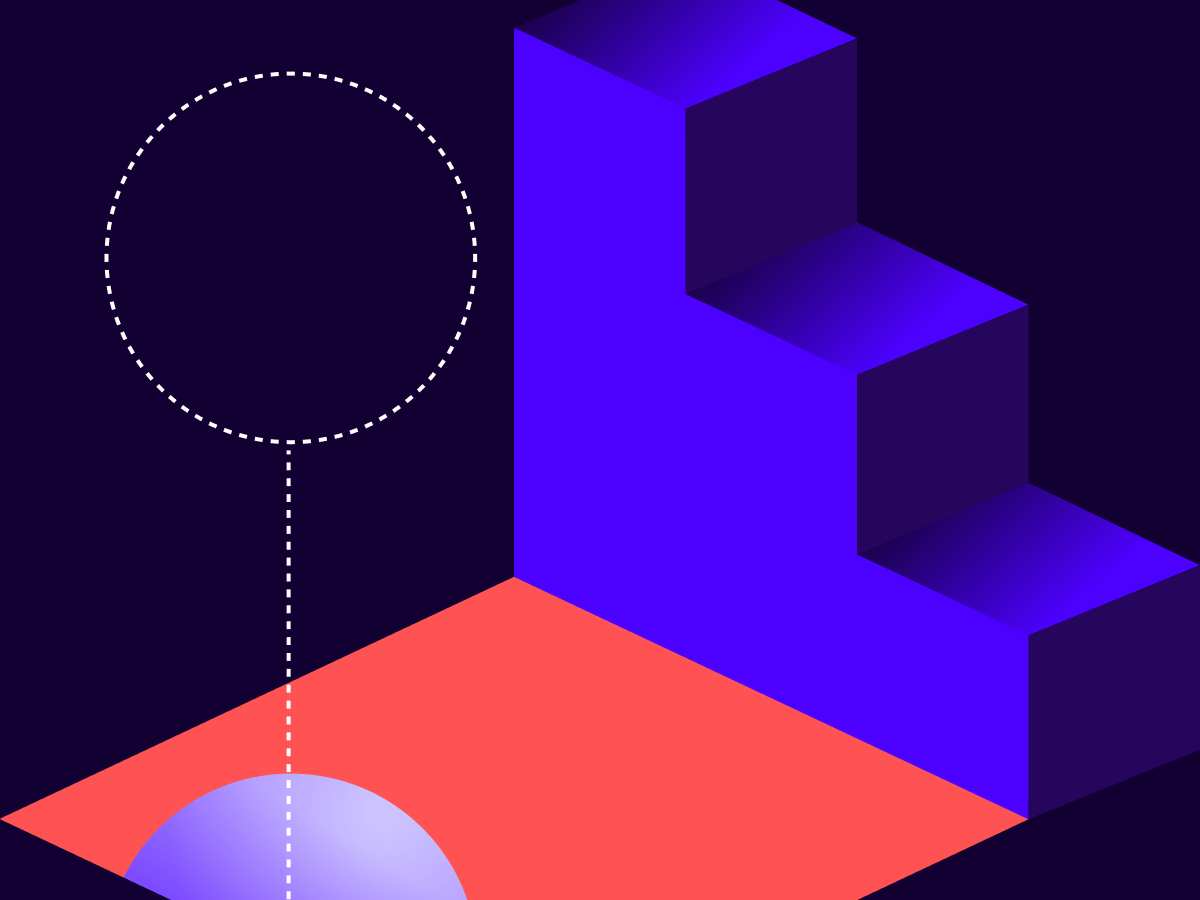
Building a React app, Part 3: The code example
Dig into the React app code example to see how to incorporate the Docusign API.
