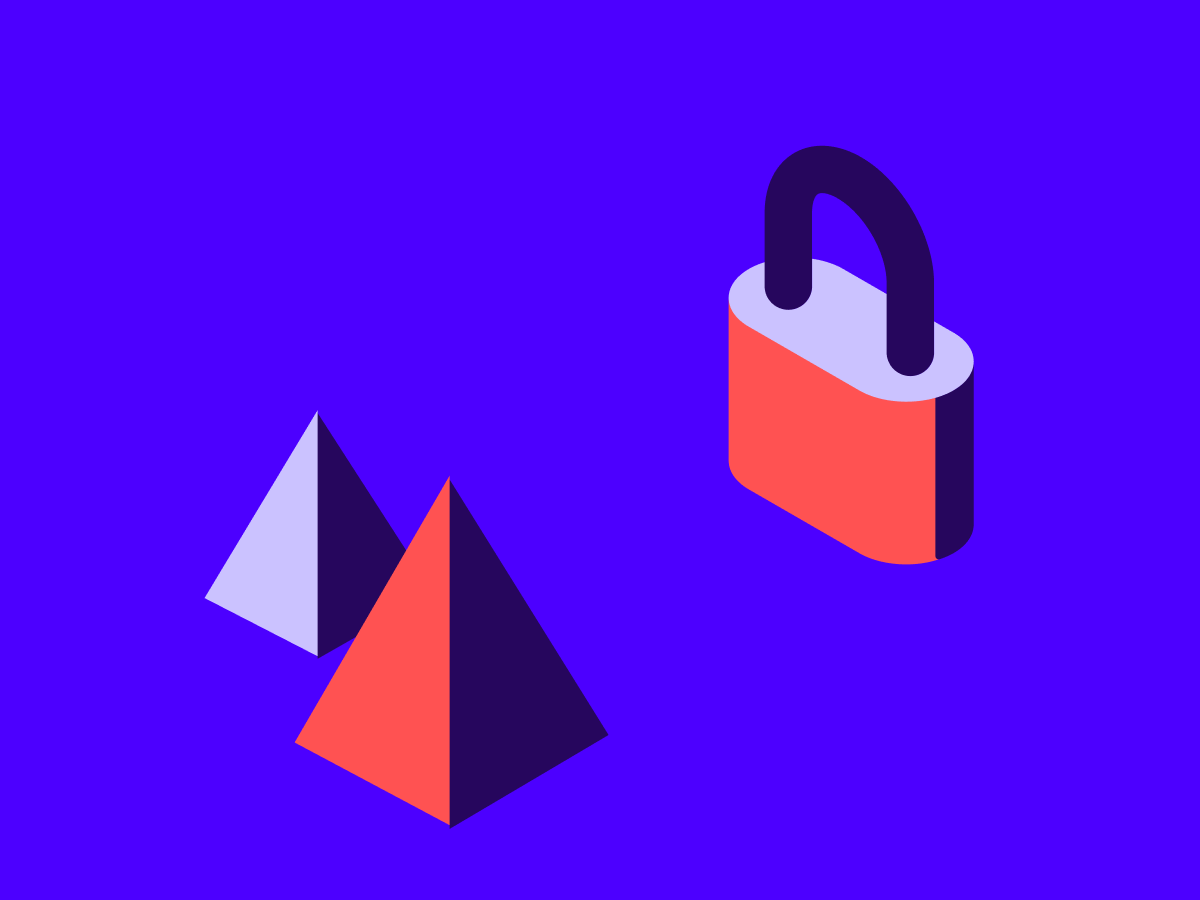
API Bytes: Updating your brand logo on envelopes
Get a byte of the API! This time, learn how to update your brand logo to customize the envelopes you send.
