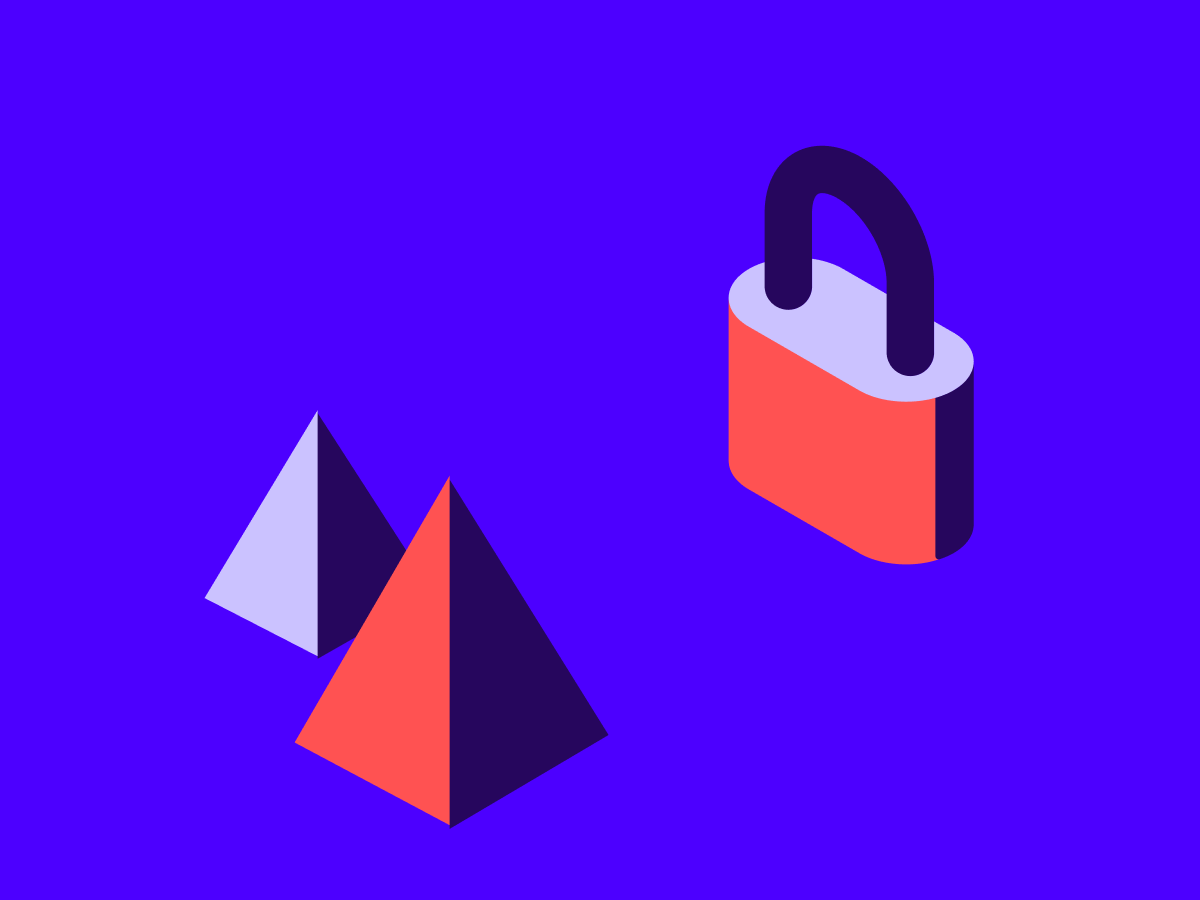
Debugging API calls
Tips and techniques for debugging Docusign API calls. API request logging and more.
