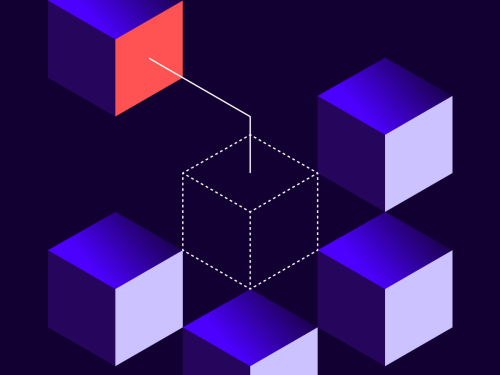
Common API Tasksš: Customize your envelopes with pre-fill fields
See how to use the eSignature REST API to include senderās pre-fill fields in your envelopes.
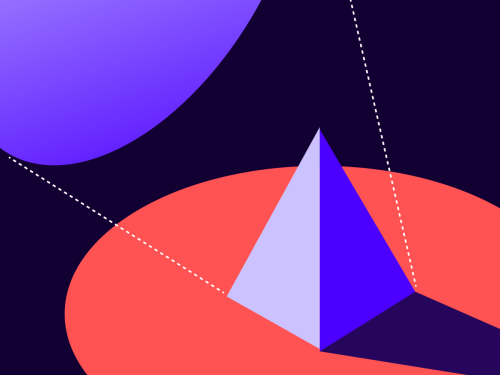
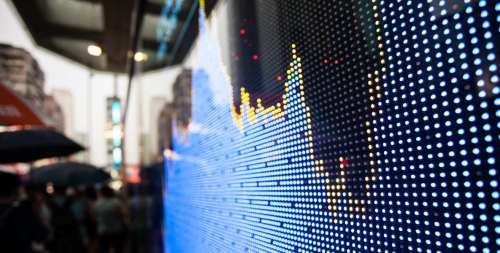
Welcome to another edition of the CATš (Common API Tasks) blog. This blog series is about giving you all you need to complete small, specific, SDK-supported tasks using one of our APIs.Ā
You can find all articles in this series on the Docusign developer blog.Ā
For this 20th installment (Iām not really keeping score, but wow, 20 issues so far!) I have a relatively new feature to tell you about, and of course, show you how to implement programmatically from the app youāre building.Ā
Imagine that you want to send a document for signature and you need to change some data in the document from time to time; say, the price on a quote. The rest of the document is always going to be the same. Instead of modifying the document every time before you send the document out, you can have the price as a field that you can change yourself.Ā
In the past, you would do that by adding yourself as a recipient of the envelope, adding a text tab for yourself to fill, and then signing it first, and only then would it go out to the real recipients with your value filled in. However, this was not an ideal experience. First, you need to act on an envelope (fill the tabs) for which you donāt necessarily want to be a recipient. You are the senderāthe person requesting signatureānot a signer.Ā
Second, you cannot modify the information once you sign the envelope. If you made a mistake, you would need to void the first envelope and then send a new envelope.Ā
These shortcomings are resolved with Docusign pre-fill fields. Pre-fill fields (also called senderās tabs) are just like any other tabs: they show on the documents in the envelopes. The big differences are that they are not associated with any of the recipients and that their value can only be set by the sender: the person or program that initiated the envelope. The fields can be added in the same UI tagger that is part of the Docusign web app when sending an envelope. In addition to textboxes, you can also use pre-fill checkboxes and radio buttons. The value and the fields can be modified at any time before any recipient acts on the envelope. (However, if the envelope has already been sent and is no longer in draft mode, you will have to initiate a correction to update the value.)Ā
Note: This feature is enabled in all developer accounts, but when it comes to production accounts, you will have to check with your account manager to see if itās included with your plan.
