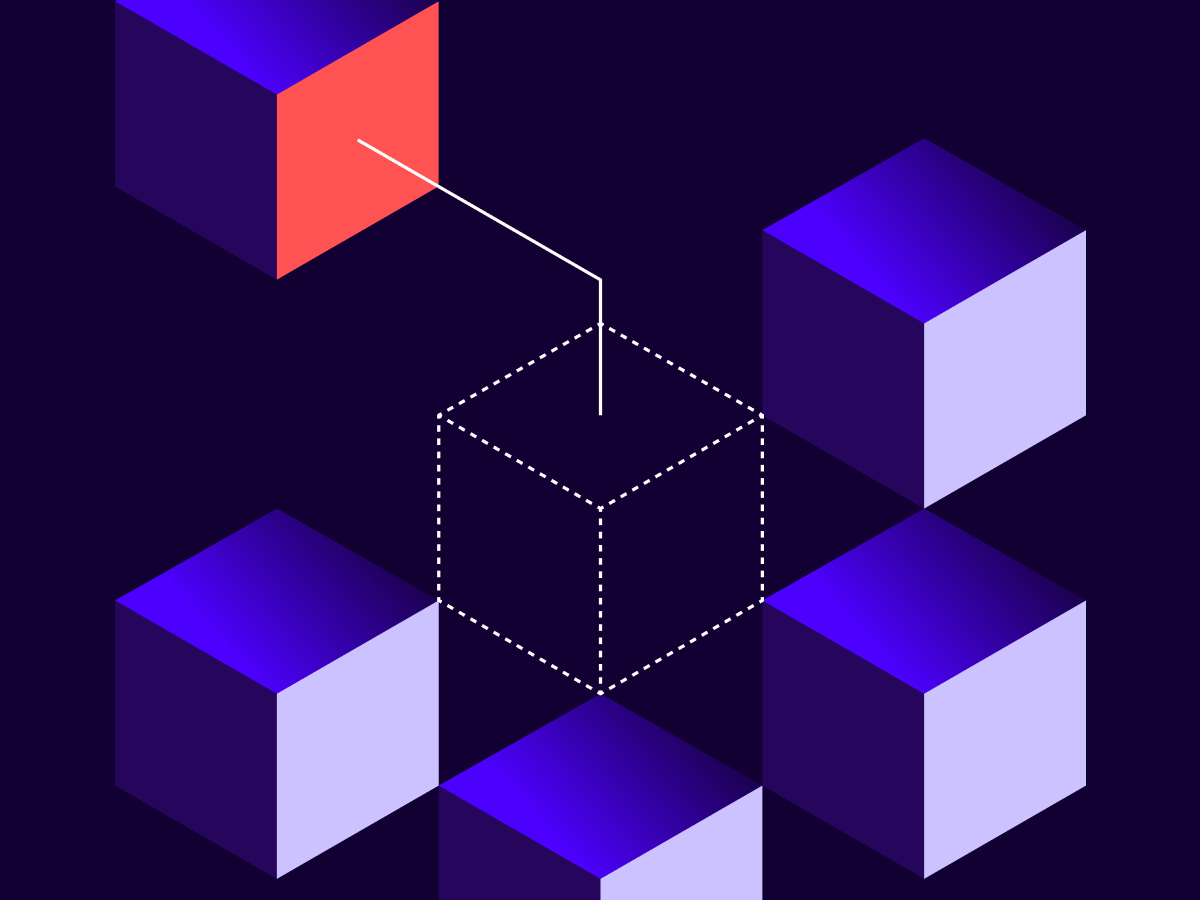
What’s new in Summer ’21 with Apex Toolkit
Our Summer '21 release offers new features that give you greater control over envelopes, documents, and delivery.
