Common API Tasks🐈: Correct an envelope from your app
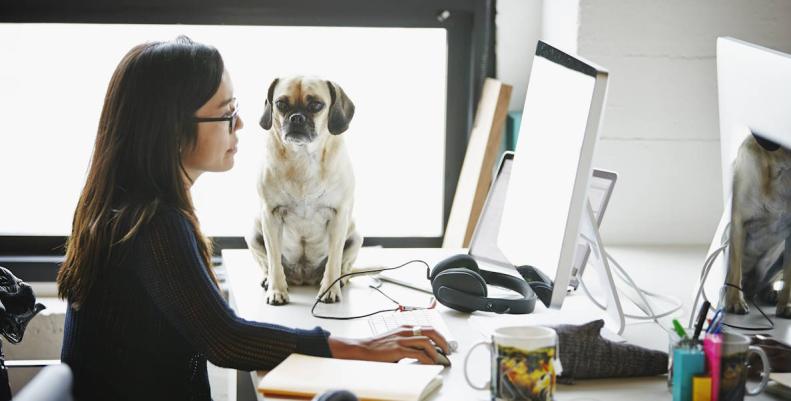
Welcome to a masterful new edition of the CAT🐈 (Common API Tasks) blog series. The CAT blogs provide all you need to complete small, specific, SDK-supported tasks using one of our APIs. You can find all articles in this series on the DocuSign Developer Blog.
Ever made a mistake? I’m sure you have. We all do. The trick is to learn from our mistakes and correct them. When you send an envelope, you (or your customers) may make mistakes—such as putting the wrong recipient, missing a recipient, attaching the wrong documents, or placing the wrong tabs. If the envelope has not been completed yet (or voided), you can still correct any mistake that was made. The correct operation enables you to make changes to an envelope after it was sent out, which is very useful because, as I just mentioned, we all make mistakes.
You can correct an envelope from the web app, or you can do this from your own API call using the DocuSign eSignature REST API. The first thing to consider is that the envelope must be yours, which means that you have access to correct it. The access token obtained to make API calls must be for a user that either was the sender of the envelope or was granted shared access to it. The second thing is to remember that, to be available for correction, the envelope can only be in specific states. It must be awaiting signatures (or actions) from recipients, which means it was already sent, but not yet completed and certainly not voided. If any of these conditions are not met, you’ll get an error from the API attempting to correct the envelope.
The code snippets here show you how to embed the correctView into your application. This is an embedded view similar to how you do embedded signing or embedded sending. The difference is that the envelope has already been sent. Maybe your application has a “correct” button and you want to enable your users to correct envelopes without leaving your app. This is how it’s done: you first lock the envelope, then generate a correct view and redirect your users to that view (DocuSign recommends not to put it in an iframe). You then redirect the user back to your application, where you have to unlock the envelope to complete the process.
Here are our usual six code snippets:
C#
var docuSignClient = new DocuSignClient(basePath);
// You will need to obtain an access token using your chosen authentication method
docuSignClient.Configuration.DefaultHeader.Add("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(docuSignClient);
// Lock the envelope so it can be corrected
var lockRequest = new LockRequest
{
LockedByApp = "C.A.T. App",
LockDurationInSeconds = "900",
LockType = "edit"
};
envelopesApi.CreateLock(accountId, envelopeId, lockRequest);
// Create correctView
var correctViewRequest = new CorrectViewRequest
{
ReturnUrl = "https://www.docusign.com",
BeginOnTagger = "true",
};
ViewUrl correctUrl = envelopesApi.CreateCorrectView(accountId, envelopeId, correctViewRequest);
string theUrl = correctUrl.Url;
Java
Configuration config = new Configuration(new ApiClient(basePath));
// You will need to obtain an access token using your chosen authentication method
config.addDefaultHeader("Authorization", "Bearer " + accessToken);
EnvelopesApi envelopesApi = new EnvelopesApi(apiClient);
// Lock the envelope so it can be corrected
LockRequest lockRequest = new LockRequest();
lockRequest.setLockedByApp( "C.A.T. App");
lockRequest.setLockDurationInSeconds("300");
lockRequest.setLockType("edit");
envelopesApi.createLock(accountId, envelopeId, lockRequest);
// Create correctView
CorrectViewRequest correctViewRequest = new CorrectViewRequest();
correctViewRequest.setReturnUrl("https://www.docusign.com");
correctViewRequest.setBeginOnTagger("true");
