Common API Tasks🐈: View a complete envelope from your app
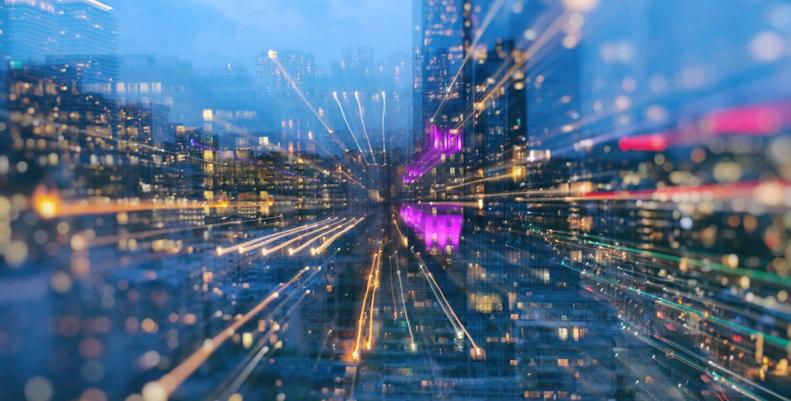
Welcome to an incredible new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the DocuSign Developer Blog.
In this edition I’m going to show you a simple thing that is frequently requested by developers, and while it’s very easy to do, it’s not often clear how to do it. As you know, when an envelope is complete, there’s a final PDF copy that represents the agreed-upon contract, the final agreement, with all the signatures and other tabs (signing elements) from all the recipients. On the Developer Center we have an article showing you how to download this PDF, as well as other related PDFs (such as the certificate of completion). That article assumes you want to download the document as a file, but what if you want to use the same DocuSign viewer that users of the DocuSign web app can use to view the complete envelope? What if you want to integrate or embed this viewer inside your own application? This can be done using the DocuSign eSignature REST API, and I’m going to show you how.
Before we get to the code snippets, here is the information you need to make this API call:
- accountId: Needed for almost all API calls, this is the GUID account ID for the DocuSign account containing the complete envelope.
- envelopeId: The unique identifier for the complete envelope.
- userName: The name of the user that sent the envelope. It can also be one of the recipients of this envelope.
- emailAddress: The email address of the user that sent the envelope. It can also be one of the recipients of this envelope.
C#
var docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(docuSignClient);
RecipientViewRequest recipientViewRequest = new RecipientViewRequest();
recipientViewRequest.UserName = userName;
recipientViewRequest.Email = emailAddress;
recipientViewRequest.ReturnUrl = "https://www.docusign.com";
// In this example, your application is responsible for authentication, and no additional authentication will be required
recipientViewRequest.AuthenticationMethod = "none";
ViewUrl viewUrl = envelopesApi.CreateRecipientView(accountId, envelopeId, recipientViewRequest);
string url = viewUrl.Url; // URL expires in 5 minutes
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
RecipientViewRequest recipientViewRequest = new RecipientViewRequest();
recipientViewRequest.setUserName(userName);
recipientViewRequest.setEmail(emailAddress);
recipientViewRequest.setReturnUrl("https://www.docusign.com");
// In this example, your application is responsible for authentication, and no additional authentication will be required
recipientViewRequest.setAuthenticationMethod("none");
ViewUrl viewUrl = envelopesApi.createRecipientView(accountId, envelopeId, recipientViewRequest);
String url = viewUrl.getUrl(); // URL expires in 5 minutes
Node
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
let recipientViewRequest = new docusign.RecipientViewRequest();
recipientViewRequest.userName = userName;
recipientViewRequest.email = emailAddress;
recipientViewRequest.returnUrl = 'https://www.docusign.com';
// In this example, your application is responsible for authentication, and no additional authentication will be required
recipientViewRequest.authenticationMethod = 'none';
let viewUrl = envelopesApi.createRecipientView(accountId, envelopeId, recipientViewRequest);
let url = viewUrl.Url; // URL expires in 5 minutes
PHP
$api_client = new \DocuSign\eSign\client\ApiClient($base_path);
$config = new \DocuSign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \DocuSign\eSign\Api\EnvelopesApi($api_client);
$recipient_view_request = new \DocuSign\eSign\Model\RecipientViewRequest();
$recipient_view_request->setUserName($user_name);
$recipient_view_request->setEmail($email_address);
$recipient_view_request->setReturnUrl('https://www.docusign.com');
# In this example, your application is responsible for authentication, and no additional authentication will be required
$recipient_view_request->setAuthenticationMethod('none');
$view_url = $envelopes_api->createRecipientView($account_id, $envelope_id, $recipient_view_request);
$url = $view_Url->getUrl(); # URL expires in 5 minutes
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
recipient_view_request = RecipientViewRequest()
recipient_view_request.user_name = user_name
recipient_view_request.email = email_address
recipient_view_request.return_url = 'https://www.docusign.com'
# In this example, your application is responsible for authentication, and no additional authentication will be required
recipient_view_request.authentication_method = 'none'
view_url = envelopes_api.create_recipient_view(account_id, envelope_id, recipient_view_request)
url = view_url.Url # URL expires in 5 minute
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
recipient_view_request = DocuSign_eSign::RecipientViewRequest.new
recipient_view_request.user_name = user_name
recipient_view_request.email = email_address
recipient_view_request.return_url = 'https://www.docusign.com'
# In this example, your application is responsible for authentication, and no additional authentication will be required
recipient_view_request.authentication_method = 'none'
view_url = envelopes_api.create_recipient_view(account_id, envelope_id, recipient_view_request)
url = view_url.Url # URL expires in 5 minute
That’s all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...