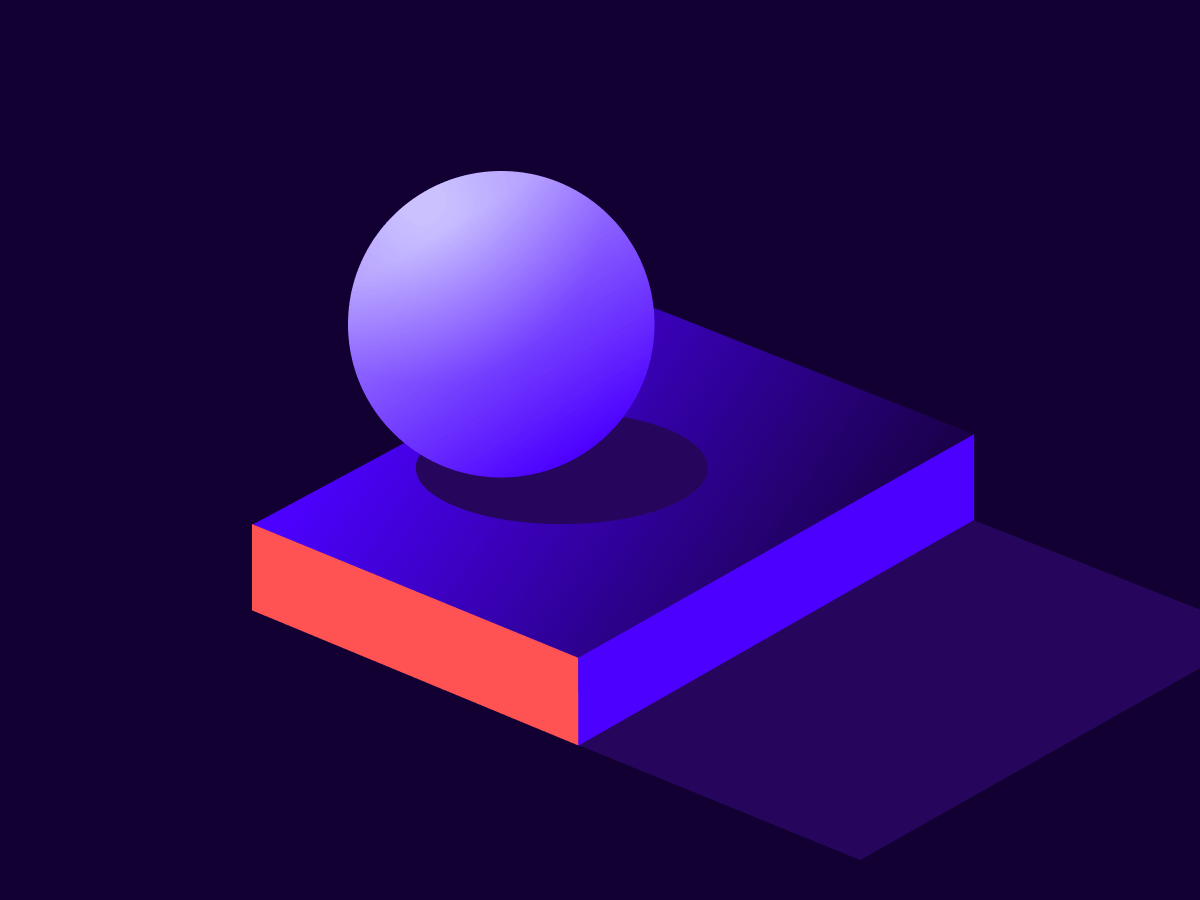
Common API Tasks🐈: add custom Connect configuration programmatically
Use the eSignature REST API to add a Connect configuration to your account programmatically.