Table of contents
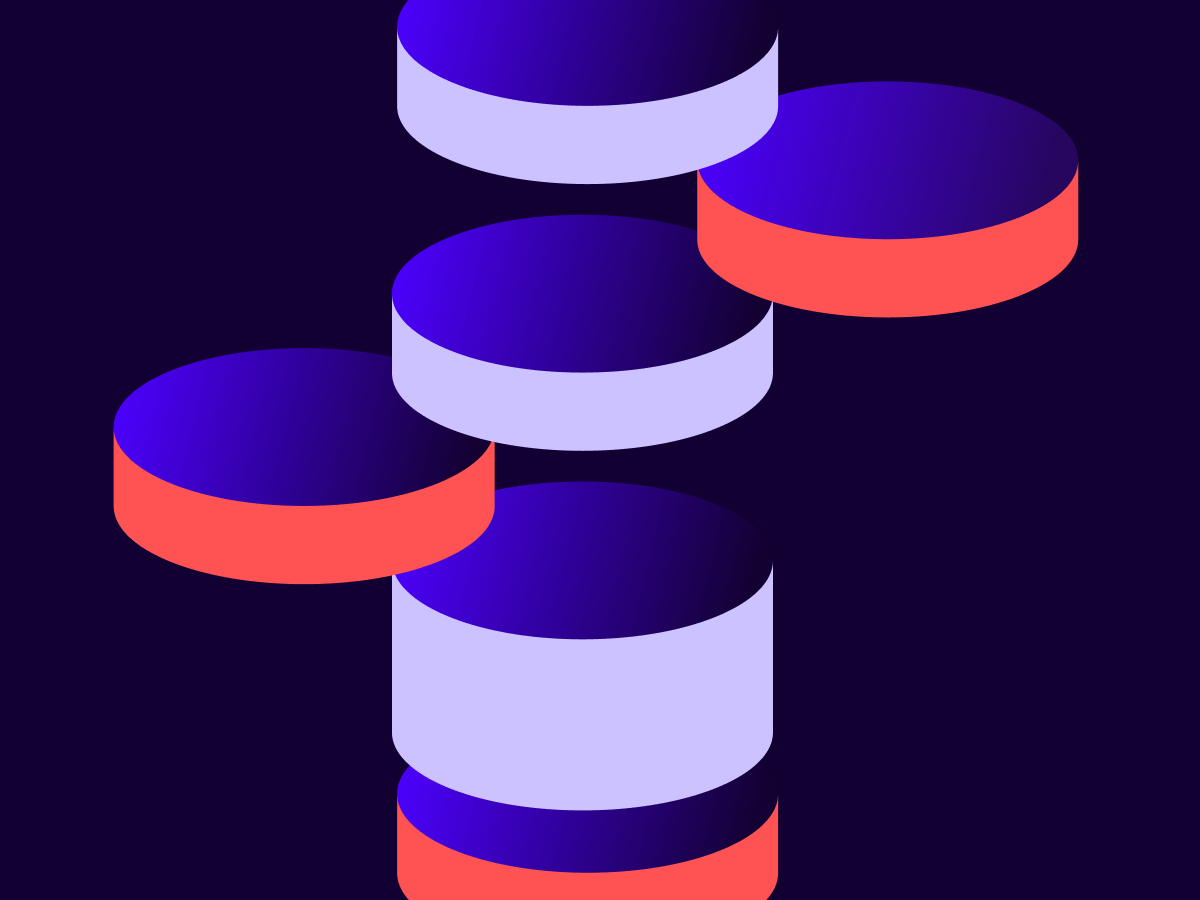
I’m introducing a new segment, “API Bytes: Byte-sized API concepts!” These may or may not contain occasional foodie themes. To kick off this byte-sized morsel of goodness, I’m going to discuss how to update your brand logo programmatically for your envelopes.
Background
Why would anyone want to update their brand logo programmatically? There are many possibilities:
Your organization acts as a holding company for subsidiary businesses.
Your company is an authorized vendor that does work on behalf of many different clients.
You have a highly seasonal business where you need to be able to easily and quickly convert the branding for your employees.
These and many other different cases can benefit from updating your branding logos for envelopes. Let’s get started!
Confirm your logo assets meet requirements
You must meet these requirements to update your custom branding logo assets:
You can only upload/change one logo per API request.
You must set an image type of:
primary (prominent, found in the upper left corner of the admin menu)
secondary (subtle, found in the upper right corner of the admin menu)
email (used at the top of the heading of branded envelopes)
Your image must fit within the following restrictions:
Maximum file size: 300 KB
Recommended dimensions: for web images (primary and secondary), 296 x 76 pixels (larger images will be resized); for email images, 150 pixels wide, with a maximum height of 150 pixels (images will not be resized)
Supported formats: JPG, GIF, PNG
Note: Changes may take up to one hour to display in all places.
Update your images via the eSignature API
With your image type chosen, upload the logo using a PUT request. If you are using one of our SDKs, instead of sending a PUT request, use the SDK's corresponding helper method to update a brand image instead. In the examples below, I'm updating the primary branding logo image located in the upper left corner of the dashboard. You can use these examples to update the secondary image or the email image as well.
