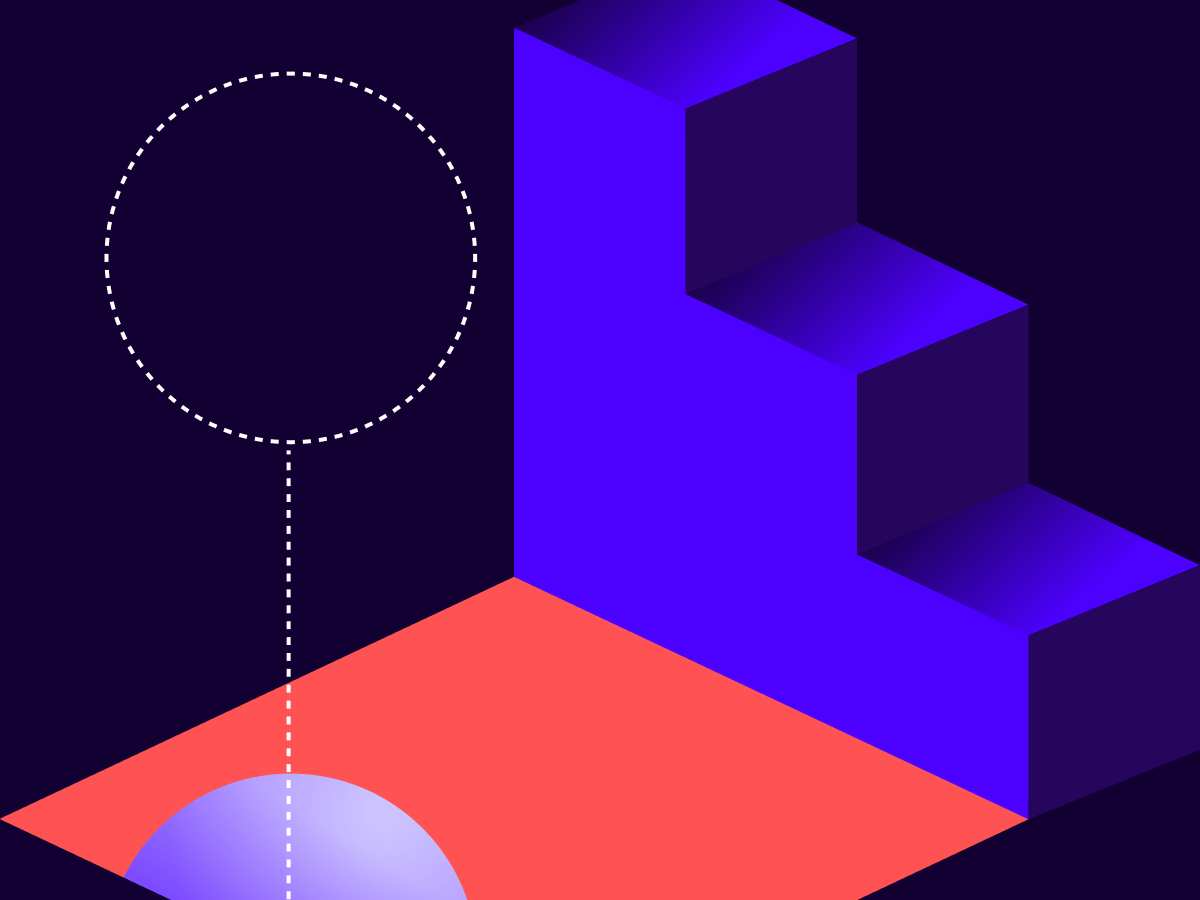
Common API Tasks🐈: Delete and undelete an envelope
See how to use the eSignature REST API to control to both delete an envelope and move it back out of the Recycling Bin into the Inbox.
