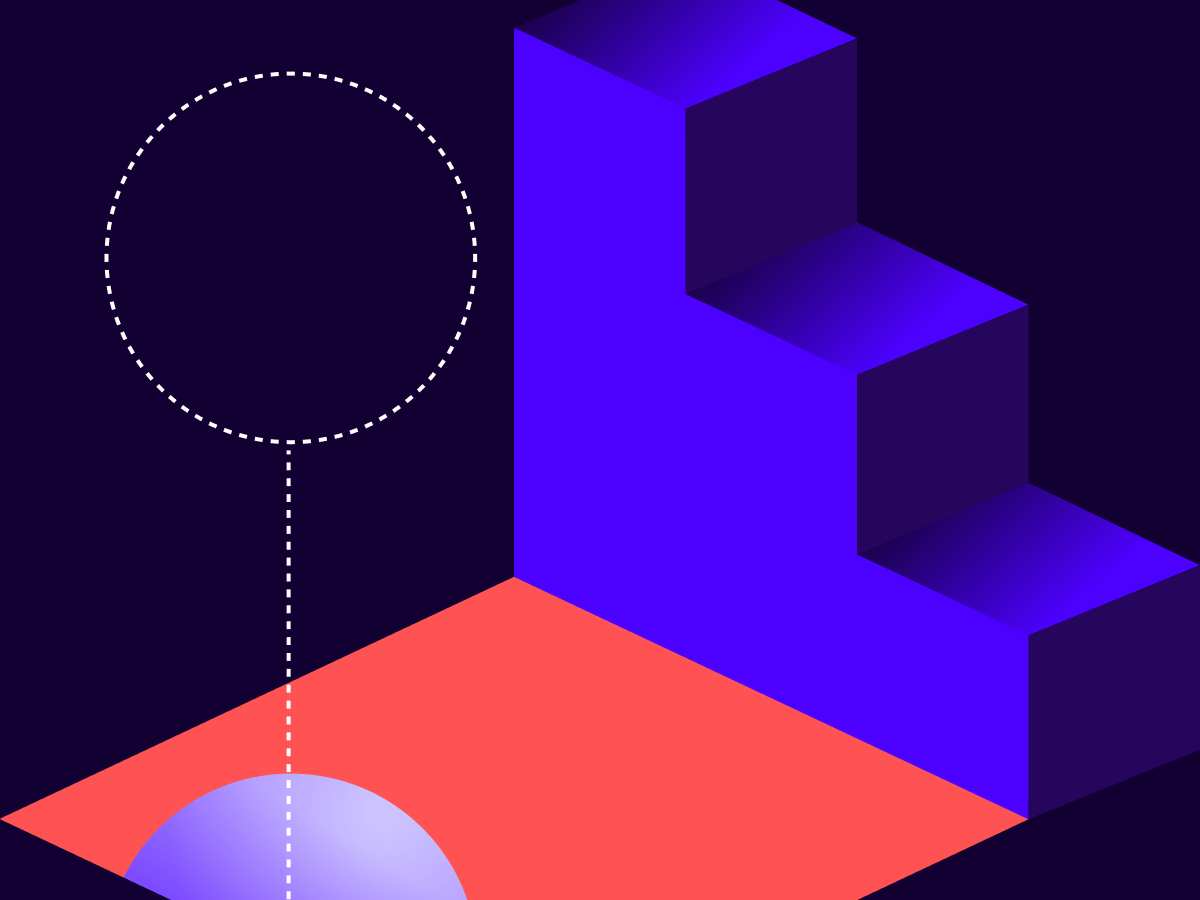
Common API Tasks🐈: Change email notifications programmatically
Inbar Gazit shows you how to use the eSignature API to change the email notification settings in your Docusign account.