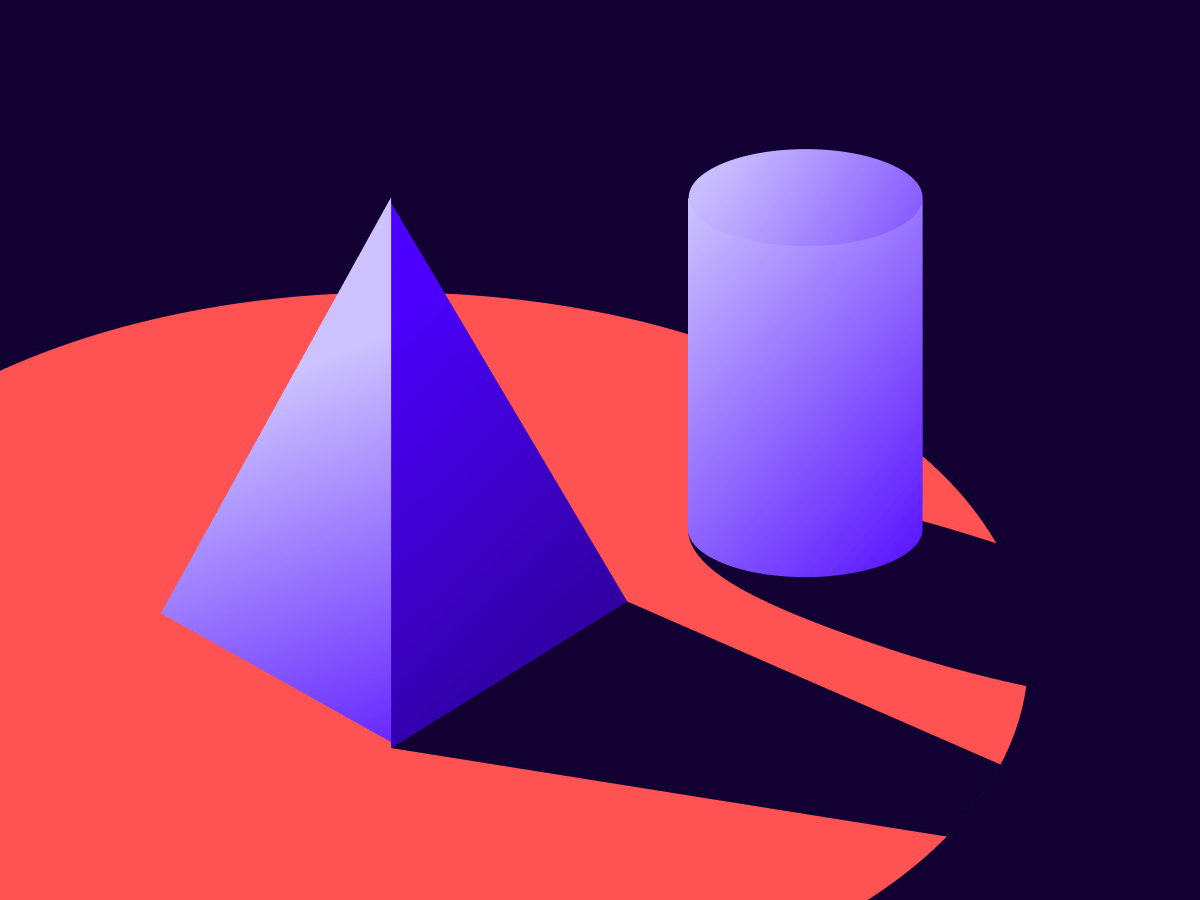
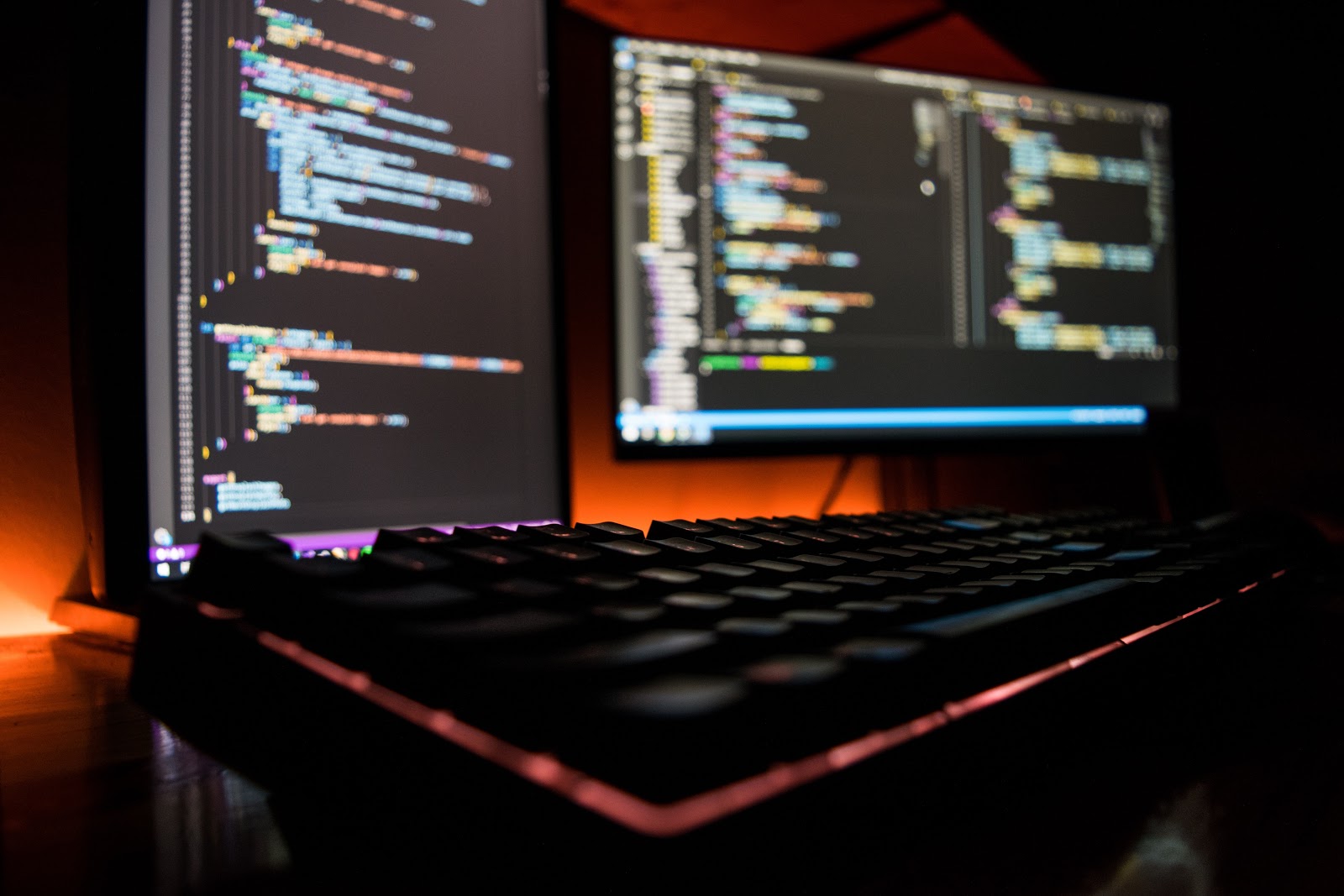
By Larry Kluger and Drew Martin
Not only is debugging and validating a software application hard, it typically takes 35-50 percent of a developer’s work day. One approach to the problem is the idea of writing code correctly in the first place, resulting in no need for debugging. (Doesn’t that sound grand!) The other is to develop skills and tools to enable a faster, less painful debugging experience.
In our work with corporate developers working on integrating Docusign into their applications, many need to debug their use of the Docusign eSignature REST API, especially if it’s the first time they’ve used a networked API (a cloud API).
Hartley Brody has written a good post on general debugging techniques. For debugging a networked API, we focus on a couple of debugging tactics:
Start with a known good code example
A very useful debugging technique is to start with “known good” software, assure yourself that it works as expected, and then update it to fit your application’s specific requirements. Docusign provides code examples for its APIs in a variety of languages for this purpose. A pro tip is to download the complete code example rather than copying the code example’s snippets from the Developer Center.
Since the code examples are open source, you can easily change them to meet the exact needs of your application.
Understand and debug the key network API steps
Making an API request to Docusign always follows the same sequence of steps. When debugging, first narrow your focus to the step that has a problem.
Step 1: Authentication
In this step you obtain a current access token from the Docusign authentication service. Depending on the OAuth flow your application uses, an access token lasts either one or eight hours. Your software needs to initially obtain an access token, and then check its age before each API call. If the access token has expired or is about to expire, obtain a new access token before attempting your API call.
How you obtain a new access token again depends on the OAuth flow your application uses. If you have a problem with authentication, you’ll receive an authentication-related error from Docusign.