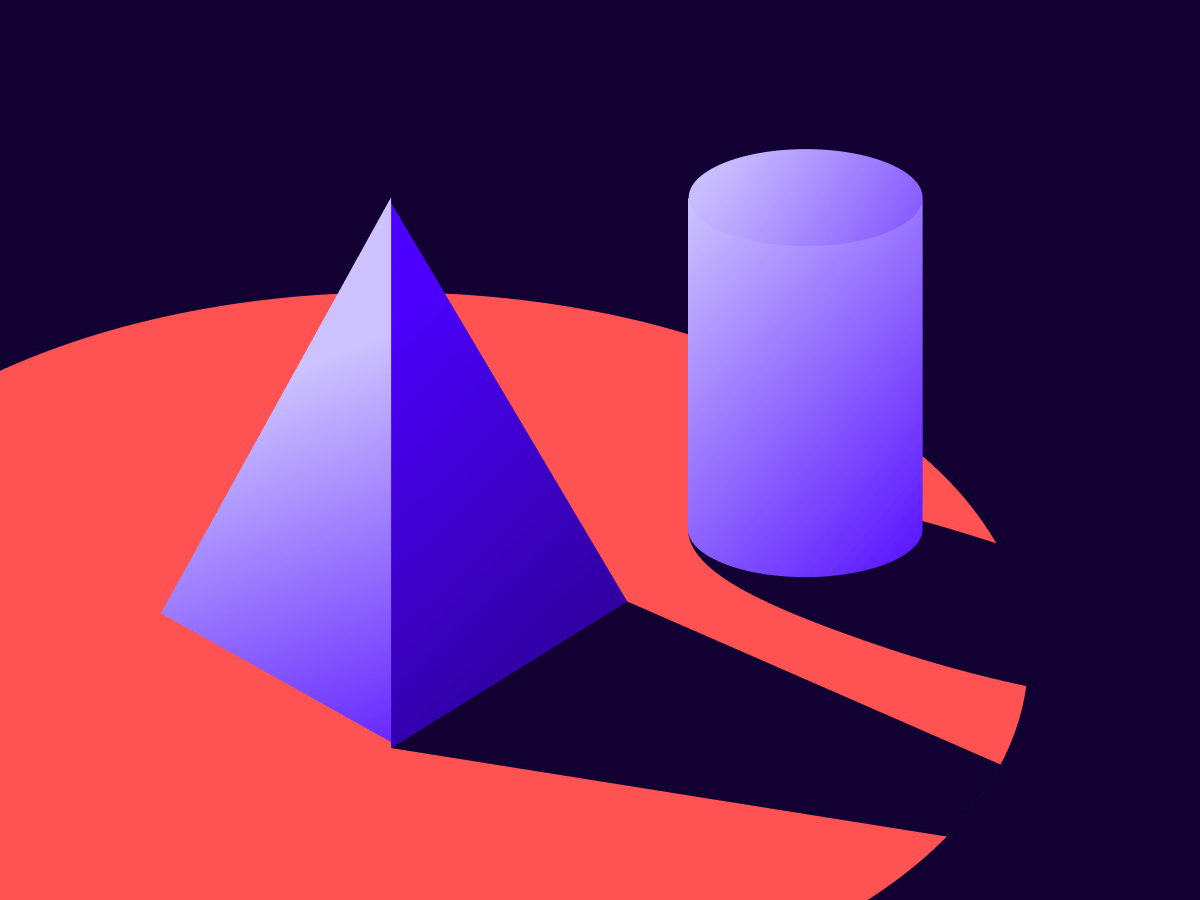
With the release of Docusign Apps Launcher Version 3.3 this summer, we’ve added a handful of new features to the Apex Toolkit. From configuring writeback options to replacing template documents, you can even send an envelope to recipients via SMS—all using the Apex Toolkit!
What is Apex Toolkit?
Apex Toolkit is a set of programmatic functions and objects that you can call in your Apex code to make use of Docusign-related features, such as configuring and sending an envelope. The toolkit is available through the Docusign Apps Launcher, a managed package available on the Salesforce AppExchange. To get started, check out How to install the Apex Toolkit on the Docusign Developer Center.
