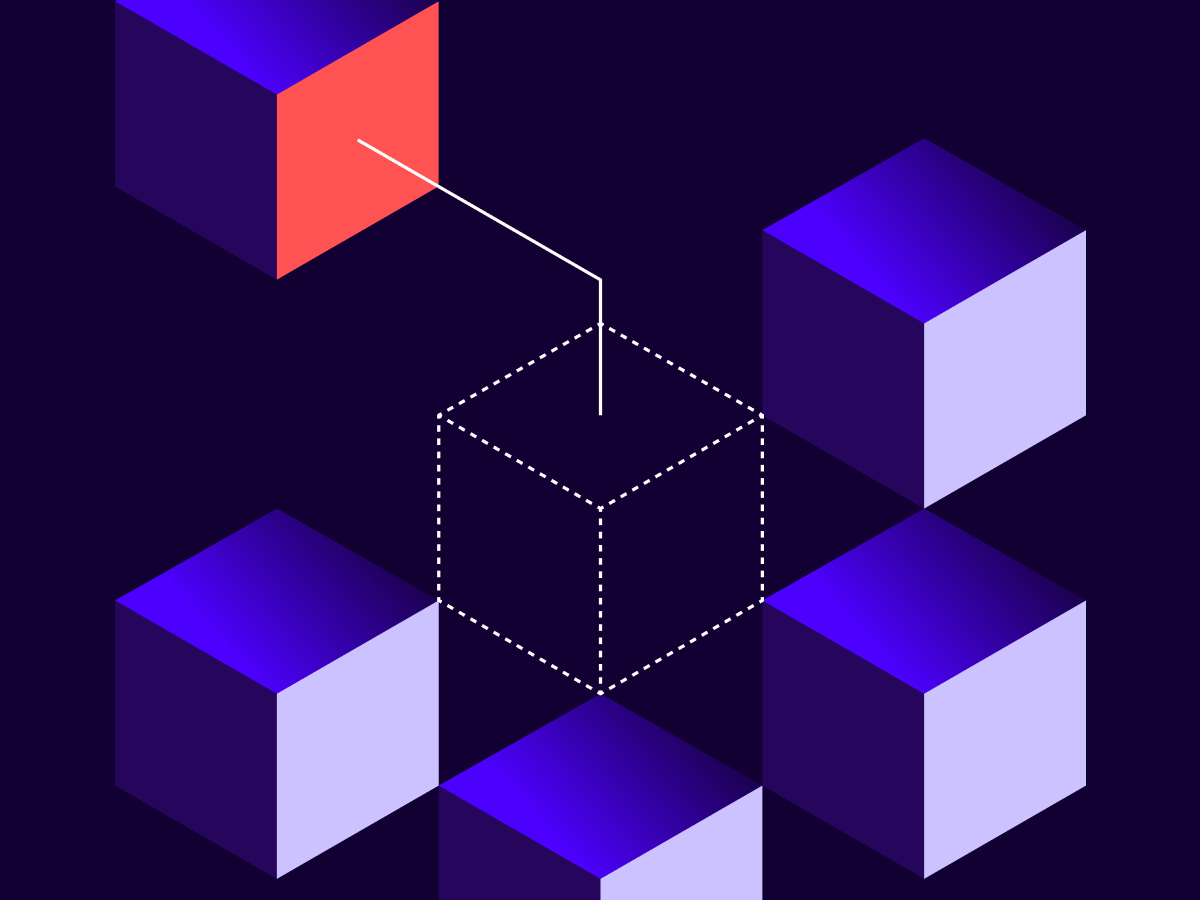
API Bytes: Updating your brand colors on envelopes
Get a byte of the API! This time, learn how to update your brand theme colors to customize the envelopes you send.
