Common API Tasksš: Deliver documents securely using Docusign without requesting a signature
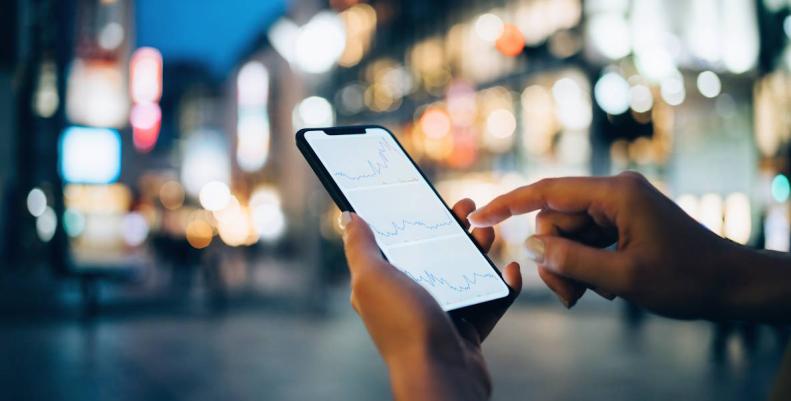
Welcome to a remarkable new edition of the CATš (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
Let me tell you a story. Recently I volunteered to be the treasurer of our Homeowners Association (HOA). Our HOW is very small, so we do our administrative work ourselves. One of my jobs as treasurer is to prepare and send the HOA annual dues to each homeowner. All I had when I started was a spreadsheet with everyoneās information and a Word document with a template of the annual statement. In prior years these statements were printed on dead trees and then mailed using āSnail Mailā to everyone. It took many weeks for everyone to even realize they received a statement, and if someone said they didnāt get it, there was no way to know what happened. We are too small to send all of these statements with certified mail; it would cost too much. Since I work for Docusign, I wanted to find out what could be done to improve the process. First, I wanted to eliminate paper. That one is obvious, and Iām sure you readers agree with me that we should try to avoid using paper whenever possible. So Docusign, right? Well, sure, we could use Document Generation and Bulk Send to take the spreadsheet I mentioned earlier and generate envelopes for each homeowner (assuming we know their email addresses or mobile numbers). However, we donāt really need them to sign anything, itās not a contract. We just want to know that they got it.Ā
That is where Certified Delivery comes into play. This is a feature that enables you to send an envelope with documents that donāt need to be signed. You do want to know that they saw them (they = the recipient of your envelope; you can combine this with IDV to verify their identity if you need that). In the web app this is a special type of recipient called Need to View, as you can see below:
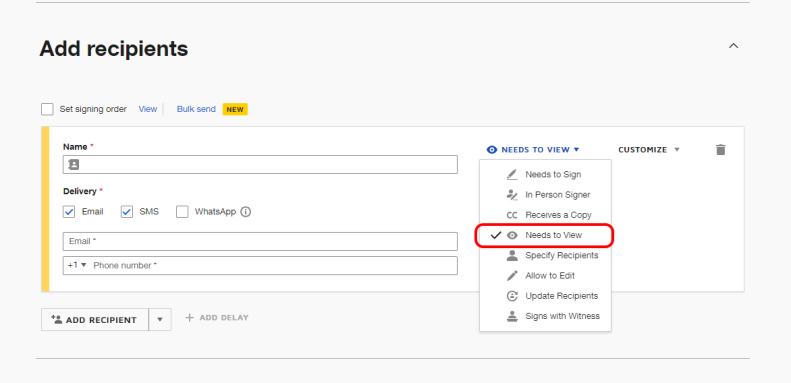
When you add a recipient like this and use Remote Signing (meaning you are not embedding it into your app, which is another way to use this feature) then the recipients will get the same type of email (or SMS, or WhatsApp). When they select the link in the message they get from Docusign, they are taken into the same type of UI youāre familiar with (which we usually call āSigningā) but they donāt need to sign; the envelope will be complete as soon as they load the document (assuming theyāre the only recipient). Easy peasy!
OK, so far for me talking, letās get to see some code, shall we?
