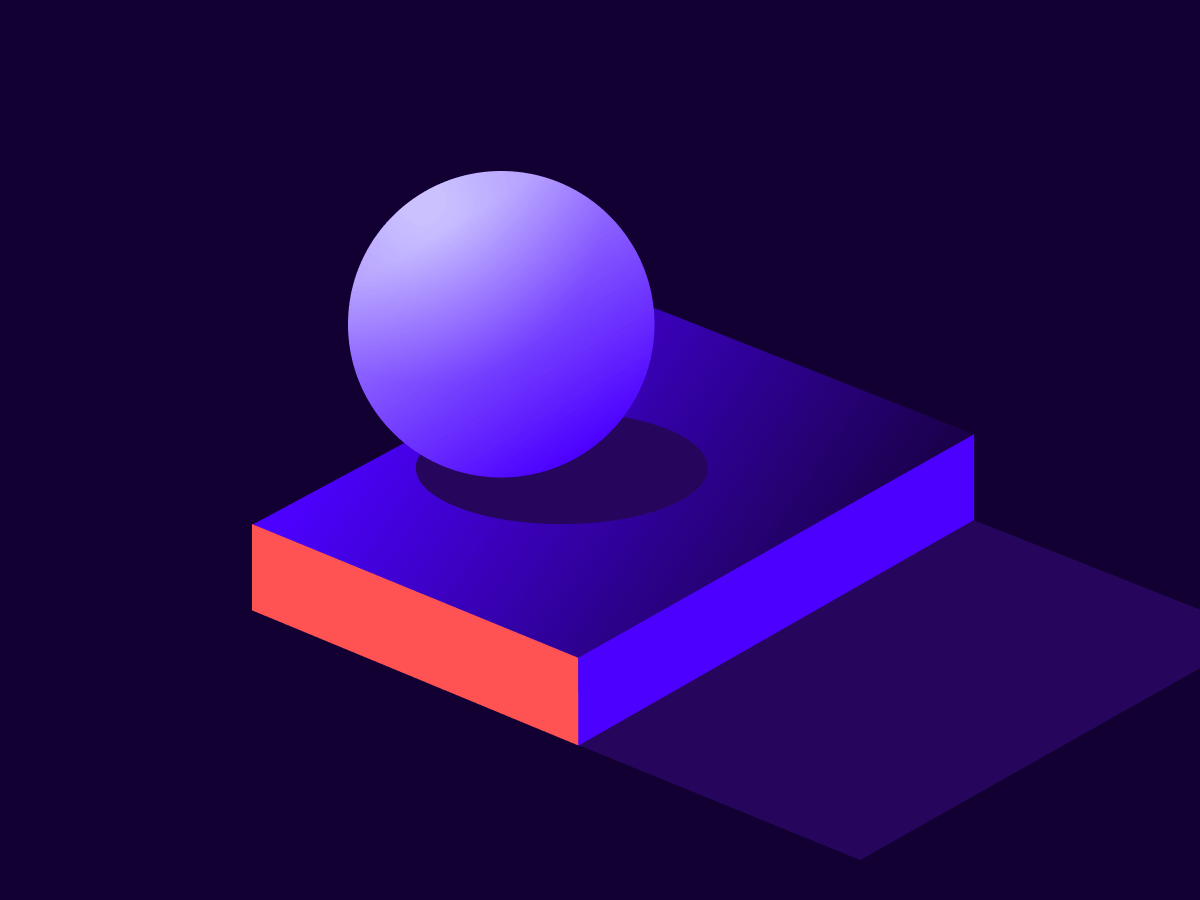
Common API Tasks🐈: Using conditional tabs
Conditional control of tab behavior through the eSignature API lets you support complex signature workflows
Table of contents
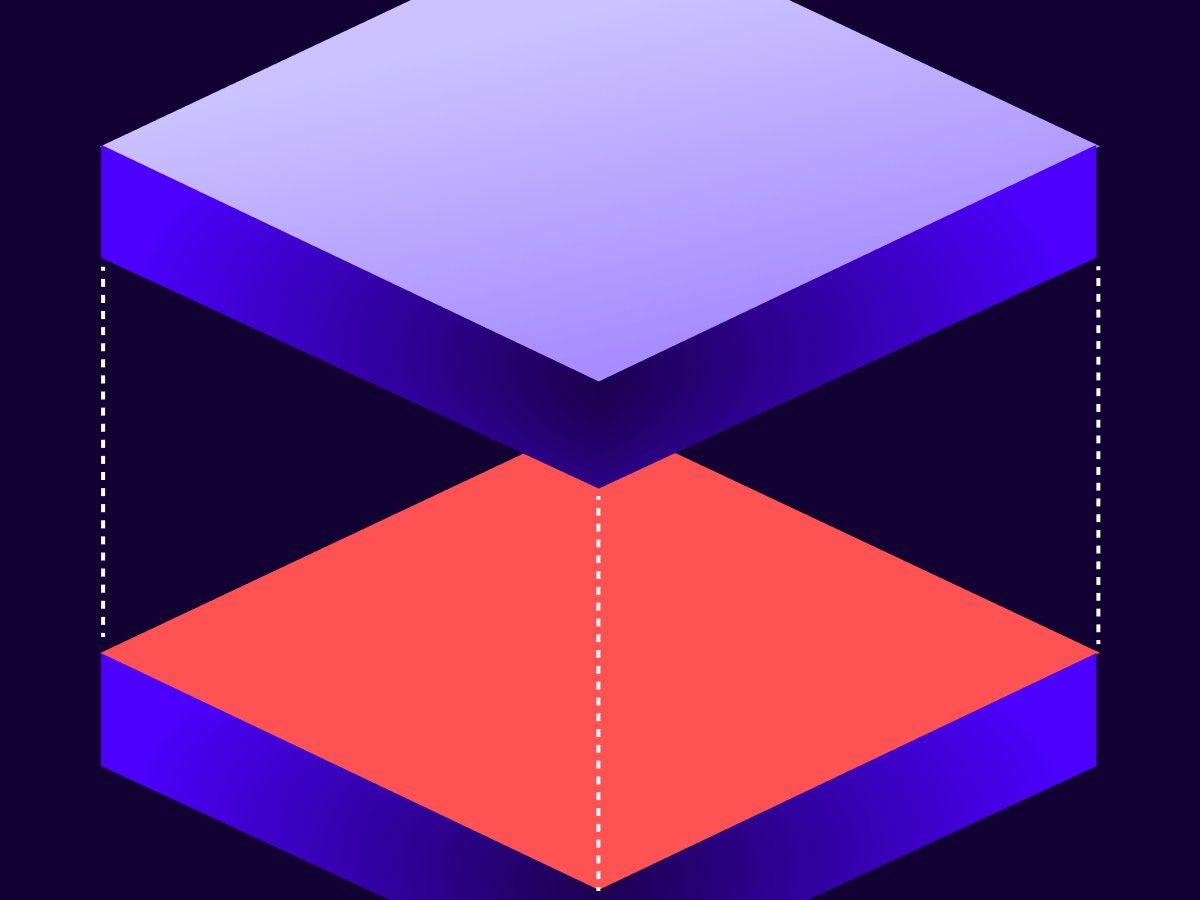
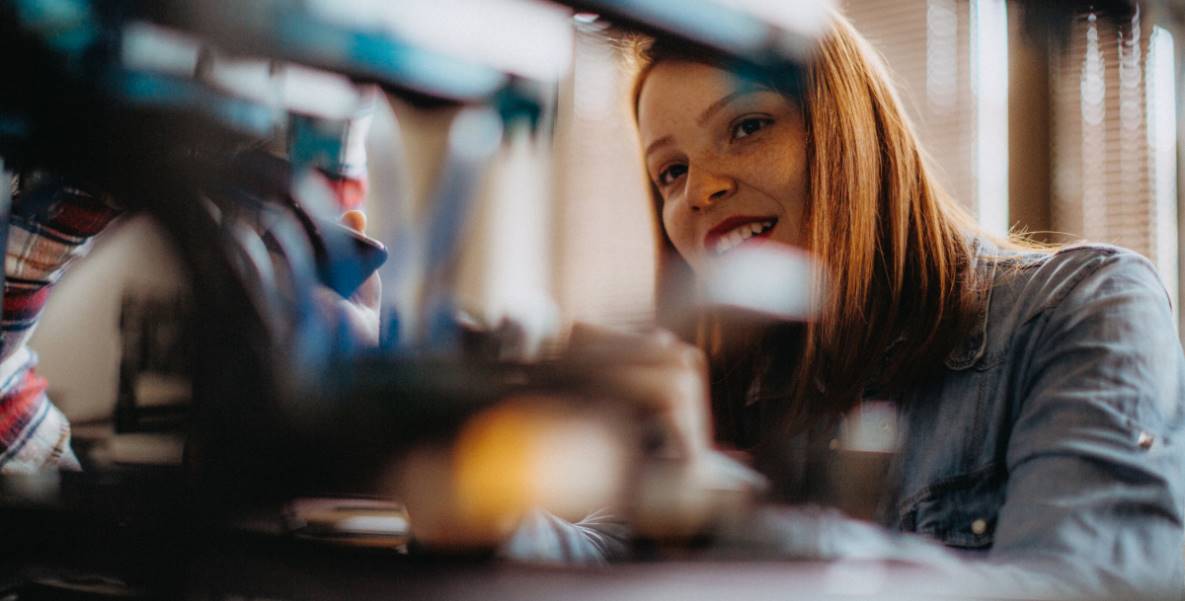
Welcome to another edition of the CAT🐈 (Common API Tasks) blog. This blog series is about giving you all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign developer blog.
In this article I’m going to dive into an advanced capability of the Docusign eSignature platform and show you how to use conditional fields (also known as tabs/tags).
Conditional tabs enable more sophisticated envelopes that take your end users (the signers) through a dynamic experience. That means some changes in the envelope can occur in response to decisions made by the signers in real time. Using conditional tabs lets your app support a wide variety of complex use cases. I will not cover all the different things you can do with conditional tabs, but will give you a taste of a simple scenario to keep the code snippets manageable. .