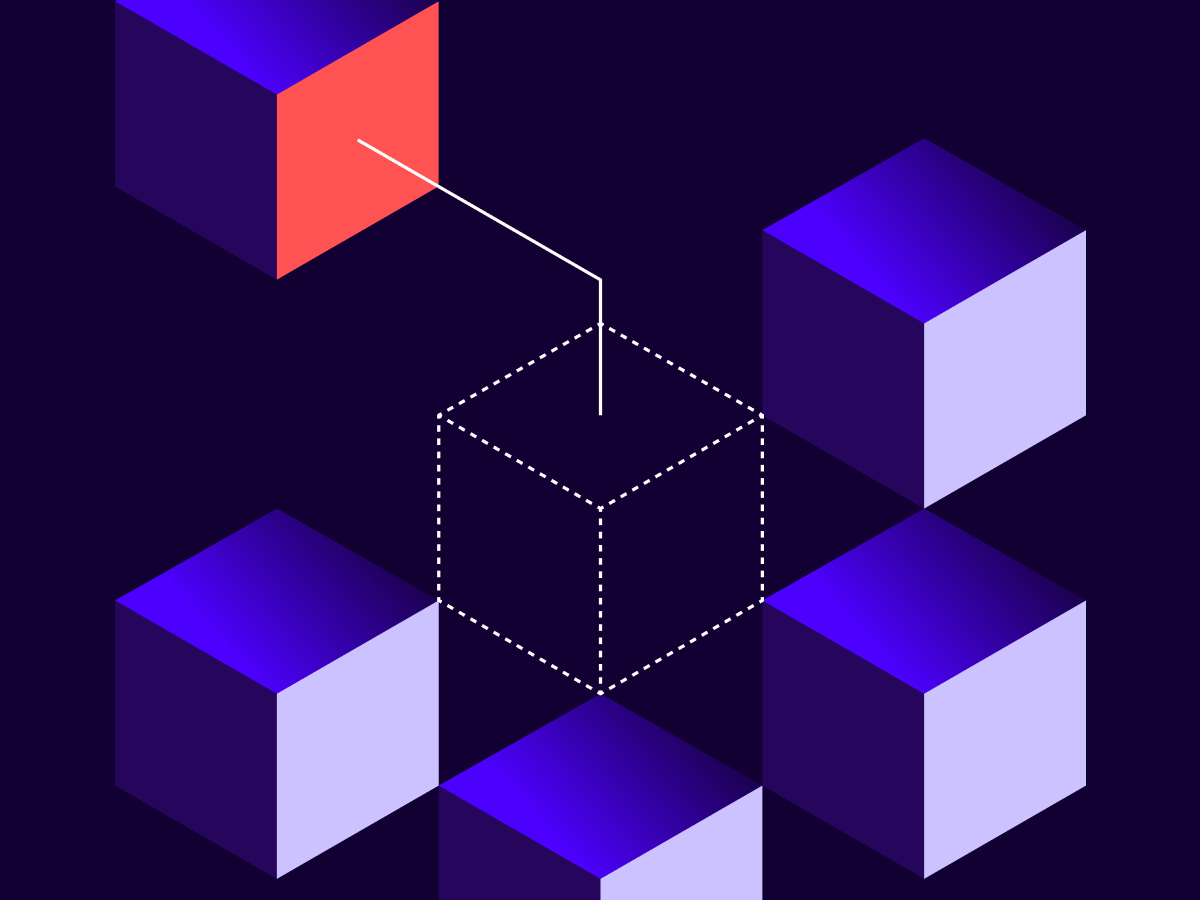
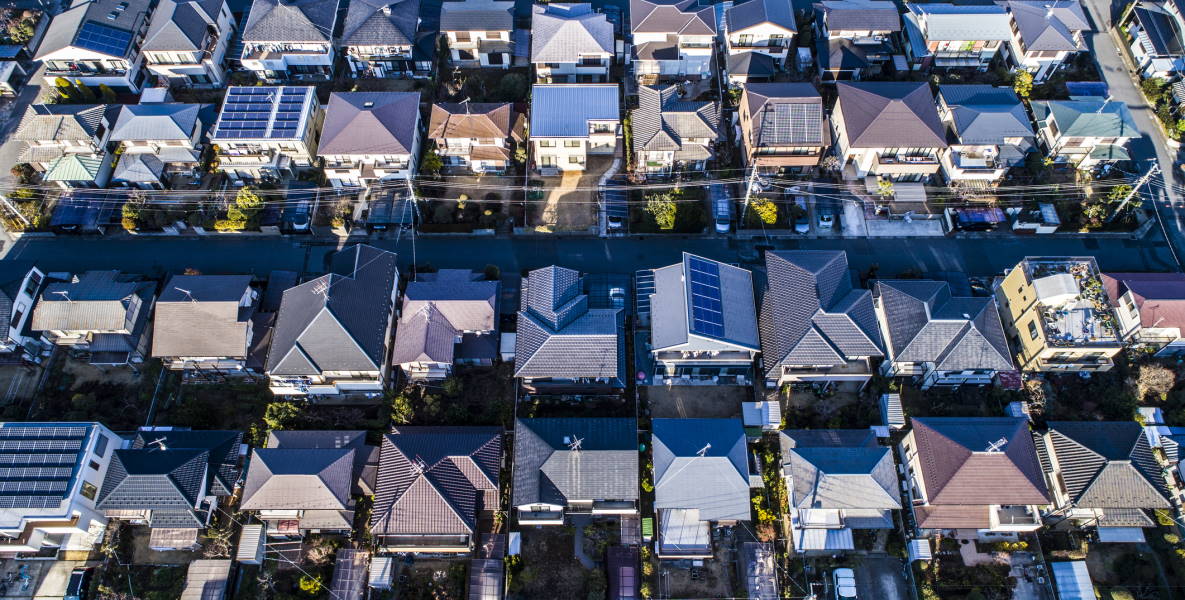
Welcome to an outstanding new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
Today we’re going to discuss deleting an envelope programmatically, and then restoring (or undeleting) it, in case your user made a mistake and wants to undo their action.
Note: Deleted envelopes are only kept for 24 hours in the Docusign Recycle Bin and then they are no longer accessible from your Docusign account, so undeleting an envelope can only be done during this time period.
Differences between voiding, deleting, and purging an envelope
All three operations—voiding, deleting, and purging an envelope—can be used to remove an envelope from being active in your account, but there are some differences between them.
Voiding an envelope just makes it invalid. However, you cannot void a completed envelope, as it has already been recorded as a complete transaction that was agreed upon among all sides. Only envelopes in progress can be voided. Voided envelopes cannot be “unvoided” even if you voided them by mistake: this is a one-way action. Deleting, on the other hand, allows you to undelete the envelope.
You can delete envelopes in any state (draft, in progress, or complete), and the essential meaning of deleting is moving the envelope temporarily into a special folder called “Recycle Bin” (You can find it by selecting Deleted on the Manage tab of your web app.)
Purging, on the other hand, is a much more destructive action than deleting. Once an envelope has been purged, there’s no way to undo this operation even if you contact Customer Support. The envelope and its contents are lost forever. This is not the case for deleted envelopes. These envelopes are still accessible to Docusign and you can restore them by contacting Customer Support and submitting a case.
