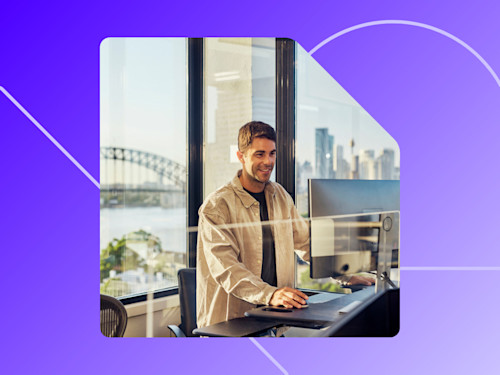
Pro tips for effectively using Docusign APIs
Follow these tips to build better performing, more reliable, and more secure Docusign integrations.
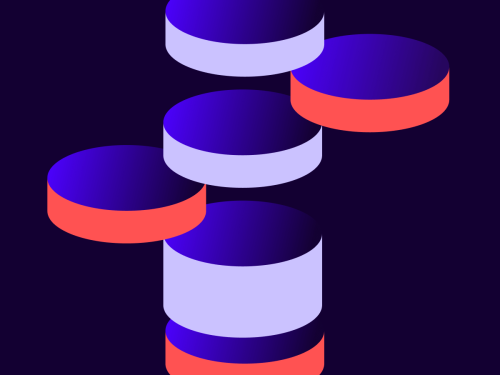
Docusign APIs provide developers with powerful tools to integrate electronic signature functionality into their applications. To ensure a smooth and secure integration process, it's important to follow best practices. This blog post includes some valuable tips on how to use Docusign APIs effectively, including structuring requests, handling errors, and securing your data. Code samples are provided to illustrate the recommended practices.
API basics
Before diving into the APIs, familiarize yourself with the basics of the Docusign platform and its terminologies. Understanding the platform’s core concepts, such as envelopes, recipients, tabs, and templates, will make your interactions with the APIs smoother and more productive.
The Docusign APIs, including the eSignature REST API, require all API calls to use TLS (make sure you check the TLS version currently supported by Docusign).
All Docusign REST APIs require developers to use an OAuth v2 flow for authentication. Docusign supports the Authorization Code Grant, Implicit Grant, and JWT Grant flows.
The Docusign eSignature API supports CORS, enabling you to create single-page applications (SPAs), which make API calls directly to Docusign from the browser. A set of live CodePen examples demonstrate the CORS technique.
Minimize the number of API calls that your application makes. This is required by the Docusign API Usage Guidelines. For example, instead of one API call to create an envelope and another to add recipients, make one API call that completely defines the envelope.
Use the developer environment
Docusign provides a developer environment for testing your API calls. It's a free non-production environment that allows you to experiment and test your applications without affecting your live data. Always test your integration in the developer environment before deploying it to production.
Leverage available resources
Docusign provides extensive documentation, SDKs, and code examples for the APIs. Make use of these resources to understand how to use the APIs effectively. The Docusign Developer Center is an invaluable resource, offering comprehensive guides and community forums to aid your development process.
Structuring API requests
Organize request parameters
Properly structure your API requests by including necessary parameters, headers, and authentication details as required by the specific API endpoints. Refer to the Docusign API documentation for detailed information on each endpoint.
Leverage composite templates
Take advantage of Docusign template functionality to streamline the creation and sending of documents. Store frequently used document templates and use them to generate new envelopes efficiently.
Composite templates let you define multiple templates that will be combined to create the final envelope. They provide a way to mix server templates, inline templates, PDF forms, and document-specific elements within a single transaction.
Composite templates provide the most flexibility for using templates, as they enable you to:
Change a remote recipient to become an embedded recipient.
Change the template's document
Set template tab values
Combine two or more templates into a single envelope
Here's an example of using composite templates in a POST request to the Envelopes: create endpoint:
POST /restapi/v2.1/accounts/{accountId}/envelopes
{
"emailSubject": "Please sign this document",
"status": "sent",
"compositeTemplates": [
{
"serverTemplates": [
{
"sequence": "1",
"templateId": "serverTemplateId1"
}
],
"inlineTemplates": [
{
"sequence": "2",
"recipients": {
"signers": [
{
"email": "sample@example.com",
"name": "John Doe",
"recipientId": "1",
"roleName": "Signer1"
}
]
}
}
]
}
]
}
In the example above, a server template is used to create an envelope.
Error and exception handling
Handle API errors gracefully
While working with APIs, it's crucial to handle exceptions properly. Docusign provides detailed error responses when API calls fail. These errors are usually returned as a 400 Bad Request HTTP status with a message body containing detailed information about the error.
Here's an example of a Docusign error response:
{
"errorCode": "INVALID_REQUEST_PARAMETER",
"message": "The request contained at least one invalid parameter. No matching clause for..."