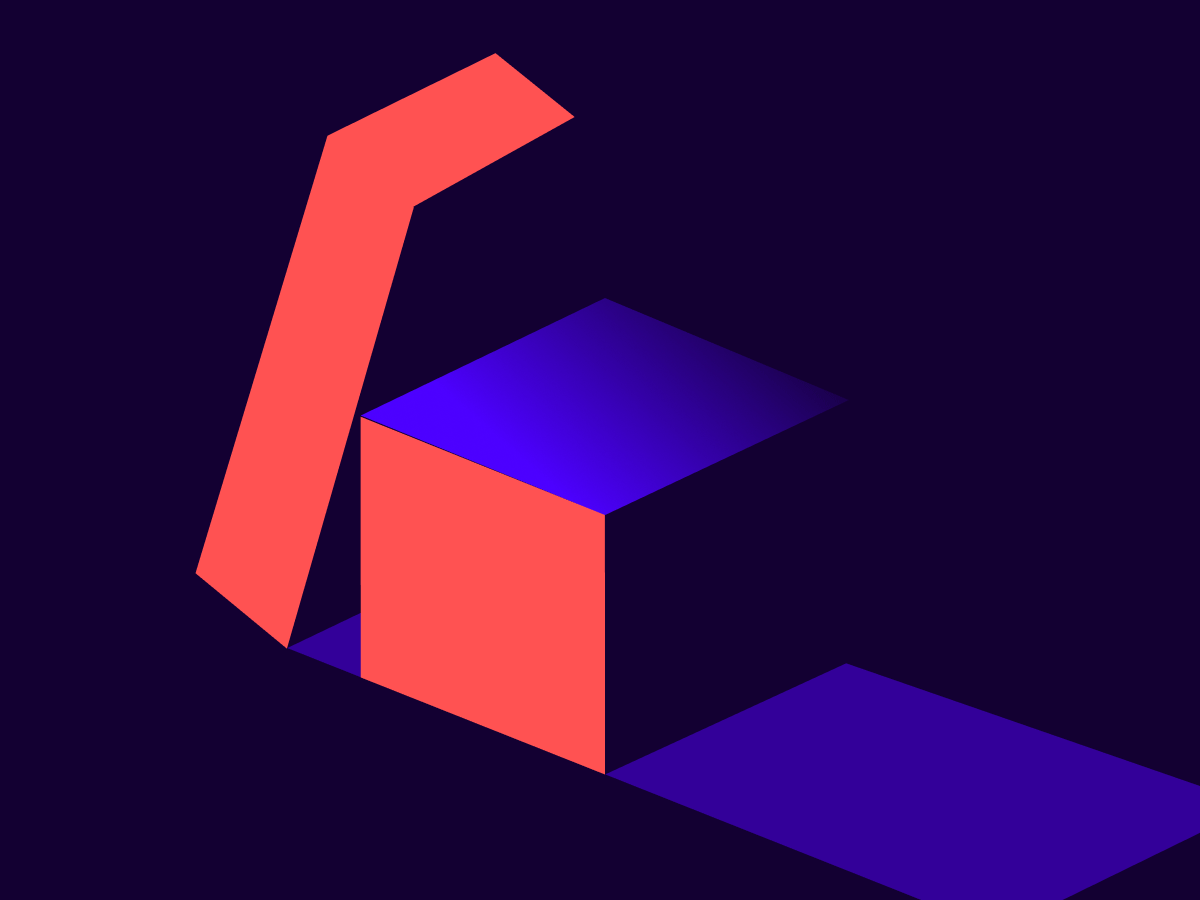
Thanks for visiting this older post. If you’re interested in CORS access to the eSignature REST API, check out its new CORS feature:
The original post:
In this multipart series, I’m covering the steps needed to add the Docusign API to a React app, including two patterns for integrated OAuth authentication.
See Part 1: Introduction, and Part 2: OAuth Information Security.
The React code example
The code example is available from the GitHub repository code-examples-react. The example was started from the React team’s Create React App toolchain. The application has not been ejected, so you can easily update the application’s libraries. The app uses the MIT license.
Follow the example’s Readme file to install, configure, and start the example.
Redirecting to the IdP
When the configuration setting DS_REDIRECT_AUTHENTICATION
is true
, the application will redirect its browser window to the Identity Provider (IdP) for authentication. account.docusign.com is the IdP for production accounts; account-d.docusign.com is for developer accounts on the demo platform. See the startLogin
method of the src/OAuthImplicit.js file.
Later, after the user has authenticated, the IdP redirects the user’s browser back to the app’s URL and the app is restarted. But the application’s URL now has a fragment indicator (a # followed by data) added by the IdP. The fragment indicator’s data is composed of the access token, expires in, and OAuth state fields.
Whenever the app is started, the componentDidMount
method in the file src/App.js checks to see if there is data in the fragment section of the URL. If there is, it is processed as a potential reply from the IdP in the receiveHash
method of the src/OAuthImplicit.js file.
Seeing the application’s URL with the long fragment data is boring, and can mess up subsequent redirects. So I reset the URL in the browser to the app’s URL:
window.history.replaceState(null, '', config.DS_APP_URL);
Opening a new tab for authentication
For some applications, it is best to open a new tab for the OAuth authentication process. This way the original application continues to run in its original browser tab, so there’s no need to save state.
You can set the React code example to open a new tab for authentication by setting the configuration item DS_REDIRECT_AUTHENTICATION
to false
.