The scenario
In this example we have a single document in the envelope, consisting of a single page. At the top of the page I’ll position two radio buttons that are part of a radio button group. I’ll also add two signature tabs (SignHere elements) below, one on the left and one on the right. The signer first has to pick one of the radio buttons at the top (one is selected by default) and that determines which of the two SignHere tabs will be shown and available to be selected. The envelope does include both SignHere tabs, but only one must be selected to complete the signing by the signer, as they are both set to be conditional based on the radio button selection.
And now for some code. Please note that the code blocks below are incomplete and only show how to create the tabs objects and add them to the envelope definition object. You would still need to create the document(s) and make the API call to create the envelope to get them working.
C#
// Create a signer object; in this case, for embedded signing
Signer signer1 = new Signer
{
Email = "signer@domain.com",
Name = "Signer One",
ClientUserId = "1001",
RecipientId = "1"
};
// Create a radio group for the radio buttons
RadioGroup radioGroup = new RadioGroup
{
GroupName = "RadioGroup1",
DocumentId = "1",
Radios = new List<radio>()
};
// Add the two radio buttons to the radio button group. The first one is defaulted by setting Selected to be "true"
radioGroup.Radios.Add(new Radio { PageNumber = "1", Value = "radio 1", XPosition = "200", YPosition = "100", Selected = "true" });
radioGroup.Radios.Add(new Radio { PageNumber = "1", Value = "radio 2", XPosition = "200", YPosition = "120", Selected = "false" });
// Create two SignHere tabs; each is conditional, based on the value of a radio button
SignHere signHere1 = new SignHere
{
DocumentId = "1",
PageNumber = "1",
XPosition = "100",
YPosition = "400",
ConditionalParentLabel = "RadioGroup1", // Must match the radio group!
ConditionalParentValue = "radio 1" // Must match the specific radio button!