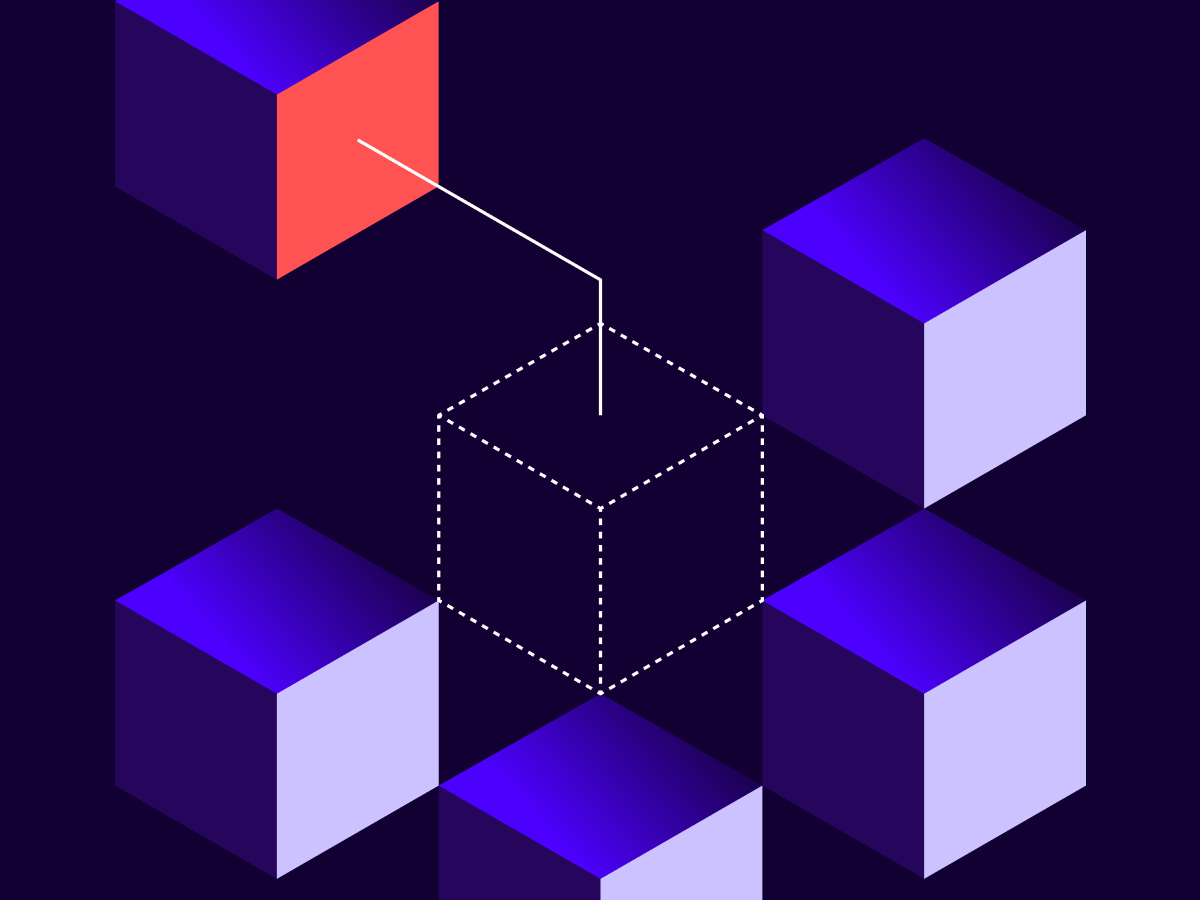
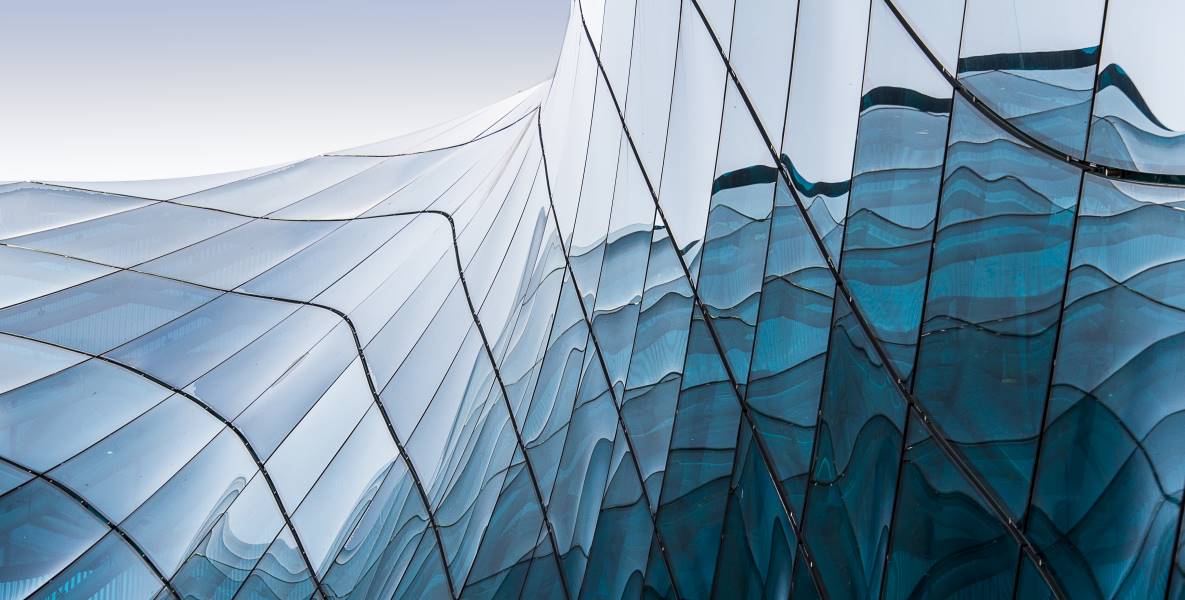
Welcome to an exceptional new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
In this edition I’m going to show you how to change your email notifications. As you may be aware, Docusign sends email notifications for various events: for example, when you are asked to sign an envelope or when an envelope is complete. You can control whether or not these emails are sent.
I’d like to start by saying that this is an often-confusing topic, and that there are multiple levers you can use to modify email notifications. In this post I’m going to focus only on a single mechanism to control email notifications for the given Docusign user, and ignore other means to control whether or not email notifications will be sent.
As a user you are able to decide if and when you will receive email notifications from Docusign. You can do this manually using the Docusign web app. You simply have to go to the My Preferences menu at the top right of the screen, and then select Notifications on the left-hand menu bar. You will then be able to pick and choose which email notifications you want to receive.
Take notice, that this option controls the notifications to your own email, for the specific Docusign user whom you are logged in as—meaning that if you send out envelopes for signatures by others, they will still be receiving notifications based on other settings (which I’m not going to cover in this article).
Of course, as you all know, the 🐈 blog series is all about writing some code to do things via the Docusign APIs. So, as I always do, I’m going to give you six code snippets to show you how to do this in our SDK languages. The code snippets below change the user’s email notifications settings such that the user no longer receives emails when envelopes are complete. You can, of course, do many other things based on your own needs. (Note that you have to specify the account ID and the user ID for this code to work properly).
