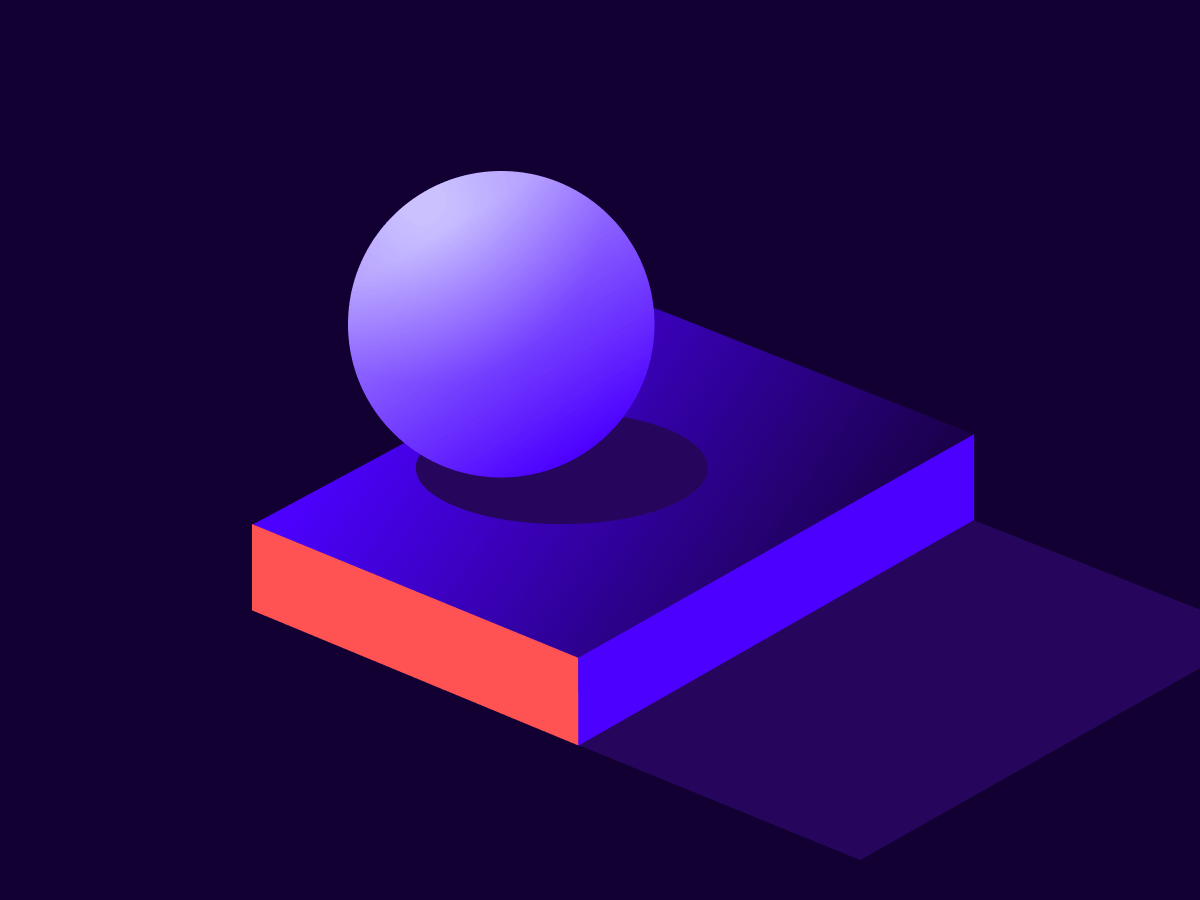
Common API Tasks🐈: Search for envelopes that need to be signed
Learn how to query using the eSignature REST API for envelopes that need to be signed.