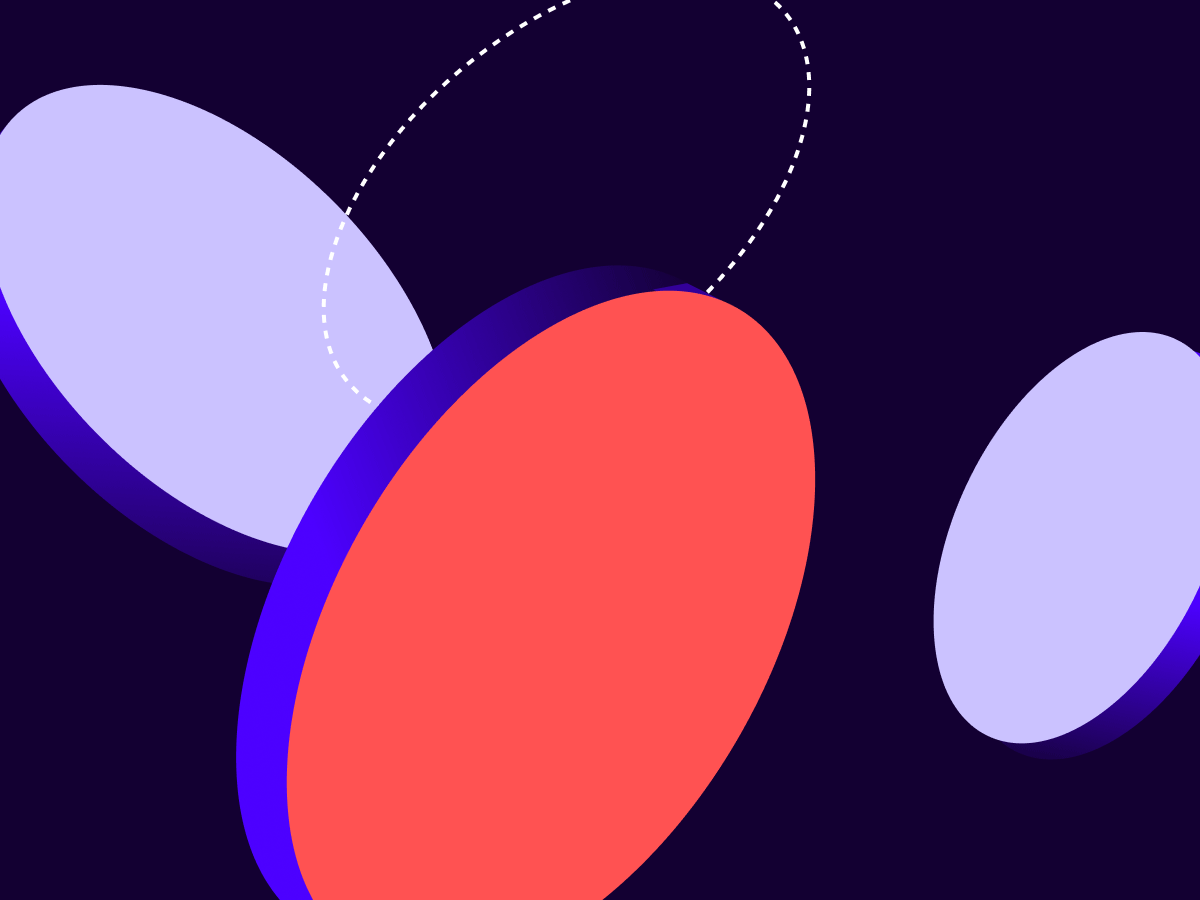
For today's tasty API Byte-sized morsel of goodness, I'm going to show you how to update your custom brand colors to your Docusign account through the eSignature AccountBrands resource to create a basic high-contrast look for the envelopes you send through the eSignature REST API.
Background
By applying custom colors with the AccountBrands resource, you can add a layer of cohesiveness to your marketing assets. Similar to logos, brand colors can touch both the envelope and the dashboard and help tailor eSignature to your own in-house branding.
What elements can I modify?
You can customize the header background, header text, button background, and button text. Your elements should have different colors between text and background to ensure that your text is visible. Let's try a combination of colors to produce a high-contrast theme. You provide these colors in a JSON object:
{
"colors": [
{
"name": "headerBackground",
"value": "#000000"
},
{
"name": "headerText",
"value": "#ffffff"
},
{
"name": "buttonPrimaryBackground",
"value": "#ffffff"
},
{
"name": "buttonPrimaryText",
"value": "#000000"
}
]
}
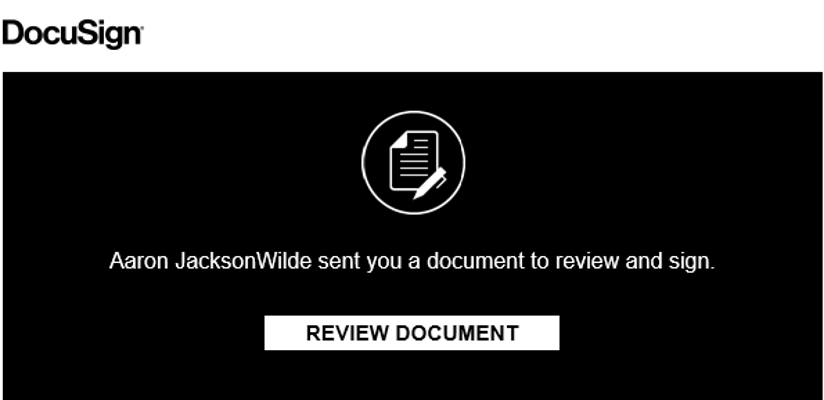
During testing, I found that if the button text and background colors were swapped, that no outline remained, which could allow you to employ a more subtle look, too:
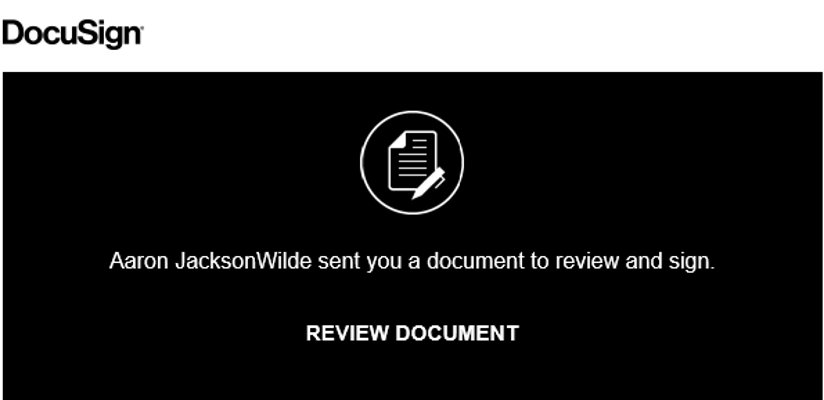
How it's done:
To update your brand colors on your envelopes, send an eSignature API PUT request to the AccountBrands resource or use the corresponding brands.update() method if using an SDK. When adding or configuring brands, any updates to values will show immediately within the response object. Just as you can set a brand image in the request body, you can do the same to update the header and button text and colors. To update the buttons, you need to use the names buttonPrimaryBackground and buttonPrimaryText to change those associated values for your button color and button text color. Here are some snippets showing you exactly how that is done:
Bash
# Update brand colors
# Step 1: Obtain your OAuth token
# Note: Substitute these values with your own
oAuthAccessToken="eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw"
#Set up variables for full code example
# Note: Substitute these values with your own
APIAccountId="2255f473-xxxx-xxxx-xxxx-c07c9abc6d76"
BrandId="95461dce-xxxx-xxxx-xxxx-68acae2ab5c0"
# Check that we're in a bash shell
if [[ $SHELL != *"bash"* ]]; then
