From the Trenches: Tabs with the Apex Toolkit, part 2
The Docusign Apex Toolkit is a set of predefined methods, classes, and utilities that enable Apex developers to incorporate Docusign functionality in their Salesforce integrations. It is used for programmatically creating and sending Docusign envelopes from within Salesforce.
The Apex Toolkit lets you define Docusign Tabs (also called tags or fields) and apply them to documents inside an envelope. In this post I’ll provide Apex code to create two checkbox tabs, a merge field text tab that pulls data from a Salesforce record, and a dropdown tab. Part one of the series has example code to create a payment, a radio button, and a formula tab.
The Checkbox Tab
Here, I’ll demonstrate how to define two checkboxes nested inside a group. When you define more than one checkbox using the standard web console, the result is an included group label. The example below follows the same format and includes two checkboxes in a group.
UI example:
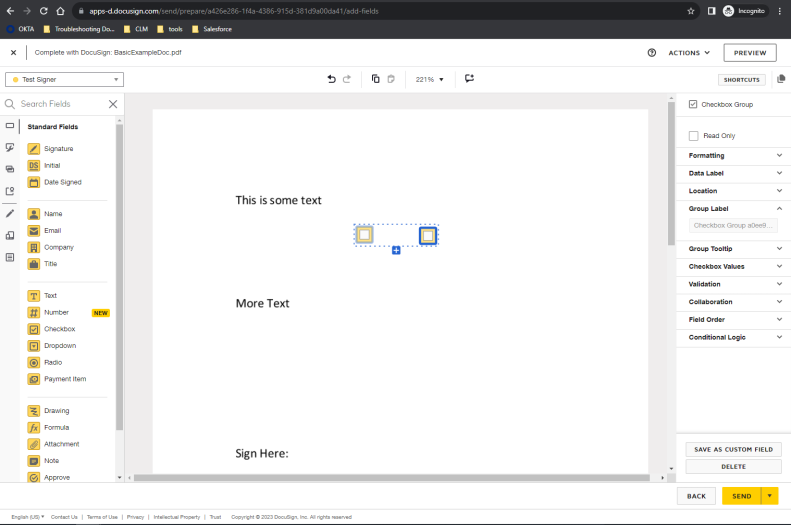
The Apex code for creating checkboxes:
String groupLabels = 'CheckboxGroup';
integer column1X = 100;
integer column2x = 130;
integer yPosition = 200;
dfsle.Tab tabgroup = new dfsle.TabGroup()
.withScope('envelope')
.withDataLabel(groupLabels)
.withPosition(
new dfsle.Tab.Position(
1, // The document to use
1, // Page number on the document
null, // X position
null, // Y position
null, // Default width
null)); // Default height
dfsle.Tab yesTab = new dfsle.CheckboxTab()
.withPosition(
new dfsle.Tab.Position(
1, // The document to use
1, // Page number on the document
column1X, // X position
yPosition, // Y position
null, // Default width
null)) // Default height
.withName('yesLabel')
.withGroupDataLabels(new List<String> {groupLabels})
.withDataLabel('yesLabel');
dfsle.Tab noTab = new dfsle.CheckboxTab()
.withPosition(
new dfsle.Tab.Position(
1, // The document to use
1, // Page number on the document
column2X, // X position
yPosition, // Y position
null, // Default width
null
)
) // Default height
.withName('noLabel')
.withGroupDataLabels(new List<String> {groupLabels})
.withDataLabel('noLabel');
dfsle.Recipient myRecipient = dfsle.Recipient.fromSource(
myContact.Name, // Recipient name
myContact.Email, // Recipient email
null, // Optional phone number
'Signer 1', // Signer role
null)
.withTabs(new List<dfsle.Tab> { // Associate the tabs with this recipient
tabGroup, yesTab, noTab
});
//add tab to recipient
myRecipient = myRecipient.withTabs(new List<dfsle.Tab> { tabGroup, yesTab, noTab});
The checkboxes produced by this code:
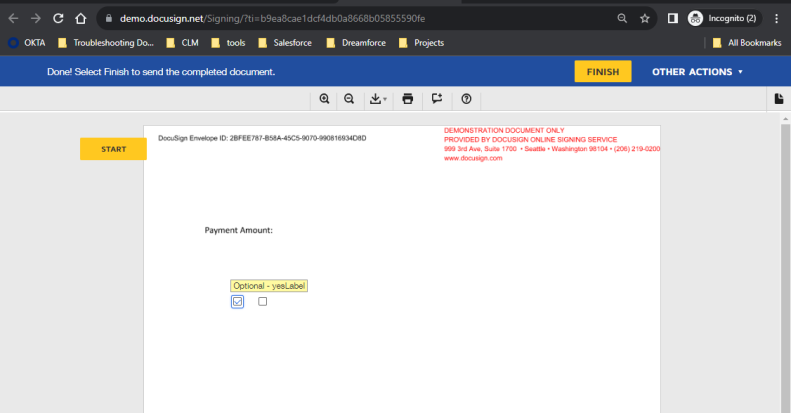
Merge Field Text Tab
The next tab will be a standard text tab that pulls in data from a Salesforce record using a merge field. Specifically, the merge field will pull in the description from a Salesforce opportunity and place the value of that field into a text tab. If the field is updated when signing, the update will be written back to the Salesforce record.