Bash
# Update Logo
# Step 1: Obtain your OAuth token
# Note: Substitute these values with your own
oAuthAccessToken="eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw"
# Set up variables for full code example
# Note: Substitute these values with your own
APIAccountId="2255f473-xxxx-xxxx-xxxx-c07c9abc6d76"
BrandId="95461dce-xxxx-xxxx-xxxx-68acae2ab5c0"
ImgLocation=".\Desktop\logo.png"
# Check that we're in a bash shell
if [[ $SHELL != *"bash"* ]]; then
echo "PROBLEM: Run these scripts from within the bash shell."
fi
base_path="https://demo.docusign.net/restapi"
#Step 2: Construct your API headers
declare -a Headers=('--header' "Authorization: Bearer ${oAuthAccessToken}" \
'--header' "Accept: application/json" \
'--header' "Content-Type: application/json" \
'--header' "Accept-Encoding:gzip,deflate,br" \
'--header' "Content-Type", "image/png" \
'--header' "Content-Disposition", "form-data;name='file';filename='logo.png'")
# Step 3: a) Call the eSignature API
# b) Display the JSON response
# Create a temporary file to store the response
response=$(mktemp /tmp/response-perm.XXXXXX)
Status=$(curl -w '%{http_code}' -i --request PUT https://demo.docusign.net/restapi/v2.1/accounts/${APIAccountId}/brands/${BrandId}/logos/email \
"${Headers[@]}" \
--data-binary @C:/Users/YourUserName/Desktop/logo.png \
--output ${response})
# If the Status code returned is greater than 201 (OK/Accepted), display an error message along with the API response
if [[ "$Status" -gt "201" ]] ; then
echo ""
echo "Failed to Update Logo Image"
echo ""
cat $response
exit 1
fi
# Remove the temporary files
rm "$request_data"
rm "$response"
C#
var basePath ="https://demo.docusign.net/restapi/v2.1/accounts/restapi";
var accessToken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw"
var APIAccountId ="2255f473-xxxx-xxxx-xxxx-c07c9abc6d76"
var BrandId = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0";
var path = "C:/Users/YourUserName/Desktop/logo.png";
var config = new Configuration(new ApiClient(basePath));
config.AddDefaultHeader("Authorization", "Bearer " + accessToken);
AccountsApi accountsApi = new AccountsApi(config);
var brands = accountsApi.ListBrands(APIAccountId);
byte[] imgBytes = File.ReadAllBytes(path);
accountsApi.UpdateBrandLogoByType(APIAccountId, BrandId, "email", imgBytes)
Java
// Update Logo
String oAuthAccessToken="eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw";
String APIAccountId="2255f473-xxxx-xxxx-xxxx-c07c9abc6d76";
String basePath="https://demo.docusign.net/restapi";
String brandId="95461dce-xxxx-xxxx-xxxx-68acae2ab5c0";
Path path = Paths.get("C:/Users/YourUserName/Desktop/logo.png");
byte[] logoFileBytes = Files.readAllBytes(path);
AccountsApi accountsApi = createAccountsApi(basePath, oAuthAccessToken);
accountsApi.updateBrandLogoByType(APIAccountId, brandId, "email", logoFileBytes);
Node.js
// Update Logo
let oAuthAccessToken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw";
let APIAccountId = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76";
let basePath = "https://demo.docusign.net/restapi";
let brandId = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0";
let logoFileBytes = fs.readFileSync('C:/Users/YourUserName/Desktop/logo.png');
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + oAuthAccessToken);
let accountsApi = new docusign.AccountsApi(dsApiClient);
accountsApi.updateBrandLogoByType(logoFileBytes, APIAccountId, brandId, "email")
PHP
// Update Logo
$oAuthAccessToken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw";
$APIAccountId = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76";
$basePath = "https://demo.docusign.net/restapi";
$brandId = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0";
$logoFileBytes = file_get_contents('C:/Users/YourUserName/Desktop/logo.png');
$config = new Configuration();
$config->setHost($basePath);
$config->addDefaultHeader('Authorization', 'Bearer ' . $oAuthAccessToken);
$apiClient = new ApiClient($config);
$accountsApi = new AccountsApi($apiClient);
$accountsApi->updateBrandLogoByType($APIAccountId, $brandId, "email", $logoFileBytes);
PowerShell
# Upload Brand Logo
# Step 1: Obtain your OAuth token
# Note: These values are not valid, but are shown for example purposes only!
$oAuthAccessToken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw"
# Set up variables for full code example
# Note: These values are not valid, but are shown for example purposes only!
$APIAccountId = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76"
$BrandId = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0"
$ImgLocation = "C:/Users/YourUserName/Desktop/logo.png'";
#Step 2: Construct your API headers
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.add("Authorization","Bearer $oAuthAccessToken")
$headers.Add("Accept-Encoding", "gzip, deflate, br")
$headers.add("Content-Type", "image/png")
$headers.add("Content-Disposition", "form-data;name='file';filename='logo.png'")
# Step 3: a) Call the eSignature API
# b) Display the JSON response
$uri = "https://demo.docusign.net/restapi/v2.1/accounts/$APIAccountId/brands/$BrandId/logos/email"
try{
write-host "Response:"
$response = Invoke-WebRequest -headers $headers -Uri $uri -Method PUT -InFile $ImgLocation
write-host "Success!" $response.StatusCode "Status code"
}
catch{
write-host "Unable to update brand logo."
