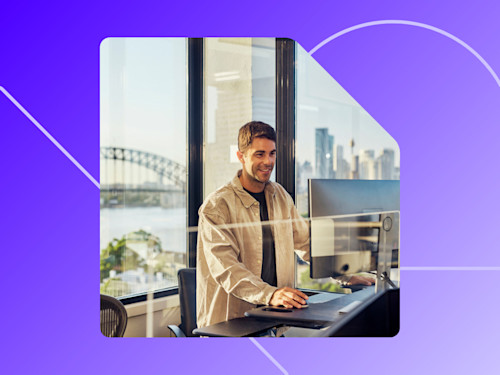
Common API Tasksš: Change the display language of embedded signing
The eSignature API lets you specify the language in which your embedded signing session is presented. Inbar shows you how to change it in your API call.
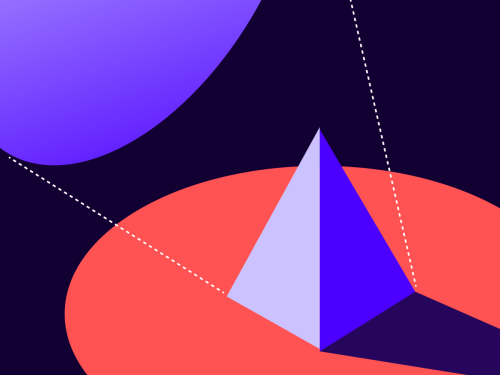
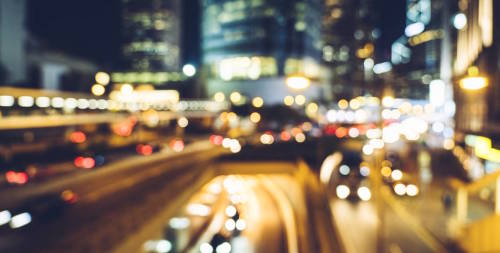
Welcome to a magnifique new edition of the CATš (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
Embedded signing is perhaps one of the most popular ways developers use the Docusign APIs. Since Docusign eSignature is the bread and butter of what we do, itās no surprise that developers would want to implement the signing process inside their own applications. That is why, for example, we chose to make embedded signing the default and automatic code example that our code example launchers will immediately execute when you download them using our Quickstart.Ā
A lot has been written about embedded signing, including a brand new Deep Dive postĀ just published by Byungjae Chung. However, what Iām going to show you is how you can have your application dynamically change the display language (or locale) so that you can have your users sign in a different language.
Docusign supports 44 signing languages (at the time of this writing) and you can find the full list at docusign.com in The Global Standard for eSignature. Each of these 44 languages comes with an ISO code of either two or five digits. The list of languages Docusign supports is updated very infrequently, so Iāll post it in here with the list of the codes. However, thereās another way to get this list: by making an API call to the Accounts:listSupportedLanguages endpoint. The endpoint returns a hardcoded JSON that looks like this:
{
"languages": [
{
"name": "Arabic (ar)",
"value": "ar"
},
{
"name": "Bulgarian (bg)",
"value": "bg"
},
{
"name": "Czech (cs)",
"value": "cs"
},
{
"name": "Chinese Simplified (zh-CN)",
"value": "zh_CN"
},
{
"name": "Chinese Traditional (zh-TW)",
"value": "zh_TW"
},
{
"name": "Croatian (hr)",
"value": "hr"
},
{
"name": "Danish (da)",
"value": "da"
},
{
"name": "Dutch (nl)",
"value": "nl"
},
{
"name": "English US (en)",
"value": "en"
},
{
"name": "English UK (en-GB)",
"value": "en_GB"
},
{
"name": "Estonian (et)",
"value": "et"
},
{
"name": "Farsi (fa)",
"value": "fa"
},
{
"name": "Finnish (fi)",
"value": "fi"
},
{
"name": "French (fr)",
"value": "fr"
},
{
"name": "French Canada (fr-CA)",
"value": "fr_CA"
},
{
"name": "German (de)",
"value": "de"
},
{
"name": "Greek (el)",
"value": "el"
},
{
"name": "Hebrew (he)",
"value": "he"
},
{
"name": "Hindi (hi)",
"value": "hi"
},
{
"name": "Hungarian (hu)",
"value": "hu"
},
{
"name": "Bahasa Indonesia (id)",
"value": "id"
},
{
"name": "Italian (it)",
"value": "it"
},
{
"name": "Japanese (ja)",
"value": "ja"
},
{
"name": "Korean (ko)",
"value": "ko"
},
{
"name": "Latvian (lv)",
"value": "lv"
},
{
"name": "Lithuanian (lt)",
"value": "lt"
},
{
"name": "Bahasa Malay (ms)",
"value": "ms"
},
{
"name": "Norwegian (no)",
"value": "no"
},
{
"name": "Polish (pl)",
"value": "pl"
},
{
"name": "Portuguese (pt)",
"value": "pt"
},
{
"name": "Portuguese Brasil (pt-BR)",
"value": "pt_BR"
},
{
"name": "Romanian (ro)",
"value": "ro"
},
{
"name": "Russian (ru)",
"value": "ru"
},
{
"name": "Serbian (sr)",
"value": "sr"
},
{
"name": "Slovak (sk)",
"value": "sk"
},
{
"name": "Slovenian (sl)",
"value": "sl"
},
{
"name": "Spanish (es)",
"value": "es"
},
{
"name": "Spanish Latin America (es-MX)",
"value": "es_MX"
},
{
"name": "Swedish (sv)",
"value": "sv"
},
{
"name": "Thai (th)",
"value": "th"
},
{
"name": "Turkish (tr)",
"value": "tr"
},
{
"name": "Ukrainian (uk)",
"value": "uk"
},
{
"name": "Vietnamese (vi)",
"value": "vi"
},
{
"name": "Armenian (hy)",
"value": "hy"
}
]
}
What you need is the value (which is also in brackets with the name) for the language you want to use.
