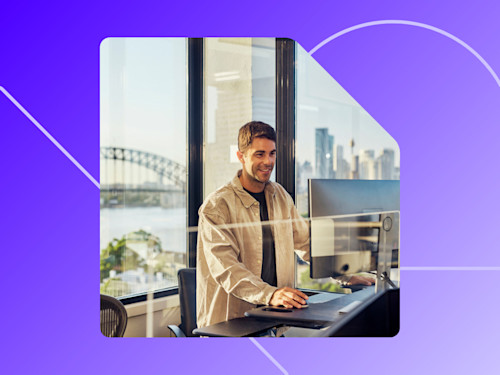
15 minutes to better UX: Enhancing embedded signing with focused view
Discover focused view, a new way to embed the Docusign experience into your app with a streamlined UI.
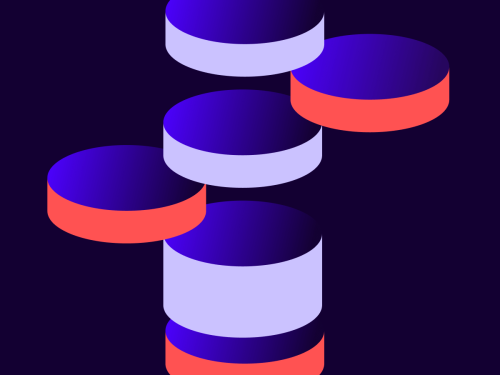
If you are a developer or a product manager keen on embedding eSignature agreements into your websites or apps seamlessly, this blog post is your guide. Docusign offers eSignature REST APIs for this purpose, allowing you to generate signing links. We've further simplified the process with a JavaScript snippet that works in tandem with these APIs. This snippet enables a feature known as focused view, allowing you to tailor the user interface. Read on to find out how this can enhance user experience and boost conversion rates.
Traditional approaches to embedded signing with eSignature REST API
Traditional methods for implementing embedded signing involve:
Creating an envelope containing an agreement
Generating a signing URL for the recipient
Presenting the agreement by:
Redirecting to the signing URL
Rendering the signing URL in an iframe
Challenges with presenting the agreement:
Both methods can create friction for users:
Redirecting can confuse some users, impacting conversion rates
Embedding in an iframe can lead to a web-page-within-a-web-page experience that can be unintuitive
Augmenting the experience: Docusign JS and focused view
To enhance user experience and improve conversions, we introduce Docusign JS and focused view.
What is Docusign JS?
Docusign JS is a JavaScript snippet that renders the Docusign agreement natively within your site. You simply initialize the script and pass in the signing URL generated by the eSignature REST API (code snippet to follow).
What is focused view?
Focused view offers a minimalistic wrapper around the agreement. It presents only the agreement and a navigational button, giving it a native feel. With Docusign JS, you can also customize the UI elements to better blend with your site.
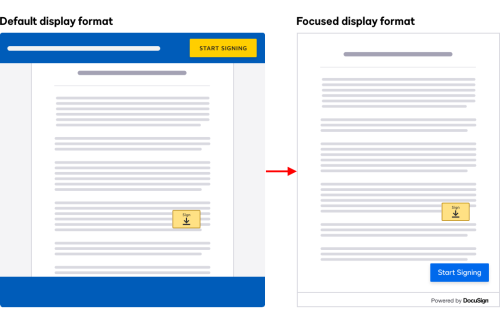
How to implement focused view in less than 15 minutes
For most use cases, implementing focused view can be done in three simple steps:
Update the API implementation to create an envelope and signing URL for the recipient
Utilize the returned signing URL with Docusign JS
Embed the agreement into your HTML and fine-tune the user experience
Let’s dive into each step.
Step 1: Update the API implementation for envelope creation and signing URL
If you haven’t set up the API for envelope creation and signing URL yet, you’ll need to use the createEnvelope POST request and the createRecipientView POST request).
Here’s a sample JSON body for the createRecipientView POST request:
{
"returnUrl": "http://my.return.url.here.com",
"authenticationMethod": "my_authentication_method",
"email": "'"${SIGNER_EMAIL}"'",
"userName": "'"${SIGNER_NAME}"'",
"clientUserId": random_client_user_id,
"frameAncestors": ["https://my.site.com", "https://apps-d.docusign.com"],
"messageOrigins": ["https://apps-d.docusign.com"]
}
Notes:
The
frameAncestors
property is an array which must include your site’s URL along with https://apps-d.docusign.com for the developer environment or https://apps.docusign.com for the production environment. Additionally, for local development and testing, http://localhost can be included. However, for embedding in production environments, HTTPS must be enabled on your site (for example, https://my.site.com).The
messageOrigins
property must include https://apps-d.docusign.com for the developer environment and https://apps.docusign.com for the production environment.Docusign JS offers event handlers corresponding to the states of
ready
andsessionEnd
. They handle events such assigning_complete
so that you do not have to send a message to the parent from the return URL.
Step 2: Utilize the returned URL with Docusign JS
The loadDocuSign
function uses your application’s integration key to identify itself.
<script>
window.Docusign.loadDocuSign("your_integration_key_guid_here")
.then((docusign) => {
const signing = docusign.signing({...});
...
