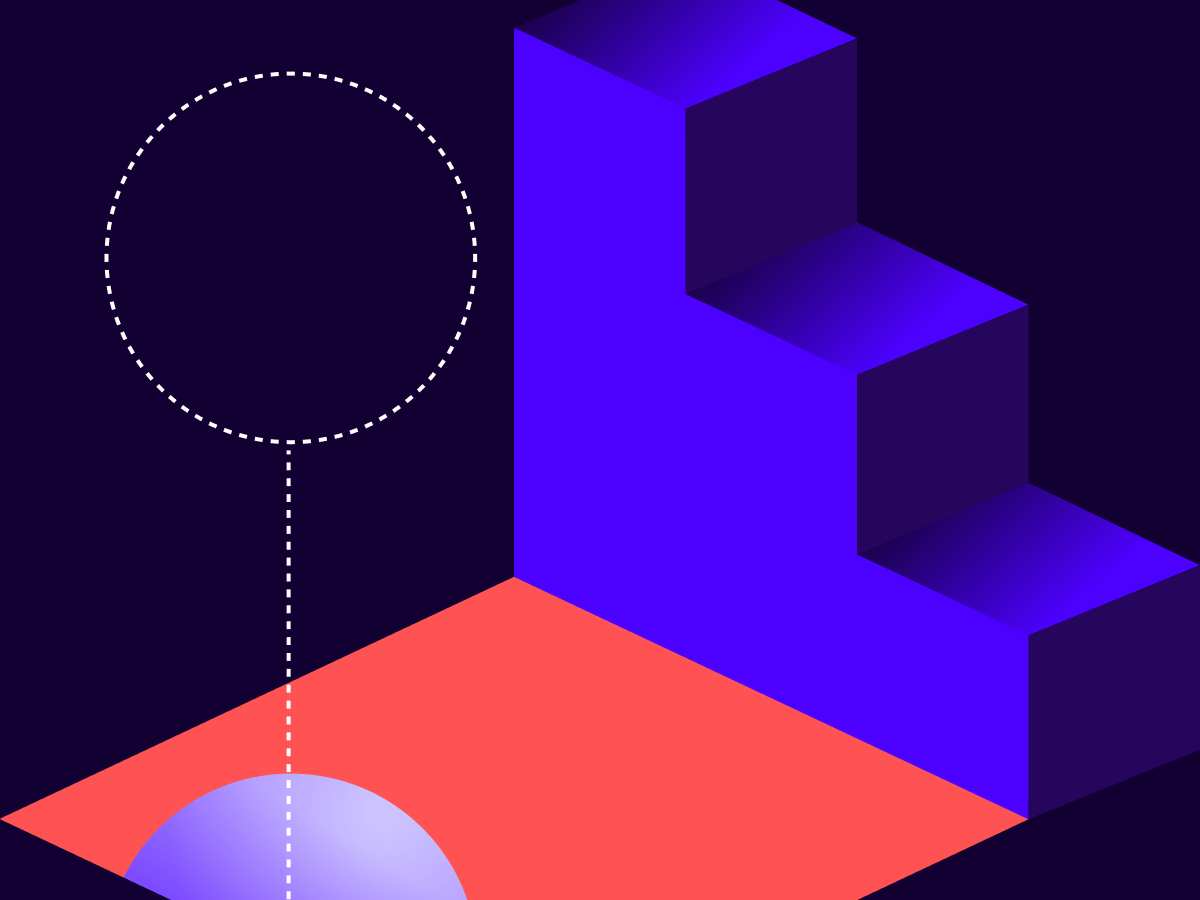
From the Trenches: Optimize your SOAP API calls with server-specific base URIs
Discover how to optimize the performance of your eSignature SOAP API calls by using the base URI specific to your account.
