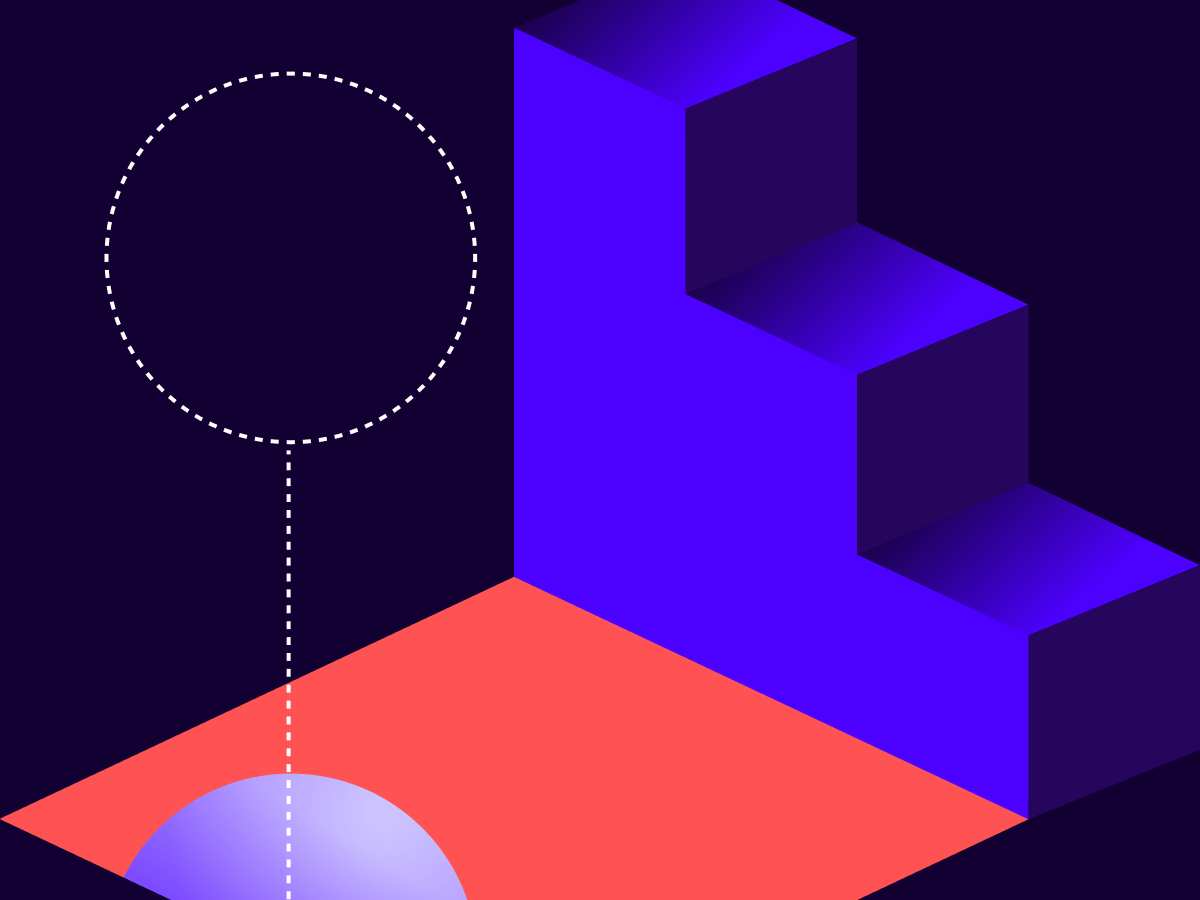
Common API Tasks🐈: Get Connect failure logs and attempt to retry webhook call
Learn how to use the Docusign eSignature REST API to retrieve webhook failures with code examples in six languages!