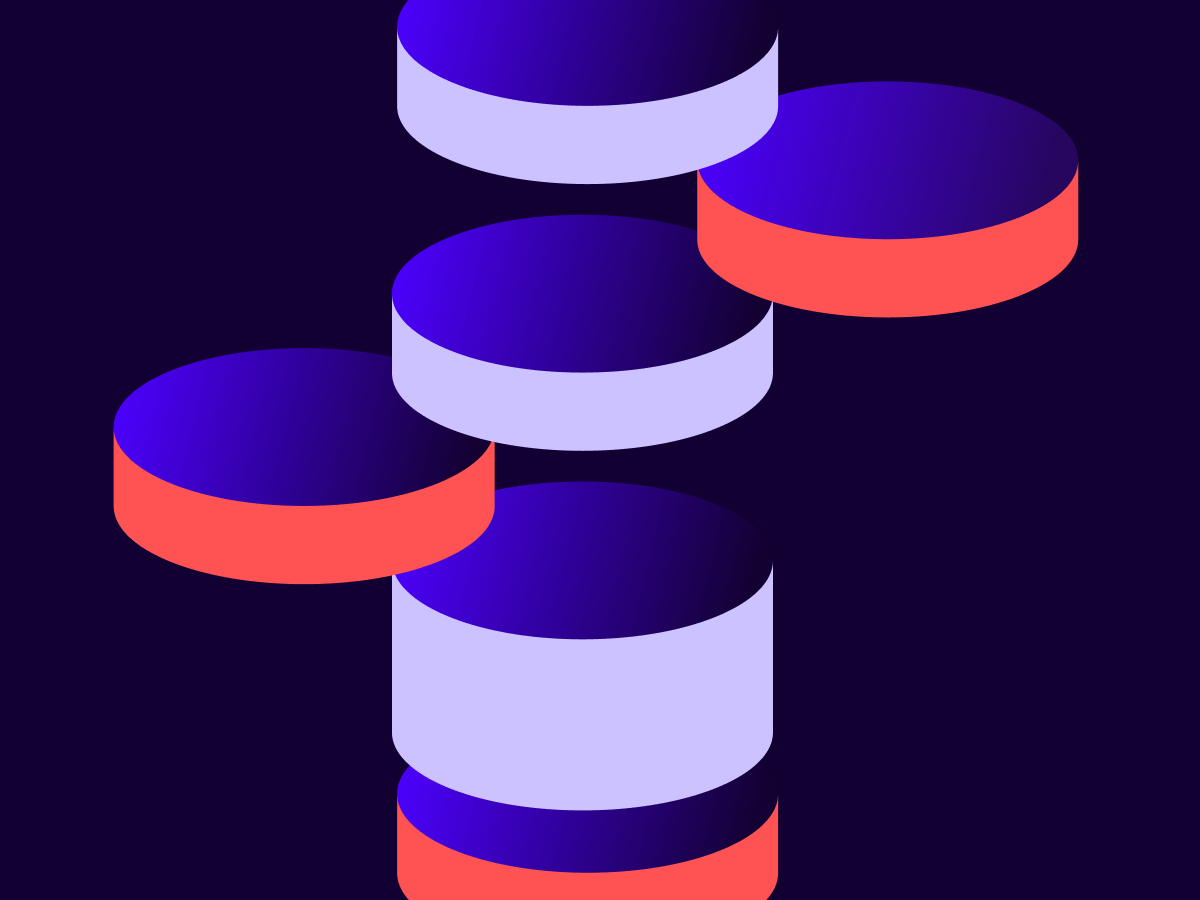
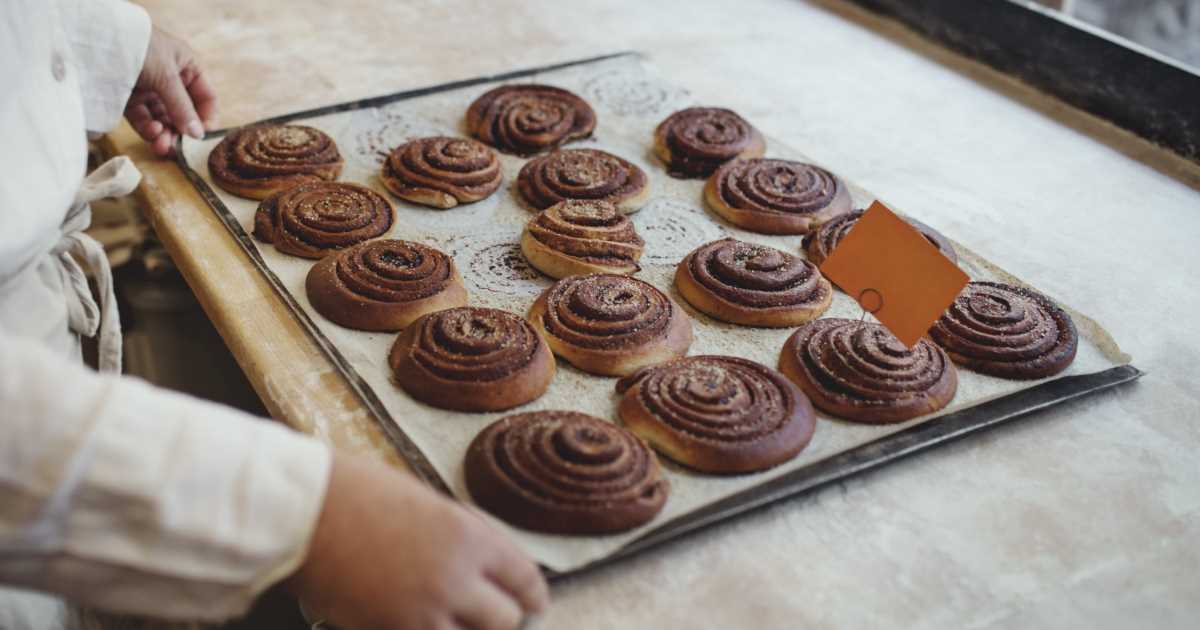
Welcome to a phenomenal new episode of the CAT🐈 (Common API Tasks) blog. In this blog series I’ll be providing all you need to know in order to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign developer blog.
Today’s episode was inspired by a question from a fellow developer on StackOverflow. This developer was building an integration that provides the end-users a web application. One of the features in their app was the ability for the user to see all the envelopes currently awaiting their signature similar to the capability you see in the Docusign web app (by selecting Manage at the top and then Action Required on the left navigation bar).
It turns out that the Docusign eSignature REST API has a robust mechanism for developers to search and filter the list of envelopes in the account. The Envelopes:listStatusChanges endpoint enables developers to provide various parameters such as the status of the envelopes, when they were sent, by whom, and more. Developers can also specify which pieces of information they’re interested in (documents, recipients, tabs) and, once they make the API call, they get back a list of envelopes corresponding to the information they provided. Powerful stuff.
Of course, you can also call this endpoint from our eSignature SDKs. The following code snippets show you a simple example. I am looking for all the envelopes sent from this account during the month of December 2021 which are awaiting my signature (note that the user who was authenticated for the purpose of obtaining an access token for API calls is the one used to filter for signers need to sign). I ask to return information about recipients; then, I just loop through the resulting envelopes. I don’t do much more. I’ll leave that to you all.