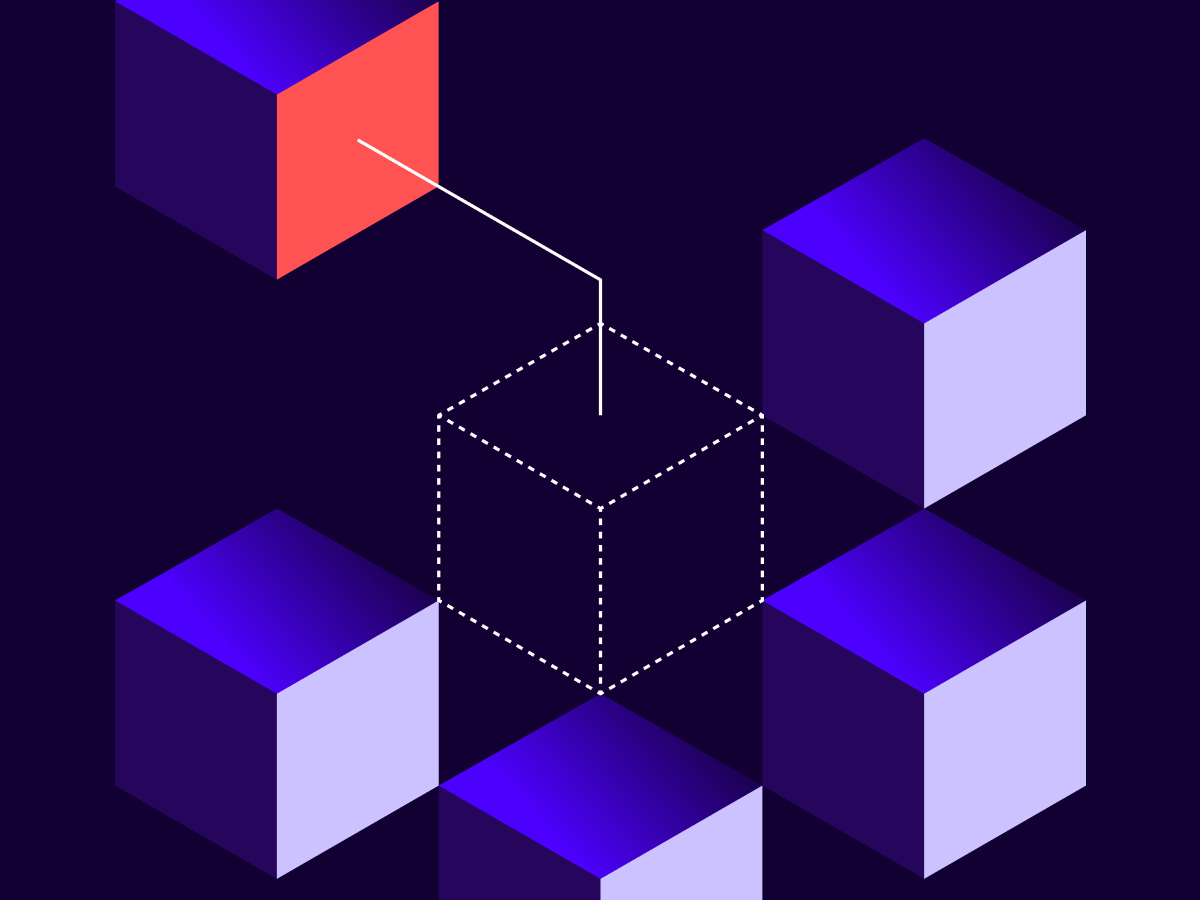
When it comes to using an API, one of the key considerations is ensuring that you are communicating with the correct server. In most cases, this means making an HTTP request against a specific endpoint URL that identifies the location of the API resources. With your Docusign account, this specific endpoint may be different depending on the region and data center where your account is located. We refer to this as your account “site” or “server”.
In Docusign’s developer environment there is only one server, named “demo”, so the topic of this article is not especially relevant for a demo integration. However, the production environment includes a number of different servers such as NA1 (www), NA2, NA3, EU, CA, and others.
Why should I care?
If you are using the eSignature SOAP API, ensuring that the server is correctly configured in your API calls can mitigate some known troubleshooting headaches and potentially improve the response times of your calls. The SOAP API behaves differently than the REST API, as the REST API will error and reject calls that are not made to the correct server.
Our SOAP API is designed in a way that you do not actually need to specify the server. It uses a cross-site forwarding proxy to automatically forward the API calls from one server to the next until it finds the one your account is on. While this may sound convenient, it does take some time to cycle through each server, which is why specifying the server in your API calls can potentially improve performance and save a couple of seconds per call.
Where can I find my server?
