Common API Tasksđ: Find all envelopes that were created for a given PowerForm
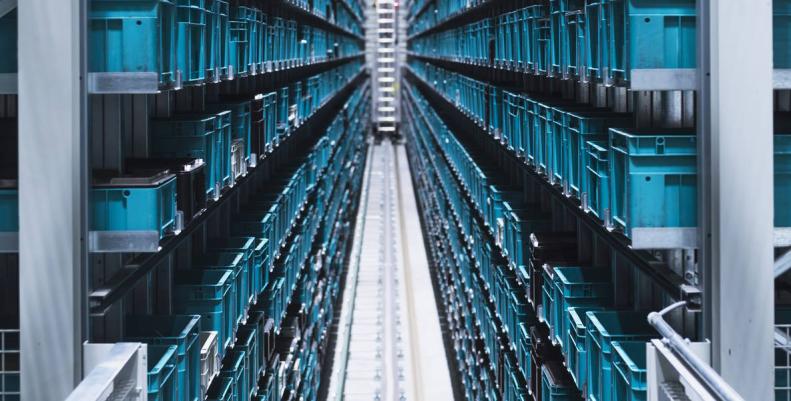
Welcome to an astounding new edition of the CATđ (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the Docusign Developer Blog.
Today weâre going to dive deeper into what you can do with PowerForms. In a previous post in this series I showed you how to use the Docusign eSignature REST API to create a PowerForm. Letâs do a quick recap of your understanding of PowerForms and what problem they solve. A PowerForm is a template that has been transformed into a custom URL. The URL can then be embedded in your website or app and be used to collect signatures using the template you already have in your account. Whenever someone selects this URL, theyâll be asked to provide their name and email address, and theyâll then be redirected to sign the document. It is important to understand that behind the scenes, the Docusign system creates an envelope from the template the same way you can do using code, by adding the details of the recipient that was provided to the PowerForm as the single signer.Â
Now, letâs say you have already created the template you need, created a PowerForm from this template, and used the URL for this PowerForm to collect signatures. As an example, maybe youâre having a birthday party and taking all your friends rock climbing. The rock climbing establishment wants each participant to provide a signed waiver, so you create a template with their document to be signed, and the PowerForm URL is then sent to your birthday guests. Now, youâre ready to go climb some rocks with your friends, but first you need to provide all the signed documents that were gathered using the PowerForm. You could do this by going to the eSignature web app, finding your PowerForm, and then looking up all the envelopes that were created from that PowerForm. Thatâs one way to do it manually. But hi, you not only have a birthday, youâre also a developer :). So you want to write some code to do that programmatically and get all the signed documents from all the envelopes that your friends signed using the PowerForm. This is what Iâm about to show you.Â
The following code snippets in our usual six coding languages show how to get the list of envelopes for a specific PowerForm (you will need the powerFormId, which is a unique GUID that identifies every PowerForm in the system) and find the names of all the recipients that signed the PowerForm. You can do other things with the envelopes, such as downloading the signed document or finding the data that the recipient entered into the envelope (by filling tabs that were set on the template). Note that I assume a simple case of a single signer.Â
C#
var docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(docuSignClient);
EnvelopesApi.ListStatusChangesOptions options = new EnvelopesApi.ListStatusChangesOptions();
options.fromDate = "01/01/2023"; // Must include a date; you can use the date you created or sent this PowerForm
options.powerformids = "e9d64c91-xxxx-xxxx-xxxx-96b043209725";
options.include = "recipients";
EnvelopesInformation envelopesInformation = envelopesApi.ListStatusChanges(accountId, options);
List<string> names = new List<string>();
foreach (Envelope env in envelopesInformation.Envelopes)
{
if (env.Status == "completed")
{
names.Add(env.Recipients.Signers[0].Name); // Assuming one recipient who is a signer
}
}
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
EnvelopesApi.ListStatusChangesOptions options = new EnvelopesApi.ListStatusChangesOptions();
options.setFromDate("01/01/2023"); // Must include a date; you can use the date you created or sent this PowerForm
options.setPowerformids("e9d64c91-xxxx-xxxx-xxxx-96b043209725");
options.setInclude("recipients");
EnvelopesInformation envelopesInformation = envelopesApi.ListStatusChanges(accountId, options);
java.util.ArrayList names = new java.util.ArrayList();
for (Envelope env : envelopesInformation.Envelopes)
{
if (env.getStatus() == "completed")
{
names.add(env.getRecipients().getSigners().get(0)); // Assuming one recipient who is a signer
}
}
Node.js
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
let options = new EnvelopesApi.ListStatusChangesOptions();
options.fromDate = '01/01/2023'; // Must include a date; you can use the date you created or sent this PowerForm
options.powerformids = 'e9d64c91-xxxx-xxxx-xxxx-96b043209725';
options.include = 'recipients';
let envelopesInformation = envelopesApi.listStatusChanges(accountId, options);
let names = [];
envelopesInformation.envelopes.foreach(env =>
if (env.status == 'completed')
{
names.push(env.recipients.signers[0].name); // Assuming one recipient who is a signer
}
);
PHP
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \Docusign\eSign\Api\EnvelopesApi($api_client);
$options = new \Docusign\eSign\Api\EnvelopesApi\ListStatusChangesOptions();
$options->setFromDate('01/01/2023'); # Must include a date; you can use the date you created or sent this PowerForm
$options->setPowerformids('e9d64c91-xxxx-xxxx-xxxx-96b043209725');
$options->setInclude('recipients');
$envelopes_information = $envelopes_api->listStatusChanges($account_id, $options);
$names = [];
foreach ($envelopes_information->getEnvelopes() as $env)
{
if ($env->getstatus() == 'completed')
{
array_push($names, $env->getRecipients()->getSigners()[0]->getName()); # Assuming one recipient who is a signer
}
}
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
options = EnvelopesApi.ListStatusChangesOptions()
options.from_date = '01/01/2023' # Must include a date; you can use the date you created or sent this PowerForm
options.powerform_ids = 'e9d64c91-xxxx-xxxx-xxxx-96b043209725'
options.include = 'recipients'
envelopes_information = envelopes_api.list_status_changes(account_id, options)
names = []
for env in envelopes_information.envelopes:
if env.status == 'completed':
names.append(env.recipients.signers[0].name) # Assuming one recipient who is a signer
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
options = EnvelopesApi.ListStatusChangesOptions.new
options.from_date = '01/01/2023' # Must include a date; you can use the date you created or sent this PowerForm
options.powerform_ids = 'e9d64c91-xxxx-xxxx-xxxx-96b043209725'
options.include = 'recipients'
envelopes_information = envelopes_api.list_status_changes(account_id, options)
names = []
envelopes_information.envelopes.each do |env|
if env.status == 'completed'
names.push(env.recipients.signers[0].name) # Assuming one recipient who is a signer
end
Thatâs all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
- Docusign Developer Center
- @DocuSignAPI on Twitter
- Docusign for Developers on LinkedIn
- Docusign for Developers on YouTube
- DocuSignAPI on Twitch
- Docusign Developer Newsletter
Â