The sample code shows adding a pre-fill textbox and setting its value. These code snippets assume you already have an envelope created and you have obtained the envelopeId (the GUID that identifies your envelope).Ā
C#
// You need to obtain an access token using your chosen authentication flow
var apiClient = new ApiClient(basePath);
apiClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
PrefillTabs prefillTabs = new PrefillTabs();
prefillTabs.TextTabs = new List<text>();
prefillTabs.TextTabs.Add(new Text { PageNumber = "1", DocumentId = "1", Value = "MyValue" });
Tabs tabs = new Tabs();
tabs.PrefillTabs = prefillTabs;
envelopesApi.CreateDocumentTabs(accountId, envelopeId, "1", tabs);
</text>
Java
// You need to obtain an access token using your chosen authentication flow
Configuration config = new Configuration(new ApiClient(basePath));
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(config);
PrefillTabs prefillTabs = new PrefillTabs();
Text textTab1 = new Text();
textTab1.setPageNumber("1");
textTab1.setDocumentId("1");
textTab1.setValue("MyValue");
Text[] textTabs = { textTab1 };
prefillTabs.setTextTabs(Arrays.asList(textTabs));
Tabs tabs = new Tabs();
tabs.setPrefillTabs(prefillTabs);
envelopesApi.CreateDocumentTabs(accountId, envelopeId, "1", tabs);
Node.js
// You need to obtain an access token using your chosen authentication flow
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
let prefillTabs = {
'prefillTabs': {
'textTabs': [
{
'value': 'MyValue',
'pageNumber': '1',
'documentId': '1',
}]}};
envelopesApi.createDocumentTabs(accountId, envelopeId, 1, {tabs: prefillTabs});
PHP
# You need to obtain an access token using your chosen authentication flow
$api_client = new \Docusign\eSign\client\ApiClient($base_path);
$config = new \Docusign\eSign\Model\Configuration($api_client);
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \Docusign\Api\EnvelopesApi($config);
$prefill_tabs = new \Docusign\eSign\Model\PrefillTabs();
$text_tab1 = new Text();
$text_tab1->setPageNumber('1');
$text_tab1->setDocumentId('1');
$text_tab1->setValue('MyValue');
$prefill_tabs->setTextTabs([]);
array_push($prefill_tabs->getTextTabs(), $text_tab1);
$tabs = new \Docusign\eSign\Model\Tabs();
$tabs->setPrefillTabs($prefill_tabs);
$envelopes_api->createDocumentTabs($account_id, $envelope_id, '1', $tabs);
Python
# You need to obtain an access token using your chosen authentication flow
api_client = ApiClient()
api_client.host = base_path
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
prefill_tabs = PrefillTabs()
text_tab1 =Text()
text_tab1.page_number = '1'
text_tab1.document_id = '1'
text_tab1.value = 'MyValue'
prefill_tabs.text_tabs = []
prefill_tabs.text_tabs.insert(text_tab1)
tabs = Tabs()
tabs.prefill_tabs = prefill_tabs
envelopes_api.create_document_tabs(account_id, '1', envelope_id, tabs=tabs)
Ruby
# You need to obtain an access token using your chosen authentication flow
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
prefill_tabs = DocuSign_eSign::PrefillTabs.new
text_tab1 =DocuSign_eSign::Text.new
text_tab1.page_number = '1'
text_tab1.document_id = '1'
text_tab1.value = 'MyValue'
prefill_tabs.text_tabs = []
prefill_tabs.text_tabs.push(text_tab1)
tabs = DocuSign_eSign::Tabs.new
tabs.prefill_tabs = prefill_tabs
envelopes_api.create_document_tabs(account_id, '1', envelope_id, tabs)
I hope you found this useful. As usual, if you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...
Additional resources
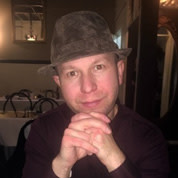
Inbar Gazit has been with Docusign since 2013 in various engineering roles. Since 2019 he has focused on developer content. Inbar works on code examples including the launchers, available on GitHub in eight languages, and helps build sample apps showcasing the various Docusign APIs. He is also active on StackOverflow, answering your questions. Inbar can be reached at inbar.gazit@docusign.com.
Related posts
Discover what's new with Docusign IAM or start with eSignature for free
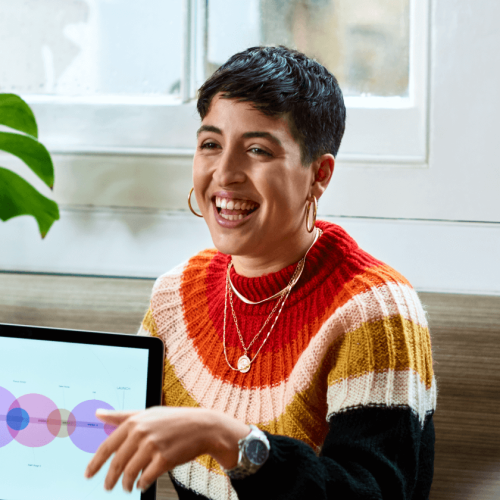