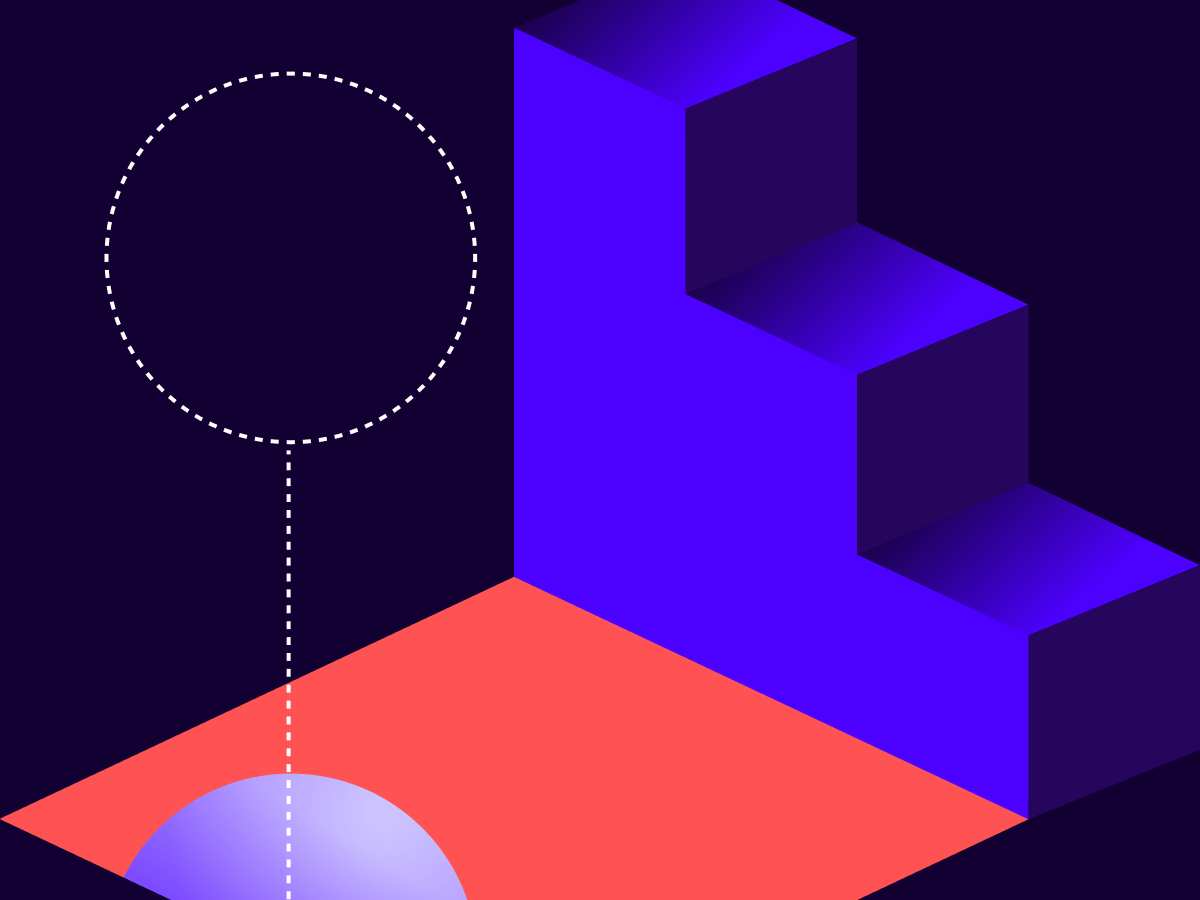
API Bytes: Onboarding your cloud storage provider
Get a byte of the API! This time, learn how to add and interface with cloud storage providers through the eSignature REST API.