Browsers only allow an application to create and destroy a browser tab if the tab was created as a direct result of a user’s action, such as clicking a button or link. For the example app, the user initiates the authentication flow by clicking a button. Next, the startLogin
method of the src/OAuthImplicit.js file creates a new tab and loads it with the URL for the authentication flow at the IdP to start the authentication process:
this.oauthWindow = window.open(url, "_blank");
Also note that the IdP is given a different return URI: the file public/oauthResponse.html. Later, the IdP redirects the browser back to the return URI (to the oauthResponse.html web page) and includes the access token in the URL’s fragment section as described above.
The JavaScript in the oauthResponse.html web page transmits the URL’s fragment content to the parent app by using the postMessage method of the page’s parent window, window.opener
. Previously, the method componentDidMount
in the file src/App.js (in the application itself) had used window.addEventListener
to register a listener for messages from the oauthResponse.html web page.
Once the fragment information has been received and processed, the browser tab is closed by the receiveHash
method of the src/OAuthImplicit.js file:
if (this.oauthWindow) {this.oauthWindow.close()}
OAuth/UserInfo and calling the eSignature REST API
After the user is authenticated, the fetchUserInfo
method of the src/OAuthImplicit.js file makes the OAuth/UserInfo call to the IdP to obtain the authenticated user’s name, email, account, and other information. This API call uses CORS, which is supported by the IdP. The default account’s base_uri
is used to choose which private CORS proxy should be used for subsequent eSignature REST API calls.
The methods for calling the eSignature REST API to create an envelope and get its status are in the file src/Docusign.js. The API is called via your private CORS proxy. See this post, which discusses how to configure the Apache server to be a CORS proxy. In addition, I’ve written and tested a CORS proxy configuration file for the popular Nginx web server.
Conclusion
The code example demonstrates how a React browser-based application can:
authenticate with Docusign
retrieve the authenticated user’s information via oauth/userinfo
use the eSignature REST API via a private CORS proxy
and close the authenticated session (logout)
Additional resources
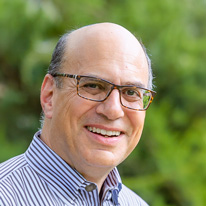
Larry Kluger has over 40(!) years of tech industry experience as a software developer, developer advocate, entrepreneur, and product manager. An award-winning speaker with a 48K StackOverflow reputation, he enjoys giving talks and helping the ISV and developer communities.
Twitter: @larrykluger
LinkedIn: https://www.linkedin.com/in/larrykluger/
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
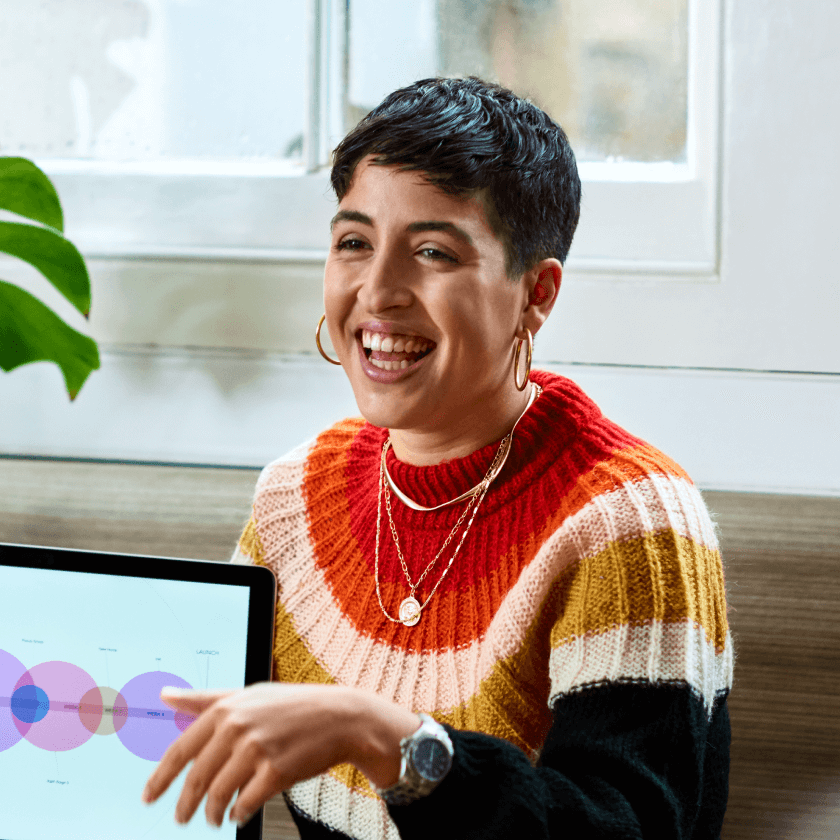