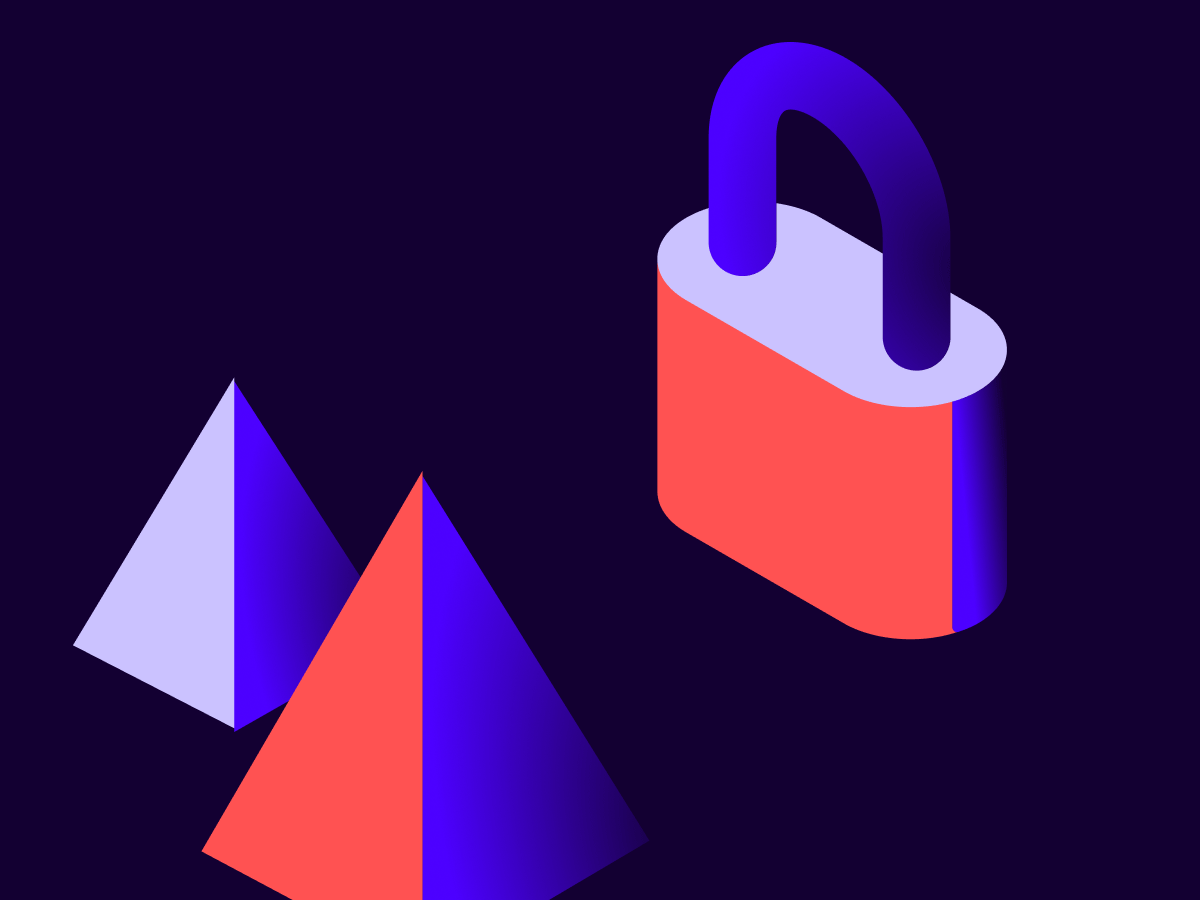
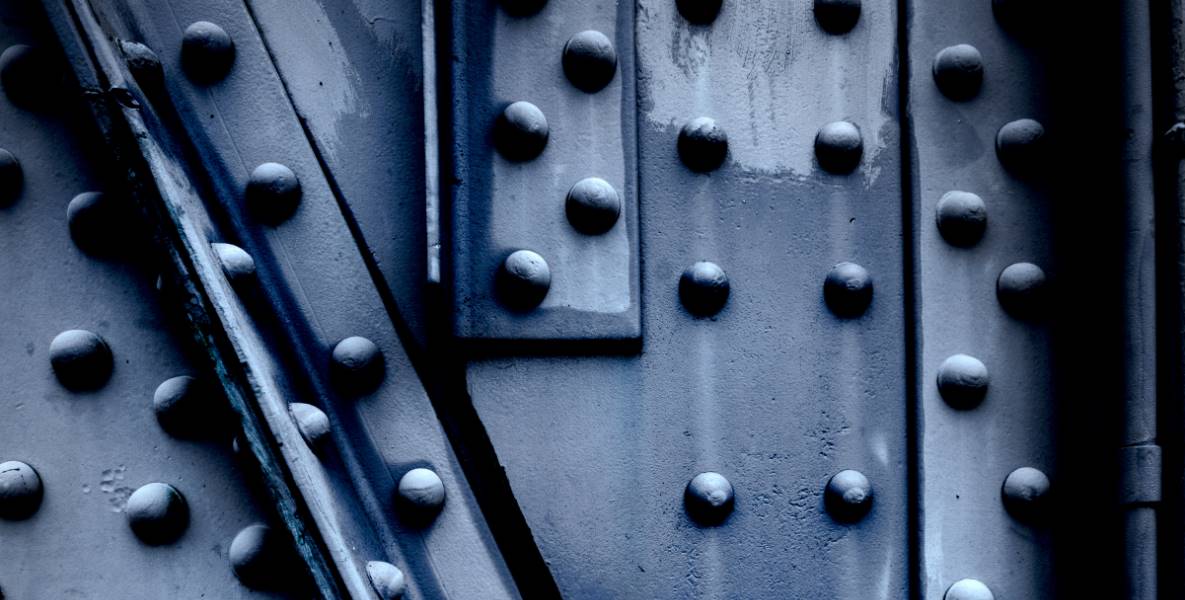
Welcome to a fabulous new episode of the CAT🐈 (Common API Tasks) blog. This blog series is about giving you all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign developer blog.
In one of the earlier CAT blogs, I showed you how you can use the eSignature REST API to add a Docusign Connect webhook for your envelopes. In that article, I explained the need for webhooks and what Docusign Connect is. Today I’m going to show a different way to use this functionality. This different approach enables you to add what we call a Connect configuration to your account so that all envelopes sent from this account will get the webhook you configured. This approach is required to support some features of Docusign Connect, such as using JSON format, securing your calls with HMAC key, and more.
Like many things I show you how to do programmatically, adding a Connect configuration can be done via the web UI. You can read more about how to use the web UI to add a Connect configuration in our Support Center.
Here are some of the basic considerations for your code:
Webhook URL: this is your URL where you want events to publish to. It must be secure with https (TLS) protocol. It must also be accessible from the internet. Tip: use a cloud provider instead of your own server.
Envelope Events and Recipient Events: At this time only these types of events are supported. The list represents the envelope or recipient statuses that you want to be notified about.
EnableLog:- highly recommend you set this to true so you can see Connect logs in the Docusign web app to enable troubleshooting of any potential issues.
Version: You should always use
"restv2.1"
, which means you’ll get back event data in JSON and not XML format.
Finally it’s important to mention that when calling the code below (using the ConnectionConfigurations::Create endpoint), you must be authenticated as a user that has administrator privileges to the Docusign account. If you create a new developer account, you’ll have that access level automatically; but you may not always have this level of access, especially if you are using the production environment.
So, where is the code?