Table of contents
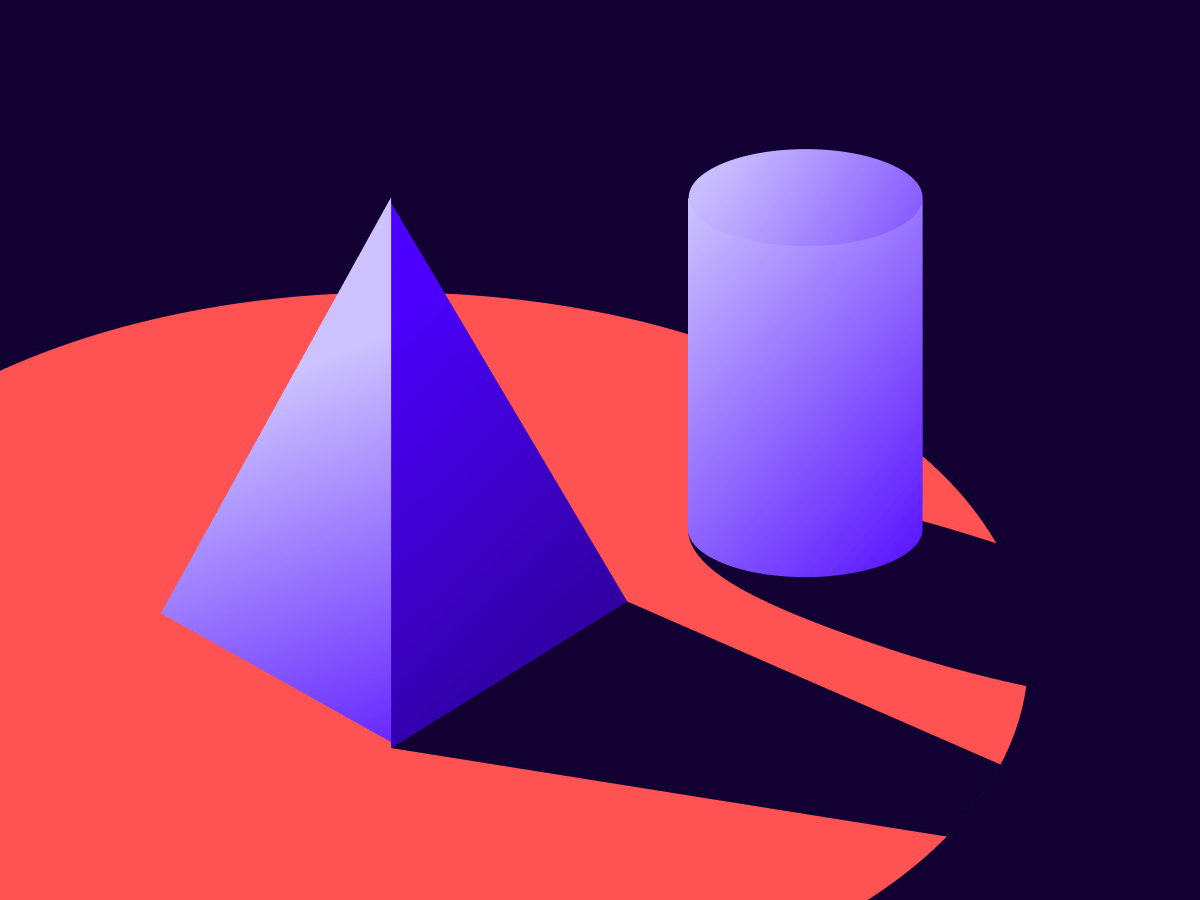
Maybe you're asking, "Who cares about the cloud?" To which I respond, "Who doesn't!" In this post I’m going to show you how to connect your Docusign integration with popular cloud storage providers.
Cloud storage providers offer the means to back up and store your files across the web, powered through the technology of cloud computing. Storing your own completed Docusign envelopes is, in and of itself, a mechanism that uses Docusign servers as a cloud storage provider. Docusign offers integrations we support through eSignature that enable you to connect your Docusign account to the following cloud storage providers: Google Drive, Dropbox, Docusign Rooms, Box, and Salesforce. If you already have services connected through them or documents and process infrastructure built in on those platforms, why not leverage them? Imagine, for instance, a scenario where you want to keep an entire document store in the cloud. The Docusign eSignature REST API enables you directly to verify document availability on a cloud storage provider without the need for a separate SDK or OAuth process.
Request an authorization URL for your cloud storage provider
To use one of the cloud storage providers I've mentioned, make the API call shown in the code below to request an authorization URL. You'll need to specify the service name and your application's callback URL, so your application can continue through its own processes. If your service name happens to include two or more words, such as "Google Drive", remove the space and set the name to lowercase, such that "Google Drive" becomes "googledrive".
Bash
# Retrieve authorization URLs
oAuthAccessToken="eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw"
AccountId="2255f473-xxxx-xxxx-xxxx-c07c9abc6d76"
# A UserId is needed to associate a specific account user within your account or organization to your cloud storage provider. The UserId is a GUID value that uniquely identifies a Docusign user. See https://developers.docusign.com/platform/configuring-app/ for details.
