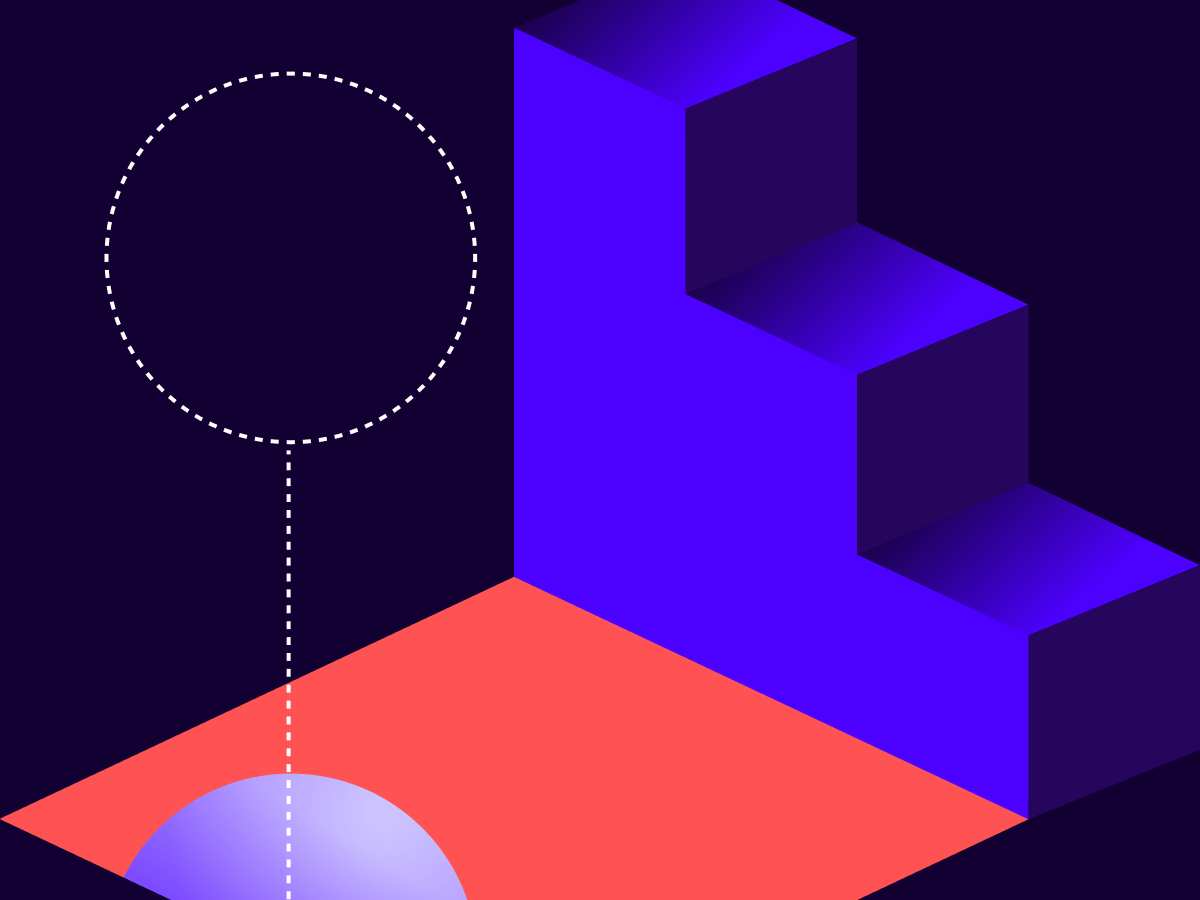
Common API Tasks🐈: Working with Favorite Templates
Find out how you can use the Docusign eSignature REST API to get and update favorite templates.
