Common API Tasks 🐈: Change email subject and body for each recipient
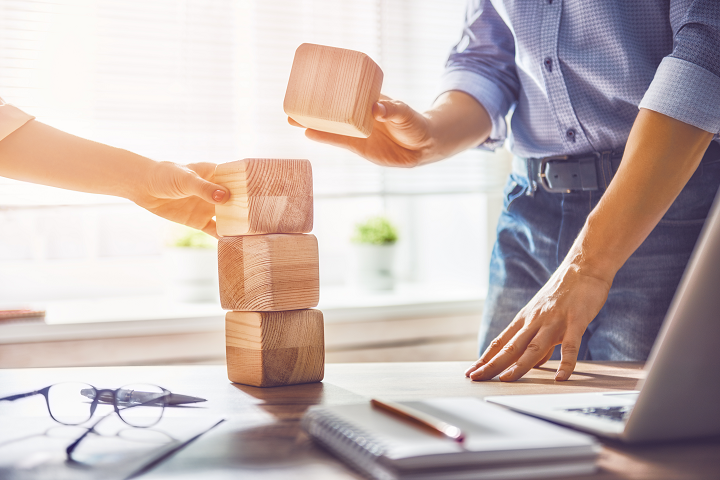
This post begins a new blog series called Common API Tasks, focusing on small useful API tasks. In each post, I'll detail a specific activity that is useful to many Docusign API developers and I'll show you how to complete that task easily. For this first post in the series, I chose customizing the content of the email message (both the subject line as well as the body of the message) that is sent to remote recipients when an envelope is routed for signature. I will even show you how to have custom emails for each of the recipients in your envelope.
As most of you probably know, you can change the subject line of the email as well as the body of the email (what we call the blurb) for the entire envelope. You do that using the EnvelopeDefinition object. Here's how to modify the email subject and body of all recipients in an envelope:
C#
// create the envelope definition
EnvelopeDefinition env = new EnvelopeDefinition();
env.EmailSubject = "This is the envelope email subject";
env.EmailBlurb = "This is the envelope email body";
Java
// create the envelope definition
EnvelopeDefinition env = new EnvelopeDefinition();
env.setEmailSubject("This is the envelope email subject");
env.setEmailBlurb("This is the envelope email body");
Node.js
// create the envelope definition
let env = new docusign.EnvelopeDefinition();
env.emailSubject = 'This is the envelope email subject';
env.emailBlurb = 'This is the envelope email body';
PHP
# create the envelope definition
$env = new \Docusign\eSign\Model\EnvelopeDefinition([
'email_subject' => 'This is the envelope email subject',
'email_blurb' => 'This is the envelope email body']);
Python
# create the envelope definition
env = EnvelopeDefinition(
email_subject = 'This is the envelope email subject',
email_blurb = 'This is the envelope email body')
Ruby
# create the envelope definition
env = DocuSign_eSign::EnvelopeDefinition.new
env.email_subject = 'This is the envelope email subject'
env.email_blurb = 'This is the envelope email body'
While this is useful, there are cases where you may want to customize the message for each of the recipients in an envelope. You can actually do that with the Docusign eSignature API!
When you add the recipients to your envelope, each recipient includes an optional RecipientEmailNotification object. This object includes the EmailSubject and EmailBody properties, which can be used to customize the email that is sent to the specified recipient only.
(EmailBody, which was previously called blurb, is an additional text that is added at the beginning of the email message. It doesn’t refer to the entire body of the email message). Here is how to customize the subject and body for a specific recipient in various languages:
C#
// create a Signer recipient to sign the document, identified by name and email
// add an EmailNotification object with a custom email subject and email body
Signer signer1 = new Signer {
Email = "inbar@example.com",
Name = "Inbar Gazit",
RecipientId = "1",
RoutingOrder = "1",
EmailNotification = new RecipientEmailNotification {
EmailSubject = "Custom email subject for signer 1",
EmailBody = "Custom email body for signer 1" }
};
Java
// create a Signer recipient to sign the document, identified by name and email
// add an EmailNotification object with a custom email subject and email body
Signer signer1 = new Signer ();
signer1.setEmail("inbar@example.com");
signer1.setName("Inbar Gazit");
signer1.setRecipientId("1");
signer1.setRoutingOrder("1");
RecipientEmailNotification ren = new RecipientEmailNotification();
ren.setEmailSubject("Custom email subject for signer 1");
ren.setEmailBody("Custom email body for signer 1");
signer1.setEmailNotification(ren);
Node.js
// create a Signer recipient to sign the document, identified by name and email
// add an EmailNotification object with a custom email subject and email body
let signer1 = docusign.Signer.constructFromObject( {
email : 'inbar@example.com',
name : 'Inbar Gazit',
recipientId : '1',
routingOrder : '1',
emailNotification = docusign.RecipientEmailNotification.constructFromObject (
{ emailSubject : 'Custom email subject for signer 1',
emailBody : 'Custom email body for signer 1' })
});
PHP
# create a Signer recipient to sign the document, identified by name and email
# add an EmailNotification object with a custom email subject and email body
$signer1 = new \Docusign\eSign\Model\Signer([
'email' => 'inbar@example.com',
'name' => 'Inbar Gazit',
'recipient_id' => '1',
'routing_order' => '1',
'email_notification' => new \Docusign\eSign\Model\RecipientEmailNotification([
'email_subject' => 'Custom email subject for signer 1',
'email_body' => 'Custom email body for signer 1'])
]);
Python
# create a Signer recipient to sign the document, identified by name and email
# add an EmailNotification object with a custom email subject and email body
signer1 = Signer(
email = 'inbar@example.com',
name = 'Inbar Gazit',
recipient_id = '1',
routing_order = '1',
email_notification = RecipientEmailNotification(
email_subject = 'Custom email subject for signer 1',
email_body = 'Custom email body for signer 1')
)
Ruby
# create a Signer recipient to sign the document, identified by name and email
# add an EmailNotification object with a custom email subject and email body
signer1 = DocuSign_eSign::Signer.new
signer1.email = 'inbar@example.com'
signer1.name = 'Inbar Gazit'
signer1.recipient_id = '1'
signer1.routing_order = '1'
ren = DocuSign_eSign::RecipientEmailNotification.new
ren.email_subject = 'Custom email subject for signer 1'
ren.email_body = 'Custom email body for signer 1'
signer1.email_notification = ren
If you have multiple recipients, you can set a custom email subject and body for each of them separately using the code above. Recipients whose email subject and body are not specified will inherit the overall envelope email subject and body, so there’s no need to specify it if you wish to just use the same one that was set for the envelope.
I hope you found this useful! I would love to get your feedback about this blog post. Feel free to email me at inbar.gazit@docusign.com with any questions.
In the next blog post in this series, I’ll show how you can use the eSignature API to retrieve envelope data. Stay tuned...