UserId="95461dce-xxxx-xxxx-xxxx-68acae2ab5c0"
# Construct your envelope JSON body
printf \
'{
"storageProviders": [
{
"redirectUrl": "localhost:8080/callback",
"service": "dropbox"
},
{
"redirectUrl": "localhost:8080/callback",
"service": "skydrive"
}
]
}' >> $request_data
base_path="https://demo.docusign.net/restapi"
# Construct your API headers
declare -a Headers=('--header' "Authorization: Bearer ${oAuthAccessToken}" \
'--header' "Accept: application/json" \
'--header' "Content-Type: application/json" )
# Call the eSignature REST API
Status=$(curl -w '%{http_code}' -i --request POST https://demo.docusign.net/restapi/v2.1/accounts/${accountId}/users/${userId}/cloud_storage \
"${Headers[@]}" \
--data-binary ${request_data} \
--output ${response})
# If the Status code returned is greater than 201 (OK/Accepted), display an error message along with the API response
if [[ "$Status" -gt "201" ]] ; then
echo ""
echo "Failed to retrieve cloud provider authorization URLs"
echo ""
cat $response
exit 1
fi
echo "Cloud provider urls:"
cat $response
# Remove the temporary files
rm "$request_data"
rm "$response"
C#
// Retrieve authorization URLS
var basePath = "https://demo.docusign.net/restapi/v2.1/accounts/restapi";
var accessToken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw";
var AccountId = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76";
// A UserId is needed to associate a specific account user within your account or organization to your cloud storage provider. The UserId is a GUID value that uniquely identifies a Docusign user. See https://developers.docusign.com/platform/configuring-app/ for details.
var userId = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0";
// Construct your API headers
var apiClient = new ApiClient(basePath);
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
CloudStorageApi CloudStorage = new CloudStorageApi(apiClient);
CloudStorageProviders storageProviders = new CloudStorageProviders();
storageProviders.StorageProviders.Add(new CloudStorageProvider { Service = "dropbox", RedirectUrl = "localhost:8080/callback", });
storageProviders.StorageProviders.Add(new CloudStorageProvider { Service = "googledrive", RedirectUrl = "localhost:8080/callback", });
// Call the eSignature REST API
CloudStorage.CreateProvider(AccountId, userId, storageProviders);
Java
String oAuthAccessToken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw";
String AccountId = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76";
// A UserId is needed to associate a specific account user within your account or organization to your cloud storage provider. The UserId is a GUID value that uniquely identifies a Docusign user. See https://developers.docusign.com/platform/configuring-app/ for details.
String userId = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0";
String basePath ="https://demo.docusign.net/restapi";
// Construct your API headers
ApiClient apiClient = new ApiClient(basePath);
apiClient.addDefaultHeader(HttpHeaders.AUTHORIZATION, BEARER_AUTHENTICATION + oAuthAccessToken);
CloudStorageApi CloudStorage = new CloudStorageApi(apiClient);
CloudStorageProvider sp1 = new CloudStorageProvider();
sp1.setService("googledrive");
sp1.setRedirectUrl("localhost:8080/callback");
CloudStorageProvider sp2 = new CloudStorageProvider();
sp1.setService("dropbox");
sp1.setRedirectUrl("localhost:8080/callback");
CloudStorageProviders csp = new CloudStorageProviders();
csp.addStorageProvidersItem(sp1);
csp.addStorageProvidersItem(sp2);
// Call the eSignature REST API
CloudStorage.createProvider(AccountId, userId, csp);
Node.js
// Retrieve authorization URLs
let oAuthAccessToken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw";
let AccountId = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76";
let basePath = "https://demo.docusign.net/restapi";
// A UserId is needed to associate a specific account user within your account or organization to your cloud storage provider. The UserId is a GUID value that uniquely identifies a Docusign user. See https://developers.docusign.com/platform/configuring-app/ for details.
let userId = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0";
// Construct your API headers
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + oAuthAccessToken);
let CloudStorageApi = new docusign.CloudStorageApi(dsApiClient);
let CloudStorageProvider = new docusign.CloudStorageProvider({
Service = "dropbox",
RedirectUrl = "localhost:8080/callback"
});
let CloudStorageProviderB = new docusign.CloudStorageProvider({
Service = "skydrive",
RedirectUrl = "localhost:8080/callback"
});
// Call the eSignature REST API
CloudStorageApi.createProvider(AccountId, userId, [CloudStorageProvider, CloudStorageProviderB]).then((res)=>{ console.log(res) });
PHP
# Retrieve authorization URLs
$oAuthAccessToken="eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw";
# A UserId is needed to associate a specific account user within your account or organization to your cloud storage provider. The UserId is a GUID value that uniquely identifies a Docusign user. See https://developers.docusign.com/platform/configuring-app/ for details.