# On failure, display a notification, X-Docusign-TraceToken, error message, and the command that triggered the error
foreach($header in $_.Exception.Response.Headers){
if($header -eq "X-Docusign-TraceToken"){ write-host "TraceToken : " $_.Exception.Response}
$int++
}
write-host "Error : "$_.ErrorDetails.Message
write-host "Command : "$_.InvocationInfo.Line
}
Python
# Update Logo
# Note: Substitute these values with your own
access_token = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw"
base_path = "https://demo.docusign.net/restapi"
api_account_id = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76"
brand_id = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0"
with open("C:/Users/YourUserName/Desktop/logo.png", "rb") as image:
f = image.read()
logo_file_bytes = bytearray(f)
api_client = create_api_client(base_path=base_path, access_token=access_token)
account_api = AccountsApi(api_client)
account_api.update_brand_logo_by_type(api_account_id, brand_id, "email", logo_file_bytes)
Ruby
# Step 1: Obtain your OAuth token
# Note: Substitute these values with your own
oAuthAccessToken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw"
base_path="https://demo.docusign.net/restapi"
#Set up variables for full code example
# Note: Substitute these values with your own
API_account_id = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76"
brand_Id = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0"
image = File.binread "C:/Users/YourUserName/Desktop/logo.png"
logo_file_bytes = image.unpack('B*')
configuration = DocuSign_eSign::Configuration.new
configuration.host = args[:base_path]
api_client = DocuSign_eSign::ApiClient.new configuration
api_client.default_headers['Authorization'] = "Bearer #{base_path}"
accounts_api = DocuSign_eSign::AccountsApi.new api_client
accounts_api.update_brand_logo_by_type(API_account_id, brand_id, logo_type, logo_file_bytes)
Having custom logos is a simple feature to harness but well worth the effort. Tailoring your branding logos can improve the UI for users or help your organization scale.
In the next segment of API Bytes, I’ll go over how to update the branding color scheme through the eSignature API. In the meantime, feel free to begin this activity right away by setting up a developer demo account through the Docusign Developer Center. If you’re just getting acquainted with the Docusign eSignature API and would like to see custom branding in action, check out the Sailboat Loan scenario in our interactive LoanCo sample application.
Additional resources
Aaron Jackson-Wilde has been with Docusign since 2019 and specializes in API-related developer content. Aaron contributes to the Quickstart launchers, How-To articles, and SDK documentation, and helps troubleshoot occasional issues on GitHub or StackOverflow.
Discover what's new with Docusign IAM or start with eSignature for free
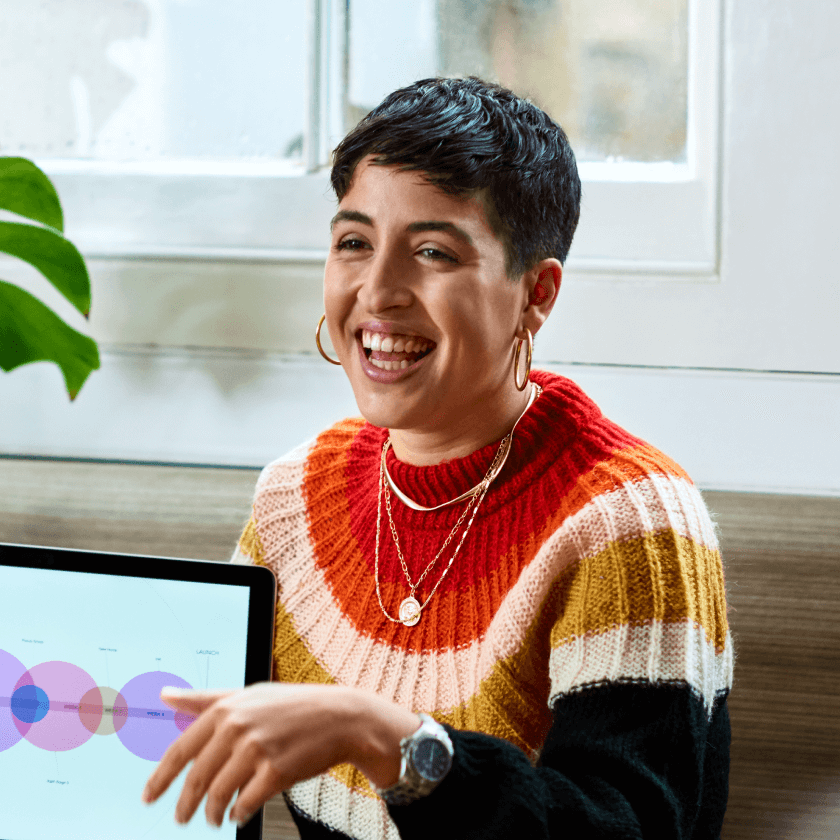