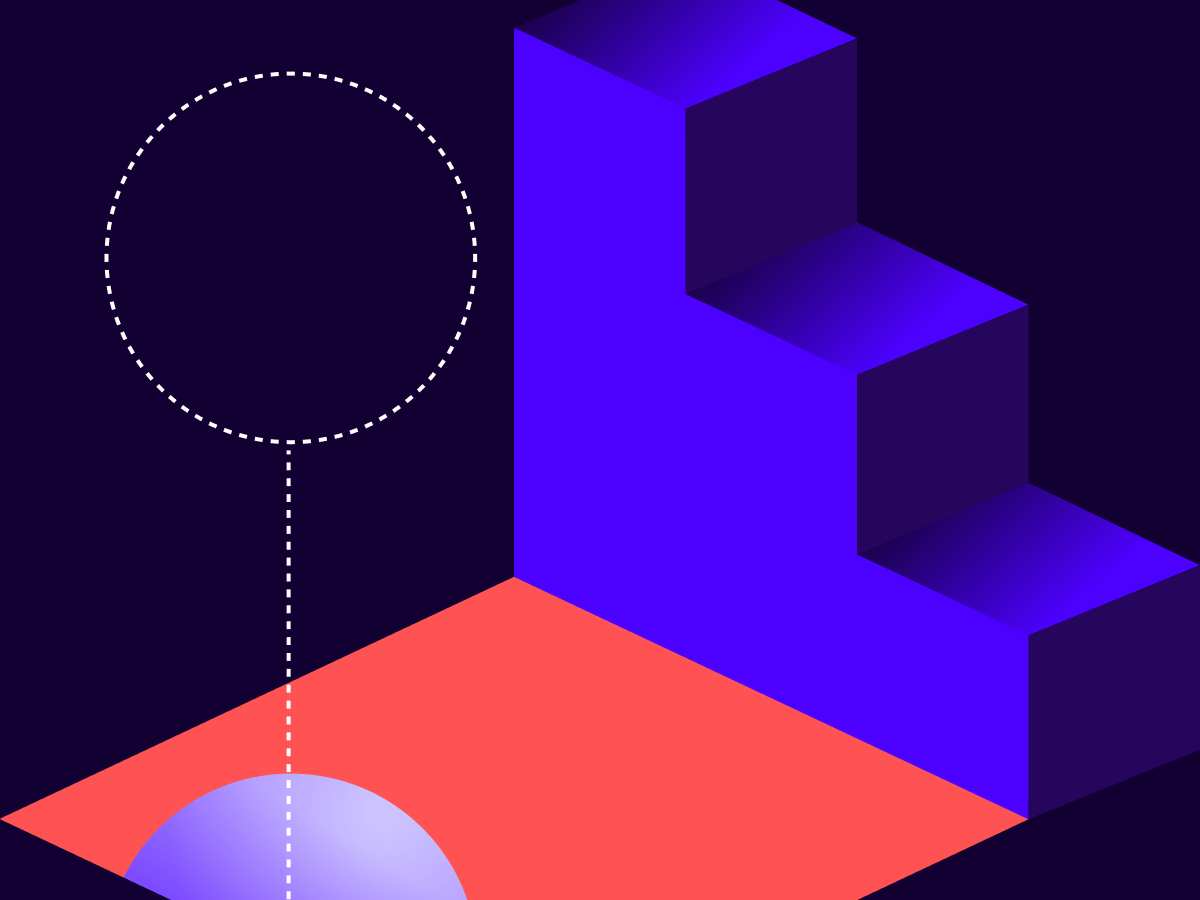
Common API Tasks🐈: Purging envelopes from your Docusign account
See how to remove/purge envelopes and their metadata from your eSignature account using the Docusign eSignature REST API
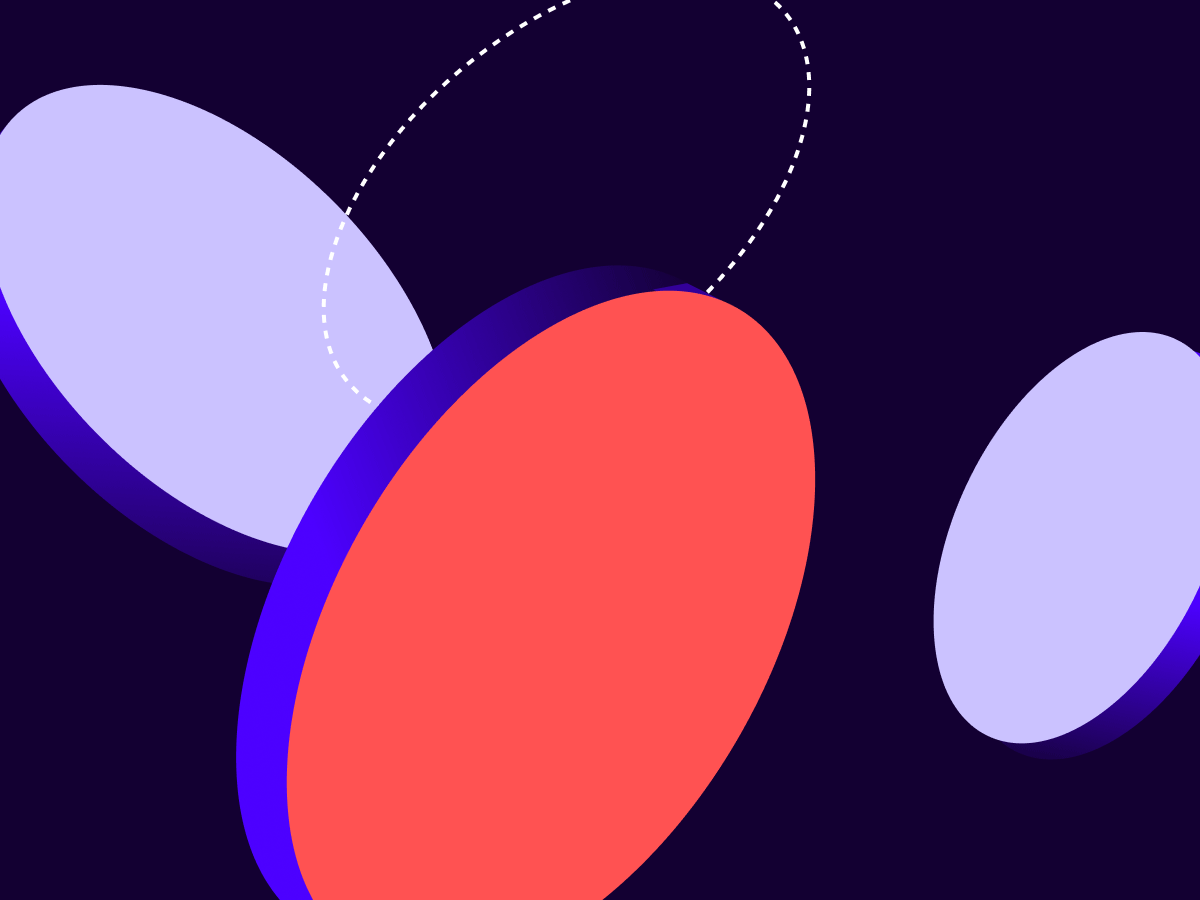
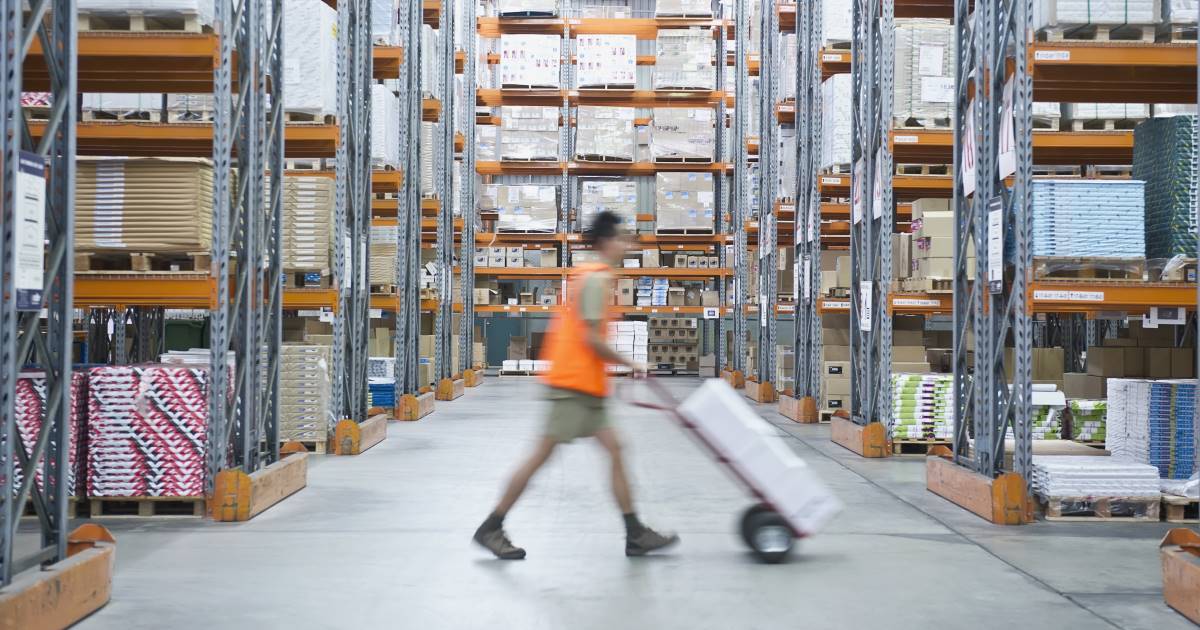
Welcome to a wonderful new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
In this blog post I'm going to discuss document retention and the ability to remove unwanted envelopes (along with the documents and data they contain) from your Docusign account permanently.
Generally speaking, Docusign stores all of your production environment envelope data in the cloud permanently (in the developer environment, your envelopes are removed after 30 days.) However, you can define your own document retention policy to have Docusign purge envelopes automatically at a given time. You can also manually purge envelopes from the Docusign eSignature Admin Settings