$UserId="95461dce-xxxx-xxxx-xxxx-68acae2ab5c0";
$AccountId="2255f473-xxxx-xxxx-xxxx-c07c9abc6d76";
$base_path="https://demo.docusign.net/restapi";
# Construct your API headers
$config = new Configuration();
$config->setHost($base_path);
$config->addDefaultHeader('Authorization', 'Bearer ' . $oAuthAccessToken);
$apiClient = new ApiClient($config);
$CloudStorageApi = new CloudStorageApi($apiClient);
$dropbox = new CloudStorageProvider(["service"=>"dropbox", "redirectUrl"=>"localhost:8080/callback" ]);
$skydrive = new CloudStorageProvider(["service"=>"skydrive", "redirectUrl"=>"localhost:8080/callback" ]);
$csp = new CloudStorageProviders([$dropbox, $skydrive]);
# Call the eSignature REST API
$CloudStorageApi->createProvider($AccountId, $UserId,$csp);
PowerShell
# Retrieve authorization URLs
$oAuthAccessToken="eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw"
$AccountId="2255f473-xxxx-xxxx-xxxx-c07c9abc6d76"
# A UserId is needed to associate a specific account user within your account or organization to your cloud storage provider. The UserId is a GUID value that uniquely identifies a Docusign user. See https://developers.docusign.com/platform/configuring-app/ for details.
$UserId="95461dce-xxxx-xxxx-xxxx-68acae2ab5c0"
# Construct your API headers
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.add("Authorization", "Bearer $oAuthAccessToken")
$headers.add("Accept", "application/json")
$headers.add("Content-Type", "application/json")
# Construct your envelope JSON body
$body = @"
{
"storageProviders": [
{
"redirectUrl": "localhost:8080/callback",
"service": "dropbox"
},
{
"redirectUrl": "localhost:8080/callback",
"service": "skydrive"
}
]
}
"@
Write-Output ""
Write-Output "Request: "
Write-Output $body
# Call the eSignature REST API
$uri = "https://demo.docusign.net/restapi/v2.1/accounts/${AccountId}/users/${UserId}/cloud_storage"
# Trap errors in the response
try {
Write-Output "Response:"
$result = Invoke-WebRequest -uri $uri -headers $headers -body $body -method POST
$result.content
}
catch {
$int = 0
foreach ($header in $_.Exception.Response.Headers) {
if ($header -eq "X-Docusign-TraceToken") { Write-Output "TraceToken : " $_.Exception.Response.Headers[$int] }
$int++
}
Write-Output "Error : "$_.ErrorDetails.Message
Write-Output "Command : "$_.InvocationInfo.Line
}
Python
# Get Authorization URLs
access_token = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw"
base_path = "https://demo.docusign.net/restapi"
api_account_id = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76"
# A UserId is needed to associate a specific account user within your account or organization to your cloud storage provider. The UserId is a GUID value that uniquely identifies a Docusign user. See https://developers.docusign.com/platform/configuring-app/ for details.
user_id = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0"
# Construct your API headers
api_client = create_api_client(base_path=base_path, access_token=access_token)
cloud_storage_api = CloudStorageApi(api_client)
dropbox = CloudStorageProvider(redirect_url="localhost:8080/callback", service="dropbox")
skydrive = CloudStorageProvider(redirect_url="localhost:8080/callback", service="skydrive")
csp = CloudStorageProviders([dropbox, skydrive])
# Call the eSignature REST API
CloudStorageApi.create_provider(api_account_id, user_id, csp)
Ruby
# Retrieve authorization URLs
oAuthAccessToken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSU.....mWD0yY6sztXipERLlTbdNjCRjruRsVFaw"
base_path="https://demo.docusign.net/restapi"
api_account_id = "2255f473-xxxx-xxxx-xxxx-c07c9abc6d76"
# A UserId is needed to associate a specific account user within your account or organization to your cloud storage provider. The UserId is a GUID value that uniquely identifies a Docusign user. See https://developers.docusign.com/platform/configuring-app/ for details.