Step 2: Create the request object
The request object is specific to the API method you’re using and your use case. For example, the Envelopes:create API method’s request object has an enormous number of optional attributes and values that can be used to create any type of Docusign envelope.
Creating a request that does what you want can be difficult. A good debugging technique is to use Docusign’s API request logging to see how the Docusign web tool creates an envelope with the features you want, then copy what the web tool does.
API request logging is also valuable for confirming exactly what your application is sending to Docusign. If your request is accepted by Docusign without an error but does not produce the envelope effects you want, try creating the envelope with the web tool and use the API log as we suggest above.
If you receive an API error message, be sure to check the body of the API response as well. It often includes additional error information. If your application doesn’t show the response body, use the API request logger to see it.
Step 3: Send the API request
Sending the API request, either directly or via an SDK, can also be a source of problems. Which base URI are you using? Is it the right base URI for the account ID? This is a frequent source of problems when moving an application from development to the Docusign production systems.
Use the /oauth/userinfo endpoint to determine the account ID and associated base URI. If your application must use a specific account ID, then use /oauth/userinfo to confirm that the authenticated user has access to the specific account ID. Remember to cache /oauth/userinfo information! For Authorization Code grant authentication, call the endpoint once when the user authenticates. For the JWT grant, don’t call it more than once a day.
Your application should also smoothly handle basic networking issues. If the computer is disconnected from the internet when your application tries to send a request to Docusign, does your application go up in flames or provide an appropriate error message? Suppose the network is available, but not DNS?