Apex code:
dfsle.Tab.MergeField OppDescptionMergeField = new dfsle.Tab.MergeField(
'opportunity.description', // The path to the Salesforce merge field
null, // The extended path to the Salesforce merge field
null, // Specifies the row number in a Salesforce table that the merge field value corresponds to
true, // When true, data entered into the merge field during Signing will update the mapped Salesforce field.
false); // When true, the sender cannot modify the value of the mergeField tab during the sending process.
//Add a text tab
dfsle.Tab t = new dfsle.TextTab()
.withMergeField(OppDescptionMergeField) // Map tab to the mergeField
.withReadOnly(false) // true = read-only or locked
// Set the tab on the anchor string Other, 40 pixels right and -50 pixels up.
.withAnchor(new dfsle.Tab.Anchor( 'Other', true, true, null, true, true, 'pixels', 80, -50))
.withDataLabel('Description');
// Add the tab to the recipient called myRecipient
myRecipient = myRecipient.withTabs(new List<dfsle.Tab> {t});
// Add Recipient to the envelope
myEnvelope = myEnvelope.withRecipients(new List<dfsle.Recipient> { myRecipient });
The end result of the code:
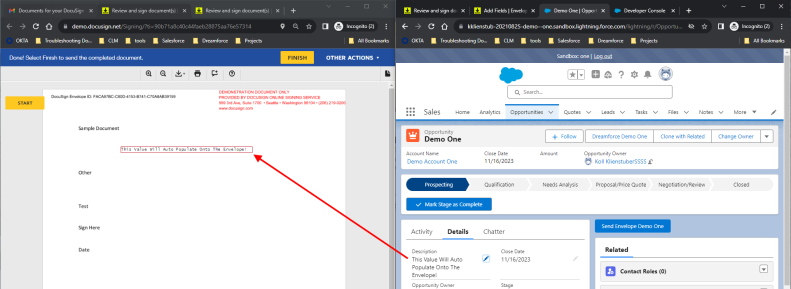
The Dropdown Tab
The last tab I’ll demonstrate is using the ListTab class.The end result is a dropdown field with three values to select from.
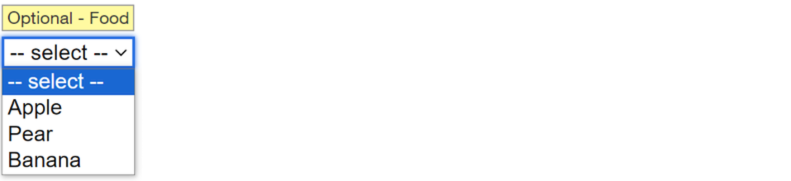
Apex code:
//https://developers.docusign.com/docs/salesforce/apex-toolkit-reference/listtab.html
List<dfsle.ListTab.Item> items = new List<dfsle.ListTab.Item>{
new dfsle.ListTab.Item('Apple',null,false),// The parameters for each dropdown are label, value, and default selected.
new dfsle.ListTab.Item('Pear',null,false),
new dfsle.ListTab.Item('Banana',null,false)
};
dfsle.Tab listTab = new dfsle.ListTab()
.withItems(items)
.withRequired(false)
.withDataLabel('Food')
.withPosition(new dfsle.Tab.Position(
1, // The document to use
1, // Page number on the document
300, // X position
300, // Y position
null, // Default width
null)); // Default height
myRecipient = myRecipient.withTabs(new List<dfsle.Tab> {listTab});
Additional tab examples of InitialHereTab, SignHereTab, and DateSignedTab are found in our documentation.