As an administrator on a Docusign account, you can find your server by finding your Account Base URI. To find this on your account, navigate to Settings > Apps and Keys. The Apps and Keys page is where you can find API information about your account and manage any integration keys that you may own. One of these pieces of API information is the Account Base URI.
The base URI will always be in the form of https://{server}.docusign.net/, where the server will be replaced with your account’s specific server. For example, here is a view of an account on the NA4 server:
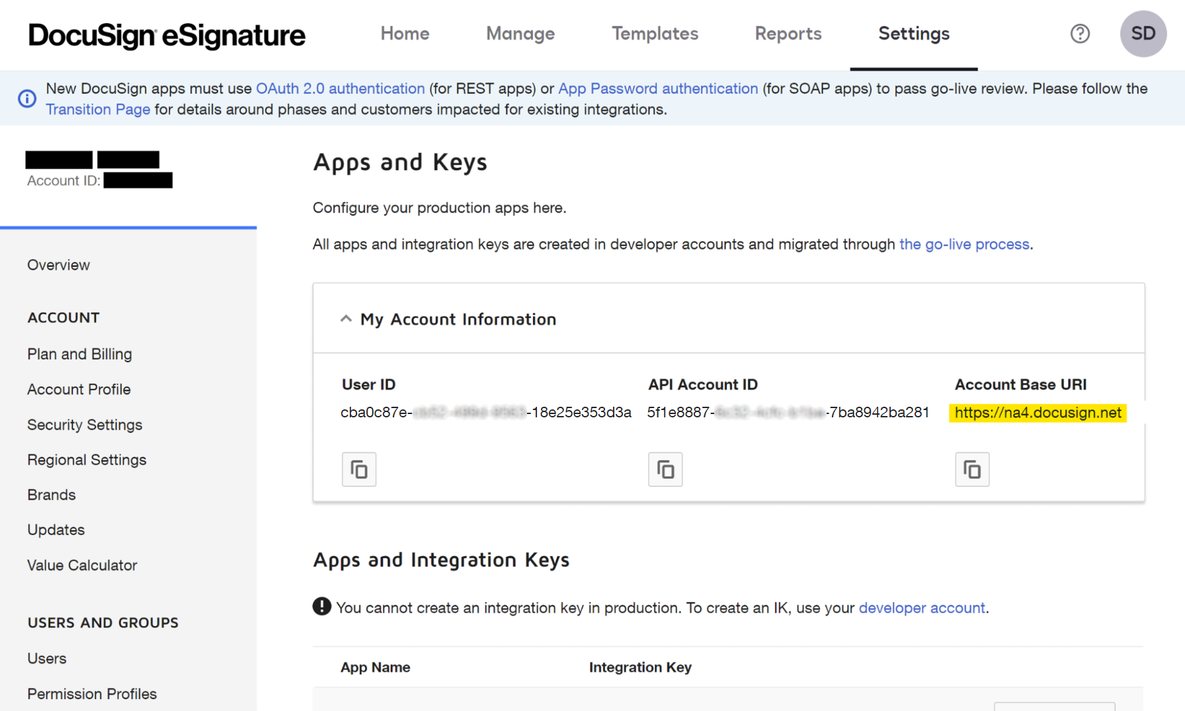
Now let's take a look at how you can get the base URI from an API call. Using the SOAP API, you can do this by calling the Credentials API Group with the Login method. Here is an example of a request and a response using this method:
Request
POST /api/3.0/credential.asmx HTTP/1.1
Host: www.docusign.net
Content-Type: text/xml; charset=utf-8
Content-Length: length
SOAPAction: "http://www.docusign.net/API/Credential/Login"
<?xml version="1.0" encoding="utf-8"?><envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"><body><login xmlns="http://www.docusign.net/API/Credential"><email>user@example.com</email><password>*omitted*</password></login></body>
</envelope>
Response
HTTP/1.1 200 OK
Content-Type: text/xml; charset=utf-8
Content-Length: length
<envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:wsa="http://schemas.xmlsoap.org/ws/2004/08/addressing" xmlns:wsse="http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd" xmlns:wsu="http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd"><header><action>http://www.docusign.net/API/Credential/LoginResponse</action><to>http://schemas.xmlsoap.org/ws/2004/08/addressing/role/anonymous</to><security><timestamp wsu:id="Timestamp-324bf44e-e98f-464a-880d-d40bd9d4798f"><created>2023-03-14T02:52:08Z</created><expires>2023-03-14T02:57:08Z</expires></timestamp></security></header><body><loginresponse xmlns="http://www.docusign.net/API/Credential"><loginresult><success>true</success><errorcode>Success</errorcode><authenticationmessage>Successful authentication</authenticationmessage><accounts><account><accountid>xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxx2cba</accountid><accountname>Example Account</accountname><userid>xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxd3e6</userid><username>Example User</username><email>user@example.com</email><baseurl>https://NA4.docusign.net/api/3.0</baseurl></account></accounts></loginresult></loginresponse></body>
</envelope>
You can see in the response here that a list of accounts is returned, which also includes a property. My example here only includes one account, but you may see more if the user is a member of multiple accounts.
