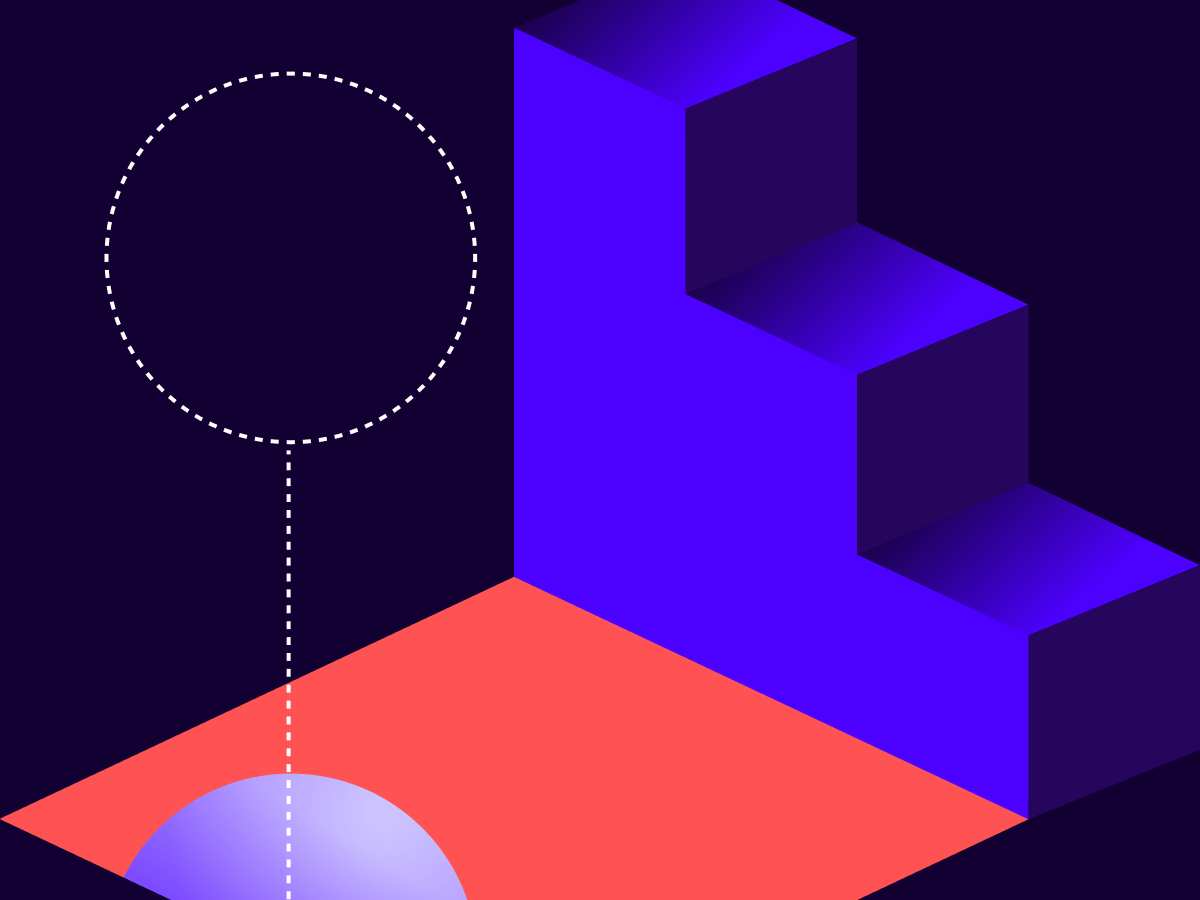
New mobile code example: Making direct API calls with Docusign Mobile SDKs
Our new example shows you in both iOS and Android how to make calls to eSignature API endpoints unsupported by SDK methods.