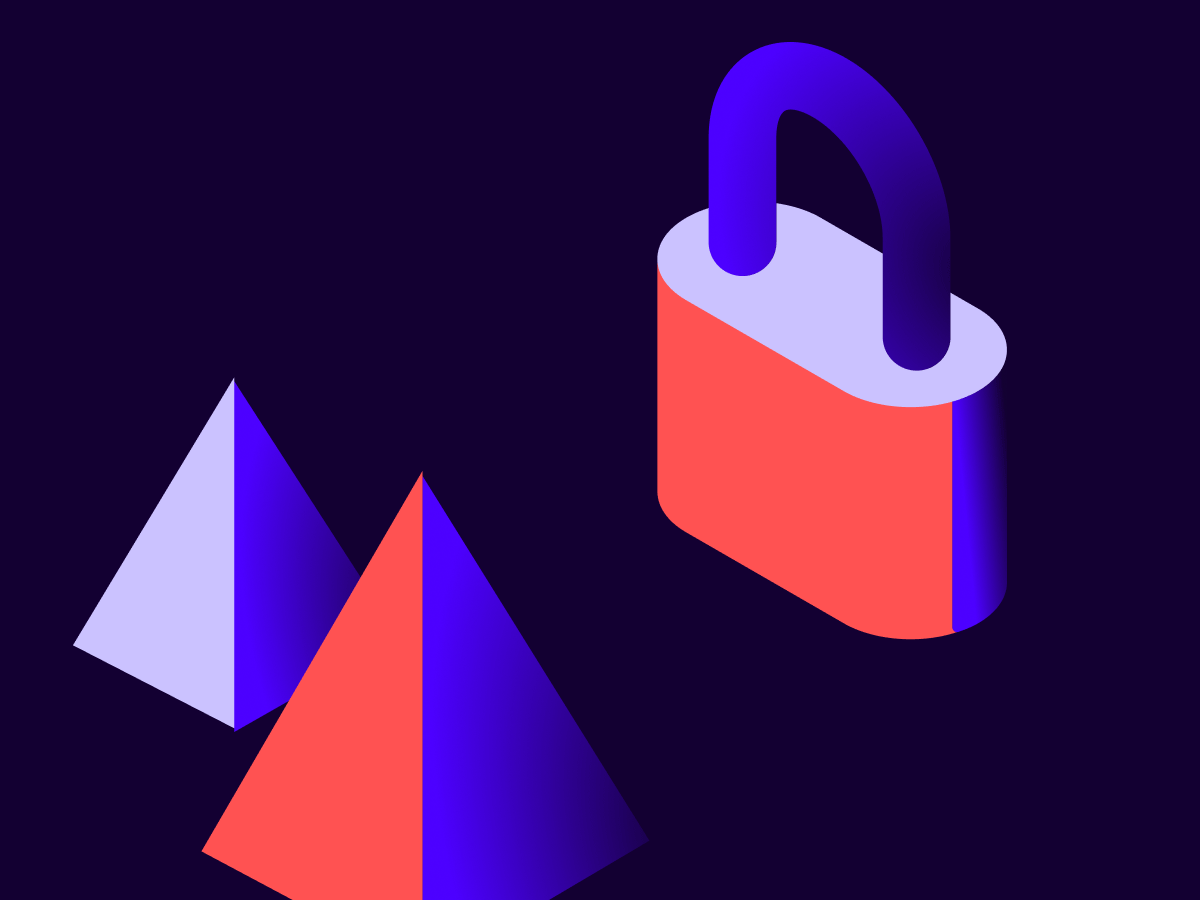
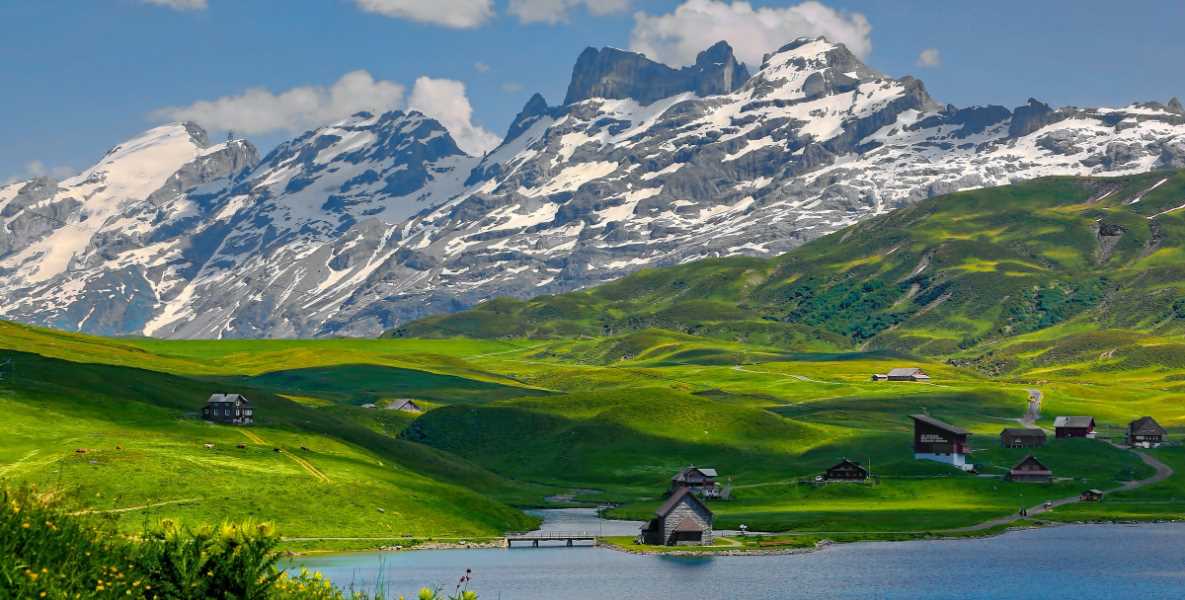
Welcome to the holiday edition of CAT (Common API Tasks)! This blog series explores various useful activities you can perform with the Docusign APIs. Since it’s the holiday season, and I really like the Julie Andrews song (“My Favorite Things”), I decided to use this blog post to talk about favorite templates. Some of you may have seen the little star icons left of the template names in the Docusign web app. There’s also a Favorites folder you can use. This is very useful if your Docusign account has many templates. Some are just your fav, while others are... not.