Step 4: Handle the response
Parsing the API response from JSON into your application’s native objects is usually a smooth process. But beware some common pitfalls:
Some JSON parsers have a relaxed mode whereby unexpected source attributes will be parsed automatically and a strict mode that will raise an error if an unexpected attribute is detected. Always used the relaxed mode. Docusign considers the addition of new attributes to an API response to be a non-breaking change. Don’t let a new attribute break your software! If you use a Docusign SDK, the SDK will take care of this step for you.
Remember that Docusign uses strings almost exclusively in its eSignature API responses. Some newer Docusign APIs use other scalar types too. If a numeric or date response is returned as a string, you’ll need to parse it accordingly. See the documentation for the API method you’re using.
If there’s an error in your application’s response handling, API logging is once again useful for seeing exactly what was sent to your application from Docusign.
Still stuck?
Ask for help via StackOverflow (use the docusignapi tag). Write a good question! As appropriate to your question, include your code, the API log, and any other relevant information. Don’t include private information, your integration key, etc.
Members of the developer community and the Docusign staff watch the StackOverflow question feed closely and usually respond to questions within a day. If you’re a Docusign support customer, you can also open an API support case. Pro tip: log in to the Docusign support portal and submit your case there.
Really stuck?
The great news is that there’s now an enormous community of Docusign developers waiting to help you. There are individual consultants, system integrators of all sizes, and Docusign Professional Services. The Docusign solution showcase includes listings for system integrators. Your company’s favorite local software consultants may also be able to assist you.
Good luck with your integration, and thank you for developing with Docusign!
______________________
Larry Kluger is the Lead Developer Advocate for Docusign. He has many years of tech industry experience as a software developer, product manager, and developer advocate. An award-winning speaker, he enjoys giving talks and meeting members of the developer community.
Drew Martin is a Developer Support Engineer for Docusign. If your debugging doesn't go so well and you reach out to Docusign Customer Support, there's a chance you'll end up working with him. He's also somewhat active in the DocuSignAPI tag on StackOverflow and enjoys a good margarita.
Additional developer resources
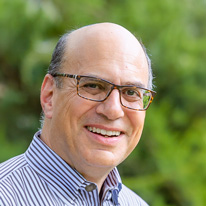
Larry Kluger has over 40(!) years of tech industry experience as a software developer, developer advocate, entrepreneur, and product manager. An award-winning speaker with a 48K StackOverflow reputation, he enjoys giving talks and helping the ISV and developer communities.
Twitter: @larrykluger
LinkedIn: https://www.linkedin.com/in/larrykluger/
Discover what's new with Docusign IAM or start with eSignature for free
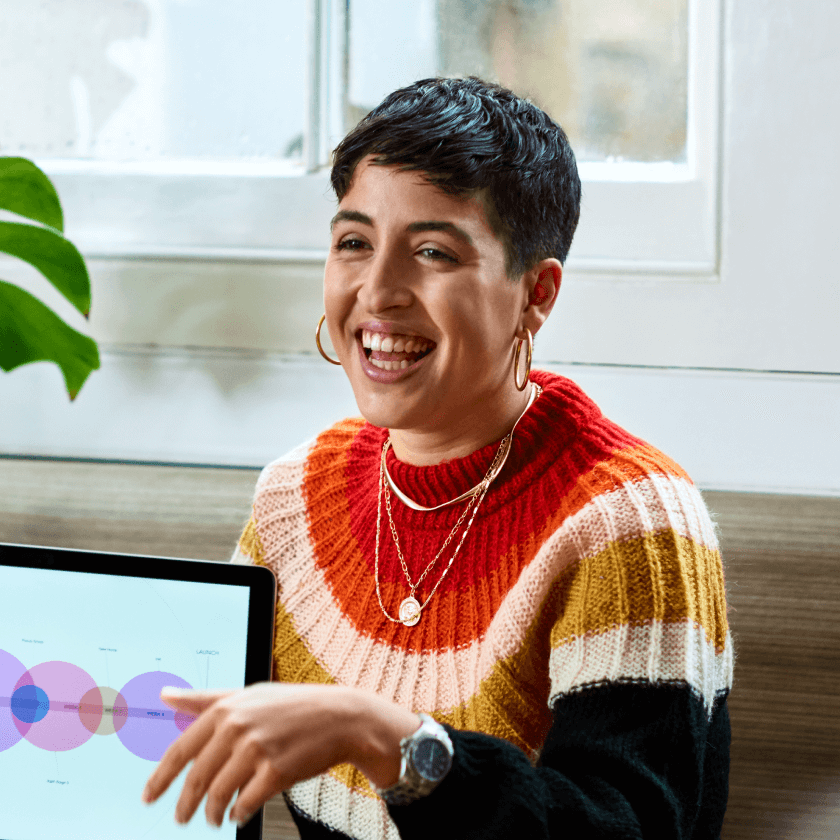