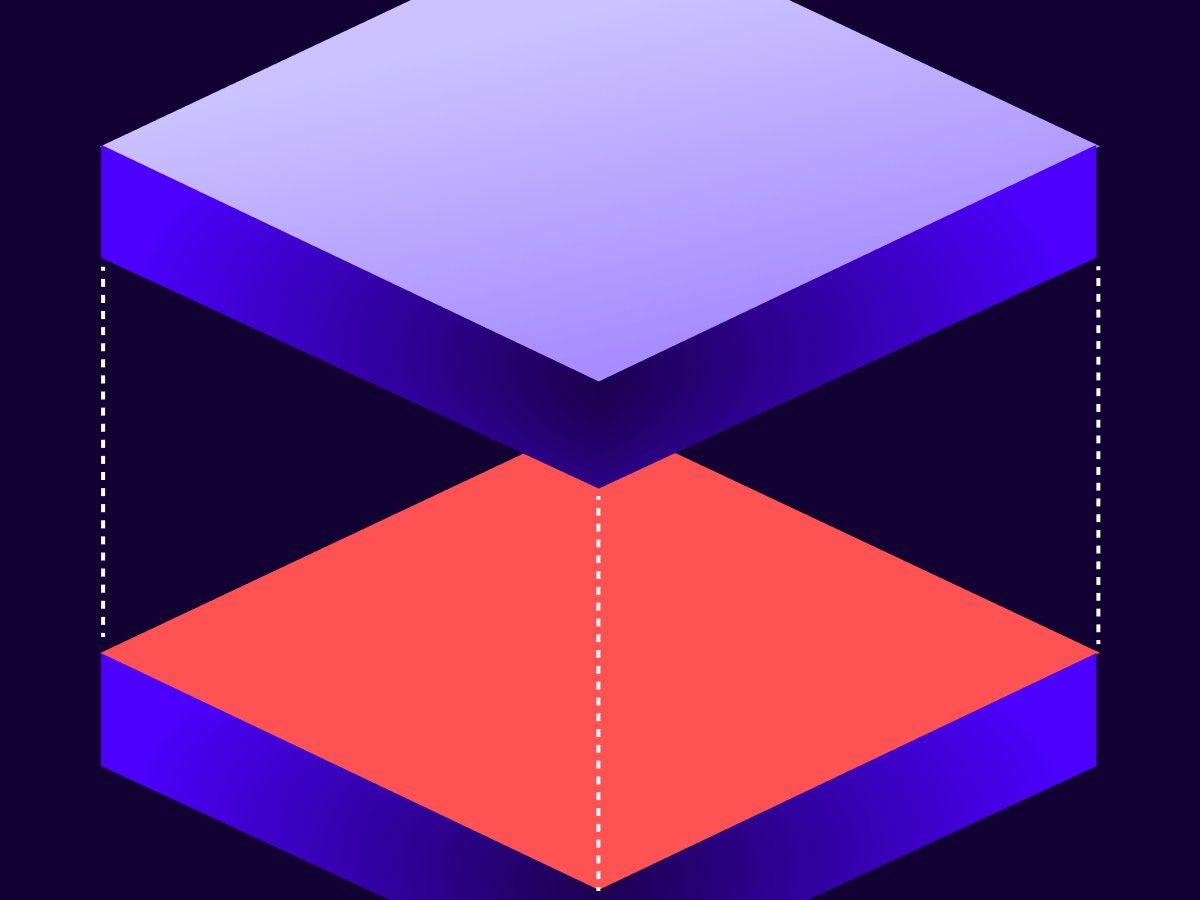
Last month I took a short break from the monthly CAT blog (Common API Tasks = CAT) in lieu of our landmark release of the new Developer Center website. This month we’re back in business with a new topic. If you remember, almost a year ago, I wrote a CAT blog post about how to add a webhook to your envelope such that you can get notifications for events related to your envelope from Docusign Connect, our webhook service. I hope many of you found it useful and started building integrations that utilize webhook technology.