};
SignHere signHere2 = new SignHere
{
DocumentId = "1",
PageNumber = "1",
XPosition = "400",
YPosition = "400",
ConditionalParentLabel = "RadioGroup1", // Must match the radio group!
ConditionalParentValue = "radio 2" // Must match the specific radio button!
};
// Add all the tabs to the Singer object
Tabs signer1Tabs = new Tabs
{
RadioGroupTabs = new List<radiogroup> { radioGroup } ,
SignHereTabs = new List<signhere> { signHere1, signHere2 }
};
signer1.Tabs = signer1Tabs;
// Add the recipient to the envelope object
Recipients recipients = new Recipients
{
Signers = new List<signer> { signer1 }
};
// Add recipients to the EnvelopeDefinition object; need to also add documents
envelopeDefinition.Recipients = recipients;
</signer></signhere></radiogroup></radio>
Java
// Create a signer object; in this case, for embedded signing
Signer signer1 = new Signer();
signer1.setEmail("signer@domain.com");
signer1.setName("Signer One");
signer1.setClientUserId("1001");
signer1.setRecipientId("1");
// Create a radio group for the radio buttons
RadioGroup radioGroup = new RadioGroup();
radioGroup.setGroupName("RadioGroup1");
radioGroup.setDocumentId("1");
radioGroup.setRadios(new java.util.List<radio>());
// Add the two radio buttons to the radio button group. The first one is defaulted by setting Selected to be "true"
Radio radio1 = new Radio();
radio1.setPageNumber("1");
radio1.setValue("radio 1");
radio1.setXPosition("200");
radio1.setYPosition("100");
radio1.setSelected("true");
Radio radio2 = new Radio();
radio2.setPageNumber("1");
radio2.setValue("radio 2");
radio2.setXPosition("200");
radio2.setYPosition("120");
radio2.setSelected("false");
radioGroup.getRadios().Add(radio1);
radioGroup.getRadios().Add(radio2);
// Create two SignHere tabs, each is conditional based on the value of a radio button
SignHere signHere1 = new SignHere();
signHere1.setDocumentId("1");
signHere1.setPageNumber("1");
signHere1.setXPosition("100");
signHere1.setYPosition("400");
signHere1.setConditionalParentLabel("RadioGroup1"); // Must match the radio group!
signHere1.setConditionalParentValue("radio 1"); // Must match the specific radio button!
SignHere signHere2 = new SignHere();
signHere2.setDocumentId("1");
signHere2.setPageNumber("1");
signHere2.setXPosition("400");
signHere2.setYPosition("400");
signHere2.setConditionalParentLabel("RadioGroup1"); // Must match the radio group!
signHere2.setConditionalParentValue("radio 2"); // Must match the specific radio button!
// Add all the tabs to the Singer object
Tabs signer1Tabs = new Tabs();
signer1Tabs.setRadioGroupTabs(new java.util.List<radiogroup>());
signer1Tabs.getRadioGroupTabs().Add(radioGroup);
signer1Tabs.setSignHereTabs(new java.util.List<signhere>());
signer1Tabs.getSignHereTabs().Add(signHere1);
signer1Tabs.getSignHereTabs().Add(signHere2);
signer1.setTabs(signer1Tabs);
// Add the recipient to the envelope object
Recipients recipients = new Recipients();
recipients.setSigners(new java.util.List<signer>());
recipients.getSigners().Add(signer1);
// Add recipients to the envelopeDefinition object; need to also add documents
envelopeDefinition.setRecipients(recipients);
</signer></signhere></radiogroup></radio>
Node.js
// Create a signer object; in this case, for embedded signing
let signer1 = new docusign.Signer();
Signer1.email = 'signer@domain.com';
Signer1.name = 'Signer One';
signer1.clientUserId = '1001';
signer1.recipientId = '1';
// Create a radio group for the radio buttons
let radioGroup = new docusign.RadioGroup();
radioGroup.groupName = 'RadioGroup1';
radioGroup.documentId ='1';
radioGroup.radios = [];
// Add the two radio buttons to the radio button group. The first one is defaulted by setting Selected to be 'true'
let radio1 = new docusign.Radio();
radio1.pageNumber = '1';
radio1.value= 'radio 1';
radio1.xPosition = '200';
radio1.yPosition = '100';
radio1.selected = 'true';
let radio2 = new docusign.Radio();
radio2.pageNumber = '1';
radio2.value= 'radio 2';
radio2.xPosition = '200';
radio2.yPosition = '120';
radio2.selected = 'false';
radioGroup.radios.push(radio1);
radioGroup.radios.push(radio2);
// Create two SignHere tabs; each is conditional based on the value of a radio button
let signHere1 = new docusign.SignHere();
signHere1.documentId= '1';
signHere1.pageNumber= '1';
signHere1.xPosition = '100';
signHere1.yPosition = '400';
signHere1.conditionalParentLabel = 'RadioGroup1'; // Must match the radio group!