menu by selecting Document Retention in the left menu and selecting TARGETED PURGE, then picking the envelopes to be purged (only completed or voided envelopes can be purged).
If you can do something manually from the Docusign web app, you can also do it using one of the Docusign APIs. This is where I come in. Let’s see how you can write code to purge a specific envelope. To be clear, you don’t get to purge an envelope immediately, but instead you add it to a purge queue for up to 14 days. Docusign will purge this envelope when it gets to the top of the queue. You’ll need your envelope’s envelopeID
(a unique GUID identifying it in the system) and you need to choose which parts of the envelope to purge and which to keep. This is decided by the purgeState parameter, which you can set to one of the following string values:
documents_queued
: Only the documents in the envelope will be removeddocuments_and_metadata_queued
: Documents and any metadata such as tabs or custom fields will be removeddocuments_and_metadata_and_redact_queued
: Documents and any metadata such as tabs or custom fields will be removed. In addition, any PII (Personal Identifiable Information) such as names, or email addresses will be redacteddocuments_dequeued
: This value is used to undo one of the previous three actions and remove the envelope from the queue. As long as the envelope hasn’t been purged yet, you can use this action to restore it
The code snippets presented here are very simple. They will add a single envelope to the purge queue (adding multiple envelopes requires multiple API calls). I choose to use documents_and_metadata_and_redact_queued
for this example, but you can change this value as needed. Note that the envelope you’re trying to purge must be in either completed or void status and the user account authenticated to make API calls must be the sender of the envelope or has permission to this envelope exclipicly granted.