And without further ado, here is the code:
C#
var apiClient = new ApiClient(basePath);
// You will need to obtain an access token using your chosen authentication method
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
UsersApi usersApi = new UsersApi(apiClient);
UserSettingsInformation userSettingsInformation = new UserSettingsInformation();
userSettingsInformation.SenderEmailNotifications = new SenderEmailNotifications();
userSettingsInformation.SenderEmailNotifications.EnvelopeComplete = "false";
usersApi.UpdateSettings(accountId, userId, userSettingsInformation);
Java
// You will need to obtain an access token using your chosen authentication flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
UsersApi usersApi = new UsersApi(config);
UserSettingsInformation userSettingsInformation = new UserSettingsInformation();
userSettingsInformation.setSenderEmailNotifications(new SenderEmailNotifications());
userSettingsInformation.SenderEmailNotifications.setEnvelopeComplete("false");
usersApi.UpdateSettings(accountId, userId, userSettingsInformation);
Node.js
// You will need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
UserApi usersApi = new docusign.UsersApi(apiClient);
let userSettingsInformation = docusign.UserSettingsInformation.constructFromObject({
senderEmailNotifications : {
envelopeComplete : 'false'
}});
usersApi.updateSettings(accountId, userId, userSettingsInformation);
PHP
# You will need to obtain an access token using your chosen authentication flow
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$users_api = new \Docusign\eSign\Api\UsersApi($api_client);
$user_settings_information = new \Docusign\eSign\Model\UserSettingsInformation();
$user_email_notifications = new \Docusign\eSign\Model\SenderEmailNotifications()
$user_email_notifications->setEnvelopeComplete('false')
$user_settings_information->setSenderEmailNotifications($user_email_notifications);
$users_api->updateSettings($account_id, $user_id, $user_settings_information);
Python
# You will need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
users_api = UsersApi(api_client)
user_settings_information = UserSettingsInformation()
user_email_notifications = SenderEmailNotifications()
user_email_notifications.envelope_complete = 'false'
user_settings_information.sender_email_notifications = user_email_notifications
users_api.update_settings(account_id, user_id, user_settings_information)
Ruby
# You will need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
users_api = DocuSign_eSign::UsersApi.new api_client
user_settings_information = DocuSign_eSign::UserSettingsInformation().new
user_email_notifications = DocuSign_eSign::SenderEmailNotifications().new
user_email_notifications.envelope_complete = 'false'
user_settings_information.sender_email_notifications = user_email_notifications
users_api.update_settings(account_id, user_id, user_settings_information)
And that’s a wrap! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
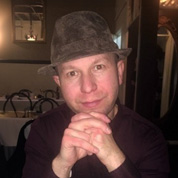
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
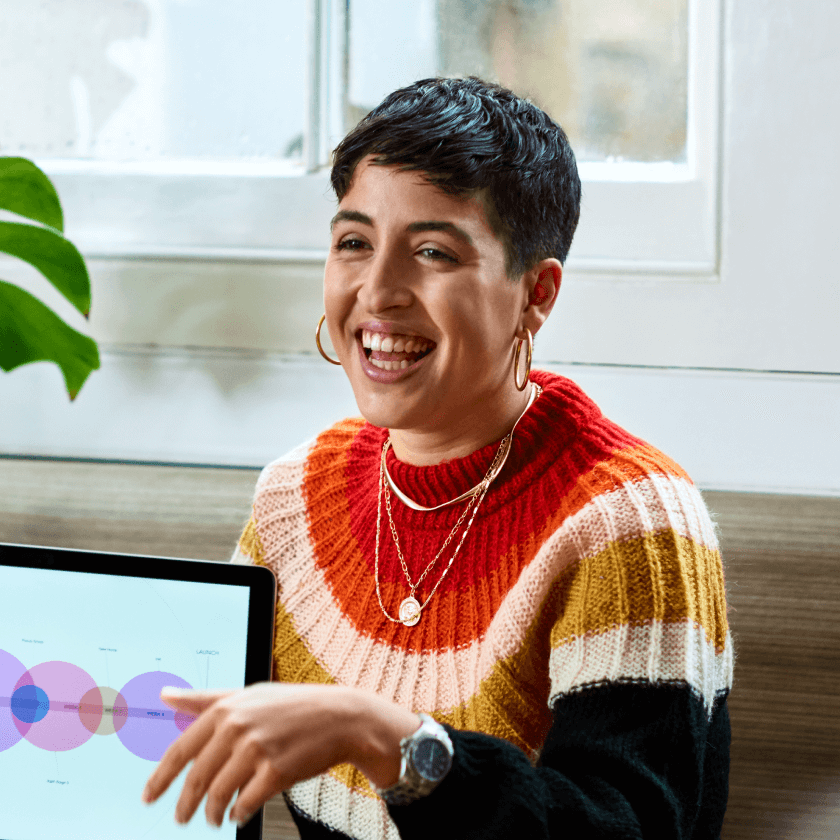