signHere1.conditionalParentValue = 'radio 1'; // Must match the specific radio button!
let signHere2 = new docusign.SignHere();
signHere2.documentId= '1';
signHere2.pageNumber= '1';
signHere2.xPosition = '400';
signHere2.yPosition = '400';
signHere2.conditionalParentLabel = 'RadioGroup1'; // Must match the radio group!
signHere2.conditionalParentValue = 'radio 2'; // Must match the specific radio button!
// Add all the tabs to the Singer object
let signer1Tabs = new docusign.Tabs();
signer1Tabs.radioGroupTabs= [];
signer1Tabs.radioGroupTabs.push(radioGroup);
signer1Tabs.signHereTabs = [];
signer1Tabs.signHereTabs.push(signHere1);
signer1Tabs.signHereTabs.push(signHere2);
signer1.tabs = signer1Tabs;
// Add the recipient to the envelope object
let recipients = new docusign.Recipients();
recipients.signers = [];
recipients.signers.push(signer1);
// Add recipients to the envelopeDefinition object; need to also add documents
envelopeDefinition.recipients = recipients;
PHP
# Create a signer object; in this case, for embedded signing
$signer1 = new \Docusign\eSign\Model\Signer();
$signer1->setEmail('signer@domain.com');
$signer1->setName('Signer One');
$signer1->setClientUserId('1001');
$signer1->setRecipientId('1');
# Create a radio group for the radio buttons
$radio_group = new \Docusign\eSign\Model\RadioGroup();
$radio_group->setGroupName('RadioGroup1');
$radio_group->setDocumentId('1');
$radio_group->setRadios([]);
# Add the two radio buttons to the radio button group. The first one is defaulted by setting Selected to be 'true'
$radio1 = new \Docusign\eSign\Model\Radio();
$radio1->setPageNumber('1');
$radio1->setValue('radio 1');
$radio1->setXPosition('200');
$radio1->setYPosition('100');
$radio1->setSelected('true');
$radio2 = new new \Docusign\eSign\Model\Radio();
$radio2->setPageNumber('1');
$radio2->setValue('radio 2');
$radio2->setXPosition('200');
$radio2->setYPosition('120');
$radio2->setSelected('false');
array_push($radio_group->getRadios(), $radio1);
array_push($radio_group->getRadios(), $radio2);
# Create two SignHere tabs; each is conditional based on the value of a radio button
$sign_here1 = new \Docusign\eSign\Model\SignHere();
$sign_here1->setDocumentId('1');
$sign_here1->setPageNumber('1');
$sign_here1->setXPosition('100');
$sign_here1->setYPosition('400');
$sign_here1->setConditionalParentLabel('RadioGroup1'); # Must match the radio group!
$sign_here1->setConditionalParentValue('radio 1'); # Must match the specific radio button!
$sign_here2 = new \Docusign\eSign\Model\SignHere();
$sign_here2->setDocumentId('1');
$sign_here2->setPageNumber('1');
$sign_here2->setXPosition('400');
$sign_here2->setYPosition('400');
$sign_here2->setConditionalParentLabel('RadioGroup1'); # Must match the radio group!
$sign_here2->setConditionalParentValue('radio 1'); # Must match the specific radio button!