user_id = "95461dce-xxxx-xxxx-xxxx-68acae2ab5c0"
configuration = DocuSign_eSign::Configuration.new
configuration.host = base_path
api_client = DocuSign_eSign::ApiClient.new configuration
# Construct your API headers
api_client.default_headers['Authorization'] = "Bearer #{oAuthAccessToken}"
cloud_storage_api = DocuSign_eSign::CloudStorageApi.new api_client
dropbox = DocuSign_esign::CloudStorageProvider.new
dropbox.service = "dropbox"
dropbox.redirect_url = "localhost:8080/callback"
skydrive = DocuSign_esign::CloudStorageProvider.new
skydrive.service = "skydrive"
skydrive.redirect_url = "localhost:8080/callback"
cloud_storage_providers = DocuSign_eSign::CloudStorageProviders.new
cloud_storage_providers
<p>The eSignature REST API will return an authorization URL that redirects first to your cloud service provider, then back to your application. Use this URL to register authorization and use of the cloud service provider and to grant consent for use. Similar to our own <a href="https://developers.docusign.com/platform/auth/">OAuth grant process</a>, you're required to select the account and to allow it to authorize consent for use.</p>
<h2>Retrieve a list of folders in cloud storage</h2>
<p>Now you can use the <a href="https://developers.docusign.com/docs/esign-rest-api/reference/cloudstorage/cloudstorage/listfolders/">CloudStorage: listFolders</a> call to determine the various folder IDs (or perhaps collect the aggregate list and parse it post processing, if you happen to make a lot of moves with new users and folders).</p>
<h3>Bash</h3>
<code class="language-bash" data-uuid="xzI0WDep">curl --request GET "https://demo.docusign.net/restapi/v2.1/accounts/${account_id}/users/${user_id}/cloud_storage/${service_id}/folders/" \
"${Headers[@]}" \
--output ${response}</code>
<h3>C#</h3>
<code class="language-cpp" data-uuid="v7ApLZ5s">CloudStorage.ListFolders(AccountId, userId, "googledrive");</code>
<h3>Java</h3>
<code class="language-java" data-uuid="pzG0etCL">CloudStorage.listFolders(AccountId, userId, serviceId);</code>
<h3>Node.js</h3>
<code class="language-javascript" data-uuid="iWeX36nG">CloudStorageApi.listFolders(AccountId, userId, serviceId ).then((res)=>{ console.log(res) });</code>
<h3>PHP</h3>
<code class="language-php" data-uuid="xHpCz8Xe">CloudStorageApi->listFolders($AccountId, $serviceId, $UserId);</code>
<h3>PowerShell</h3>
<code class="language-bash" data-uuid="yQsv7xhR">$uri = "https://demo.docusign.net/restapi/v2.1/accounts/${AccountId}/users/${UserId}/cloud_storage/${serviceId}/folders"</code>
<h3>Python</h3>
<code class="language-python" data-uuid="np8eUA1O">CloudStorageApi.list_folders(api_account_id, service_id, user_id)</code>
<h3>Ruby</h3>
<code class="language-ruby" data-uuid="oWsJ1uIy">cloud_storage_api.list_folders(api_account_id, service, user_id)</code>
<h2>Retrieve file information from cloud folders</h2>
<p>With the folder IDs in hand, you can then use the <a href="https://developers.docusign.com/docs/esign-rest-api/reference/cloudstorage/cloudstorage/list/">CloudStorage: list</a> API call to retrieve the attribute information about the documents stored on your cloud storage provider. If your application has an Extract Transform Load (ETL) capability of some sort, then being able to parse the contents of your cloud storage providers is instrumental to those efforts.</p>