C#
var apiClient = new ApiClient(basePath);
// You will need to obtain an access token using your chosen authentication method
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
string envId = "0e3b78b9-xxxx-xxxx-xxxx-dbf35ba8f58a";
Envelope env = new Envelope();
env.EnvelopeId = envId;
env.PurgeState = "documents_and_metadata_and_redact_queued";
envelopesApi.Update(accountId, envId, env);
Java
// You will need to obtain an access token using your chosen authentication flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(config);
String envId = "0e3b78b9-xxxx-xxxx-xxxx-dbf35ba8f58a";
Envelope env = new Envelope();
env.setEnvelopeId(envId);
env.setPurgeState("documents_and_metadata_and_redact_queued");
envelopesApi.Update(accountId, envId, env);
Node.js
// You will need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(apiClient);
let envId = '0e3b78b9-xxxx-xxxx-xxxx-dbf35ba8f58a';
let env = new docusign.Envelope();
env.envelopeId = envId;
env.purgeState = 'documents_and_metadata_and_redact_queued';
envelopesApi.update(accountId, envId, env);
PHP
# You will need to obtain an access token using your chosen authentication flow
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \Docusign\eSign\Api\EnvelopesApi($api_client);
$env_id = '0e3b78b9-xxxx-xxxx-xxxx-dbf35ba8f58a';
$env = new \Docusign\eSign\Model\Envelope();
$env->setEnvelopeId($env_id);
$env->setPurgeState('documents_and_metadata_and_redact_queued');
$envelopes_api->update($account_id, $env_id, $env);
Python
# You will need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
env_id = '0e3b78b9-xxxx-xxxx-xxxx-dbf35ba8f58a'
env = Envelope()
env.envelope_id = env_id
env.purge_state = 'documents_and_metadata_and_redact_queued'
envelopes_api.update(account_id, env_id, env)
Ruby
# You will need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
env_id = '0e3b78b9-xxxx-xxxx-xxxx-dbf35ba8f58a'
env = Envelope.new
env.envelope_id = env_id
env.purge_state = 'documents_and_metadata_and_redact_queued'
envelopes_api.update(account_id, env_id, env)
That’s all folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
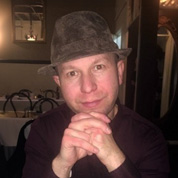
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
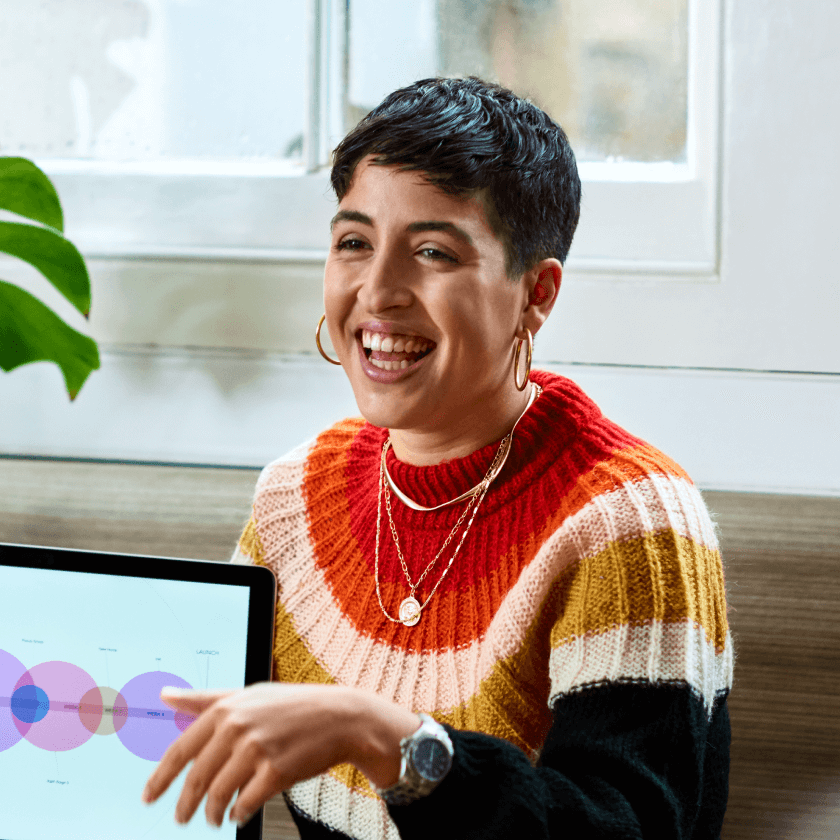