Now, as we developers know, things don’t always work perfectly in the real world. Sometimes we need to debug issues, and webhook-related problems are up there on the list of things developers may need to deal with. Fortunately, Docusign provides a detailed log of your webhook calls with all the relevant information, including any failures that may occur. This log can be found using the Docusign Settings application (select Settings at the top when logged into your Docusign account) by selecting Connect from the left navigation menu. Select Failures to see the detailed logs of failed publishes. It looks like this:
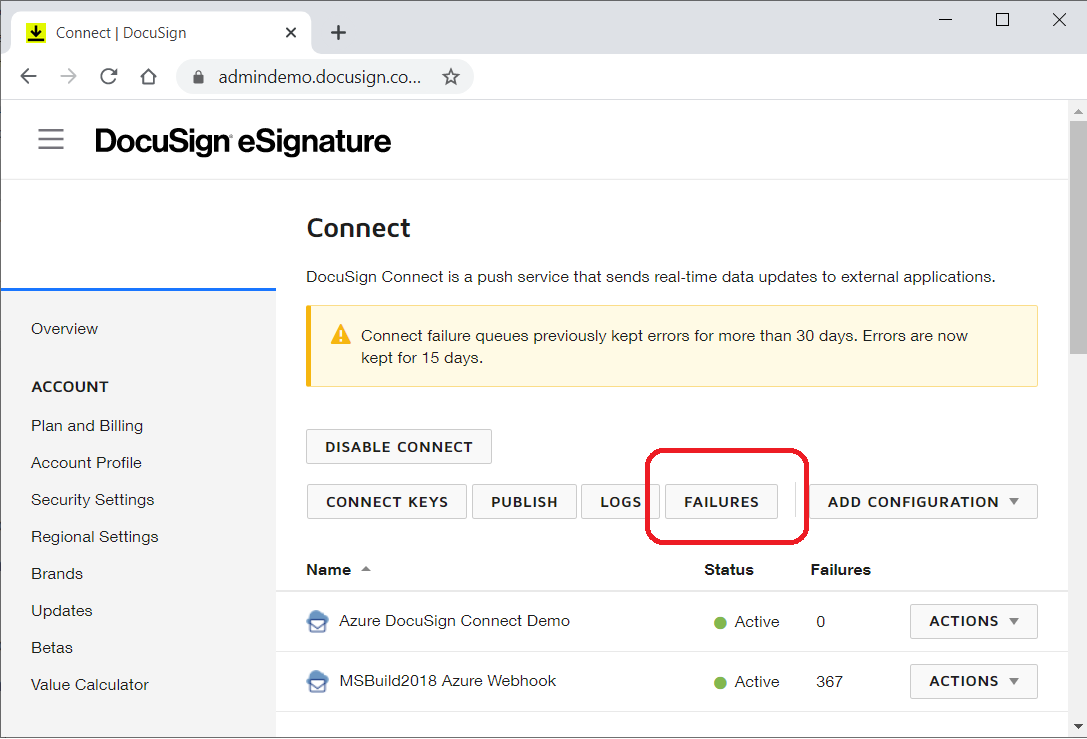
Figure 1: The connect page on Docusign Settings can be used to find Connect Logs
You can also, however, do everything programmatically. After all, this is what this blog series is all about. What I will show you here is how to obtain the list of Connect failures, and if there are, how to retry the failed webhook call.
You could use this mechanism as a backup for the automatic retry that Docusign performs for Connect webhook failures. Typically, if a webhook call fails (which means an error was received or an HTTP status code 400 and above) a retry would only happen after an ever-increasing time period from the last attempt (starting with 5 min and doubling to 15 days). This is done to avoid the queue being too busy with retry of existing issues instead of prioritizing new callbacks. However, if you know that your endpoint is likely to correctly handle the Docusign Connect webhook call, you can trigger the retry right away. Here's how this is done in code using our six SDKs languages.
C#
// You will need to obtain an access token using your chosen authentication flow
var apiClient = new ApiClient(basePath);
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
var connectApi = new ConnectApi(apiClient);
ConnectLogs connectLogs = connectApi.ListEventFailureLogs(accountId);
// See if any envelope webhook failed
if (connectLogs.Failures.Count > 0)
{
foreach (var failure in connectLogs.Failures)
{
// The Failure object includes a lot more information like LastTry, RetryCount, ConnectDebugLog and more...
Console.WriteLine("Connect failure - " + failure.Error);
// Try again right now
connectApi.RetryEventForEnvelope(accountId, failure.EnvelopeId);
}
}
Java
// You will need to obtain an access token using your chosen authentication flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
ConnectApi accountsApi = new ConnectApi(config);
ConnectLogs connectLogs = connectApi.listEventFailureLogs(accountId);
// See if any envelope webhook failed
if (connectLogs.Failures.length > 0)
{
for (int i = 0; i < connectLogs.Failures.length; i++)
{
// The Failure object includes a lot more information like lastTry, retryCount, connectDebugLog and more...
System.out.println("Connect failure - " + connectLogs.Failures[i].getError());
// Try again right now
connectApi.RetryEventForEnvelope(accountId, connectLogs.Failures[i].getEnvelopeId());
}
}
Node.js
// You will need to obtain an access token using your chosen authentication flow
let apiClient = new ApiClient(basePath);
let config = new docusign.Configuration(apiClient);
config.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let connectApi = new docusign.ConnectApi(config);
let connectLogs = connectApi.listEventFailureLogs(accountId);
// See if any envelope webhook failed
if (connectLogs.Failures.length > 0)
{
for (var i = 0; i < connectLogs.failures.length; i++)
{
// The Failure object includes a lot more information like lastTry, retryCount, connectDebugLog and more...