C#
var apiClient = new ApiClient(basePath);
// You need to obtain an access token using your chosen authentication flow
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
var connectApi = new ConnectApi(apiClient);
var connectEventData = new ConnectEventData();
connectEventData.Version = "restv2.1";
var connectCustomConfiguration = new ConnectCustomConfiguration();
connectCustomConfiguration.Name = "my custom connect configuration";
connectCustomConfiguration.ConfigurationType = "custom";
connectCustomConfiguration.AllUsers = "true";
connectCustomConfiguration.IncludeHMAC = "false";
connectCustomConfiguration.EnableLog = "true";
// The URL you publish events to (your webhook) must be TLS-secure (https protocol)
connectCustomConfiguration.UrlToPublishTo = "https://www.example.com/cat/meow";
connectCustomConfiguration.EventData = connectEventData;
connectCustomConfiguration.EnvelopeEvents = new List<string> { "Completed" };
connectApi.CreateConfiguration(accountId, connectCustomConfiguration);
</string>
Java
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
ConnectApi connectApi = new ConnectApi(config);
ConnectEventData connectEventData = new ConnectEventData();
connectEventData.Version = "restv2.1";
ConnectCustomConfiguration connectCustomConfiguration = new ConnectCustomConfiguration();
connectCustomConfiguration.setName("my custom connect configuration");
connectCustomConfiguration.setConfigurationType("custom");
connectCustomConfiguration.setAllUsers("true");
connectCustomConfiguration.setIncludeHMAC("false");
connectCustomConfiguration.setEnableLog("true");
// The URL you publish events to (your webhook) must be TLS-secure (https protocol)
connectCustomConfiguration.setUrlToPublishTo("https://www.example.com/cat/meow");
connectCustomConfiguration.setEventData(connectEventData);
java.util.List<string> envelopeEventsList = new java.util.List<string>();
envelopeEventsList.add("Completed");
connectCustomConfiguration.setEnvelopeEvents(envelopeEventsList);
connectApi.CreateConfiguration(accountId, connectCustomConfiguration);
</string></string>
Node.js
// You need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let connectApi = new docusign.ConnectApi(dsApiClient);
let connectEventData = new docusign.ConnectEventData();
connectEventData.version = 'restv2.1';
let connectCustomConfiguration = new docusign.ConnectCustomConfiguration();
connectCustomConfiguration.name = 'my custom connect configuration';
connectCustomConfiguration.configurationType = 'custom';
connectCustomConfiguration.allUsers = 'true';
connectCustomConfiguration.includeHMAC = 'false';
connectCustomConfiguration.enableLog = 'true';
// The URL you publish events to (your webhook) must be TLS-secure (https protocol)
connectCustomConfiguration.urlToPublishTo = 'https://www.example.com/cat/meow';
connectCustomConfiguration.eventData = connectEventData;
connectCustomConfiguration.envelopeEvents = [ 'Completed' ];
connectApi.createConfiguration(accountId, connectCustomConfiguration);
PHP