})
.catch((ex) => {
// Any configuration or API limits will be caught here
});
</script>
Step 3: Embed agreement in HTML and fine-tune UX
Here is a sample HTML setup that uses a div
to mount the signing URL. The agreement is designed to occupy a height of 400px by default and the entire width of the div
where it is embedded. We recommend overriding these defaults by defining the height
and width
of the div
appropriately to ensure the agreement feels native to your website. In the example below, the div
height
is set to 800px while the width
is set to 100%.
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Signing</title>
<style>
html,
body {
padding: 0;
margin: 0;
font: 13px Helvetica, Arial, sans-serif;
}
// container styles
@media (max-width: 768px){
.docusign-agreement-container {
width: 50%
}
}
@media (min-width: 769px) (max-width: 1024px) {
.docusign-agreement-container {
width: 70%
}
}
@media (min-width: 1025px) {
.docusign-agreement-container {
width: 100%
}
}
</style>
</head>
<body>
<header><h1> Demo</h1></header>
<div class="docusign-agreement-container" id="docusign"></div>
<script src="script.js"></script>
</body>
</html>
<meta charset="utf-8"><title>Signing</title><style>
html,
body {
padding: 0;
margin: 0;
font: 13px Helvetica, Arial, sans-serif;
}
// container styles
@media (max-width: 768px){
.docusign-agreement-container {
width: 50%
}
}
@media (min-width: 769px) (max-width: 1024px) {
.docusign-agreement-container {
width: 70%
}
}
@media (min-width: 1025px) {
.docusign-agreement-container {
width: 100%
}
}
</style><header><h1> Demo</h1></header><div class="docusign-agreement-container" id="docusign"></div>
<script src="script.js"></script>
To fine-tune the UX, you can adjust styles, button text, and other elements to make the embedded agreement mesh seamlessly with your application.
Here’s how you can customize the UI with JavaScript:
script.js
<script src="https://js-d.docusign.com/bundle.js"></script>
<!-- when this script is loaded, Docusign is added to the window -->
<!-- use src="https://js.docusign.com/bundle.js" for production -->
<script>
window.Docusign.loadDocuSign('INTEGRATION_KEY')
.then((docusign) => {
const signing = docusign.signing({
url:'...Signing URL from the API...',
displayFormat:'focused',
style:{
/** High-level variables that mirror our existing branding APIs. Reusing the branding name here for familiarity. */
branding:{
primaryButton:{
/** Background color of primary button */
backgroundColor:'#333',
/** Text color of primary button */
color:'#fff',
}
},
/** High-level components we allow specific overrides for */
signingNavigationButton:{
finishText:'Custom Button Text',
/** 'bottom-left'|'bottom-center'|'bottom-right', default: bottom-right */
position:'bottom-left'
}
}
});
/** Event handlers **/
signing.on('ready' , (event) => {
console.log('UI is rendered');
});
signing.on('sessionEnd',(event) => {
/** The event here denotes what caused the sessionEnd to trigger, such as signing_complete, ttl_expired etc../
console.log('sessionend', event);
});
signing.mount('#agreement');
})
.catch((ex) => {
// Any configuration or API limits will be caught here
});
</script>
Additional recommendations during implementation
Improve performance
Load the script asynchronously for better performance, even before the signing URL is initialized into the loadDocuSign
function.
Trigger next steps with event handlers
Event handlers such as ready
(tracks that the UI has successfully loaded) and sessionEnd
(indicates the end of signing) can be used for more streamlined workflows. Events that can trigger sessionEnd
include :
'signing_complete'
'cancel'
'decline'
'exception'
'fax_pending'
'session_timeout'
'ttl_expired'
'Viewing_complete'
Reduce steps
You can turn off the Electronic Records and Signature Disclosure dialog by setting useDisclosure
to false
in the createEnvelope
API method. (Make sure this is approved by your legal team.)
Conclusion
Implementing focused view in your embedded signing experience is not only straightforward, but also highly beneficial, both for developers and their end users. By following the steps outlined in this guide, you can integrate this feature in less than 15 minutes for most use cases.
Whether you're a seasoned developer or just starting your journey in the world of digital transactions, these insights will help you build a more efficient, secure, and intuitive signing experience for your users.
Thank you for reading, and as always, happy coding!
Additional resources
Palash Agrawal currently serves as a Product Manager for the Docusign eSignature product. Prior, Palash worked as a tech entrepreneur, built products from scratch, and scaled them to serve millions of users.
Palash holds an MBA from MIT Sloan School of Management and a B.Tech from the Indian Institute of Technology, Bombay.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
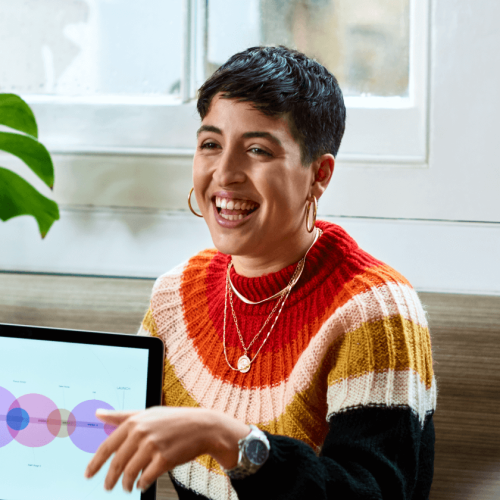