C#
DocuSignClient docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(docuSignClient);
// Generate an object with the envelopeās metadata
EnvelopeDefinition envelopeDefinition = new EnvelopeDefinition();
envelopeDefinition.EmailSubject = "Your annual HOA statement";
envelopeDefinition.Status = "Sent";
// Add our document
envelopeDefinition.Documents = new List<Document>();
Document document = new Document();
document.Name = "Annual Statement";
document.DocumentBase64 = base64Doc; // Generate this string from the document
document.DocumentId = "1";
document.FileExtension = "pdf";
envelopeDefinition.Documents.Add(document);
// Add our recipient
envelopeDefinition.Recipients = new Recipients();
envelopeDefinition.Recipients.CertifiedDeliveries = new List<CertifiedDelivery>();
CertifiedDelivery certifiedDelivery = new CertifiedDelivery();
certifiedDelivery.Email = "inbar.gazit@docusign.com";
certifiedDelivery.Name = "Inbar Gazit";
certifiedDelivery.RecipientId = "1";
envelopeDefinition.Recipients.CertifiedDeliveries.Add(certifiedDelivery);
// Make the eSignature API call to send the envelope
EnvelopeSummary envelopeSummary = envelopesApi.CreateEnvelope(accountId, envelopeDefinition);
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
// Generate an object with the envelopeās metadata
EnvelopeDefinition envelopeDefinition = new EnvelopeDefinition();
envelopeDefinition.setEmailSubject("Your annual HOA statement");
envelopeDefinition.setStatus("Sent");
// Add our document
Document document = new Document();
document.setName("Annual Statement");
document.setDocumentBase64(base64Doc); // Generate this string from the document
document.setDocumentId("1");
document.setFileExtension("pdf");
envelopeDefinition.setDocuments().Add(Arrays.asList(document));
// Add our recipient
envelopeDefinition.setRecipients(new Recipients());
CertifiedDelivery certifiedDelivery = new CertifiedDelivery();
certifiedDelivery.setEmail("inbar.gazit@docusign.com");
certifiedDelivery.setName("Inbar Gazit");
certifiedDelivery.setRecipientId("1");
envelopeDefinition.getRecipients().setCertifiedDeliveries(Arrays.asList(certifiedDelivery));
// Make the eSignature API call to send the envelope
EnvelopeSummary envelopeSummary = envelopesApi.CreateEnvelope(accountId, envelopeDefinition);
Node.js
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
// Generate an object with the envelopeās metadata
let envelopeDefinition = new docusign.EnvelopeDefinition();
envelopeDefinition.emailSubject = 'Your annual HOA statement';
envelopeDefinition.status = 'Sent';
// Add our document
let document = new docusign.Document();
document.name = 'Annual Statement';
document.documentBase64 = base64Doc; // Generate this string from the document
document.documentId = '1';
document.fileExtension = 'pdf';
envelopeDefinition.documents = [document];
// Add our recipient
envelopeDefinition.Recipients = new docusign.Recipients();
let certifiedDelivery = new docusign.CertifiedDelivery();
certifiedDelivery.email = 'inbar.gazit@docusign.com';
certifiedDelivery.name = 'Inbar Gazit';
certifiedDelivery.recipientId = '1';
envelopeDefinition.recipients.certifiedDeliveries = [certifiedDelivery];
// Make the eSignature API call to send the envelope
let envelopeSummary = envelopesApi.createEnvelope(accountId, envelopeDefinition);
PHP
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \Docusign\eSign\Api\EnvelopesApi($api_client);
# Generate an object with the envelopeās metadata
$envelope_definition = new \Docusign\eSign\Model\EnvelopeDefinition();
$envelope_definition->setEmailSubject('Your annual HOA statement');
$envelope_definition->setStatus('Sent');
# Add our document
$document = new \Docusign\eSign\Model\Document();
$document->setName('Annual Statement');
$document->setDocumentBase64($base64_Doc); // Generate this string the from document
$document->setDocumentId('1');
$document->setFileExtension('pdf');
$envelope_definition->setDocuments().Add([$document]);
# Add our recipient
$envelope_definition->setRecipients(new \Docusign\eSign\Model\Recipients());
$certified_delivery = new \Docusign\eSign\Model\CertifiedDelivery();
$certified_delivery->setEmail('inbar.gazit@docusign.com');
$certified_delivery->setName('Inbar Gazit');
$certified_delivery->setRecipientId('1');
$envelope_definition->getRecipients()->setCertifiedDeliveries([$certified_delivery]);
# Make the eSignature API call to send the envelope
$envelope_summary = envelopesApi->CreateEnvelope($account_id, $envelope_definition);
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
# Generate an object with the envelopeās metadata
envelope_definition = EnvelopeDefinition()
envelope_definition.email_subject = 'Your annual HOA statement'
envelope_definition.status = 'Sent'
# Add our document
document = Document()
document.name = 'Annual Statement'
document.document_base64 = base64_doc; // Generate this string from the document
document.document_id = '1'
document.file_extension = 'pdf'
envelope_definition.documents = [document]
# Add our recipient
envelope_definition.recipients = Recipients()
certified_delivery = CertifiedDelivery()
certified_delivery.email = 'inbar.gazit@docusign.com'
certified_delivery.name = 'Inbar Gazit'
certified_delivery.recipient_id = '1'
envelope_definition.recipients.certified_deliveries = [certified_delivery]
# Make the eSignature API call to send the envelope
envelope_summary = envelopes_api.create_envelope(account_id, envelope_definition)
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
# Generate an object with the envelopeās metadata
envelope_definition = DocuSign_eSign::EnvelopeDefinition().new
envelope_definition.email_subject = 'Your annual HOA statement'
envelope_definition.status = 'Sent'
# Add our document
document = DocuSign_eSign::Document().new
document.name = 'Annual Statement'
document.document_base64 = base64_doc; // Generate this string from the document
document.document_id = '1'
document.file_extension = 'pdf'
envelope_definition.documents = [document]
# Add our recipient
envelope_definition.recipients = DocuSign_eSign::Recipients().new
certified_delivery = DocuSign_eSign::CertifiedDelivery().new
certified_delivery.email = 'inbar.gazit@docusign.com'
certified_delivery.name = 'Inbar Gazit'
certified_delivery.recipient_id = '1'
envelope_definition.recipients.certified_deliveries = [certified_delivery]
# Make the eSignature API call to send the envelope
envelope_summary = envelopes_api.create_envelope(account_id, envelope_definition)
Thatās all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...