System.out.println("Connect failure - " + connectLogs.failures[i].error);
// Try again right now
connectApi.RetryEventForEnvelope(accountId, connectLogs.failures[i].envelopeId);
}
}
PHP
# You will need to obtain an access token using your chosen authentication flow
$api_client = new \Docusign\eSign\Client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$connect_api = new \Docusign\eSign\Api\ConnectApi($config);
$connect_logs = $connect_api->listEventFailureLogs($account_id);
# See if any envelope webhook failed
if (count($connect_logs->getFailures()) > 0)
{
for ($i = 0; $i < count($connect_logs->getFailures()); $i++)
{
# The Failure object includes a lot more information like LastTry, RetryCount, ConnectDebugLog and more...
print_r('Connect failure - ' + $connect_logs->getFailures()[$i]->getError());
# Try again right now
$connect_api->retryEventForEnvelope($account_id, $connect_logs->getFailures()[$i]->getEnvelopeId());
}
}
Python
# You will need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
connect_api = ConnectApi(config)
connect_logs = connect_api.list_event_failure_logs(account_id)
# See if any envelope webhook failed
if connect_logs.failures.len() > 0 :
for failure in connect_logs.failures :
# The Failure object includes a lot more information like last_try, retry_count, connect_debug_log and more...
print('Connect failure - ' + failure.error)
# Try again right now
connect_api.retry_event_for_envelope(account_id, failure.envelope_id)
Ruby
# You will need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
connect_api = DocuSign_eSign::ConnectApi.new api_client
connect_logs = connect_api.list_event_failure_logs(account_id)
# See if any envelope webhook failed
if connect_logs.failures.length() > 0
i = 0
while i < connect_logs.failures.length()
# The Failure object includes a lot more information like last_try, retry_count, connect_debug_log and more...
print('Connect failure - ' + connect_logs.failures[i].error)
# Try again right now
connect_api.retry_event_for_envelope(account_id, connect_logs.failures[i].envelope_id)
i++
end
end
I hope you found this useful. As usual, if you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
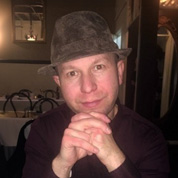
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
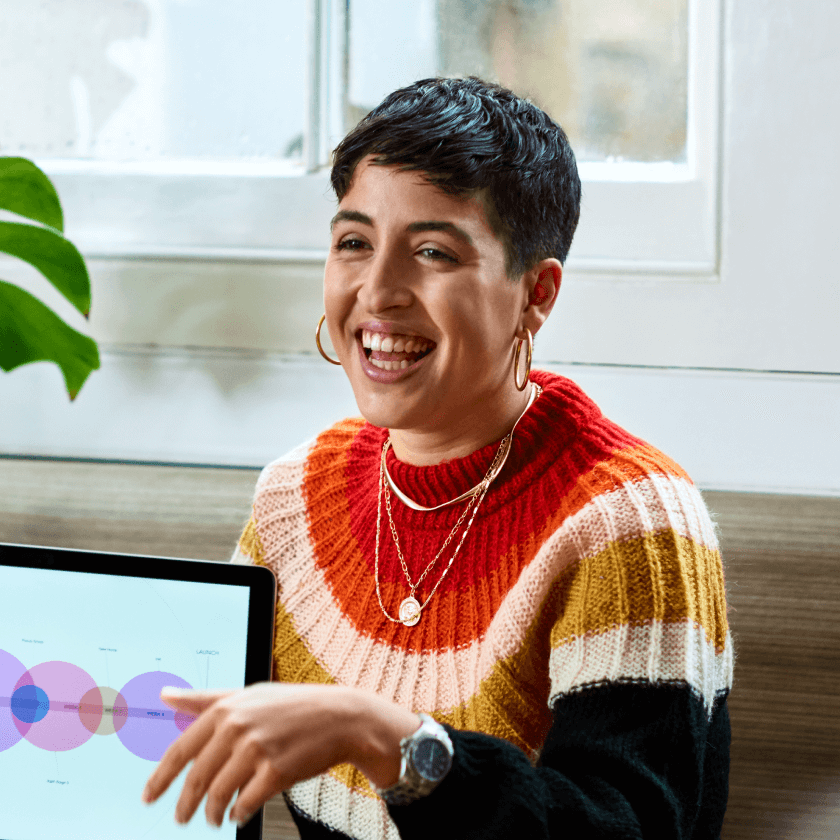