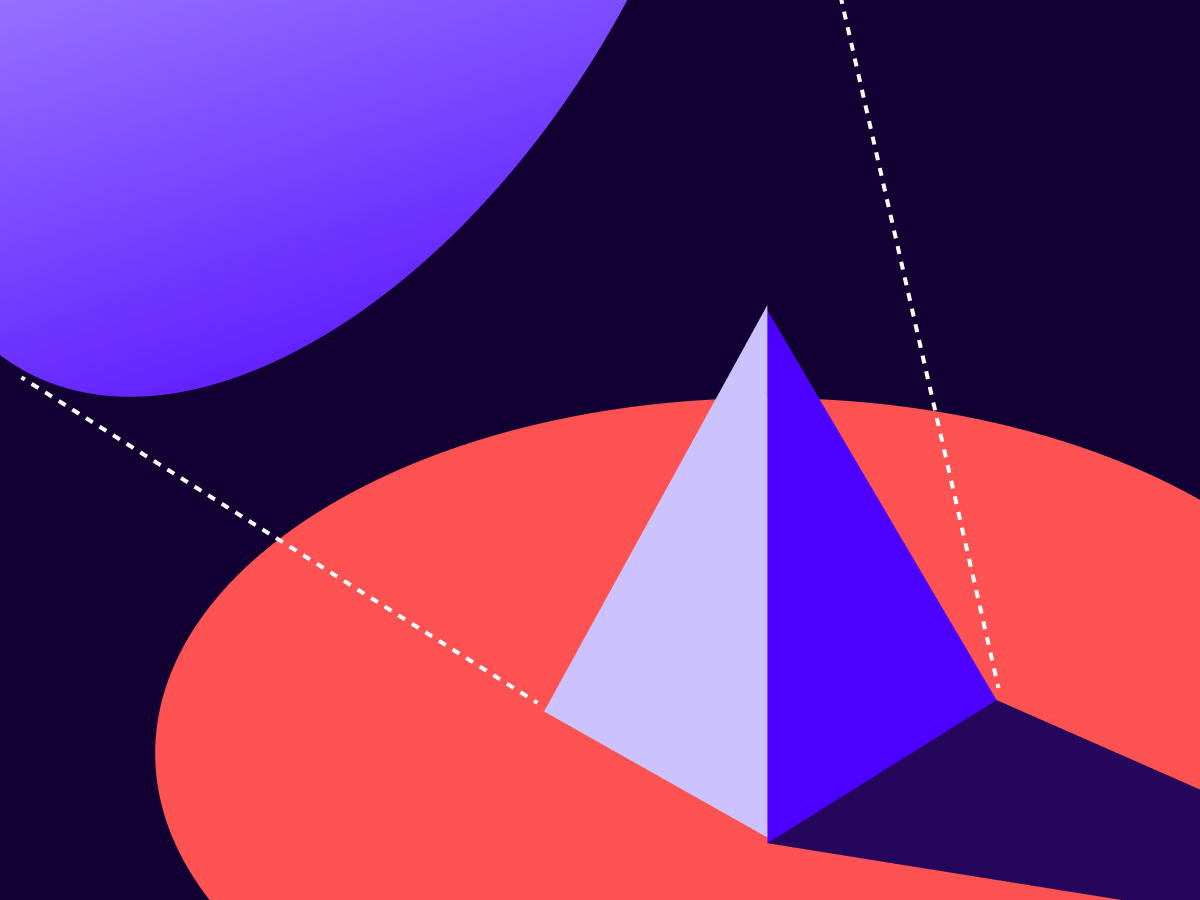
Today, I will cover an exciting feature for the eSignature REST API mobile SDKs. Our new How to make a direct API call example, available for both iOS and Android, demonstrates how app developers can make direct API calls to the eSignature REST API from the mobile SDKs. You may find this useful if you want to incorporate eSignature REST API functions where there is no existing SDK method.
Note: For this post, I chose to demonstrate this feature using the Android SDK in Java.
Configuring your app for Docusign mobile SDKs
To try out the new example, you first need to integrate the Docusign mobile SDK frameworks into your app. You can find the instructions on how to do this in the readme.md files for the Android SDK and iOS SDK.
Once you’ve integrated the mobile SDKs in your app, ensure that you have the following dependencies in your build.gradle file for Android to invoke the eSign REST API directly:
dependencies {
implementation 'com.docusign:androidsdk:1.7.6'
implementation 'com.docusign:sdk-common:1.7.6'
implementation 'com.docusign:sdk-esign-api:1.7.6'
}
If you want to use additional components, you can check which dependencies you need to include on the Docusign SDK Components section of the Android SDK ReadMe.
