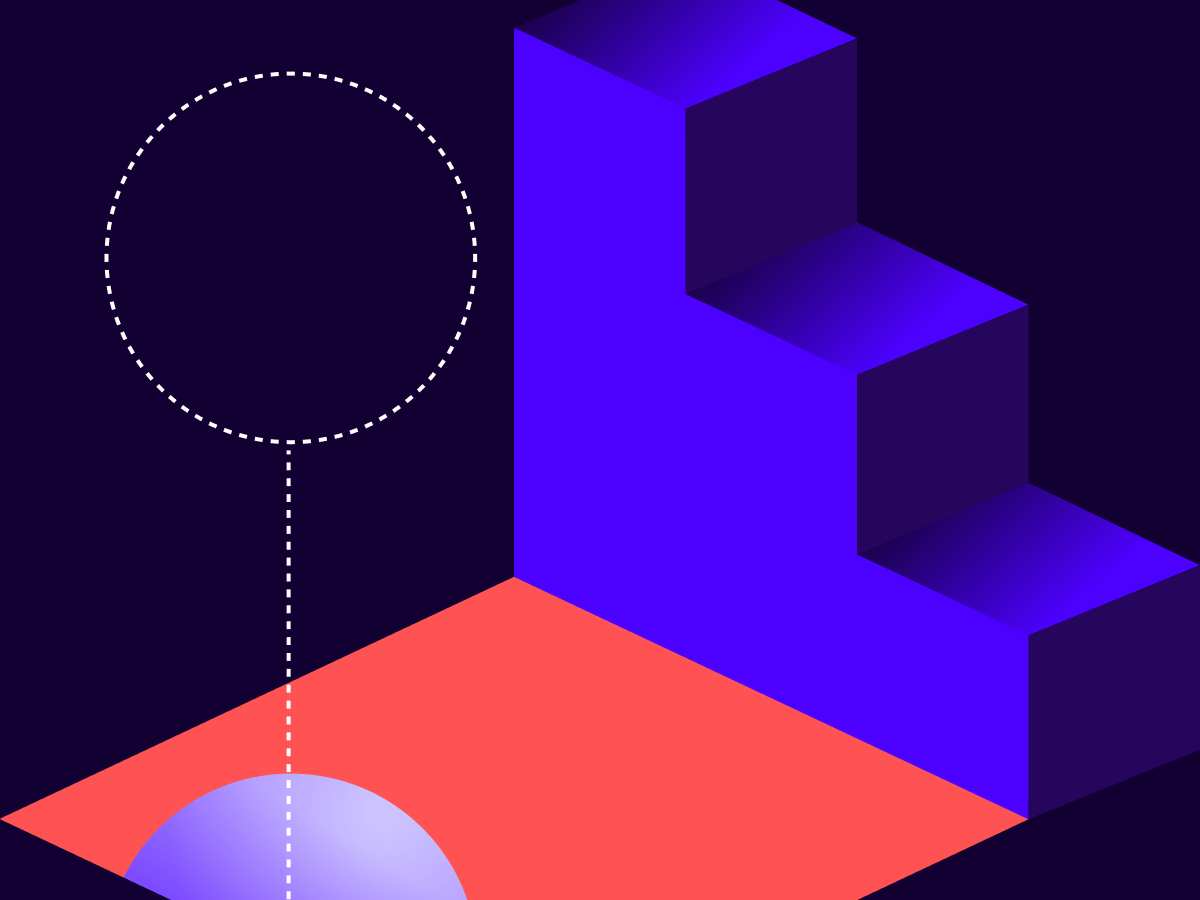
Demystifying JWT Token Debuggers
Everything you always wanted to know about JWT.IO
Table of contents
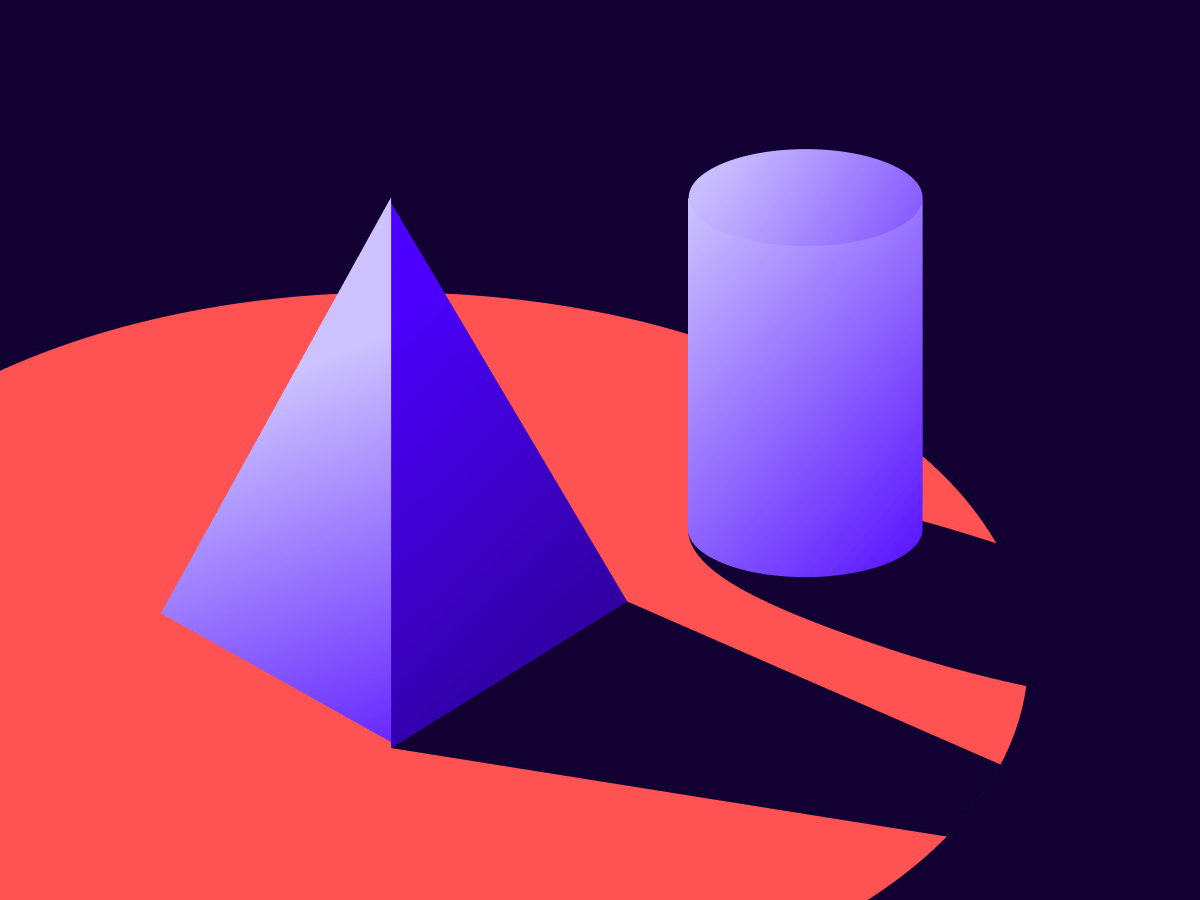
If you’ve ever followed the instructions on the Docusign JWT access token page, you have likely ended up on Jwt.io and used their online debugger (or any other JWT token debugger for that matter) to verify your token. If you’re like me, you have also probably wondered how the debugger is working underneath the hood. How does it sign the payload? And how does it verify the signature? Let’s take a moment and demystify the workings of how the debugger works.
TL;DR
JWT token debuggers encrypt your payload using your private key and use your public key to verify the encoding.
Long version
For the purpose of this example, assume you have a payload and you’re trying to sign it using the RS256 algorithm.
Here is your payload:
{
'iss':'xxxxx-xxxx-xxx-xxx',
'sub':'xxxxx-xxxx-xxx-xxx',
'iat':'1605233231',
'exp':'1607710548',
'aud':'some.cool.server.com',
'scope':'random scope'
}
Now, use the pyJwt library to create a digital signature of this payload with the RS256 algorithm.