echo "PROBLEM: Run these scripts from within the bash shell."
fi
base_path="https://demo.docusign.net/restapi"
# Step 2: Construct your API headers
declare -a Headers=('--header' "Authorization: Bearer ${oAuthAccessToken}" \
'--header' "Accept: application/json" \
'--header' "Content-Type: application/json")
# Step 3: Construct your request body
request_data=$(mktemp /tmp/request-update-colors.XXXXX)
printf \
'{
"colors": [
{
"name": "headerBackground",
"value": "#000000"
},
{
"name": "headerText",
"value": "#ffffff"
},
{
"name": "buttonPrimaryBackground",
"value": "#ffffff"
},
{
"name": "buttonPrimaryText",
"value": "#000000"
}
]
}' >> $request_data
# Step 4: a) Call the eSignature API
# b) Display the JSON response
# Create a temporary file to store the response
response=$(mktemp /tmp/response-brandcolors.XXXXXX)
Status=$(curl -w '%{http_code}' -i --request PUT https://demo.docusign.net/restapi/v2.1/accounts/$APIAccountId/brands/$BrandId/ \
"${Headers[@]}" \
--data-binary @${request_data} \
--output ${response})
# If the Status code returned is greater than 201 (OK/Accepted), display an error message along with the API response
if [[ "$Status" -gt "201" ]] ; then
echo ""
echo "Failed to update brand colors"
echo ""
cat $response
exit 1
fi
# Remove the temporary files
rm $request_data
rm $response
PowerShell:
# Update brand colors
# Step 1: Obtain your OAuth token
# Note: These values are not valid, but are shown for example purposes only!
$oAuthAccessToken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw"
# Set up variables for full code example
# Note: These values are not valid, but are shown for example purposes only!
$APIAccountId = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76"
$BrandId = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0"
# Step 2: Construct your API headers
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.add("Authorization","Bearer $oAuthAccessToken")
$headers.Add("Content-Type", "application/json")
# Step 3: Construct your request body
$body = @"
{
"colors": [
{
"name": "headerBackground",
"value": "#000000"
},
{
"name": "headerText",
"value": "#ffffff"
},
{
"name": "buttonPrimaryBackground",
"value": "#ffffff"
},
{
"name": "buttonPrimaryText",
"value": "#000000"
}
]
}
"@
# Step 4: a) Call the eSignature API
# b) Display the JSON response
$uri = "https://demo.docusign.net/restapi/v2.1/accounts/$APIAccountId/brands/$BrandId"
try{
write-host "Response:"
$response = Invoke-WebRequest -headers $headers -Uri $uri -Method PUT -Body $body
write-host "Success!"