Document and data writeback
Prior to Docusign Apps Launcher 3.3, the only way to control writeback options was to use an envelope template. Now, you can directly control these options with the Apex Toolkit. We’ve exposed the dfsle.Envelope.withOptions
method, which controls default auto-place tag placement, document writeback, and data writeback on various envelope and recipient-level events.
For example, to write back completed documents and update the Opportunity stage when an envelope is completed, you can use the following code:
myEnvelope.withOptions(new dfsle.Envelope.Options(
// Whether to populate auto-place tags for default roles
false,
new dfsle.Document.WriteBack(
// Where to link the completed document(s)
opportunityId,
// The completed document(s) file name format
dfsle.Document.WRITE_BACK_ENVELOPE_STATUS_PDF,
// Whether to combine all the documents into a single PDF
true,
// Whether to include the Certificate of Completion (CoC)
true),
// Envelope status event updates
new Map<string map object>> {
// Set Opportunity.StageName to "Closed Won" upon completion
dfsle.Envelope.STATUS_COMPLETED => new Map<string object> {
'Opportunity.StageName' => 'Closed Won'
}
},
// Recipient status event updates
new Map<string map object>>()));
</string></string></string>
You’re not limited to the number of fields you can update, but we recommend that you update a minimal number of fields using this method and leverage standard Salesforce features such as Process Builder to implement more complex updates like creating new records.
SMS delivery
The SMS delivery feature allows envelopes to be sent to recipients via text message, enhancing the way documents can be sent and accessed for speeding up business transactions. This feature can now be used with Apex toolkit!
When using the SMS delivery feature, keep in mind its feature limitations. These will come in handy when troubleshooting any issues you might run into.
In your Docusign Apps Launcher installation, you’ll need to connect a Docusign developer account with the SMS delivery feature enabled to use SMS delivery in Apex Toolkit. Check out SMS delivery on the Docusign Developer Center to find out how to enable this feature for your account.
Once you’ve connected your SMS-enabled account to Docusign Apps Launcher, you’re ready to get started!
SMS delivery can be configured by simply applying the dfsle.Recipient.withSmsDelivery
method, like this:
myRecipient.withSmsDelivery(myRecipient.phone);
The function takes in a single String
argument representing the phone number of the recipient who will receive the envelope via SMS. For SMS delivery to be properly set to a recipient, the value must be a non-empty string and align with the format:
+_countryCode phoneNumber_
where the substring _countryCode_
is prefixed with a ‘+’ and separated from the rest of the value, _phoneNumber_
, by a space. Here are some examples of phone values that are considered valid:
9255550123 (Note: Country code defaults to +1.)
+1 9255550123
+1 925-555-0123
+1 (925)-555-0123
Once you’ve applied dfsle.Recipient.withSmsDelivery
to the desired recipient(s), all that’s left would be to attach the recipient(s) to an Envelope
object instance and then send the envelope for signature. For example:
for (dfsle.Recipient r : myRecipients) {
r.withSmsDelivery(r.phone); // Set SMS delivery
}
envelope.withRecipients(myRecipients);
EnvelopeService.sendEnvelope(envelope, true);
When you run your code to send the envelope, the recipient(s) you’ve set SMS delivery for will receive it via text message. They can then access and sign the envelope on their phones.
That’s it! Now you know how to use the Apex Toolkit to send envelopes via SMS.
Replacement documents
When using Docusign templates, you can now replace their documents using the Apex Toolkit. This is useful for when you have dynamic document versions and want to retain the tags set in the Docusign template. Right now this is restricted to a single document replacement per template. To replace a template document, use the dfsle.Document.withReplacement
method.