Now that you know your Base URL, you can use it in your API calls. This will ensure the calls coming from your integration are pointing to the correct server. So in my example of an account on the NA4 server, if I am using the DSAPI.ASMX service endpoint, I will update my endpoints as follows:
Old: https://www.docusign.net/api/3.0/dsapi.asmx
New: https://na4.docusign.net/api/3.0/dsapi.asmx
Again, this example uses the DSAPI.ASMX service endpoint, but this will also work for the accountmanagement.ASMX and API.ASMX service endpoints.
One important thing to note is that you are updating the request endpoint, but you are not updating the “SOAPAction” header. The SOAPAction header should still use the https://www.docusign.net convention for its base path.
What if I don’t own the integration?
If you are using a third-party application that is integrated with Docusign, then you will likely have very little control over the code that is making the API calls to Docusign. However, many integrations that use our SOAP API have settings or configuration pages that ask you to input your base URI. Some older guides may suggest for you to use the https://www.docusign.net base URI because it will work just fine, but as you learned above, specifying your base URI will always be preferred and may improve your overall performance. If this option is not available for configuration, please reach out to the integration owner for guidance and support.
Additional resources
Steve DiCicco is an experienced support engineer who has been with Docusign since 2022. You can reach Steve on LinkedIn.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
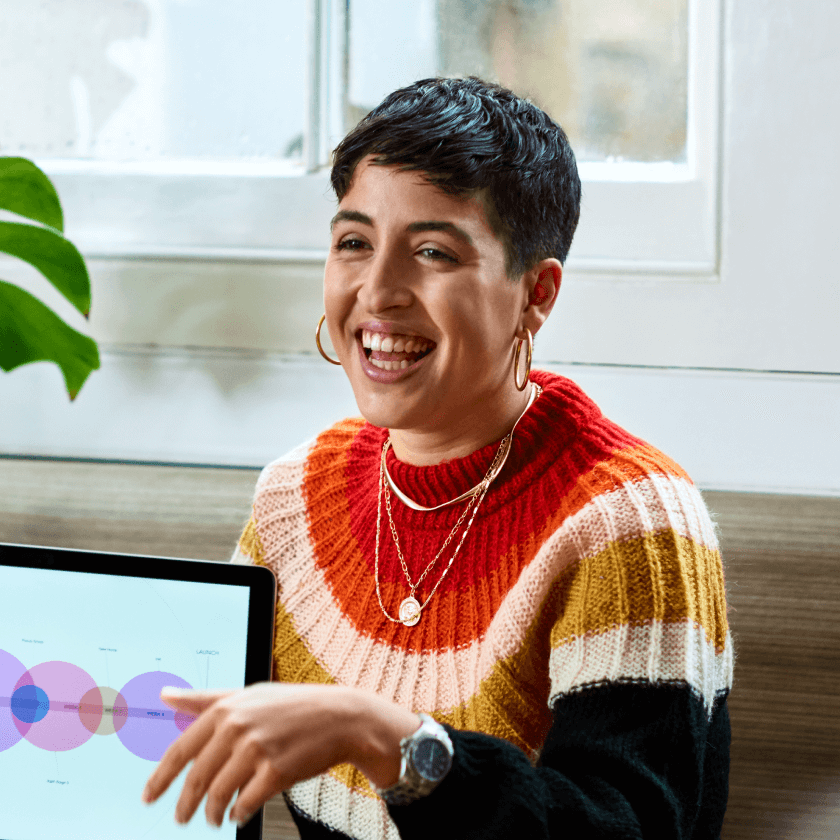