$response
}
catch{
write-host "Unable to update brand colors."
write-host "Error : "$_.ErrorDetails.Message
write-host "Command : "$_.InvocationInfo.Line
}
C#
// Update brand colors
// Step 1: Obtain your OAuth token
// # Note: Substitute these values with your own
var accessToken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw";
// Set up variables for full code example
// Note: Substitute these values with your own
var accountId = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76";
var brandId = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0";
var basePath = "https://demo.docusign.net/restapi";
// Step 2. Construct your API headers
var apiClient = new ApiClient(basePath);
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
var colors = new List();
colors.Add(new NameValue { Name = "headerBackground", Value = "#000000" });
colors.Add(new NameValue { Name = "headerText", Value = "#ffffff" });
colors.Add(new NameValue { Name = "buttonPrimaryBackground", Value = "#000000" });
colors.Add(new NameValue { Name = "buttonPrimaryText", Value = "#ffffff" });
// Step 3. Construct your request body
Brand brand = new Brand
{
BrandName = brandName,
DefaultBrandLanguage = defaultBrandLanguage,
Colors = colors
};
// Step 4. Call the eSignature REST API
var accountsApi = new AccountsApi(apiClient);
var results = accountsApi.UpdateBrand(accountId, brandId, brand);
And there you have it! Update the custom theme profile for your various brands to take the level of customization up a notch for your next project or wow your prospective clients by tailoring their signing experience to their own matching thematic branding. In my next API Bytes post, I'll demonstrate revoking application consent through the API. It will be an exciting one, so keep on the lookout!
Additional resources
Aaron Jackson-Wilde has been with Docusign since 2019 and specializes in API-related developer content. Aaron contributes to the Quickstart launchers, How-To articles, and SDK documentation, and helps troubleshoot occasional issues on GitHub or StackOverflow.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
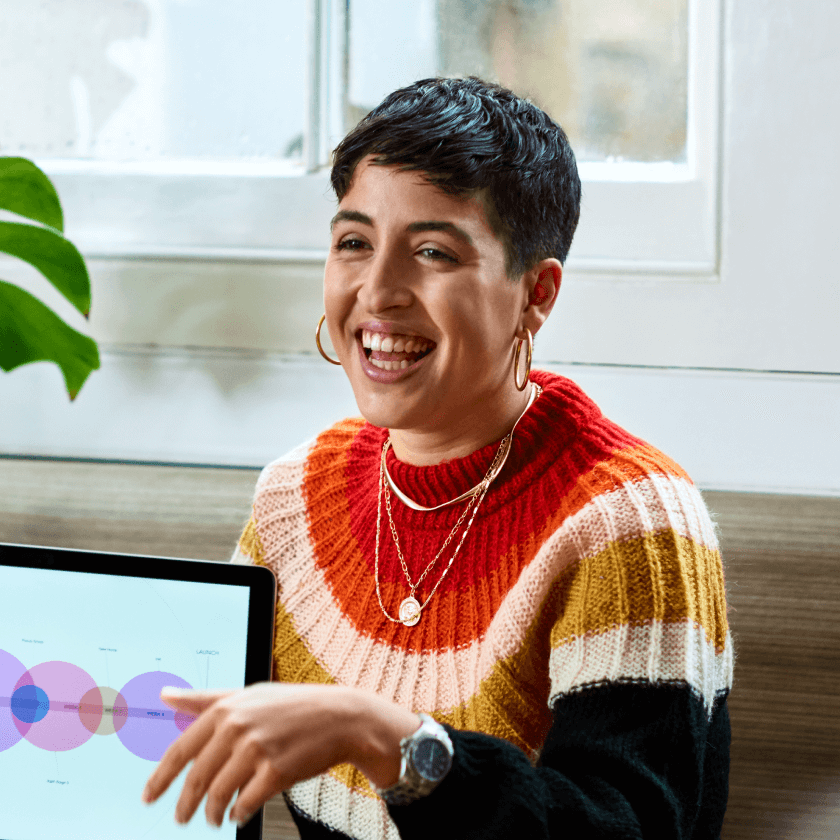