<h3>Bash</h3>
<code class="language-bash" data-uuid="la3Dd3cG">curl --request GET "https://demo.docusign.net/restapi/v2.1/accounts/${account_id}/users/${user_id}/cloud_storage/${service_id}/folders/${folder_id}" \
"${Headers[@]}" \
--output ${response}</code>
<h3>C#</h3>
<code class="language-cpp" data-uuid="eQp8TwDJ">CloudStorage.List(AccountId, userId, "googledrive", folderId);</code>
<h3>Java</h3>
<code class="language-java" data-uuid="sR3VaWe3">CloudStorage.list(AccountId, userId, serviceId, folderId);</code>
<h3>Node.js</h3>
<code class="language-javascript" data-uuid="coFWC51c">CloudStorageApi.list(AccountId, userId, serviceId,folderId).then((res)=>{ console.log(res) });</code>
<h3>PHP</h3>
<code class="language-php" data-uuid="vxaexhXP">$CloudStorageApi->callList($AccountId, $folderId, $serviceId, $UserId)</code>
<h3>PowerShell</h3>
<code class="language-bash" data-uuid="jnZ7f5HB">$uri = "https://demo.docusign.net/restapi/v2.1/accounts/${AccountId}/users/${UserId}/cloud_storage/${serviceId}/folders/${folderId}"</code>
<h3>Python</h3>
<code class="language-python" data-uuid="hGHbFpFC">CloudStorageApi.list(api_account_id, folder_id, service_id, user_id)</code>
<h3>Ruby</h3>
<code class="language-ruby" data-uuid="y7yHjRuT">cloud_storage_api.list(api_account_id, folder_id, service, user_id)</code>
<p>And that's about as byte-sized as we can go this round. If you're looking for ways to harness external cloud storage providers within your own systems, use the eSignature REST API to ease headaches within your ETL process and stop having to juggle OAuth tokens.</p>
<p>We're always on the lookout for the next big question to be answered regarding Docusign APIs. If you have a burning question or wish to see a concept outlined, don't hesitate to reach out via email with your comments or concerns to <a href="mailto:developers@docusign.com">developers@docusign.com</a>. We appreciate you. </p>
<h2>Additional resources</h2>
<ul><li><a href="https://www.docusign.com/blog/developers/updated-docusign-university-learning-portal-developers">Updated Docusign University Learning Portal for Developers</a></li>
<li><a href="https://www.docusign.com/blog/developers/debugging-api-calls">Debugging API Calls</a></li>
<li><a href="https://www.docusign.com/blog/developers/building-react-app-part-3-the-code-example">Building a React app, Part 3</a></li>
<li><a href="https://www.docusign.com/blog/developers/new-api-explorer-generally-available">New API Explorer generally available</a></li>
<li><a href="https://developers.docusign.com/esign-rest-api/guides/building-integration">Guide: Build an eSignature Integration</a></li>
</ul>
Aaron Jackson-Wilde has been with Docusign since 2019 and specializes in API-related developer content. Aaron contributes to the Quickstart launchers, How-To articles, and SDK documentation, and helps troubleshoot occasional issues on GitHub or StackOverflow.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
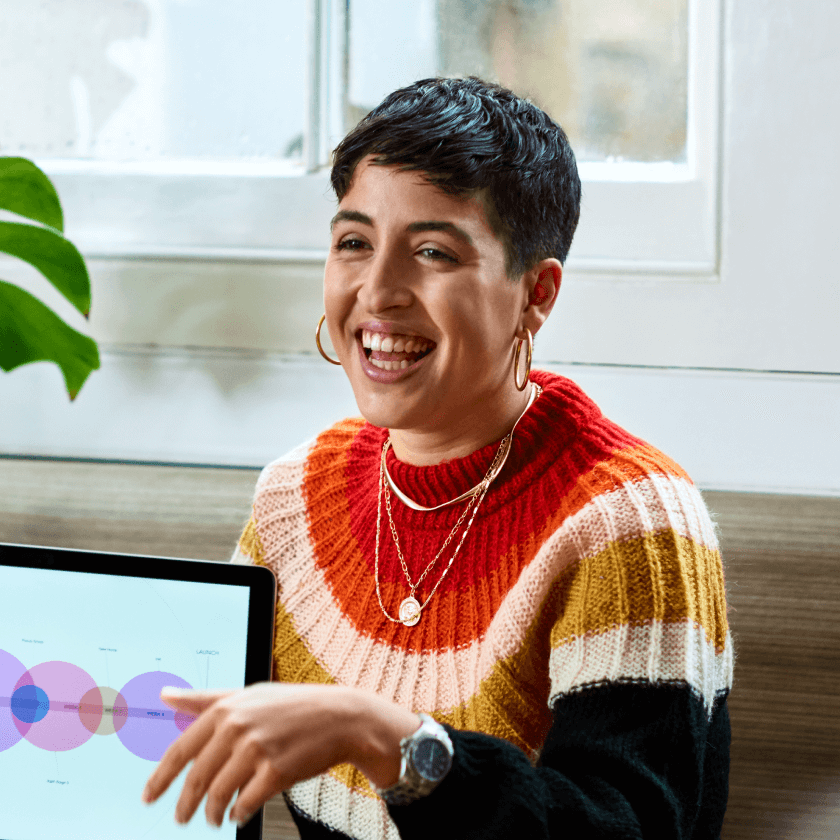