Now, let’s get to some code, shall we? I’m going to show you code snippets in the usual six languages. Note that each of these code snippets does both the deletion and immediately the undeletion (technically, you are moving the envelope to the Inbox folder). So running this code as written gets your envelope right back to where it came from (assuming it was in your inbox), but feel free to take just the code you need and add it to your application as appropriate. And without further ado, here is the code:
C#
// Delete an envelope
ApiClient apiClient = new ApiClient(basePath);
// You will need to obtain an access token using your chosen authentication method
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
FoldersApi foldersApi = new FoldersApi(apiClient);
string envelopeId = "9e2b1deb-xxxx-xxxx-xxxx-2540322a31c4";
FoldersRequest folderRequest = new FoldersRequest();
folderRequest.EnvelopeIds = new List<string>();
folderRequest.EnvelopeIds.Add(envelopeId);
foldersApi.MoveEnvelopes(accountId, "recyclebin", folderRequest);
// Move the deleted envelope back to the Inbox folder
string inboxFolderId = "";
string recycleBinFolderId = "";
FoldersResponse foldersResponse = foldersApi.List(accountId);
// Search for the folders you are interested in
foreach (Folder folder in foldersResponse.Folders)
{
if (folder.Name == "Inbox")
{
inboxFolderId = folder.FolderId;
}
else if (folder.Name == "Deleted Items")
{
recycleBinFolderId = folder.FolderId;
}
}
folderRequest.FromFolderId = recycleBinFolderId;
folderRequest.EnvelopeIds = new List<string>();
folderRequest.EnvelopeIds.Add(envelopeId);
foldersApi.MoveEnvelopes(accountId, inboxFolderId, folderRequest);
</string></string>
Java
// Delete an envelope
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
FoldersApi foldersApi = new FoldersApi(config);
String envelopeId = "9e2b1deb-xxxx-xxxx-xxxx-2540322a31c4";
FoldersRequest folderRequest = new FoldersRequest();
folderRequest.setEnvelopeIds(new java.util.ArrayList<String>());
folderRequest.getEnvelopeIds().add(envelopeId);
foldersApi.MoveEnvelopes(accountId, "recyclebin", folderRequest);
// Move the deleted envelope back to the Inbox folder
String inboxFolderId = "";
String recycleBinFolderId = "";
FoldersResponse foldersResponse = foldersApi.List(accountId);
// Search for the folders you are interested in
for (Folder folder : foldersResponse.getFolders())
{
if (folder.getName() == "Inbox")
{
inboxFolderId = folder.getFolderId();
}
else if (folder.getName() == "Deleted Items")
{
recycleBinFolderId = folder.getFolderId();
}
}
folderRequest.setFromFolderId(recycleBinFolderId);
folderRequest.setEnvelopeIds(new java.util.ArrayList<String>());
folderRequest.getEnvelopeIds().add(envelopeId);
foldersApi.MoveEnvelopes(accountId, inboxFolderId, folderRequest);
Node.js
// Delete an envelope
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let foldersApi = new docusign.FoldersApi(apiClient);
let envelopeId = '9e2b1deb-xxxx-xxxx-xxxx-2540322a31c4';
let folderRequest = new docusign.FoldersRequest();
folderRequest.EnvelopeIds = [envelopeId];
foldersApi.moveEnvelopes(accountId, 'recyclebin', 'foldersRequest' : folderRequest);
// Move the deleted envelope back to the Inbox folder