Now letās talk about the code. How do you actually do this in your integration? First, you have to do the same thing you otherwise would if you wanted to use embedded signing. You have to have an envelope, and for any recipient that you want to embed, you need to have a clientUserId
set for them on the envelope. Then, you make another API call to the EnvelopeViews:createRecipient endpoint, providing the aforementioned clientUserId
, so that you can get back the URL to use for embedded signing. (Remember: This URL expires in 5 minutes.)
Once you get the URL for embedded signing and you also know which locale code to use (from the list of languages above), itās just a matter of adding ?locale=vi
(as an example) to the URL before using it in your application.
The code snippets I provide below make the call to create a recipient view, then append the locale to it. The resulting URL can be used to either redirect the browser or be the target of an iframe.
C#
DocuSignClient docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(docuSignClient);
RecipientViewRequest viewRequest = new RecipientViewRequest();
viewRequest.ClientUserId = "1234";
viewRequest.UserName = "Inbar Gazit";
viewRequest.Email = "inbar.gazit@docusign.com";
viewRequest.AuthenticationMethod = "none";
ViewUrl results = envelopesApi.CreateRecipientView(accountId, envelopeId, viewRequest);
string redirectUrl = results.Url + "&locale=hy"; // Pick your locale
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
RecipientViewRequest viewRequest = new RecipientViewRequest();
viewRequest.setClientUserId("1234");
viewRequest.setUserName("Inbar Gazit");
viewRequest.setEmail("inbar.gazit@docusign.com");
viewRequest.setAuthenticationMethod("none");
ViewUrl results = envelopesApi.CreateRecipientView(accountId, envelopeId, viewRequest);
String redirectUrl = results.getUrl() + "&locale=hy"; // Pick your locale
Node.js
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
let viewRequest = new docusign.RecipientViewRequest();
viewRequest.clientUserId = '1234';
viewRequest.userName = 'Inbar Gazit';
viewRequest.email = 'inbar.gazit@docusign.com';
viewRequest.authenticationMethod = 'none';
let results = envelopesApi.createRecipientView(accountId, envelopeId, viewRequest);
let redirectUrl = results.Url + '&locale=hy'; // Pick your locale
PHP
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \Docusign\eSign\Api\EnvelopesApi($api_client);
$view_request = new \Docusign\eSign\Model\RecipientViewRequest();
$view_request->setClientUserId('1234');
$view_request->setUserName('Inbar Gazit');
$view_request->setEmail('inbar.gazit@docusign.com');
$view_request->setAuthenticationMethod('none');
$results = envelopes_api->CreateRecipientView($account_id, $envelope_id, $view_request);
$redirect_url = $results->getUrl() + '&locale=hy'; # Pick your locale
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
view_request = RecipientViewRequest()
view_request.client_user_id = '1234'
view_request.user_name = 'Inbar Gazit'
view_request.email = 'inbar.gazit@docusign.com'
view_request.authentication_method = 'none'
results = envelopes_api.create_recipient_view(account_id, envelope_id, view_request)
redirect_url = results.url + '&locale=hy' # Pick your locale
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
view_request = DocuSign_eSign::RecipientViewRequest.new
view_request.client_user_id = '1234'
view_request.user_name = 'Inbar Gazit'
view_request.email = 'inbar.gazit@docusign.com'
view_request.authentication_method = 'none'
results = envelopes_api.create_recipient_view(account_id, envelope_id, view_request)
redirect_url = results.url + '&locale=hy' # Pick your locale
Thatās all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
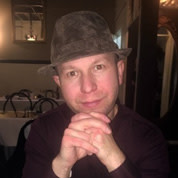
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
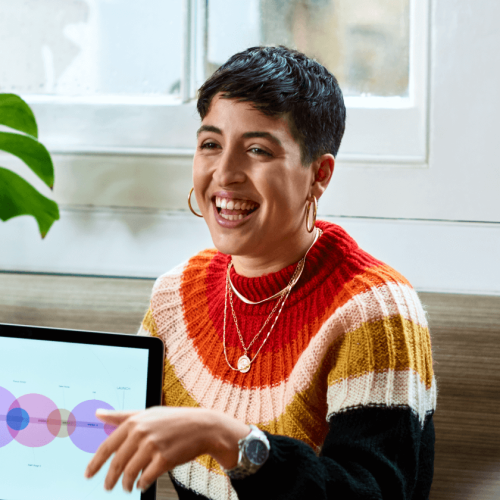