# Add all the tabs to the Singer object
$signer1_tabs = new \Docusign\eSign\Model\Tabs();
$signer1_tabs->setRadioGroupTabs([]);
array_push($signer1_tabs->getRadioGroupTabs(), $radio_group);
$signer1_tabs->setSignHereTabs([]);
array_push($signer1_tabs->getSignHereTabs(), $sign_here1);
array_push($signer1_tabs->getSignHereTabs(), $sign_here2);
$signer1->setTabs($signer1_tabs);
# Add the recipient to the envelope object
$recipients = new \Docusign\eSign\Model\Recipients();
recipients->setSigners([]);
array_push($recipients->getSigners(), $signer1);
# Add $recipients to the $envelope_definition object; need to also add documents
$envelope_definition->setRecipients($recipients);
Python
# Create a signer object; in this case, for embedded signing
signer1 = Signer()
signer1.email = 'signer@domain.com'
signer1.name = 'Signer One'
signer1.client_user_id = '1001'
signer1.recipient_id = '1'
# Create a radio group for the radio buttons
radio_group = RadioGroup()
radio_group.group_name = 'RadioGroup1'
radio_group.document_id = '1'
radio_group.radios = []
# Add the two radio buttons to the radio button group. The first one is defaulted by setting Selected to be 'true'
radio1 = Radio()
radio1.page_Number = '1'
radio1.value = 'radio 1'
radio1.x_position = '200'
radio1.y_position = '100'
radio1.selected = 'true'
radio2 = Radio()
radio2.page_Number = '1'
radio2.value = 'radio 2'
radio2.x_position = '200'
radio2.y_position = '120'
radio2.selected = 'false'
radio_group.radios.insert(radio1)
radio_group.radios.insert(radio2)
# Create two SignHere tabs; each is conditional based on the value of a radio button
sign_here1 = SignHere()
sign_here1.document_id = '1'
sign_here1.page_number = '1'
sign_here1.x_position = '100'
sign_here1.y_position = '400'
sign_here1.conditional_parent_label = 'RadioGroup1' # Must match the radio group!
sign_here1.conditional_parent_value = 'radio 1' # Must match the specific radio button!
sign_here2 = SignHere()
sign_here2.document_id = '1'
sign_here2.page_number = '1'
sign_here2.x_position = '400'
sign_here2.y_position = '400'
sign_here2.conditional_parent_label = 'RadioGroup1' # Must match the radio group!
sign_here2.conditional_parent_value = 'radio 2' # Must match the specific radio button!
# Add all the tabs to the Singer object
signer1_tabs = Tabs()
signer1_tabs.radio_group_tabs = []
signer1_tabs.radio_group_tabs.insert(radio_group)
signer1_tabs.sign_here_tabs = []
signer1_tabs.sign_here_tabs.insert(sign_here1)
signer1_tabs.sign_here_tabs.insert(sign_here2)
signer1.tabs = signer1_tabs
# Add the recipient to the envelope object
recipients = Recipients()
recipients.signers = []
recipients.signers.insert(signer1)
# Add recipients to the envelope_definition object; need to also add documents
envelope_definition.recipients = recipients
Ruby
# Create a signer object; in this case, for embedded signing
signer1 = DocuSign_eSign::Signer.new
signer1.email = 'signer@domain.com'
signer1.name = 'Signer One'
signer1.client_user_id = '1001'
signer1.recipient_id = '1'
# Create a radio group for the radio buttons
radio_group = DocuSign_eSign::RadioGroup.new
radio_group.group_name = 'RadioGroup1'
radio_group.document_id = '1'
radio_group.radios = []
# Add the two radio buttons to the radio button group. The first one is defaulted by setting Selected to be 'true'
radio1 = DocuSign_eSign::Radio.new
radio1.page_Number = '1'
radio1.value = 'radio 1'
radio1.x_position = '200'
radio1.y_position = '100'
radio1.selected = 'true'
radio2 = DocuSign_eSign::Radio.new
radio2.page_Number = '1'
radio2.value = 'radio 2'
radio2.x_position = '200'
radio2.y_position = '120'
radio2.selected = 'false'
radio_group.radios.append(radio1)
radio_group.radios.append(radio2)
# Create two SignHere tabs; each is conditional based on the value of a radio button
sign_here1 = DocuSign_eSign::SignHere.new
sign_here1.document_id = '1'
sign_here1.page_number = '1'
sign_here1.x_position = '100'
sign_here1.y_position = '400'
sign_here1.conditional_parent_label = 'RadioGroup1' # Must match the radio group!