ViewUrl correctUrl = envelopesApi.CreateCorrectView(accountId, envelopeId, correctViewRequest);
String theUrl = correctUrl.getUrl();
Node.js
let dsApiClient = new docusign.ApiClient();
dsApiClient.setBasePath(basePath);
// You will need to obtain an access token using your chosen authentication method
dsApiClient.addDefaultHeader('Authorization', 'Bearer ' + accessToken);
let envelopesApi = new docusign.EnvelopesApi(dsApiClient);
// Lock the envelope so it can be corrected
let lockRequest = new docusign.lockRequest.constructFromObject({
LockedByApp : 'C.A.T. App',
lockDurationInSeconds : '300',
lockType : 'edit' });
envelopesApi.createLock(accountId, envelopeId, lockRequest);
// Create correctView
let correctViewRequest = new docusign.correctViewRequest.constructFromObject({
returnUrl : 'https://www.docusign.com',
beginOnTagger: 'true'});
let correctUrl = envelopesApi.createCorrectView(accountId, envelopeId, correctViewRequest);
let theUrl = correctUrl.url();
PHP
$api_client = new \DocuSign\eSign\client\ApiClient($base_path);
$config = new \DocuSign\eSign\Model\Configuration($api_client);
# You will need to obtain an access token using your chosen authentication method
$config->addDefaultHeader('Authorization', 'Bearer ' + $access_token);
$envelopes_api = new \DocuSign\eSign\Api\EnvelopesApi($api_client);
# Lock the envelope so it can be corrected
$lock_request = new \DocuSign\eSign\Model\lockRequest();
$lock_request->setLockedByApp('C.A.T. App');
$lock_request->setlockDurationInSeconds('300');
$lock_request->setlockType('edit');
$envelopes_api->createLock($account_id, $envelope_id, $lock_request);
# Create correctView
$correct_view_request = new CorrectViewRequest();
$correct_view_request->setReturnUrl('https://www.docusign.com');
$correct_view_request->setBeginOnTagger('true');
$correct_url = $envelopes_api->createCorrectView($account_id, $envelope_id, $correct_view_request);
$the_url = $correct_url->getUrl();
Python
api_client = ApiClient()
# You will need to obtain an access token using your chosen authentication method
api_client.set_default_header('Authorization', 'Bearer ' + access_token)
envelopes_api = EnvelopesApi(api_client)
# Lock the envelope so it can be corrected
lock_request = LockRequest()
lock_request.locked_by_app = 'C.A.T. App'
lock_request.lock_duration_in_seconds = '300'
lock_request.lock_type = 'edit'
envelopes_api.create_lock(account_id, envelope_id, lock_request)
# Create correctView
correct_view_request = CorrectViewRequest()
correct_view_request.retun_url = 'https://www.docusign.com'
correct_view_request.begin_on_tagger = 'true'
correct_url = envelopes_api.create_correct_view(account_id, envelope_id, correct_view_request)
the_url = correct_url.url()
Ruby
config = DocuSign_eSign::Configuration.new
config.host = base_path
api_client = DocuSign_eSign::ApiClient.new config
# You will need to obtain an access token using your chosen authentication method
api_client.DefaultHeader['Authorization'] = 'Bearer ' + access_token
envelopes_api = DocuSign_eSign::EnvelopesApi.new api_client
# Lock the envelope so it can be corrected
lock_request = DocuSign_eSign::LockRequest.new
lock_request.locked_by_app = 'C.A.T. App'
lock_request.lock_duration_in_seconds = '300'
lock_request.lock_type = 'edit'
envelopes_api.create_lock(account_id, envelope_id, lock_request)
# Create correctView
correct_view_request = DocuSign_eSign::CorrectViewRequest.new
correct_view_request.retun_url = 'https://www.docusign.com'
correct_view_request.begin_on_tagger = 'true'
correct_url = envelopes_api.create_correct_view(account_id, envelope_id, correct_view_request)
the_url = correct_url.url()
That’s all, folks! I hope you found it useful. If you have any questions, comments, or suggestions for topics for future Common API Tasks posts, feel free to email me. Until next time...