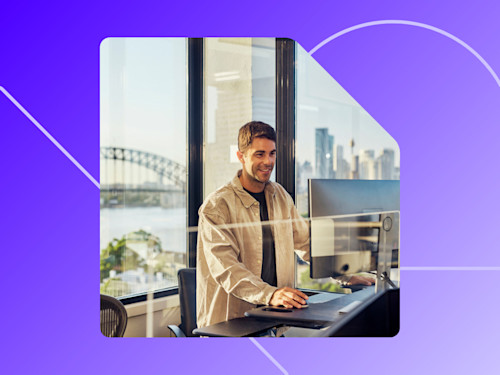
From the Trenches: Automate resending email notifications after updates
Docusign lets you send a corrected envelope when you need to update the document or tabs. See how to automate this process through the eSignature REST API.
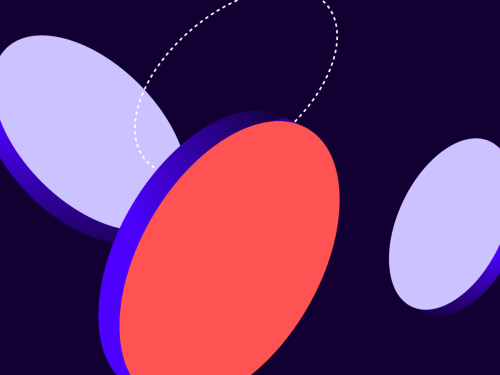
Let’s suppose you send an envelope with the wrong document attached, or an incorrect tab (field) applied. When this happens, you may want to update the envelope and resend the email notification to the recipients to inform them that the envelope is updated.
Docusign provides multiple types of email notifications to inform the recipients of any updates in the envelope. However, the notifications by design will not be sent when correcting an envelope to replace or add a document or tabs. Therefore, you need to explicitly send the notification to the recipients once the documents or tabs are corrected.
If you don’t use the eSignature REST API, you can resend the email notification in the Docusign UI once you update the envelope. This blog post, however, will show you how you can automate resending the email notification via the API when you update a document or tab.
Workflow: Update the document/field and resend the email notification
Lock the envelope: Since you already sent the envelope to the recipient, the recipient and sender can access the envelope at the same time, which might cause unexpected behavior. To prevent this, Docusign recommends locking the envelope prior to making any changes. A lock is used to control access to the envelope: it allows exclusive access to a single party at a time to avoid unexpected behavior, such as a conflict on data updates.
You can lock the envelope via EnvelopeLocks:create. If you use a Docusign SDK, refer to the code implementation introduced in Inbar Gazit’s blog post, Common API Tasks🐈: Locking and unlocking envelopes.
curl --location '{baseUrl}/v2.1/accounts/{accountId}/envelopes/{envelopeId}/lock' \ --header 'Authorization: Bearer eyJ0eX...LHeNDw' \ --data '{ "lockType": "edit", "lockDurationInSeconds": "600" }'
Update the document or tab: Once you lock the envelope, it’s time to update the document or field. I’m only showing you how to update the document. If you want to update a field, just replace the API used in this step with the EnvelopeRecipientTabs:update API call.You can update the document via EnvelopeDocuments:update. Since you locked the envelope, you need to specify the X-Docusign-Edit header, which proves you are the owner of the lock, and provide the lock token you received from the EnvelopeLocks:create API call.
curl --location --request PUT '{baseUrl}/v2.1/accounts/{accountId}/envelopes/{envelopeId}/documents/{documentId}' \ --header 'Authorization: Bearer eyJ0eX...LHeNDw' \ --header 'X-DocuSign-Edit: {"LockToken": "{LOCK_TOKEN}", "LockDurationInSeconds": "600"}' \ --header 'Content-Type: application/pdf' \ --header 'Content-Disposition: filename="newDocument"' \ --data-binary '@/location/of/document.pdf'
Unlock the envelope: Once you finish updating the document, unlock the envelope via EnvelopeLocks:delete.
curl --location --request DELETE '{baseUrl}/v2.1/accounts/{accountId}/envelopes/{envelopeId}/lock' \ --header 'Authorization: Bearer eyJ0eX...LHeNDw' \ --header 'X-DocuSign-Edit: {"LockToken": "{LOCK_TOKEN}", "LockDurationInSeconds": "600"}'
Send the email notification: As the document is now updated, use EnvelopeRecipients:update to send the email notification to the recipient. Set the resend_envelope query parameter to true and specify the recipient in the request body to send the email notification. The recipient information can be obtained via EnvelopeRecipients:list.
