Common API Tasks🐈: Use a transactionId to find the envelope you created
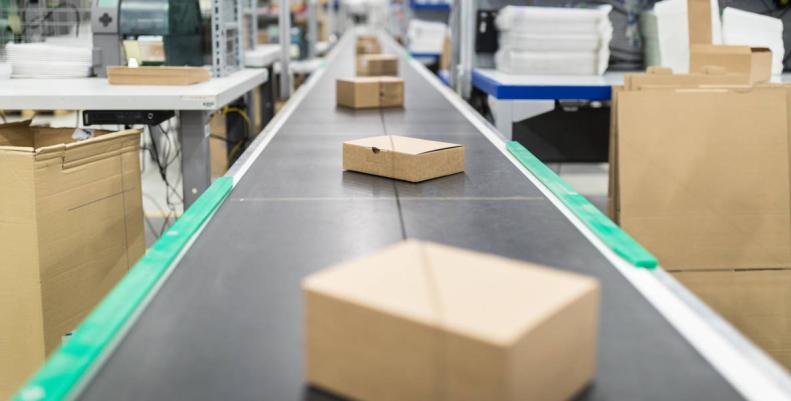
Welcome to a stellar new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the DocuSign Developer Blog.
In this post, I’m going to show you a useful technique to locate envelopes that your integration created in situations where you didn’t receive a response from the DocuSign eSignature REST API. (Typically, that response includes the envelopeId, which is the unique identifier primarily used to locate an envelope in the DocuSign platform.)
Why, you might ask, would you not have the API response available to your application? I can think of several reasons this may occur. First, in a situation where the internet connection is slow or unavailable, you may be able to create the envelope, but you will not be able to receive a timely response to your API call. Second, when you create an envelope containing a large document, the processing of the API may take longer than usual, and your application may choose to make the call asynchronously instead of waiting for the response from the server. Lastly, you may run code that creates a large number of envelopes one after the other, and you may wish to make it more efficient, by not waiting for a response for each envelope before you create the next one. In that case, your application will also not have the information about the envelopeId readily available to be used later.
This is where the transactionId property comes in handy. This string is one that your application chooses. You can use any string you wish. I would recommend using something unique within the system that your application manages (using GUIDs is a common way to ensure uniqueness). These special identifiers are added to the createEnvelope call that your application makes such that you can later use them to get the information about that envelope. You do that by using the listStatus endpoint and providing one or more transactionIDs to locate the envelope(s) you previously created. Note that the transactionIDs are stored in the system for seven days only and do not have to be unique across accounts (meaning that if you and I use different accounts, we can use the same transactionId without an issue.)
Note: Before I show you how to do this in code, I want to warn you about the polling technique I use here. It is not the intention of this blog post to suggest you should use polling to query the status of your envelope. You should use a Connect webhook instead and get information about the envelope without having to poll DocuSign for the latest status of the envelope. The purpose of this blog post is just to show you how you can utilize a transactionId of your choosing to find the envelope you created later.
The code snippets here show two things. First, I will briefly show how to add a transactionId when you create an envelope. The little snippet doesn’t show you all the code to create an envelope; see How to request a signature by email for complete code to create an envelope. Second, I will show you how to find the envelope that was created with the transactionId previously.
C#
DocuSignClient docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
// Create an envelope
EnvelopeDefinition envelope = new EnvelopeDefinition();
envelope.TransactionId = "test-from-CAT-blog";
// ... Rest of the code to create an envelope...
// Find the envelope and its envelopeId
EnvelopesApi envelopesApi = new EnvelopesApi(docuSignClient);
EnvelopeIdsRequest envelopeIdsRequest = new EnvelopeIdsRequest();
EnvelopesApi.ListStatusOptions options = new EnvelopesApi.ListStatusOptions();
options.transactionIds = "test-from-CAT-blog"; // Use a comma if you need more than one
EnvelopesInformation envelopesInformation = envelopesApi.ListStatus(accountId, envelopeIdsRequest, options);
string myEnvelopeId = envelopesInformation.Envelopes[0].EnvelopeId;
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
// Create an envelope
EnvelopeDefinition envelope = new EnvelopeDefinition();
envelope.setTransactionId("test-from-CAT-blog");
// ... Rest of the code to create an envelope...
// Find the envelope and its envelopeId
EnvelopesApi envelopesApi = new EnvelopesApi(config);
EnvelopeIdsRequest envelopeIdsRequest = new EnvelopeIdsRequest();
EnvelopesApi.ListStatusOptions options = envelopesApi.new ListStatusOptions();
options.setTransactionIds("test-from-CAT-blog"); // Use a comma if you need more than one
EnvelopesInformation envelopesInformation = envelopesApi.ListStatus(accountId, envelopeIdsRequest, options);
string myEnvelopeId = envelopesInformation.getEnvelopes().get(0).getEnvelopeId();
Node.js
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
// Create an envelope
EnvelopeDefinition envelope = new EnvelopeDefinition();
envelope.transactionId = 'test-from-CAT-blog';
// ... Rest of the code to create an envelope...
// Find the envelope and its envelopeId
let envelopesApi = new EnvelopesApi(dsApiClient);
let envelopesInformation = envelopesApi.listStatus(accountId, {'transactionIds' : 'test-from-CAT-blog'}); // Use a comma if you need more than one
let myEnvelopeId = envelopesInformation.envelopes[0].envelopeId;
PHP
$api_client = new \DocuSign\eSign\client\ApiClient($base_path);
$config = new \DocuSign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
# Create an envelope
$envelope = new EnvelopeDefinition();
$envelope->setTransactionId('test-from-CAT-blog');
# ... Rest of the code to create an envelope...
# Find the envelope and its envelopeId
$envelopes_api = new \DocuSign\eSign\Api\EnvelopesApi($api_client);
$envelope_ids_request = new \DocuSign\eSign\Model\EnvelopeIdsRequest();
$options = new ListStatusOptions();
$options->setTransactionIds('test-from-CAT-blog'); # Use a comma if you need more than one
$envelopes_information = $envelopesApi->ListStatus($account_id, $envelope_ids_request, $options);
$my_envelope_id = envelopesInformation->getEnvelopes()[0]->getEnvelopeId();
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
# Create an envelope
envelope = new EnvelopeDefinition();
envelope.transactionId = 'test-from-CAT-blog'
# ... Rest of the code to create an envelope...
# Find the envelope and its envelopeId
envelopes_api = EnvelopesApi(api_client)
envelope_ids_request = EnvelopeIdsRequest()
options = ListStatusOptions()
options.transaction_ids = 'test-from-CAT-blog' # Use a comma if you need more than one
envelopes_information = envelopes_api.list_status(account_id, envelope_ids_request, options)
my_envelope_id = envelopes_information.envelopes[0].envelope_id
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
# Create an envelope
envelope = new EnvelopeDefinition();
envelope.transactionId = 'test-from-CAT-blog'
# ... Rest of the code to create an envelope...
# Find the envelope and its envelopeId
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
envelope_ids_request = DocuSign_eSign::EnvelopeIdsRequest.new
options = DocuSign_eSign::ListStatusOptions.new
options.transaction_ids = 'test-from-CAT-blog' # Use a comma if you need more than one
envelopes_information = envelopes_api.list_status(account_id, envelope_ids_request, options)
my_envelope_id = envelopes_information.envelopes[0].envelope_id