C#
var apiClient = new ApiClient(basePath);
// You will need to obtain an access token using your chosen authentication flow
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
var options = new EnvelopesApi.ListStatusChangesOptions();
options.include = "recipients";
options.fromDate = "11/1/2021";
options.toDate = "12/1/2021";
options.folderIds = "awaiting_my_signature";
var searchResults = envelopesApi.ListStatusChanges(accountId, options);
foreach (var envelope in searchResults.Envelopes)
{
// do something useful
}
Java
// You will need to obtain an access token using your chosen authentication flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(config);
EnvelopesApi.ListStatusChangesOptions options = envelopesApi.new ListStatusChangesOptions();
options.setInclude("recipients");
options.setFromDate( "11/1/2021");
options.setToDate("12/1/2021");
options.setFolderIds("awaiting_my_signature");
EnvelopesInformation searchResults = envelopesApi.listStatusChanges(accountId, options);
for (Envelope envelope : searchResults.getEnvelopes())
{
// do something useful
}
Node.js
// You will need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
let options = {'include' : 'recipients', 'fromDate' : '11/1/2021', 'toDate' : '12/1/2021', "folderIds" : 'awaiting_my_signature'};
let searchResults = envelopesApi.listStatusChanges(accountId, options);
searchResults.envelopes.forEach(envelope => {
// do something useful
});
PHP
# You will need to obtain an access token using your chosen authentication flow
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \Docusign\eSign\\Api\EnvelopesApi($config);
$options = envelopesApi.new ListStatusChangesOptions();
$options->setInclude('recipients');
$options->setFromDate('11/1/2021');
$options->setToDate('12/1/2021');
$options->setFolderIds('awaiting_my_signature');
$search_results = $envelopes_api->listStatusChanges($account_id, $options);
$envelopes = $search_results->getEnvelopes();
foreach ($envelopes as $envelope)
{
# do something useful
}
Python
# You will need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
options = ListStatusChangesOptions()
options.include= 'recipients'
options.from_date ='11/1/2021'
options.to_date = '12/1/2021'
options.folder_ids = 'awaiting_my_signature'
search_results = envelopes_api.list_status_changes(account_id, options)
for envelope in search_results.envelopes:
# do something useful
Ruby
# You will need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
options = DocuSign_eSign::ListStatusChangesOptions.new
options.include= 'recipients'
options.from_date ='11/1/2021'
options.to_date = '12/1/2021'
options.folder_ids = 'awaiting_my_signature'
search_results = envelopes_api.list_status_changes(account_id, options)
for envelope in search_results.envelopes
# do something useful
end
I hope you found this useful. As usual, if you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time…
Additional resources
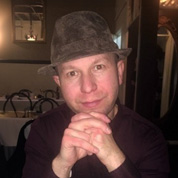
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
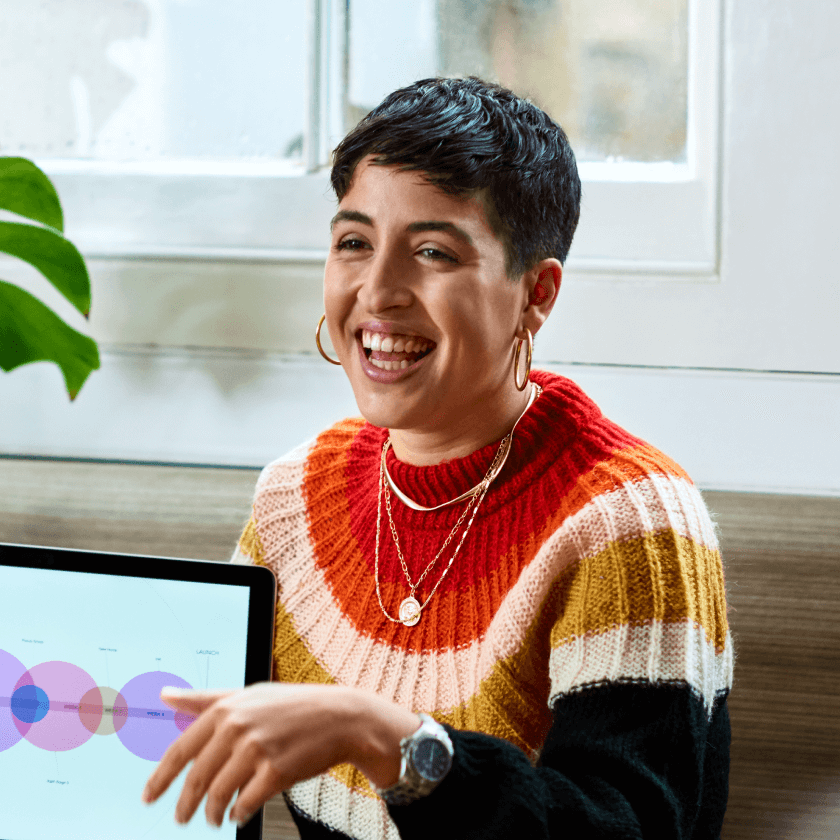