Common API Tasksđ: Check if your access token has expired
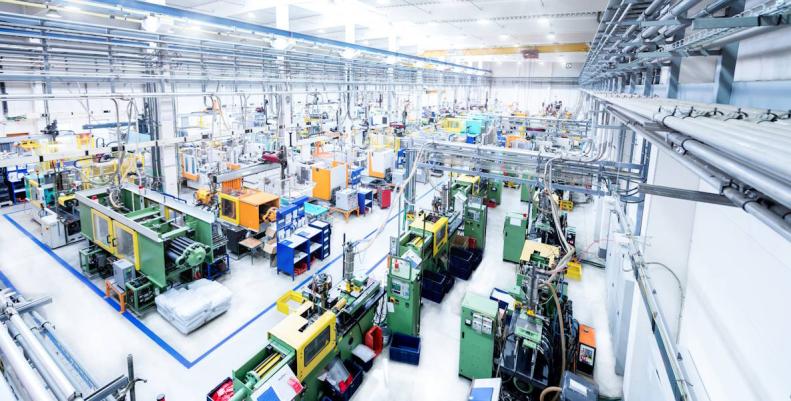
Welcome to a wondrous new edition of the CATđ (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the DocuSign Developer Blog.
Today weâre going back to the basics: authentication. As we all know, you cannot make API calls to DocuSign without obtaining an access token. That access token is required for any API call on any one of the DocuSign APIs. However, itâs important to be aware that access tokens have a short lifespan. They expire quickly, which is why you never hardcode an access token into your code. You never rely on an access token that was obtained in the past. It may not work! So, how do you know if the access token you obtained is valid? More specifically, how do you know if it has already expired or not?Â
When you obtain an access token, the JSON that comes back when you make a request includes another property in addition to the token itself. Itâs called expired_in and that is how long, in seconds, you have before the token expires. Itâs important to note that, as the famous saying goes, time waits for no man (or woman) which means this value becomes obsolete within a second of obtaining the token. So the best way to handle this is to have the next line after you obtain the token (and this is true for both JWT Grant or Authorization Code Grant) perform a simple calculation that tells you when the token expires by adding the number of seconds to the value of Now() (each language has a similar method). That way you have the exact time when the token expires in your system, and when you use that token, you can have a simple check to see if this time has passed or not (again using the Now() function, method, or property). If the token has not yet expired, you can use it. If the token expires, you will have to obtain a new one.Â
One thing to consider is that this check and the API call youâll make will take a few milliseconds, and to avoid a situation where the token had a few milliseconds left, you checked and the code says it has not expired, but by the time you made the call it did, itâs recommended that you give yourself an extra one second and not use a token that has less than one second before it expiresâjust in case.Â
OK, and now, here are the code snippets that do what I just described. I am not showing here how to obtain an access token. This has been documented exhaustively and discussed in detail on the Dev Center Authentication section. You can also use our Quickstart downloadable personalized code that helps you jumpstart your DocuSign API integration by setting up the needed configuration and authentication code for you automatically.
C#
OAuth.OAuthToken tokenInfo;
// Obtain the access token code not shown...
DateTime tokenExpiresTime;
tokenExpiresTime = DateTime.Now.AddSeconds(tokenInfo.expires_in ?? 0);
tokenExpiresTime = tokenExpiresTime.AddSeconds(-1); // One second just in case
// Your appâs code doing things ...
if (DateTime.Now < tokenExpiresTime)
{
// Token is good - you can use it to make API calls
}
else
{
// Token expired - obtain a new one
}
Java
OAuthToken tokenInfo;
// Obtain the access token code not shown...
LocalDateTime tokenExpiresTime;
tokenExpiresTime = LocalDateTime.now().plusSeconds(tokenInfo.expires_in);
tokenExpiresTime = tokenExpiresTime.plusSeconds(-1); // One second just in case
// Your appâs code doing things ...
if (LocalDateTime.now() < tokenExpiresTime)
{
// Token is good - you can use it to make API calls
}
else
{
// Token expired - obtain a new one
}
Node.js
let tokenInfo;
// Obtain the access token code not shown...
let tokenExpiresTime = moment().add(tokenInfo.body.expires_in, 's');
tokenExpiresTime = tokenExpiresTime.subtract(1, 's'); // One second just in case
// Your appâs code doing things ...
if (moment() < tokenExpiresTime)
{
// Token is good - you can use it to make API calls
}
else
{
// Token expired - obtain a new one
}
PHP
$token_info;
# Obtain the access token code not shown...
$token_expires_time = time()->add($token_info->getExpiresIn();
$token_expires_time = $token_expires_time->add(-1); # One second just in case
# Your appâs code doing things ...
if (time() < $token_expires_time)
{
# Token is good - you can use it to make API calls
}
else
{
# Token expired - obtain a new one
}
Python
token_info
# Obtain the access token code not shown...
token_expires_time = datetime.now() + timedelta(seconds = token_info.expires_in)
token_expires_time = token_expires_time + timedelta(seconds = -1); # One second just in case
# Your appâs code doing things ...
if datetime.now() < token_expires_time:
# Token is good - you can use it to make API calls
else:
# Token expired - obtain a new one
Ruby
token_info
# Obtain the access token code not shown...
token_expires_time = Time.now() + token_info.expires_in.to_i.seconds
token_expires_time = token_expires_time - 1.seconds; # One second just in case
# Your appâs code doing things ...
if Time.now() < token_expires_time
# Token is good - you can use it to make API calls
else
# Token expired - obtain a new one
end
Thatâs all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next timeâŚ