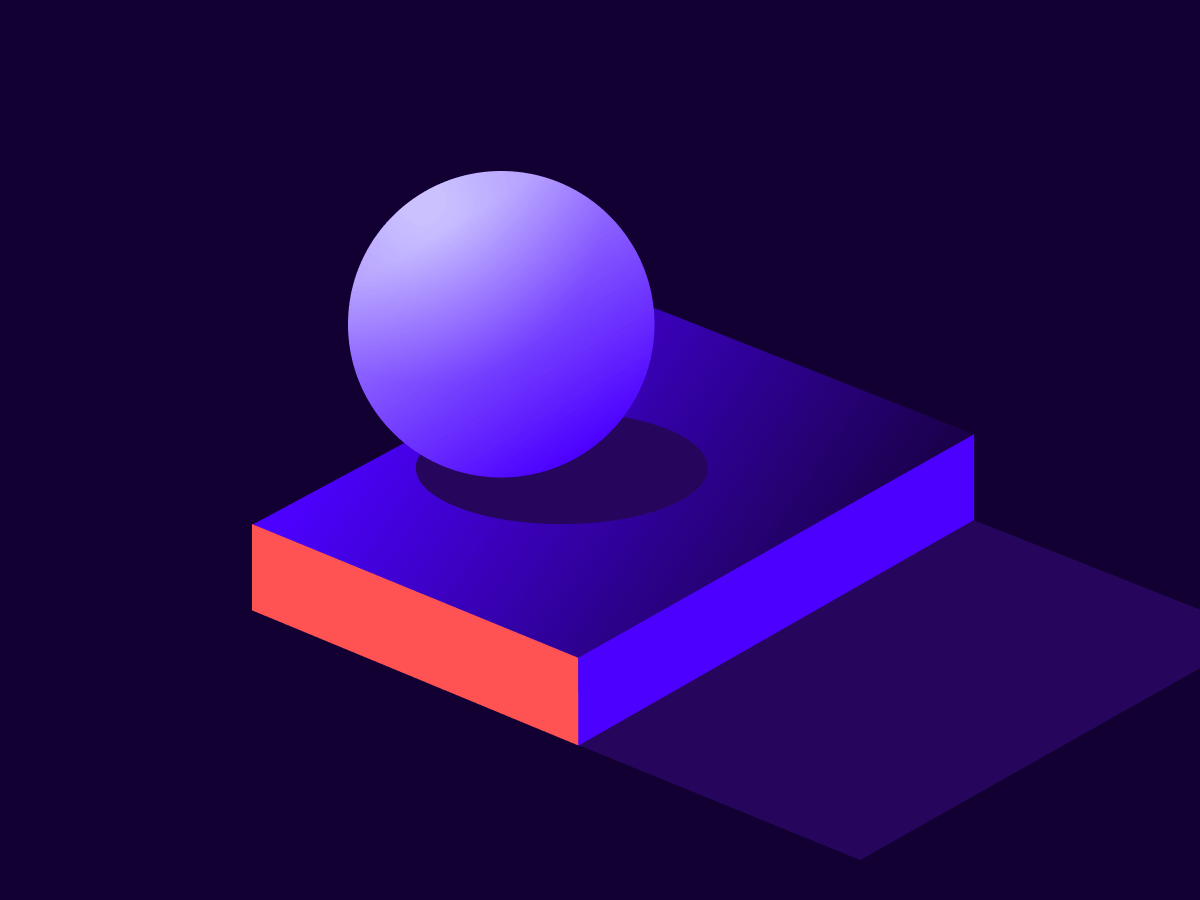
Common API Tasksđ: Inviting someone to a Docusign room
Programmatically invites others to your Docusign Room using the Docusign Rooms API and the Docusign Rooms SDKs
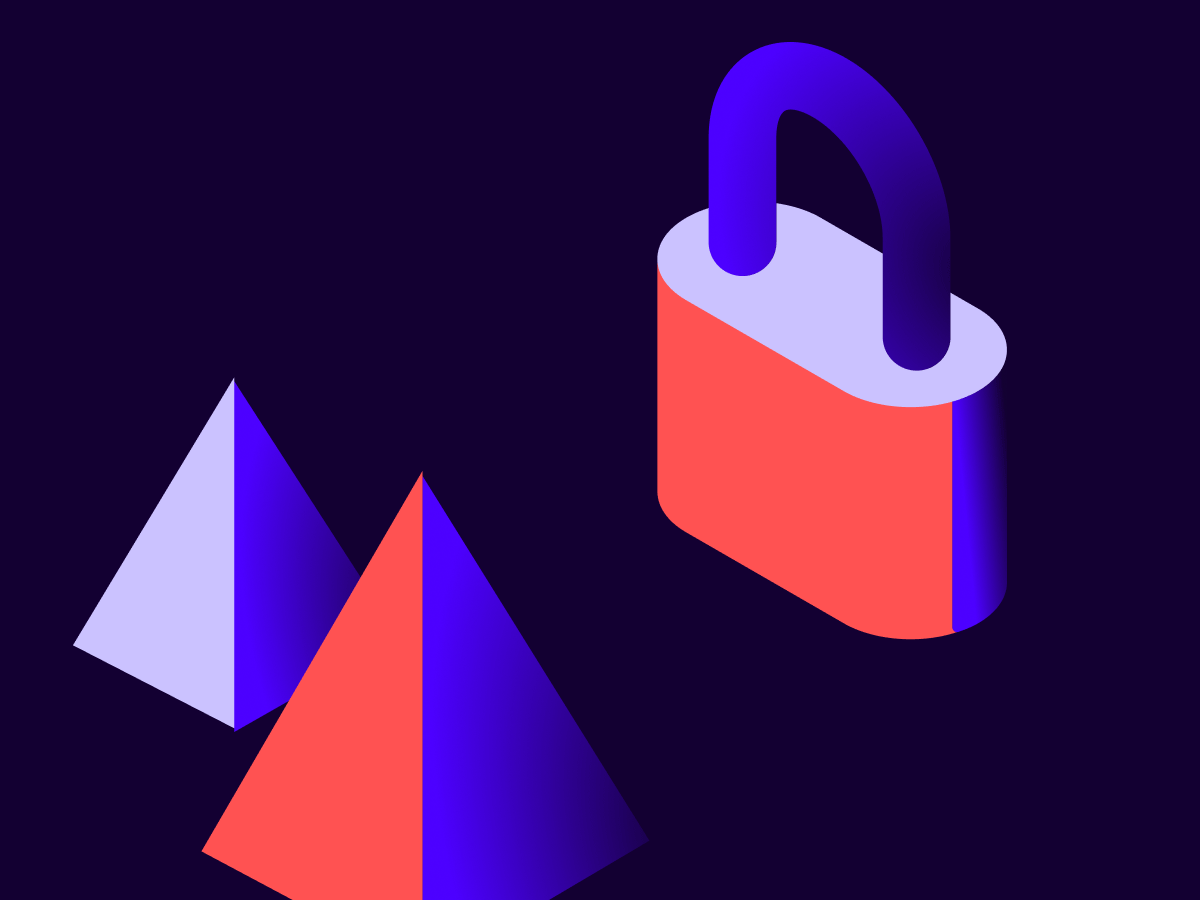
Greetings, developers and avid readers! Believe it or not, this is the 14th post in my CAT (Common API Tasks) blog series. These blog posts are meant to help you with simple, yet powerful Docusign APIârelated activities you plan to complete as part of building your app. The first 13 entries in this series were all related to our award-winning eSignature REST API that is probably what most of you know. However, in this post Iâm going to discuss a common API task related to the Docusign Rooms API.