let foldersResponse = foldersApi.list(accountId);
// Search for the folders you are interested in
foldersResponse.folders.forEach(folder =>
{
if (folder.name == 'Inbox')
{
inboxFolderId = folder.folderId;
}
else if (folder.name == 'Deleted Items')
{
recycleBinFolderId = folder.folderId;
}
});
folderRequest.fromFolderId = recycleBinFolderId;
folderRequest.EnvelopeIds = [envelopeId];
foldersApi.moveEnvelopes(accountId, inboxFolderId, folderRequest);
PHP
# Delete an envelope
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$folders_api = new FoldersApi(config);
$envelope_id = '9e2b1deb-xxxx-xxxx-xxxx-2540322a31c4';
$folder_request = new \Docusign\eSign\model\FoldersRequest();
$folder_Request->setEnvelopeIds([$envelope_id]);
$folders_api->moveEnvelopes($account_id, 'recyclebin', $folder_request);
# Move the deleted envelope back to the Inbox folder
$folders_response = $folders_api->list($account_id);
# Search for the folders you are interested in
for ($folders_response->getFolders() as $folder)
{
if ($folder->getName() == 'Inbox')
{
$inbox_folder_id = $folder->getFolderId();
}
elseif ($folder->getName() == 'Deleted Items')
{
$recycle_bin_folder_id = $folder->getFolderId();
}
}
$folder_request->setFromFolderId($recycle_bin_folder_id);
$folder_request->setEnvelopeIds([$envelope_id]);
$folders_api->moveEnvelopes($account_id, $inbox_folder_id, $folder_request);
Python
# Delete an envelope
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
folders_api = FoldersApi(apiClient)
envelope_id = '9e2b1deb-xxxx-xxxx-xxxx-2540322a31c4'
folder_request = FoldersRequest()
folder_request.envelope_ids = [envelope_id]
foldersApi.move_envelopes(account_id, 'recyclebin', folder_request)
# Move the deleted envelope back to the Inbox folder
folders_response = folders_api.list(account_id)
# Search for the folders you are interested in
for folder in folders_response.folders:
if folder.name == 'Inbox':
inbox_folder_id = folder.folder_id
elif folder.name == 'Deleted Items':
recycle_bin_folder_id = folder.folder_id
folder_request.from_folder_id = recycle_bin_folder_id
folder_request.envelope_ids = [envelope_id]
folders_api.move_envelopes(account_id, inbox_folder_id, folder_request)
Ruby
# Delete an envelope
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
folders_api = DocuSign_eSign::FoldersApi.new apiClient
envelope_id = '9e2b1deb-xxxx-xxxx-xxxx-2540322a31c4'
folder_request = DocuSign_eSign::FoldersRequest.new
folder_request.envelope_ids = [envelope_id]
foldersApi.move_envelopes(account_id, 'recyclebin', folder_request)
# Move the deleted envelope back to the Inbox folder
folders_response = folders_api.list(account_id)
# Search for the folders you are interested in
Folders_response.folders.each
{ |folder|
if (folder.name == 'Inbox')
{
inbox_folder_id = folder.folder_id
}
elsif (folder.name == 'Deleted Items')
{
recycle_bin_folder_id = folder.folder_id
}
}
folder_request.from_folder_id = recycle_bin_folder_id
folder_request.envelope_ids = [envelope_id]
folders_api.move_envelopes(account_id, inbox_folder_id, folder_request)
That’s all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
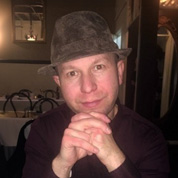
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
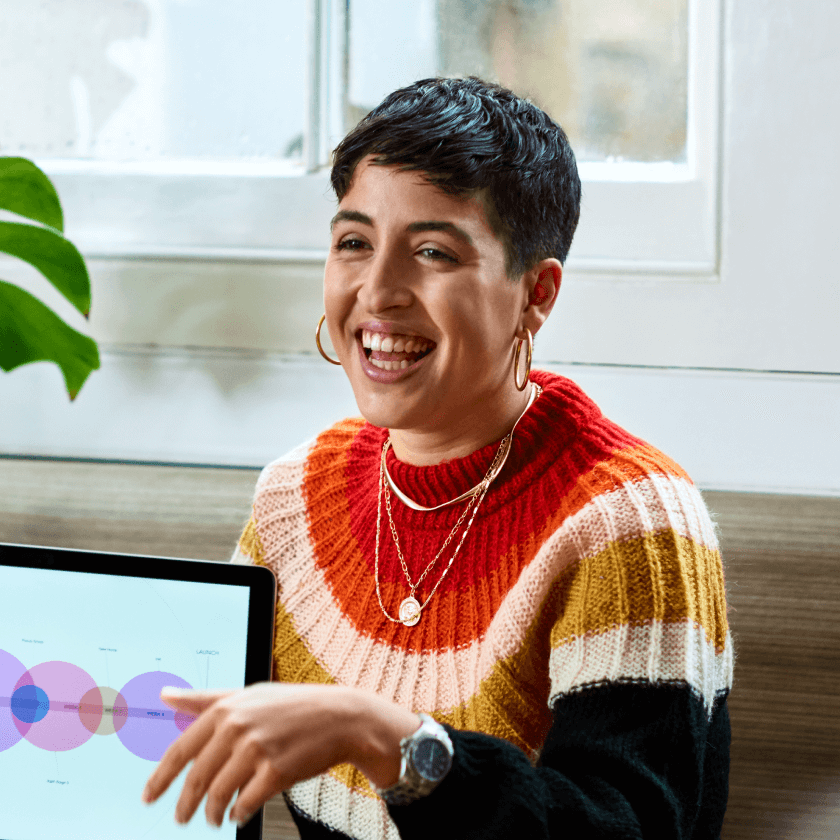