Almost everything you can do via the Docusign web app can also be accomplished programmatically via the Docusign eSignature REST API. This is where I can help. The following code snippets show how to do three things:
Get a list of all the favorite templates.
Add a template as your favorite (Note: you can add more than one at a time).
Remove a template from favorites. We call this to unfavorite the template.
To use these code examples, you’ll need to get the latest versions of the eSignature SDKs for the specific language you’re interested in.
C#
// You will need to obtain an access token using your chosen authentication flow
var apiClient = new ApiClient(basePath);
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
var accountsApi = new AccountsApi(apiClient);
FavoriteTemplatesInfo favs = accountsApi.GetFavoriteTemplates(accountId);
// Go over the list of templates and extract the templateId of each
if (favs.FavoriteTemplates != null) // If there are no favorites, we get null, not an empty list
foreach (var fav in favs.FavoriteTemplates)
{
Console.WriteLine(fav.TemplateId);
}
favs.FavoriteTemplates.Clear();
// Nnow add a new favorite template based on the templateId
favs.FavoriteTemplates.Add(new FavoriteTemplatesContentItem { TemplateId = "19e50551-xxxx-xxxx-xxxx-aab75c994226" });
accountsApi.UpdateFavoriteTemplate(accountId, favs);
// Unfavorite this template, again based on the templateId
accountsApi.UnFavoriteTemplate(accountId, favs);
Java
// You will need to obtain an access token using your chosen authentication flow
ApiClient apiClient = new ApiClient(basePath);
apiClient.addDefaultHeader("Authorization", "Bearer " + accessToken);
AccountsApi accountsApi = new AccountsApi(apiClient);
FavoriteTemplatesInfo favs = accountsApi.getFavoriteTemplates(accountId);
// Go over the list of templates and extract the templateId of each
if (favs.getFavoriteTemplates() != null) // If there are no favorites, we get null, not an empty list
for (int i = 0; i
<h2>Node.js</h2>
<code class="language-javascript" data-uuid="kg14qdYX">// You will need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let accountsApi = new docusign.AccountsApi(dsApiClient);
let favs = accountsApi.getFavoriteTemplates(accountId);
// Go over the list of templates and extract the templateId of each
if (favs.favoriteTemplates != undefined) // If there are no favorites, we get null, not an empty list
for (int i = 0; i
<h2>PHP</h2>
<code class="language-php" data-uuid="vLC0oi2r"># You will need to obtain an access token using your chosen authentication flow
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$accountss_api = new \Docusign\Api\AccountsApi($config);
$favs = $accounts_api->getFavoriteTemplates($account_id);
# Go over the list of templates and extract the templateId of each
if ($favs->getFavoriteTemplates() != null) // If there are no favorites, we get null, not an empty list
for ($i = 0; $i getFavoriteTemplates()[$i]->getTemplateId());
}
$favs->setFavoriteTemplates([]);