Tell me more about Docusign Rooms
For those of you who are unfamiliar with Docusign Rooms, it enables companies to streamline complex agreements through a secure digital workspace that can be optimized to their specific workflow needs.
You can think of a Room as a container to manage all the tasks, documents, people, and approvals involved in a multi-step or multi-party transaction, such as buying a house or applying for a mortgage.
Inviting users to join a room
Docusign Rooms enables users to invite additional people to join a room. If we consider real estate agents and their workflow as an example, thatâs typically the buyer or seller that they would invite to join a room that is created to facilitate their real estate transaction. The buyers and the sellers do not have to be Docusign customers, and may not have a Docusign account. They just need access to the room that is used for their property, and their access level is limited. They cannot invite others, remove anything, or change other settings. They can only review documents, add additional documents, fill forms, and sign envelopes. Â
This type of role is called a client in the set of predefined roles that Docusign Rooms includes. (Docusign Rooms administrators can modify or add other roles that could be used for this purpose.)
When you make a request to the Rooms API to add someone to a room, you are inviting that client to join the room, so you need to provide their roleId, name and email address, and to designate whether theyâre the buyer or the seller. The client you added will then receive an email that looks like this:
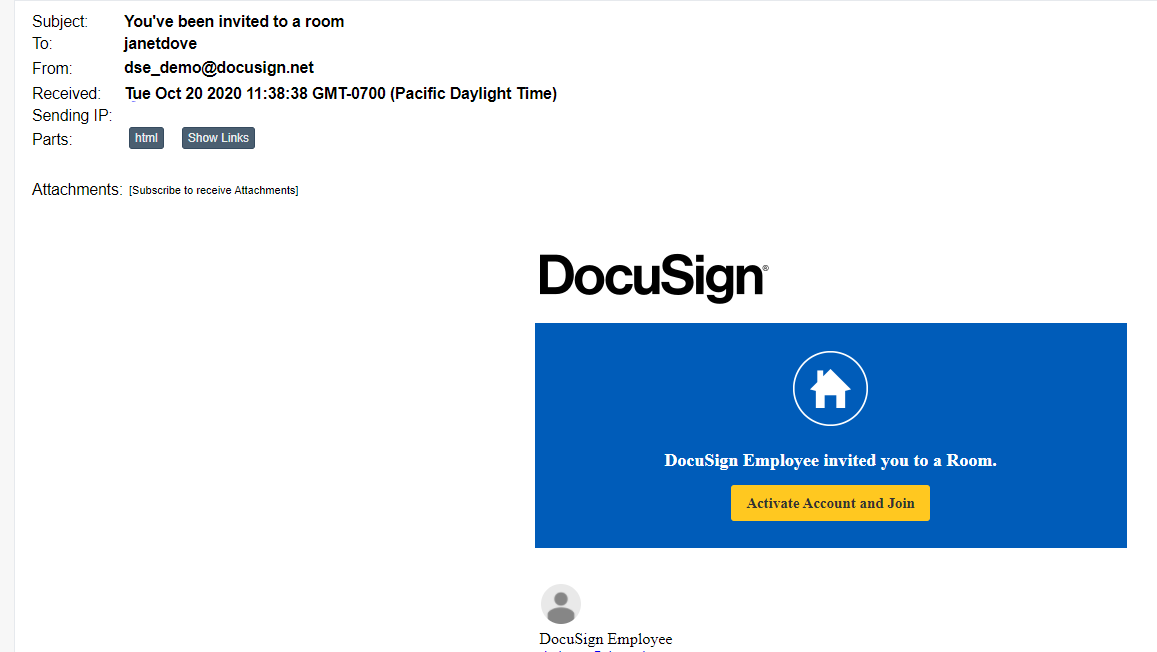
OK, and now to the part where I give you some code. Note that we recently released new SDKs that are specifically designed for the Rooms API. You need these packages to run the code snippets in this blog post. (The PHP SDK is coming soon, and Iâll update this blog post as soon as itâs available/)
Also note that you would have to obtain the roomId of the room youâre inviting a user to join, but Iâm not showing that here.