Making a direct API call
Once you’ve integrated the mobile SDK frameworks, you’ll be able to use Docusign mobile SDK methods and calls in your app. The direct API call demonstrated for this example will be retrieving a user’s signature ID. To retrieve a user’s signature ID, you will need to include their account ID and user ID parameters in the request. The Java code below shows how to make that call, using a new instance of getESignApiDelegate:
DSESignApiDelegate eSignApiDelegate = Docusign.getInstance().getESignApiDelegate();
final UsersApi usersApi = eSignApiDelegate.createApiService(UsersApi.class);
if (usersApi != null) {
DSAuthenticationDelegate authDelegate = Docusign.getInstance().getAuthenticationDelegate();
final DSUser user = authDelegate.getLoggedInUser(getApplicationContext());
If you want to make other direct API calls, you can check the SDK references for Android and iOS to see which parameters must be passed to make a request to the endpoint you want to use.
To continue this example: Once you have retrieved the account ID and user ID, you can use this information to request the signature ID, shown with the Java code below:
eSignApiDelegate.invoke(new DSESignApiListener() {
@Override
public <T> void onSuccess(T response) {
if (response instanceof UserSignaturesInformation) {
UserSignature userSignature = ((UserSignaturesInformation) response).getUserSignatures().get(0);
Log.d("makeDirectApiCall", "Signature Id: " + userSignature.getSignatureId());
}
}
}, () -> usersApi.userSignaturesGetUserSignatures(user.getAccountId(), user.getUserId(), "signature"));
Upon success, the app will return the signature ID:
D/makeDirectApiCall: Signature Id: 7faf2365-xxxx-xxxx-xxxx-1cbd9e6dff30
Now that you’ve learned how this example works, feel free to try it out on your own. You can find the how-to articles for this code example in Android and iOS in the Developer Center. You can see how to incorporate our other mobile code examples in your app under Next steps in the how-to guide. You can also check out other new additions to the Android SDK in our What’s new in the Docusign Android SDK blog post.
Additional resources
Raileen Del Rosario has been with Docusign since 2022. As a Programmer Writer on the Developer Content team, she writes content and code to help developers learn how to use Docusign APIs.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
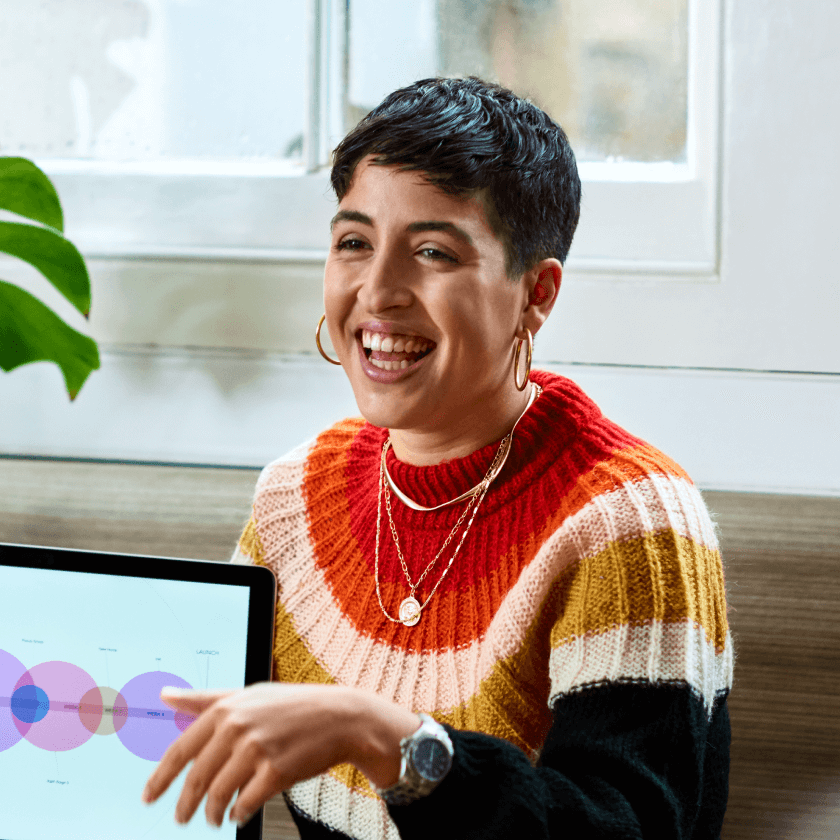