# You need to obtain an access token using your chosen authentication flow
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$api_client = new \Docusign\eSign\client\ApiClient($config);
$connect_api = new \Docusign\Api\ConnectApi($api_client);
$connect_event_data = new \Docusign\eSign\Model\ConnectEventData();
$connect_event_data->setVersion('restv2.1');
$connect_custom_configuration = new \Docusign\eSign\Model\ConnectCustomConfiguration();
$connect_custom_configuration->setName('my custom connect configuration');
$connect_custom_configuration->setConfigurationType('custom');
$connect_custom_configuration->setAllUsers('true');
$connect_custom_configuration->setIncludeHmac('false');
$connect_custom_configuration->setEnableLog('true');
# The URL you publish events to (your webhook) must be TLS-secure (https protocol)
$connect_custom_configuration->setUrlToPublishTo('https://www.example.com/cat/meow');
$connect_custom_configuration->setEventData($connect_event_data);
$connect_custom_configuration->setEnvelopeEvents([ 'Completed' ]);
$connect_api->createConfiguration($account_id, $connect_custom_configuration);
Python
# You need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.host = base_path
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
connect_api = ConnectApi(api_client)
connect_event_data = ConnectEventData()
connect_event_data.version = 'restv2.1'
connect_custom_configuration = ConnectCustomConfiguration()
connect_custom_configuration.name = 'my custom connect configuration'
connect_custom_Configuration.configuration_type = 'custom'
connect_custom_configuration.all_users = 'true'
connect_custom_configuration.include_hmac = 'false'
connect_custom_configuration.enable_log = 'true'
# The URL you publish events to (your webhook) must be TLS-secure (https protocol)
connect_custom_configuration.url_to_publish_to = 'https://www.example.com/cat/meow'
connect_custom_configuration.event_eata = connect_event_data
connect_custom_configuration.envelope_events = [ 'Completed' ]
connect_api.create_configuration(account_id, connect_custom_configuration)
Ruby
# You need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
connect_api = DocuSign_eSign::ConnectApi.new api_client
connect_event_data = DocuSign_eSign::ConnectEventData.new
connect_event_data.version = 'restv2.1'
connect_custom_configuration = DocuSign_eSign::ConnectCustomConfiguration.new
connect_custom_configuration.name = 'my custom connect configuration'
connect_custom_Configuration.configuration_type = 'custom'
connect_custom_configuration.all_users = 'true'
connect_custom_configuration.include_hmac = 'false'
connect_custom_configuration.enable_log = 'true'
# The URL you publish events to (your webhook) must be TLS-secure (https protocol)
connect_custom_configuration.url_to_publish_to = 'https://www.example.com/cat/meow'
connect_custom_configuration.event_eata = connect_event_data
connect_custom_configuration.envelope_events = [ 'Completed' ]
connect_api.create_configuration(account_id, connect_custom_configuration)
Additional resources
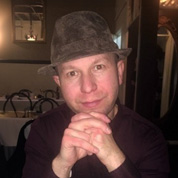
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
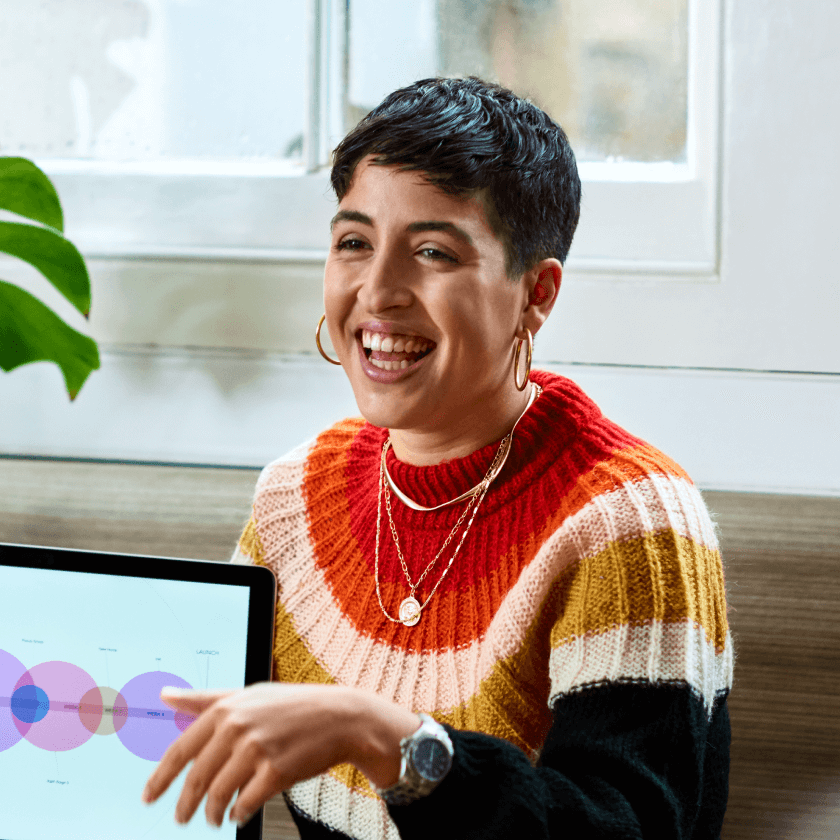