First, import the library and read your private key as a string:
>>> import jwt
>>> private_key = open(‘private.pub’, ‘r’).read()
Then, define your payload:
>>> payload = {'iss':'xxxxx-xxxx-xxx-xxx','sub':'xxxxx-xxxx-xxx-
xxx','iat':'1605233231','exp':'1607710548','aud':'some.cool.server.com',
'scope':'random scope'}
Next, sign the payload:
>>> encoded = jwt.encode(payload, private_key, algorithm='RS256')
There are a couple things to note here. You do not need to specify the headers to the API, as it already knows what the header looks like for RS256. And the output will look something like:
>>> encoded b'eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiJ9.ey*****************Vg'
Now verify you would be getting the same signature from JWT.io’s token debugger:
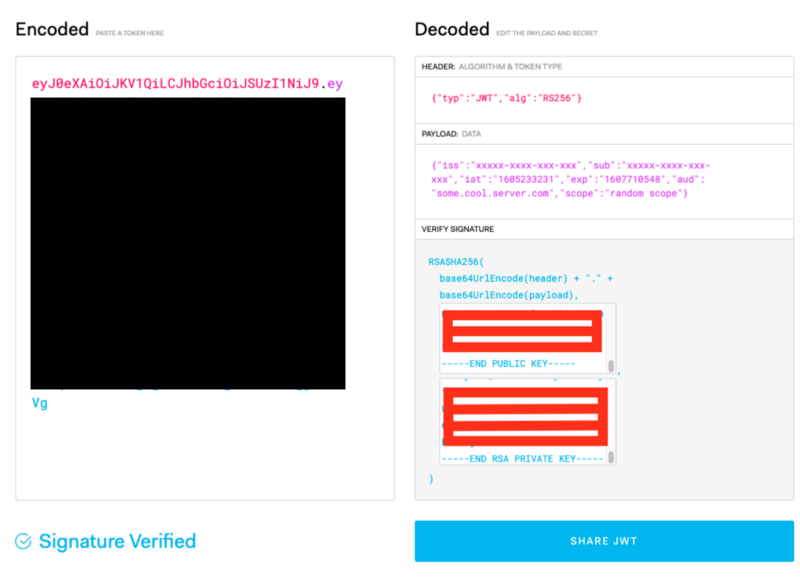
They both match. Now, that’s an encoded string that can be safely transferred, and only someone with a public key can decode that message.
Now you might ask: why does the JWT.io generator ask for both a private and a public key? As you might have guessed, the tool will decode the encoded payload and ensure the original payload is obtained. That’s equivalent to doing:
>>> public_key = open('public.pub', 'r').read()
>>> decoded = jwt.decode(encoded, public_key, algorithms='RS256')
That’s it. I hope this helps you understand how jwt.io token debugger works under the hood.
Additional resources
Nima Poulad is a Senior Developer Advocate at Docusign. He has years of experience in the industry as a software developer and as an advocate. He enjoys meeting the members of developer community and passionate about sharing what he knows with others.
Find him on Twitter: @NimaPoulad or LinkedIn.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
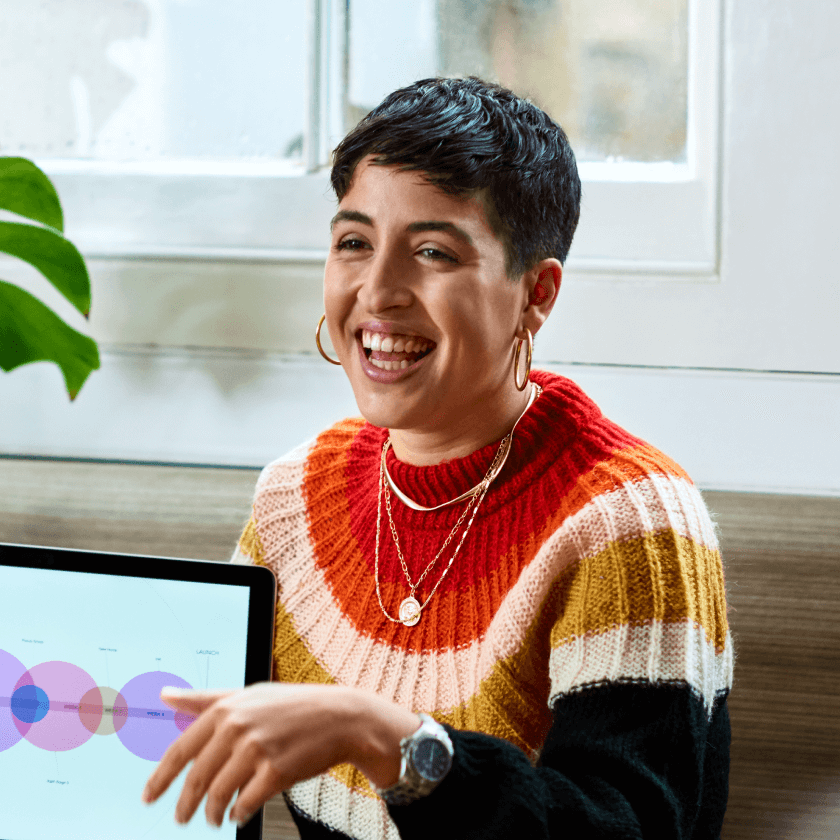