C#
var apiClient = new ApiClient(basePath); // For the Rooms API, the base path is https://demo.rooms.docusign.com for the developer account
// You will need to obtain an access token using your chosen auth flowÂ
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);Â
var roomsApi = new RoomsApi(apiClient);
var rolesApi = new RolesApi(apiClient);
var roomInvite = new RoomInvite();
// The only built-in role that can be used to invite external participants to join a room is "client"
RoleSummary clientRole = rolesApi.GetRoles(accountId, new RolesApi.GetRolesOptions { filter = "Client" }).Roles.First();
roomInvite.RoleId = clientRole.RoleId;
roomInvite.Email = "someone@domain.com";
roomInvite.FirstName = "Janet";
roomInvite.LastName = "Dove";
roomInvite.TransactionSideId = "buy"; // This value can also be "sell", "listbuy", or "refi"
roomsApi.InviteUser(accountId, roomId, roomInvite);
Java
ApiClient apiClient = new ApiClient(basePath); // For the Rooms API, the base path is https://demo.rooms.docusign.com for the developer account
// You will need to obtain an access token using your chosen auth flowÂ
apiClient.addDefaultHeader("Authorization", "Bearer " + accessToken);Â
RoomsApi roomsApi = new RoomsApi(apiClient);
RolesApi rolesApi = new RolesApi(apiClient);
RoomInvite roomInvite = new RoomInvite();
// The only built-in role that can be used to invite external participants to join a room is "client"
RoleSummary clientRole = rolesApi.getRoles(accountId).stream().filter(role-> role.getName() == âClientâ).findFirst().get();
roomInvite.setRoleId(clientRole.getRoleId());
roomInvite.setEmail("someone@domain.com");
roomInvite.setFirstName("Janet");
roomInvite.setLastName("Dove");
roomInvite.setTransactionSideId("buy"); // This value can also be "sell", "listbuy", or "refi"
roomsApi.inviteUser(accountId, roomId, roomInvite);
Node.js
let apiClient = new docusignRooms.ApiClient();
apiClient.setBasePath(basePath); // For the Rooms API, the base path is https://demo.rooms.docusign.com for the developer account
// You will need to obtain an access token using your chosen auth flow
apiClient.addDefaultHeader('Authorization', 'Bearer ' + args.accessToken);
let roomsApi = new docusignRooms.RoomsApi(apiClient);
let rolesApi = new docusignRooms.RolesApi(apiClient);
let roomInvite = new docusignRooms.RoomInvite();
let roles = await rolesApi.getRoles(accountId);
// The only built-in role that can be used to invite external participants to join a room is âclientâ