sign_here1.conditional_parent_value = 'radio 1' # Must match the specific radio button!
sign_here2 = DocuSign_eSign::SignHere.new
sign_here2.document_id = '1'
sign_here2.page_number = '1'
sign_here2.x_position = '400'
sign_here2.y_position = '400'
sign_here2.conditional_parent_label = 'RadioGroup1' # Must match the radio group!
sign_here2.conditional_parent_value = 'radio 2' # Must match the specific radio button!
# Add all the tabs to the Singer object
signer1_tabs = DocuSign_eSign::Tabs.new
signer1_tabs.radio_group_tabs = []
signer1_tabs.radio_group_tabs.append(radio_group)
signer1_tabs.sign_here_tabs = []
signer1_tabs.sign_here_tabs.append(sign_here1)
signer1_tabs.sign_here_tabs.append(sign_here2)
signer1.tabs = signer1_tabs
# Add the recipient to the envelope object
recipients = DocuSign_eSign::Recipients.new
recipients.signers = []
recipients.signers.append(signer1)
# Add recipients to the envelope_definition object; need to also add documents
envelope_definition.recipients = recipients
And there you have it! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
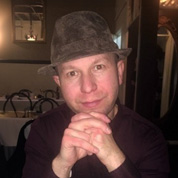
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
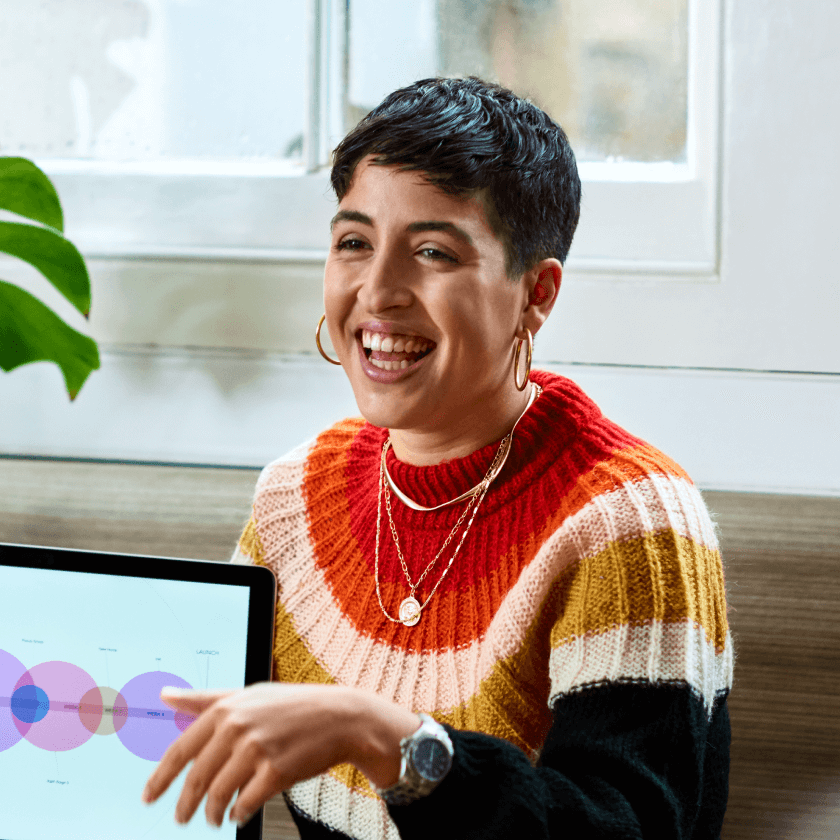