# Now add a new favorite template based on the templateId
$new_fav = new \Docusign\eSign\Model\FavoriteTemplatesContentItem();
$new_fav->setTemplateId('19e50551-xxxx-xxxx-xxxx-aab75c994226');
array_push($favs->getFavoriteTemplates(), $new_fav);
$accounts_api->updateFavoriteTemplate($account_id, $favs);
# Unfavorite this template, again based on the templateId
$accounts_api->unFavoriteTemplate($account_id, $favs);
</code>
<h2>Python</h2>
<code class="language-python" data-uuid="csC4HFMt"># You will need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
accounts_api = AccountsApi(config)
favs = accounts_api.get_favorite_templates(account_id)
# Go over the list of templates and extract the templateId of each
if favs.favorite_templates != None: # If there are no favorites, we get None, not an empty list
for fav in favs.favorite_Templates:
print(favs.favorite_templates[i].template_id)
favs.favorite_templates = []
# Now add a new favorite template based on the templateId
new_fav = FavoriteTemplatesContentItem()
new_fav.template_id = '19e50551-xxxx-xxxx-xxxx-aab75c994226'
favs.favorite_templates.insert(new_fav)
accounts_api.update_favorite_template(account_id, favs)
# Unfavorite this template, again based on the templateId
accounts_api.un_favorite_template(account_id, favs)
</code>
<h2>Ruby</h2>
<code class="language-ruby" data-uuid="xKZjmdVb"># You will need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
account_api = DocuSign_eSign::AccountsApi.new api_client
favs = accounts_api.get_favorite_templates(account_id)
# Go over the list of templates and extract the templateId of each
if favs.favorite_templates != nil # If there are no favorites, we get nil, not an empty list
for fav in favs.favorite_Templates
puts(favs.favorite_templates[i].template_id)
favs.favorite_templates = []
# Now add a new favorite template based on the templateId
new_fav = DocuSign_eSign::FavoriteTemplatesContentItem.new
new_fav.template_id = '19e50551-xxxx-xxxx-xxxx-aab75c994226'
favs.favorite_templates.push(new_fav)
accounts_api.update_favorite_template(account_id, favs)
# Unfavorite this template, again based on the templateId
accounts_api.un_favorite_template(account_id, favs)
</code>
<p>I hope you found this useful. As usual, if you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to <a href="mailto:inbar.gazit@docusign.com">email me</a>. Until next time...</p>
<h2>Additional resources</h2>
<ul><li><a href="https://developers.docusign.com">Docusign Developer Center</a></li>
<li><a href="https://twitter.com/docusignapi">@DocuSignAPI on Twitter</a></li>
<li><a href="https://www.linkedin.com/showcase/docusign-for-developers/">Docusign for Developers on LinkedIn</a></li>
<li><a href="https://www.youtube.com/playlist?list=PLXpRTgmbu4opxdx2IThm4pDYS8tIKEb0w">Docusign for Developers on YouTube</a></li>
<li><a href="https://developers.docusign.com/#newsletter-signup">Docusign Developer Newsletter</a></li>
</ul></code>
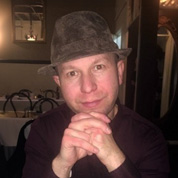
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
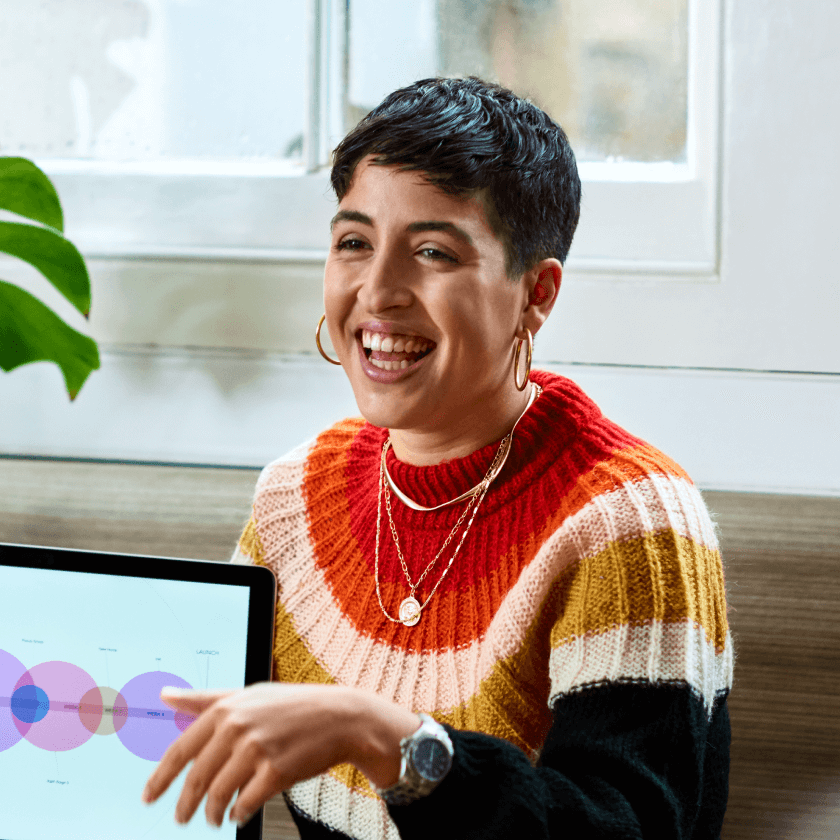