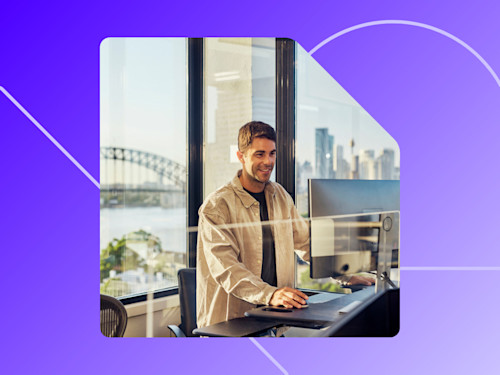
Building a React app, Part 2: OAuth information security
This mutipart series show you how to use the Docusign eSignature REST API in a React app. Part 2 covers authentication and information security.
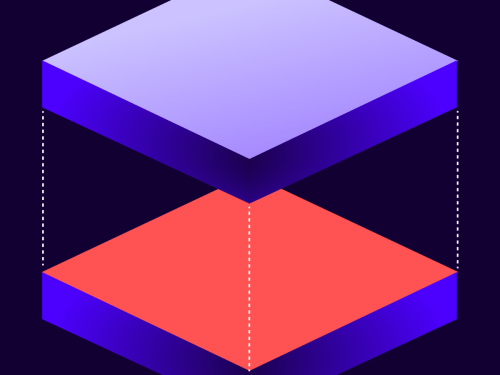
Thanks for visiting this older post. If you’re interested in CORS access to the eSignature REST API, check out its new CORS feature:
The original post:
In this multipart series, I’m covering the steps needed to add the Docusign API to a React app, including two patterns for integrated OAuth authentication.
See Part 1: Introduction for more information.
Authentication grant flow
The Docusign eSignature REST API is sessionless: every API request must include an access token. Your application obtains an access token via one of the OAuth v2.0 grant flows supported by Docusign. OAuth v2.0 is defined by multiple standards and recommendations documents. RFC 6749 is the OAuth 2.0 Core specification.
RFC 6819 recommends security practices for OAuth and should always be followed. The most common OAuth pattern is a web application in which the application runs on a secured web server. These types of web apps commonly use the OAuth Authorization Code Grant flow.
RFC 6749 § 2.1 defines public and confidential clients. Clients that are locally installed (downloaded to your computer or mobile) and web browser-based applications (including React apps) are public clients and cannot be used to store OAuth secrets or private keys; see RFC 6819 § 5.2.3.1. Docusign supports the Implicit Grant flow for public clients.
Docusign’s Authorization Code and JWT grant flows cannot be used with public clients, because they require the client to safeguard a secret key. Confidential clients include server-side authentication code, Platform as a Service code (such as AWS Lambda), and other secure cloud services. Authorization Code and JWT grant flows should be used with confidential clients.
Preventing CSRF attacks
The Identity Provider (IdP) is the server that issues access tokens and manages identities. For Docusign, account-d.docusign.com is the IdP for the developer account (demo) system. RFC 6819 § 5.3.5 recommends that the OAuth grant flow’s state value should be used to prevent CSRF attacks. To do so, a random value is sent to the IdP as the state value in the first step of the OAuth grant flow. Later, the response from the IdP will also contain the state value, and the client checks that the two values match.
Preventing clickjacking attacks
To prevent clickjacking attacks, RFC 6819 § 4.4.1.9 recommends that the authentication dialog between the user and the IdP not be allowed in an iframe. Docusign IdPs implement this restriction. Therefore, application developers have two options:
The application opens a new browser tab, which is then used for Docusign authentication. Since the application itself opened the new tab, it is also able to programmatically close the tab when it’s no longer needed.
When the application starts the OAuth flow, it redirects the browser to the OAuth IdP. For a browser-based application, this means that the application is stopped and its code is discarded by the browser when the browser loads the OAuth URL from the IdP. Later, when the IdP redirects the browser back to the application, it will be restarted on the browser.Before the app redirects to the IdP, the app can store its state information in the browser using cookies or the Web Storage API.
Both patterns of opening a new tab for the IdP and redirecting to the IdP work well. The React example implements both techniques; see the ex\ample’s configuration setting DS_REDIRECT_AUTHENTICATION
.
Supporting SSO
One of the many advantages of OAuth is that SSO (Single Sign-on) is automatically supported by the Authorization Code and Implicit grant flows. As a friend of mine says, it’s neat-o-matic! When someone authenticates, the IdP (account-d.docusign.com or account.docusign.com) enables the user to select Use Company Login. This prompts Docusign’s IdP to look up the upstream IdP (the SSO server) associated with the claimed domain name of the user’s email address. The user then completes the authentication flow with the SSO server. No application changes or settings are needed to use the SSO flow.
Conclusion
To keep an OAuth implementation secure, RFC 6819 should be followed. It adds some restrictions to your application, but they are all minor.
In the next part of this series, I’ll discuss some of the software patterns used in the example React app itself.
Additional resources
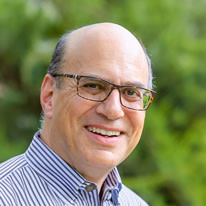
Larry Kluger has over 40 years of tech industry experience as a software developer, developer advocate, entrepreneur, and product manager. An award-winning speaker prominent StackOverflow contributor, he enjoys giving talks and helping the ISV and developer communities.
Twitter: @larrykluger
LinkedIn: https://www.linkedin.com/in/larrykluger/
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
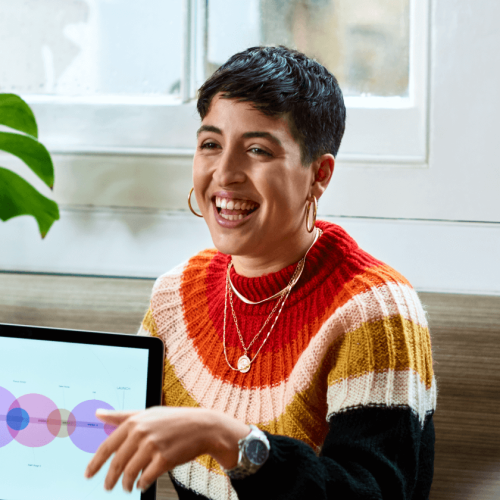