Common API Tasks🐈: Resend your envelope programmatically
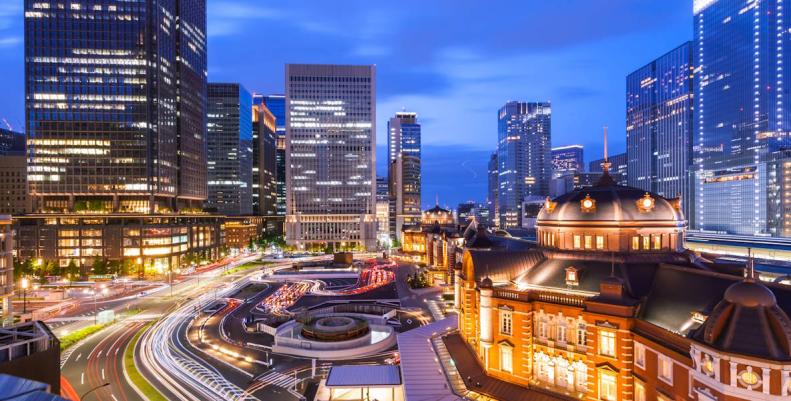
Welcome to a superb new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the DocuSign Developer Blog.
Generally speaking, there are two main ways to obtain signatures on envelopes. The first, is what we call embedded signing. When you use that approach, the signing takes place embedded inside your application and no emails or text messages are sent to the signers.
The second approach is to use remote signing: when you send your recipients either an email message or a text message (SMS) to inform them that they have to act on the envelope. They then have the option to complete their signing process asynchronously in the DocuSign eSignature web app, not as part of your application.
When your recipients haven’t acted on your initial messages, you can configure reminders for the envelopes as well, to make sure that your signers are informed again that they still need to act on the envelope. However, there’s also a way to trigger an ad-hoc message to resend the envelope to the same one or more recipients that need to act on it. That will send the same email message(s) and/or the same SMS message(s) to the recipient(s) that are next in line to sign the envelope.
As with most things in this blog series, you can absolutely do this from the DocuSign web application. When you select the Manage tab, you will find all the envelopes that are not yet complete and waiting for others and you can then select the RESEND button on the right to have it sent again to recipients.
You can also trigger the resend programmatically from your application. This can be useful if you want to provide a resend functionality inside your application or if you want to trigger the resend as an action that automatically occurs when something happens in your system. The option to resend an envelope requires that your integration has access to it. That means you can only resend an envelope you sent yourself or an envelope that was shared with you using the Shared Access feature.
The code below is used to resend an envelope. We’re using the endpoint to update an envelope but with the resend option set to true. We do not modify anything else on the envelope. Note that you cannot resend envelopes that are in draft or voided or completed status. The envelope must be in progress and awaiting the next set of recipients.
C#
var docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(docuSignClient);
string envelopeId = "967a1aad-xxxx-xxxx-xxxx-e162c10e01fc";
EnvelopesApi.UpdateOptions options = new EnvelopesApi.UpdateOptions();
options.resendEnvelope = "true";
Envelope envelope = new Envelope();
envelope.EnvelopeId = envelopeId;
envelopesApi.Update(accountId, envelopeId, envelope, options);
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
String envelopeId = "967a1aad-xxxx-xxxx-xxxx-e162c10e01fc";
UpdateOptions options = EnvelopesApi.new UpdateOptions();
options.setResendEnvelope("true");
Envelope envelope = new Envelope();
envelope.setEnvelopeId(envelopeId);
envelopesApi.update(accountId, envelopeId, envelope, options);
Node.js
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
let envelopeId = '967a1aad-xxxx-xxxx-xxxx-e162c10e01fc';
let options = { resendEnvelope: 'true' };
let envelope = new docusign.Envelope();
envelope.envelopeId = envelopeId;
envelopesApi.update(accountId, envelopeId, envelope, options);
PHP
$api_client = new \DocuSign\eSign\client\ApiClient($base_path);
$config = new \DocuSign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \DocuSign\eSign\Api\EnvelopesApi($api_client);
$envelope_id = '967a1aad-xxxx-xxxx-xxxx-e162c10e01fc';
$options = new \DocuSign\eSign\Api\EnvelopesApi\UpdateOptions();
$options->setResendEnvelope('true');
$envelope = new \DocuSign\eSign\Model\Envelope();
$envelope->setEnvelopeId($envelope_id);
$envelopes_api->update($account_id, $envelope_id, $envelope, $options);
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
envelope_id = '967a1aad-xxxx-xxxx-xxxx-e162c10e01fc'
envelope = Envelope()
envelope.envelope_id = envelope_id
envelopes_api.update(account_id, envelope_id, envelope=envelope, resend_envelope='true')
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
envelope_id = '967a1aad-xxxx-xxxx-xxxx-e162c10e01fc'
options = DocuSign_eSign::UpdateOptions.new
options.resend_envelope = 'true'
envelope = DocuSign_eSign::Envelope.new
envelope.envelope_id = envelope_id
envelopes_api.update(account_id, envelope_id, envelope=envelope,options)
That’s all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...