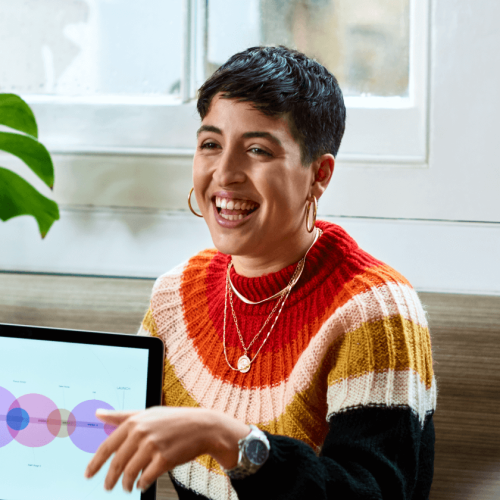