let clientRole = result.roles.filter(r => r.name == 'Client')[0];
roomInvite.roleId = clientRole.roleId;
roomInvite.email = 'someone@domain.com';
roomInvite.firstName = 'Janet';
roomInvite.lastName = 'Dove';
roomInvite.transactionSideId = 'buy'; // This value can also be âsellâ, âlistbuyâ, or ârefiâ
roomsApi.inviteUser(accountId, roomId, roomInvite)
Python
api_client = ApiClient(host = base_path) # For the Rooms API, the base path is https://demo.rooms.docusign.com for the developer account
# You will need to obtain an access token using your chosen auth flowÂ
api_client.set_default_header(
    header_name='Authorization',
    header_value=f'Bearer {access_token}')
rooms_api = RoomsApi(api_client)
roles_api = RolesApi(api_client)
roles = roles_api.get_roles(account_id)
# The only built-in role that can be used to invite external participants to join a room is âclientâ
role_id = [role.role_id for role in roles.roles if role.name == 'Client'][0]
room_invite = RoomInvite()
room_invite.roleId = role_id
room_invite.email = 'someone@domain.com'
room_invite.first_name = 'Janet'
room_invite.last_Nname = 'Dove'
room_invite.transaction_side_id = 'buy'; # This value can also be âsellâ, âlistbuyâ, or ârefiâ
rooms_api.invite_user(account_Iid, room_id, room_invite)
Ruby
configuration = DocuSign_Rooms::Configuration.new
configuration.host = base_path # For the Rooms API, the base path is https://demo.rooms.docusign.com for the developer account
api_client = DocuSign_Rooms::ApiClient.new(configuration)
# You will need to obtain an access token using your chosen auth flowÂ
api_client.set_default_header('Authorization', 'Bearer #{access_token}')
rooms_api = DocuSign_Rooms::RoomsApi.new(api_client)
roles_api = DocuSign_Rooms::RolesApi.new(api_client)
roles = roles_api.get_roles(account_id)
# The only built-in role that can be used to invite external participants to join a room is âclientâ
role_id = roles.select {|role| role.name == 'Client' }[0].role_id
room_invite = DocuSign_Rooms::RoomInvite.new()
room_invite.roleId = role_id
room_invite.email = 'someone@domain.com'
room_invite.first_name = 'Janet'
room_invite.last_name = 'Dove'
room_invite.transaction_side_id = 'buy'; # This value can also be âsellâ, âlistbuyâ, or ârefiâ
rooms_api.invite_user(account_Iid, room_id, room_invite)
Additional resources
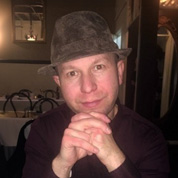
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
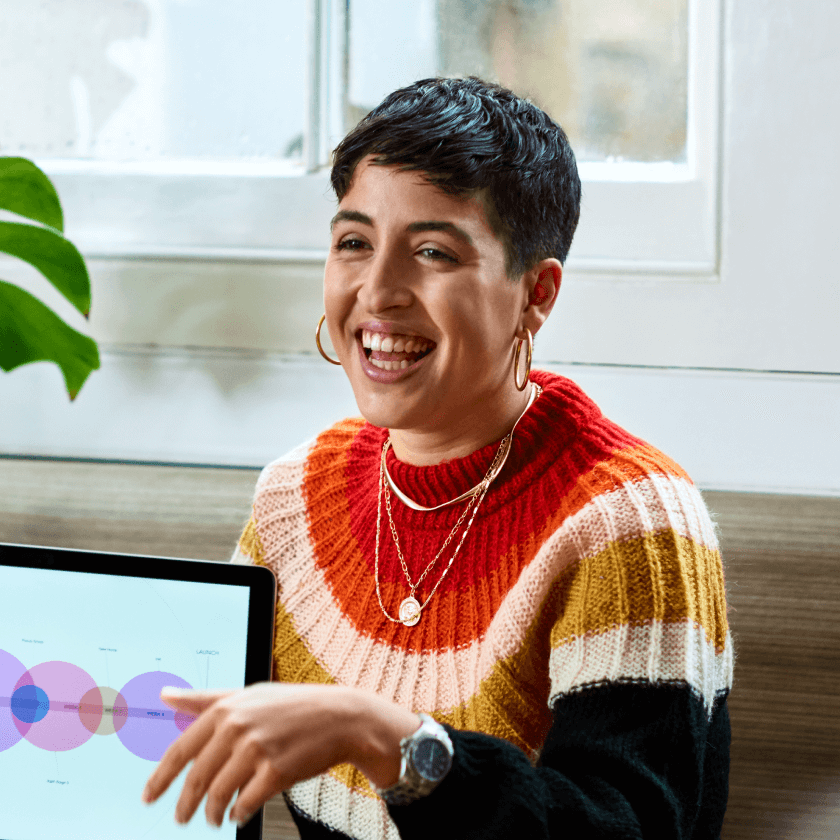