}
In your applications, you should build in error-handling logic that logs these errors and provides meaningful feedback. Here's an example in Python:
import requests
import json
headers = {
'Authorization': 'Bearer {accessToken}',
'Content-Type': 'application/json'
}
try:
response = requests.post('https://demo.docusign.net/restapi/v2.1/accounts/{accountId}/envelopes', headers=headers, data=json.dumps(payload))
response.raise_for_status()
except rest_framework.exceptions.APIException as err:
error_response = response.json()
print(f"Error occurred: {error_response['errorCode']}")
print(f"Error message: {error_response['message']}")
raise SystemExit(err)
In this Python code above, I’m using the requests library to send a POST request to the Docusign API. If the API call fails, an exception is raised, and the error details are extracted from the response.
Retry for transient errors
Transient errors are temporary errors that occur due to issues such as network glitches or timeouts, server overload, DNS issues, or other problems not intrinsic to your application. To identify transient errors, when encountering an API error, check the HTTP status code. If it's one of the temporary error codes (such as 429 Too Many Requests), it's good practice to implement a retry mechanism with exponential backoff (where appropriate) to ensure the request is eventually processed successfully.
Here's an illustrative Python snippet:
import time
import requests
import json
def send_request(data, max_retries=5):
headers = {
'Authorization': 'Bearer {accessToken}',
'Content-Type': 'application/json'
}
url = 'https://demo.docusign.net/restapi/v2.1/accounts/{accountId}/envelopes'
wait_time = 5 # wait time in seconds
for i in range(max_retries):
try:
response = requests.post(url, headers=headers, data=json.dumps(data))
response.raise_for_status()
return response.json()
except requests.exceptions.HTTPError as err:
if response.status_code in [429, 500, 503]:
print("Transient error occurred. Retrying...")
time.sleep(wait_time)
wait_time *= 5 # exponential backoff
else:
print(f"Error occurred: {response.json()['errorCode']}")
print(f"Error message: {response.json()['message']}")
raise SystemExit(err)
return None
send_request(payload)
In the above code snippet, the send_request function attempts to send a request to the Docusign API, retrying up to max_retries times if it encounters a transient error. The wait_time between retries doubles after each attempt, thus implementing an exponential backoff strategy.
Data security
Protect sensitive information: Safeguard sensitive data, such as API keys, access tokens, or user credentials. Use secure storage methods and avoid hardcoding these credentials in your application's source code. Consider using environment variables or secure credential management systems. Don’t store them in source control such as GitHub!
Employ encryption: Encrypt sensitive data at rest and in transit. Utilize industry-standard encryption algorithms and protocols to ensure the confidentiality and integrity of your data.
Implement proper access controls: Follow the principle of least privilege. Grant only the necessary permissions and access levels to API credentials and users. Regularly review and update access permissions to mitigate potential security risks.
API rate limits and throttling
Respect rate limits: Adhere to the rate limits imposed by the Docusign API to avoid excessive API calls. Consider implementing rate limiting mechanisms within your application to comply with the API's rate limits.
Handle rate limit errors: If you encounter rate limit errors, handle them gracefully by implementing appropriate retry and backoff strategies. Exponential backoff can be used to progressively increase the time between retries.
Use webhooks for event notifications (NO POLLING)
Instead of polling Docusign’s servers for status updates, use Docusign Connect (webhook notifications). Docusign Connect pushes status notifications to your chosen endpoints, reducing network traffic and ensuring timely updates.Note: webhooks at the envelope level are included with all eSignature plans.
If the application is not available on the internet to receive the incoming webhooks, PAAS systems can be used to receive the notifications. See https://www.docusign.com/blog/dsdev-webhook-listeners-part-4
Conclusion
By following these best practices, you can use Docusign APIs effectively in your applications. Structuring API requests, handling errors, and securing your data are crucial aspects of a successful integration. Remember to refer to the official Docusign API documentation for detailed specifications and guidelines. Happy integrating with Docusign!
Additional resources
Mohamed Shousha started his journey with Docusign in 2021. He is our leader in EMEA for the Developer Support and Advisory teams. With years of experience in implementing and supporting complex infrastructures and technical solutions for leaders in the industry, Mohamed is expert in various areas of knowledge such as Tech Support, Risk, Compliance, Audit, Advisory, Consultancy and Solution Design.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
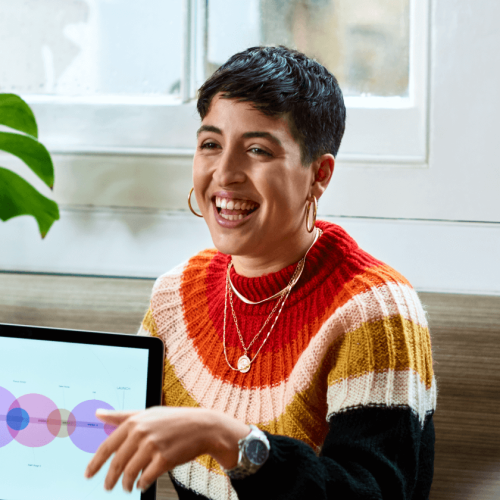