For example, to replace a template document with a newly-generated ContentVersion
, you can specify the following code:
myTemplate.withReplacement(dfsle.Document.fromFile(myGeneratedFile));
Supplemental documents
You can now specify supplemental documents in your code. Supplemental documents are used to provide additional information to recipients that don’t require a signature, such as legal disclosures or terms and conditions. To mark a document as supplemental, use the dfsle.Document.withSupplementalOptions
method. For example:
myDocument.withSupplementalOptions(new
dfsle.Document.SupplementalOptions(
// Signer must view and accept this document
dfsle.Document.SIGNER_ACKNOWLEDGEMENT_VIEW_ACCEPT,
// Include this document in the combined document download.
true));
PDF form field transformation
If you want to convert PDF form fields automatically to Docusign tabs, the Apex Toolkit has got you covered. PDF form field transformation is now possible using the dfsle.Document.withPdfOptions
method.
You can use the following code to transform PDF form fields and assign them to the first envelope recipient, like this:
myDocument.withPdfOptions(new dfsle.Document.PdfOptions(
// Whether to transform PDF form fields.
true,
// The recipient for which to apply the tags
1));
Conclusion
The Apex Toolkit enables developers to use Docusign’s eSignature API for their custom Salesforce implementation. The latest update provides more options around how to configure envelopes, including writeback, documents, and SMS delivery. Want to learn more about the Apex Toolkit? Check out some of our other Apex Toolkit blog posts with code examples, such as Sending a Docusign Template with Apex Toolkit and New support for tabs in the Apex Toolkit.
Additional resources
Rafaeli has been working at Docusign since 2019. As a software engineer on the Salesforce Integration team, she contributes to developing features on Docusign Apps Launcher, a managed package of Docusign products available through the Salesforce AppExchange.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
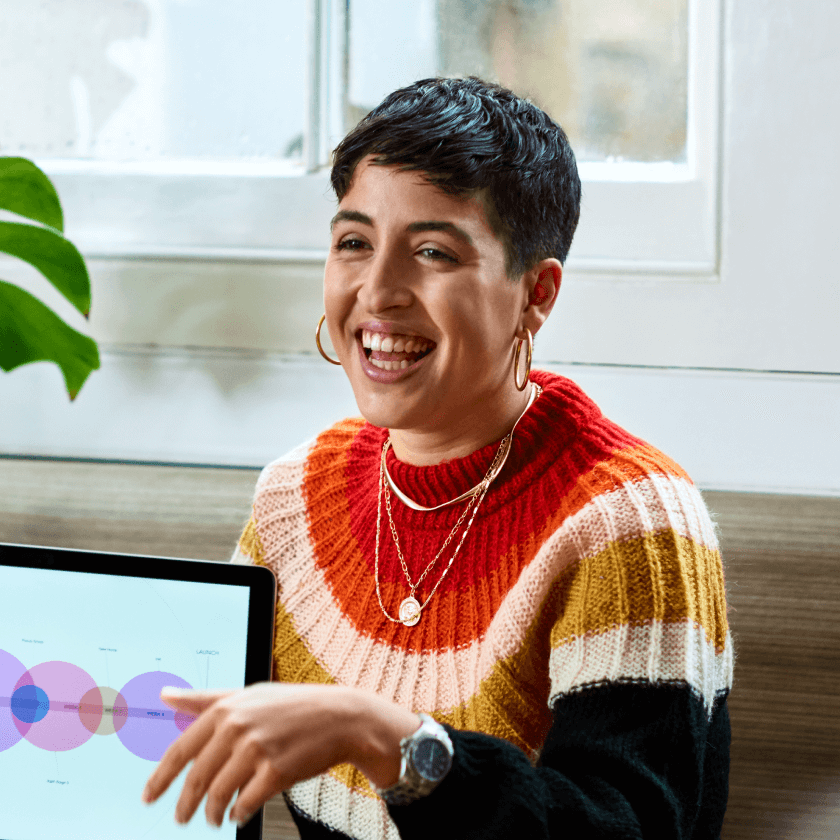