Note that the email subject and body cannot be changed once the envelope is sent. However, leaving a private message to each recipient is possible by defining a note property in the request body. Once the private message is defined, the recipient can see it in the email notification.
curl --location --request PUT '{baseUrl}/v2.1/accounts/{accountId}/envelopes/{envelopeId}/recipients?resend_envelope=true' \ --header 'Authorization: Bearer eyJ0eX...LHeNDw' \ --header 'Content-Type: application/json' \ --data-raw '{ "signers": [ { "recipientId": "1", "name": "Bob", "email": "bob@exmaple.com", "note": "The document is updated." } ] }'
If you want to send the email notification to all pending recipients (anyone who's yet to come in the routing order and hasn't yet completed the envelope), you may choose to use Envelopes:update, as it is simpler and easier. All you need to do is specify the resend_envelope query parameter as true with the empty object in the request body.
curl --location --request PUT '{baseUrl}/v2.1/accounts/{accountId}/envelopes/{envelopeId}?resend_envelope=true' \ --header 'Authorization: Bearer eyJ0eX...LHeNDw' \ --header 'Content-Type: application/json' \ --data '{}'
Now your recipients will see another email notification of this envelope with the updated document. As a matter of fact, the recipient can see the updated document by accessing the envelope through the original email notification. Therefore, the purpose of resending the email notification is just to inform the recipient again that the envelope is waiting for their signature.
What about the “The sender corrects an envelope” option?
As I mentioned at the start of this post, you can control the email notification in Docusign eSignature Settings. Among these options, you can configure the The sender corrects an envelope option. When this option is enabled, Docusign sends an email notification like the image below when the recipient’s email is changed. The original email recipient will be notified that they no longer have access when they click REVIEW DOCUMENTS in the original email.
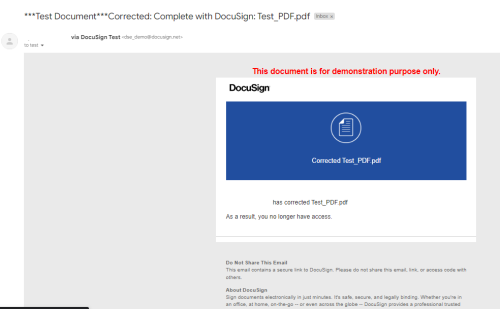
Thus, even though this option is enabled, no new notifications will be sent automatically when you correct an envelope to replace or add a document or fields.
Additional resources
Byungjae Chung has been a Developer Support Engineer for Docusign since 2022. He specializes in helping developers resolve issues they encounter when developing applications using Docusign APIs. You can reach Byungjae on LinkedIn.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
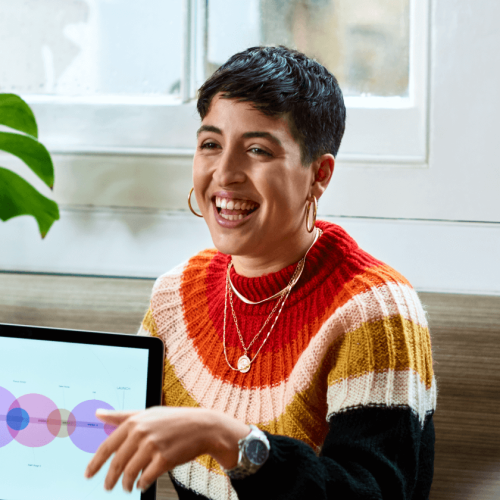